The `std::isnumeric` function in C++ checks if a character is a numeric digit (0-9) and can be used in conjunction with standard algorithms to filter out non-numeric characters from a string.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
#include <cctype>
#include <string>
int main() {
std::string str = "C++ 123";
for (char ch : str) {
if (std::isdigit(ch)) {
std::cout << ch << " is a numeric digit." << std::endl;
}
}
return 0;
}
What is isnumeric?
The `isnumeric` function in C++ is a utility that checks whether a given string represents a numeric value. This functionality is essential in various applications, especially for validating user inputs and ensuring that data being processed adheres to expected formats.
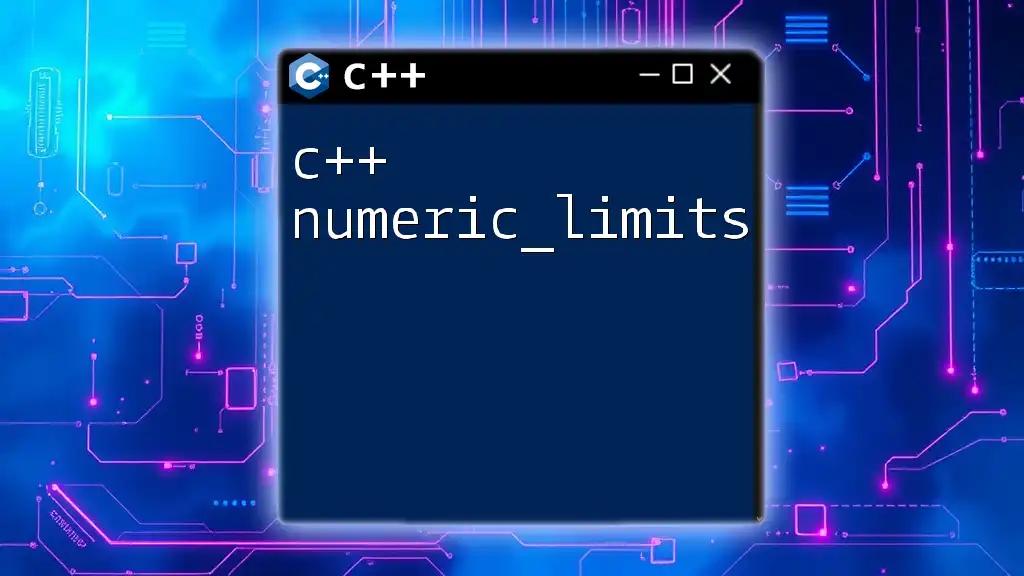
Importance of isnumeric in C++
Recognizing numeric values accurately is a critical task in software development. This capability is utilized in form validations, data parsing, and error handling. Implementing `isnumeric` effectively ensures that only valid numerical data is processed, which helps maintain data integrity and prevents runtime errors.

Understanding isnumeric in C++
Definition and Syntax
The `isnumeric` function is defined in the C++ standard library and is used to ascertain whether the characters in a string are all digits. The basic syntax for using `isnumeric` is as follows:
bool isnumeric(const char* str);
This function evaluates each character in the string and returns `true` if every character is numeric; otherwise, it returns `false`.
Header Files and Library Requirements
To use the `isnumeric` function, you must include the appropriate header. Most often, this will be:
#include <cctype>
The `cctype` library contains functions for character classification, including the `isdigit()` function, which is commonly leveraged in custom implementations of `isnumeric`. Understanding how `isnumeric` fits into the larger framework of C++ can help you use it more effectively.
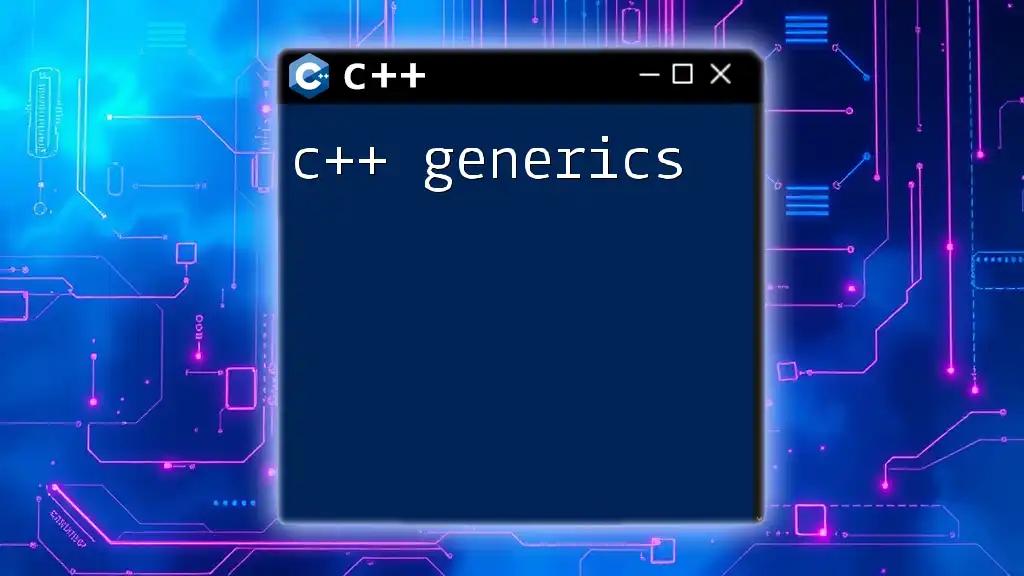
Practical Examples of isnumeric
Basic Example of isnumeric
Here’s a straightforward implementation that checks if a string contains only numeric characters.
#include <cctype>
#include <iostream>
using namespace std;
bool isNumeric(const string &str) {
for (char c : str) {
if (!isdigit(c)) {
return false;
}
}
return true;
}
int main() {
cout << isNumeric("12345") << endl; // Output: 1 (true)
cout << isNumeric("abc123") << endl; // Output: 0 (false)
}
In this example, the `isNumeric` function iterates through each character in the string and employs `isdigit` to determine if the character is a digit. The results are straightforward: it outputs `1` for valid numeric strings and `0` for invalid ones.
Advanced Usage of isnumeric
Building upon the basic example, we can utilize C++ STL functionalities for a more concise implementation. Below is an advanced version leveraging `all_of`:
#include <cctype>
#include <algorithm>
#include <iostream>
using namespace std;
bool isNumeric(const string &str) {
return !str.empty() && all_of(str.begin(), str.end(), ::isdigit);
}
int main() {
string input;
cout << "Please enter a number: ";
cin >> input;
if(isNumeric(input)) {
cout << "Input is a numeric value!" << endl;
} else {
cout << "Input is not numeric!" << endl;
}
}
This snippet uses `all_of` to check if all characters in the input string are digits. Additionally, it incorporates a safety check for empty strings. Such an implementation can enhance the user experience by promptly validating input.
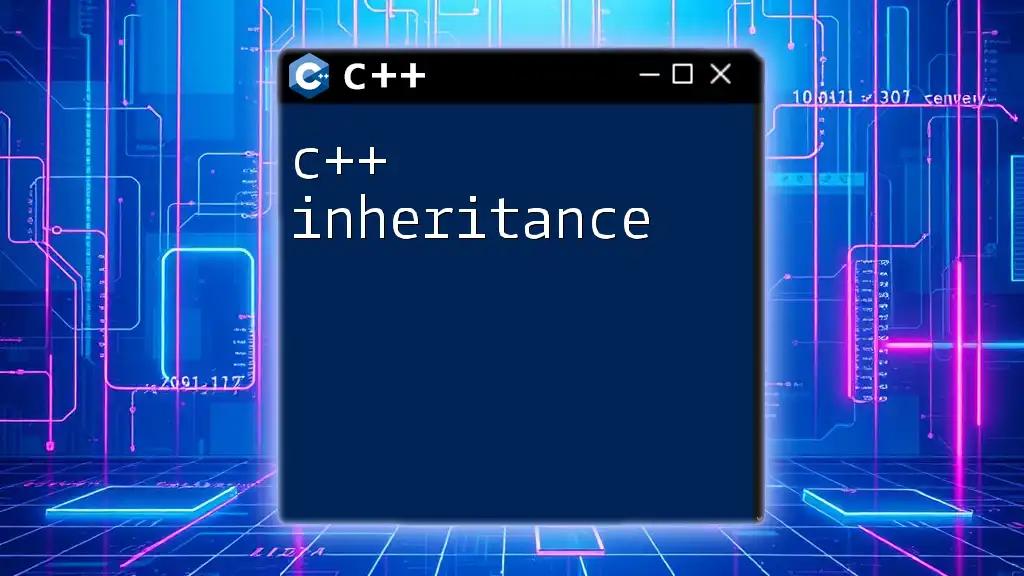
Common Pitfalls When Using isnumeric
Handling Edge Cases
While using `isnumeric`, it is vital to consider edge cases:
-
Negative Numbers: If a string such as `-123` is valid in your context, the current `isNumeric` implementation would deem it invalid because of the `-` character.
-
Decimal Points: Strings like `123.45` also get flagged as invalid due to the presence of the decimal point.
To handle these edge cases, you may need to expand your logic to account for characters `-`, `+`, and `.`.
Performance Considerations
When dealing with large datasets, it's crucial to consider the efficiency of your `isnumeric` implementation. The simple iterative approach may work for small strings but could slow down performance as input size and complexity grow. Using functions like `all_of` may offer slight performance improvements.
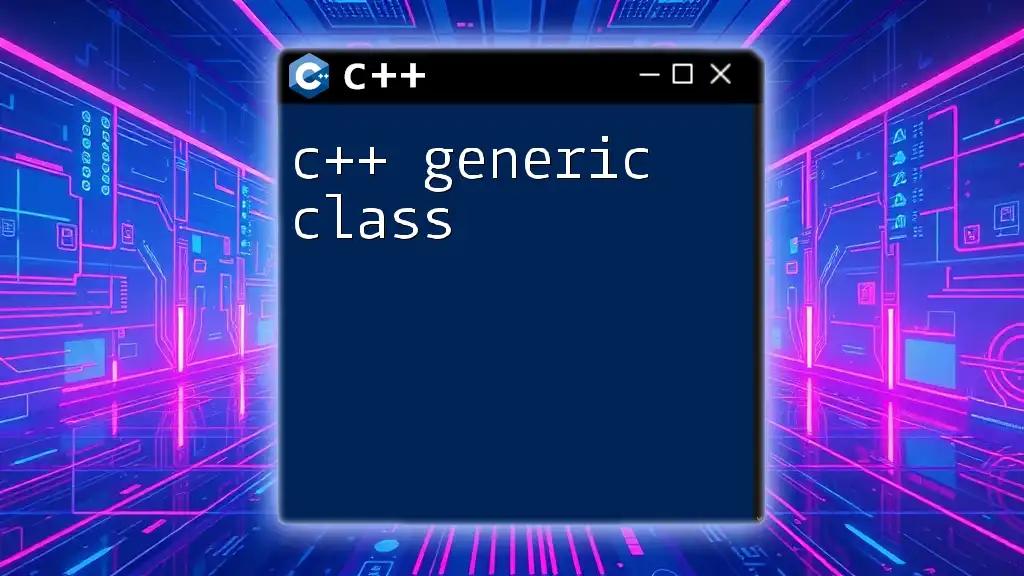
Comparison of isnumeric and isnumber in C++
Defining isnumber
The function `isnumber` in C++ provides similar functionality; however, it could potentially belong to libraries such as `<locale>`. While not part of the C++ standard library, it serves to check the encapsulating locale.
Differences and Similarities
When considering which function to use, here are a few distinctions:
-
Return Types: `isnumeric` primarily operates at a character level, whereas `isnumber` functions may evaluate the entire context depending on locale settings.
-
Use Cases: If you require strict numeric validation without contextual nuances, `isnumeric` may be preferable. Conversely, if you need locale-sensitive checks, `isnumber` might be the better option.

Real-life Applications of isnumeric
Validation in User Inputs
Using `isnumeric` is paramount in web forms, command-line interfaces, or any user-interactive platforms. By validating inputs before processing them, developers can prevent errors and simplify user error handling.
Data Processing and Analytics
In the world of data analytics, ensuring that the data being processed is numeric before performing any calculations is essential. Suppose the dataset contains irrelevant characters; using `isnumeric` can help filter through invalid entries and ensure only reliable data is analyzed.

Conclusion
The `isnumeric` function in C++ serves as a vital tool for handling character validation effectively. By understanding its usage, syntax, and nuances, developer can leverage it for robust input validation and data processing tasks. You are encouraged to practice with `isnumeric` through additional coding exercises and projects to enhance your understanding.

Additional Resources
For further learning, consider exploring books like "The C++ Programming Language" by Bjarne Stroustrup and seeking online courses that dive deeper into C++ programming. Online forums like Stack Overflow or dedicated C++ communities can also provide valuable insights and assistance.