C++ numeric limits define the minimum and maximum values that can be stored in various data types, providing a way to understand the constraints of your variables.
Here's a code snippet demonstrating how to use the `std::numeric_limits` class:
#include <iostream>
#include <limits>
int main() {
std::cout << "int: " << std::numeric_limits<int>::min() << " to "
<< std::numeric_limits<int>::max() << std::endl;
std::cout << "float: " << std::numeric_limits<float>::min() << " to "
<< std::numeric_limits<float>::max() << std::endl;
return 0;
}
What Are Numeric Limits in C++?
C++ numeric limits refer to the minimum and maximum values representable by different numeric types within the C++ programming language. Understanding these limits is essential for developers, as they directly influence data safety and integrity in applications, particularly when arithmetic operations may lead to conditions such as overflow or underflow.
Numeric limits play a significant role in various applications, especially in fields like finance, scientific computing, and graphics, where precision and data fidelity are paramount.
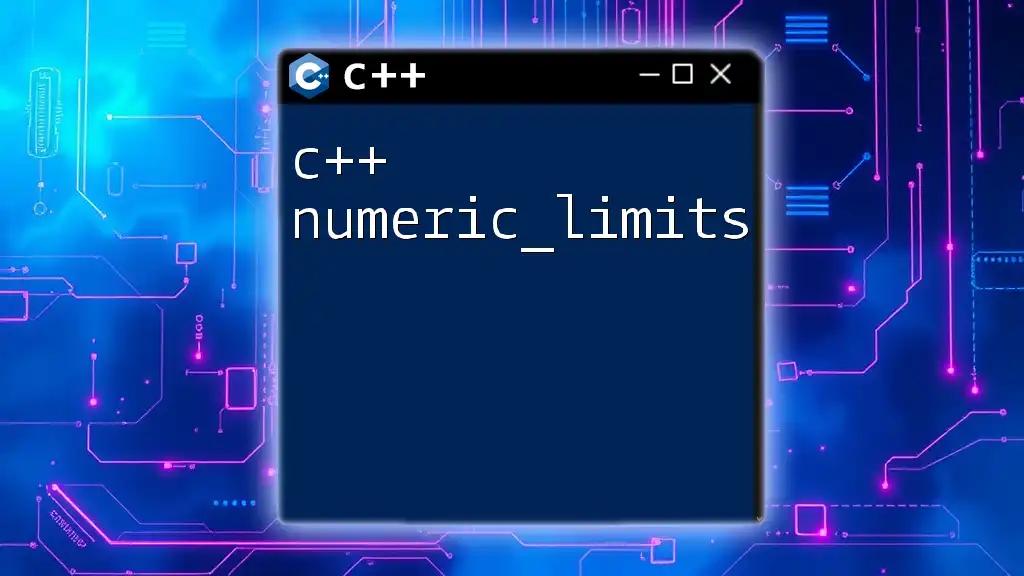
Overview of Data Types in C++
Fundamental Data Types
In C++, the default numeric data types include:
- Integer Types: These types store whole numbers, such as `int`, `short`, and `long`.
- Floating-Point Types: These represent real numbers and include `float`, `double`, and `long double`.
- Character Types: Though primarily for text, they are often linked to numeric limits in terms of ASCII values.
Each data type has its size and range, which can influence your choice based on the application requirements.
Understanding Data Type Sizes
Data types have specific sizes, which determines how much memory they occupy and what range of values they can hold. For example, an `int` might typically occupy 4 bytes of memory, allowing it to represent values from -(2^31) to (2^31 - 1), whereas a `float` occupies 4 bytes but holds a different range due to its floating-point representation.

C++ `<limits>` Header
Introduction to the `<limits>` Library
The `<limits>` header in C++ provides a standardized way to access numeric limits for all built-in data types. This functionality helps developers dynamically retrieve maximum and minimum values without hardcoding them.
Accessing Numeric Limits
You can access these limits by using the `std::numeric_limits` template class, which allows you to get limits for all numeric types simply and elegantly.
An example of its usage is shown below:
#include <limits>
#include <iostream>
int main() {
std::cout << "The maximum value for int: " << std::numeric_limits<int>::max() << std::endl;
std::cout << "The minimum value for int: " << std::numeric_limits<int>::min() << std::endl;
return 0;
}
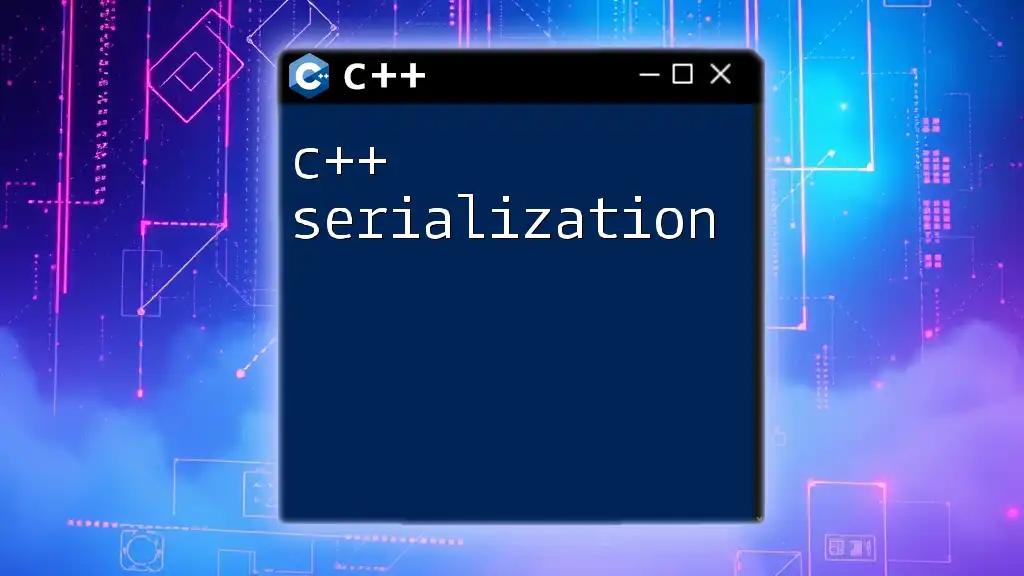
Numeric Limits for Integral Types
Understanding Limits for Integral Data Types
Integral types store discrete values and include types like `int`, `short`, `long`, and `char`. Each of these types has specific numeric limits determined by system architecture and bit representation.
Code Examples
Using `std::numeric_limits`, an illustrative snippet can show how you can check the limits of integral types, as shown in the following code:
#include <iostream>
#include <limits>
int main() {
std::cout << "Minimum int: " << std::numeric_limits<int>::min() << std::endl;
std::cout << "Maximum int: " << std::numeric_limits<int>::max() << std::endl;
std::cout << "Minimum short: " << std::numeric_limits<short>::min() << std::endl;
std::cout << "Maximum long: " << std::numeric_limits<long>::max() << std::endl;
return 0;
}
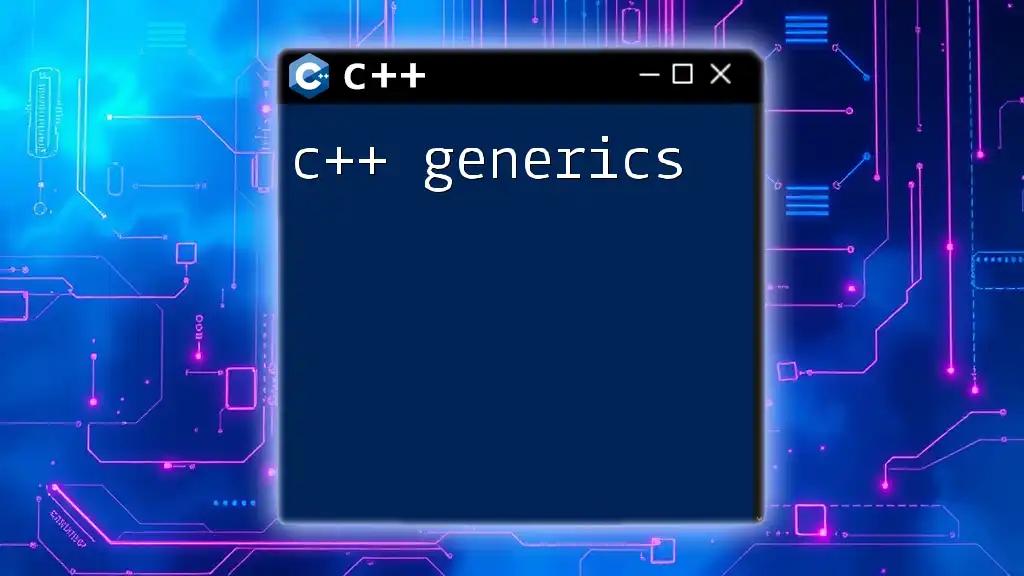
Numeric Limits for Floating-Point Types
Limits for Floating-Point Data Types
Floating-point types such as `float`, `double`, and `long double` are designed to handle real numbers and provide a wider range than integral types but at the cost of precision due to binary representation. This can lead to scenarios where very small or very large numbers are approximated.
Precision and Representation
The key difference between these types revolves around their precision and the range of values they can represent. For example, a `float` provides about 7 digits of precision, whereas a `double` enhances that to about 15 digits, allowing for more accurate representation of significant figures in calculations.
Code Examples
Here's how to access the numeric limits of floating-point types:
#include <iostream>
#include <limits>
int main() {
std::cout << "Minimum float: " << std::numeric_limits<float>::min() << std::endl;
std::cout << "Maximum double: " << std::numeric_limits<double>::max() << std::endl;
return 0;
}

Special Values
Exploring Special Values in `<limits>`
The `<limits>` header goes further than just providing maximum and minimum values; it also lets you access special values like positive and negative infinity and NaN (Not a Number). These elements help manage exceptional arithmetic scenarios.
Code Examples
You can check for these special values in the following manner:
#include <iostream>
#include <limits>
int main() {
if (std::numeric_limits<float>::has_infinity) {
std::cout << "Float infinity available!" << std::endl;
}
if (std::numeric_limits<float>::has_quiet_NaN) {
std::cout << "Float NaN available!" << std::endl;
}
return 0;
}
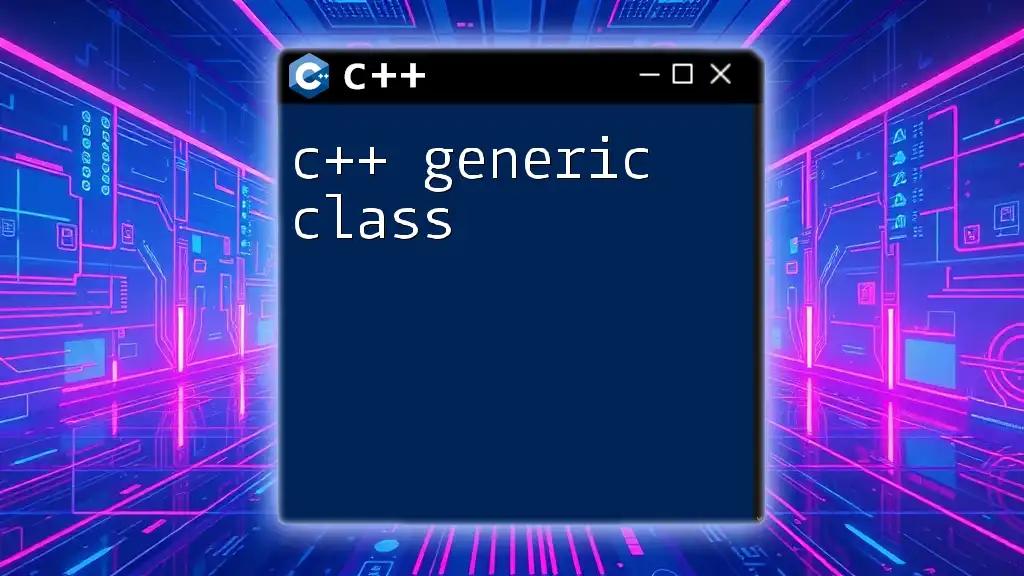
Applications of Numeric Limits
Practical Use Cases
Understanding and leveraging numeric limits is crucial in various domains. For instance, financial applications often require precise decimal representation to avoid rounding errors, while scientific computations need to handle very small or very large numbers without loss in accuracy.
Avoiding Errors in Calculations
Employing numeric limits proactively helps prevent errors in calculations. For example, before performing arithmetic operations, checking whether the result would exceed defined limits can protect against unintended behavior in algorithms.

Common Pitfalls
Overflows/Underflows in C++
A common challenge developers face with numeric limits includes overflow and underflow scenarios. Overflow occurs when a calculation exceeds the maximum limit of a numeric type, while underflow happens when a number becomes too small for representation.
Best Practices to Handle Limits
To combat these issues, developers should adopt best practices such as:
- Validating Inputs: Always validate inputs against the limits of the data type before performing calculations.
- Using Appropriate Types: Select data types that are appropriate for the range of values you expect.

Conclusion
Understanding C++ numeric limits is integral for any developer working with data types. It not only helps in preserving data integrity but also ensures your applications run without unexpected errors.
Being aware of these limits and properly utilizing the `<limits>` header can greatly improve the quality of your code and prevent common pitfalls. Take this knowledge and explore further as you dive deeper into C++ programming.
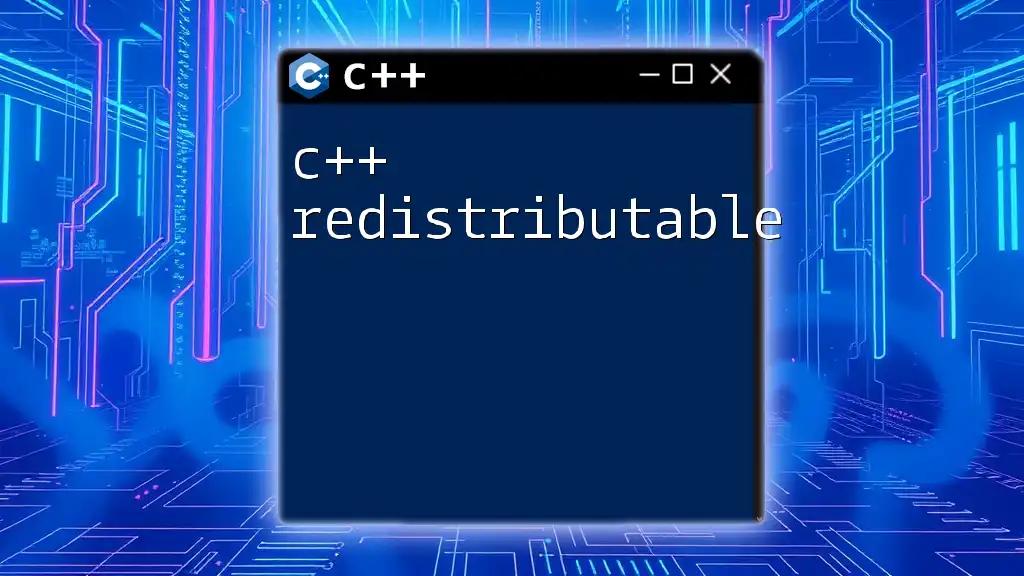
Further Reading
For a more in-depth understanding, refer to the C++ documentation, tutorials, or courses focusing on data representation and safety in programming.
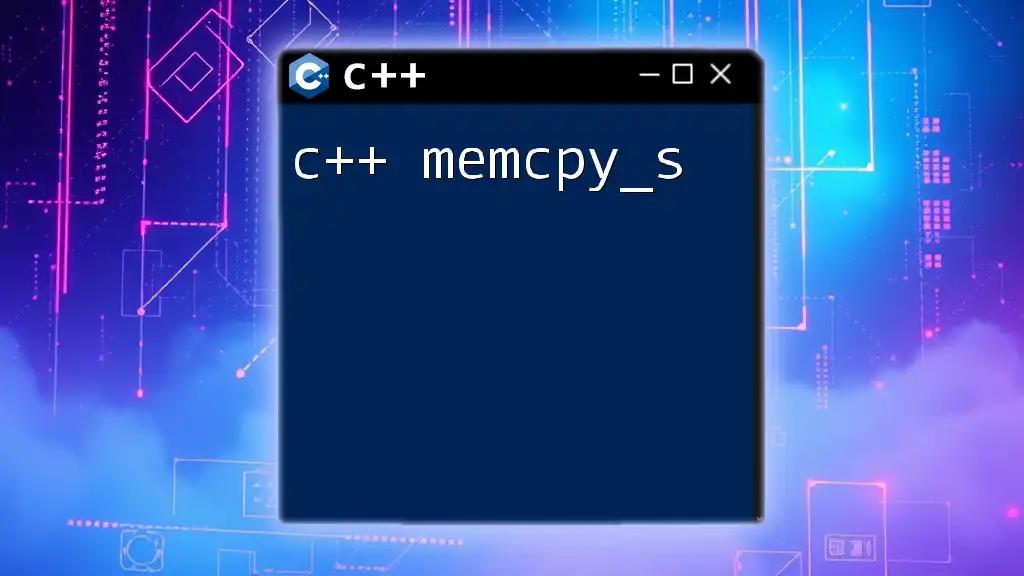
Call to Action
Join our learning community to further explore C++ programming and elevate your skills through interactive lessons and resources!