C++ is derived from the C programming language, incorporating object-oriented features and allowing for more complex software development.
Here’s a simple example of a C++ program that demonstrates basic syntax:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
The Evolution of Programming Languages
The journey of programming languages begins with the need to communicate with machines. Early languages, such as Assembly and Fortran, laid the groundwork for higher-level abstractions, emphasizing efficiency and performance. They allowed programmers to express algorithms in a more human-readable format while still being closely tied to the hardware.
With the advent of Object-Oriented Programming (OOP) in the 1980s, the landscape shifted dramatically. OOP introduced concepts such as encapsulation, inheritance, and polymorphism, facilitating more modular and maintainable code. This paradigm would eventually become pivotal in the development of C++.
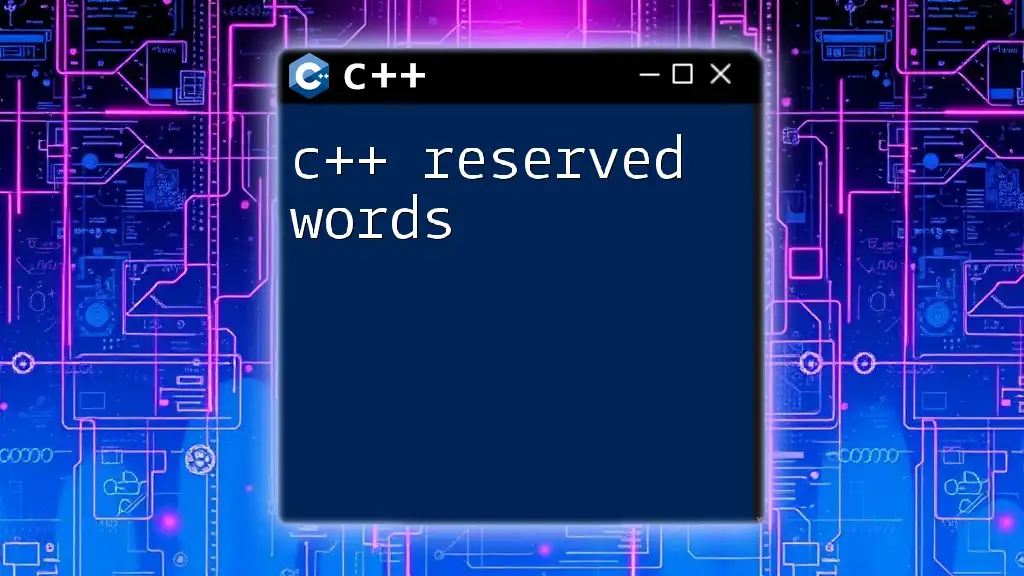
The Legacy of Bjarne Stroustrup
Who is Bjarne Stroustrup?
Bjarne Stroustrup, a Danish computer scientist, is the creator of C++. His background in both engineering and programming uniquely positioned him to recognize the limitations of existing languages. Stroustrup aimed to create a language that combined the performance of C with the higher level of abstraction found in Simula, the first object-oriented programming language.
The Year of Creation
C++ was born in the early 1980s at Bell Labs, where Stroustrup sought to improve C to better support the object-oriented paradigm. The design of C++ enables modularity and code reuse, which are essential for large-scale software development. Understanding that C could serve as a strong foundation, Stroustrup built upon it, ultimately leading to the release of C++ in 1985.
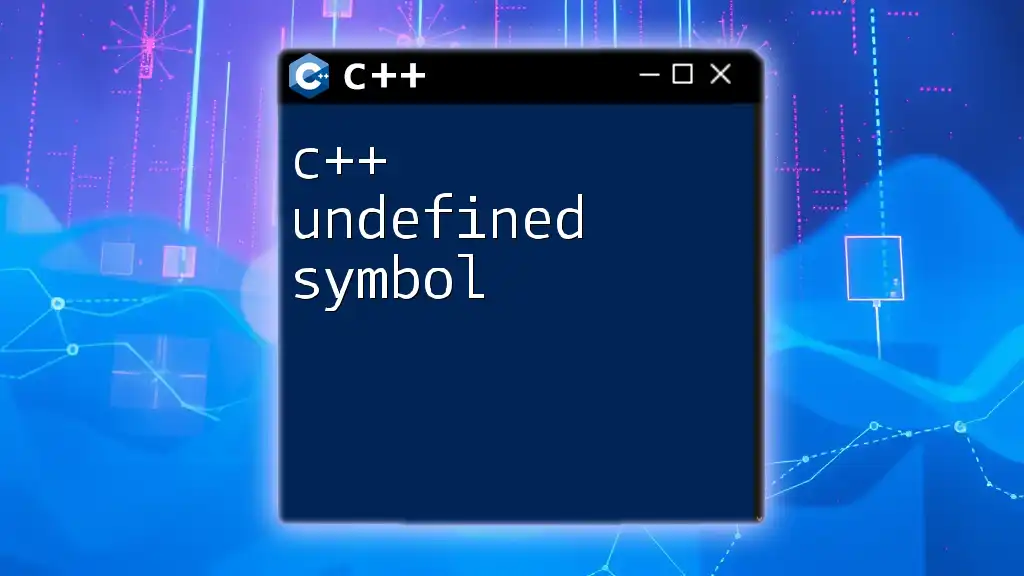
C: The Backbone of C++
Overview of the C Programming Language
To truly appreciate C++, one must first understand its predecessor, C. Created in the early 1970s, C offered lower-level access to memory, efficient performance, and powerful constructs for manufacturing operating systems and applications. Its syntax is straightforward but highly expressive, making it efficient for programming tasks.
How C Influences C++
C++ retains the core syntax and features of C while expanding upon them. This dual nature means that many C programs can be compiled with a C++ compiler with little to no modification. However, C++ introduces significant enhancements, especially in the realm of object-oriented capabilities.
Consider the following code snippet that contrasts a fundamental C program with its C++ counterpart:
// C code
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
// C++ code
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Here, you can see that C++ introduces a more modern way of outputting data with `std::cout`, which simplifies string handling and is more extensible.
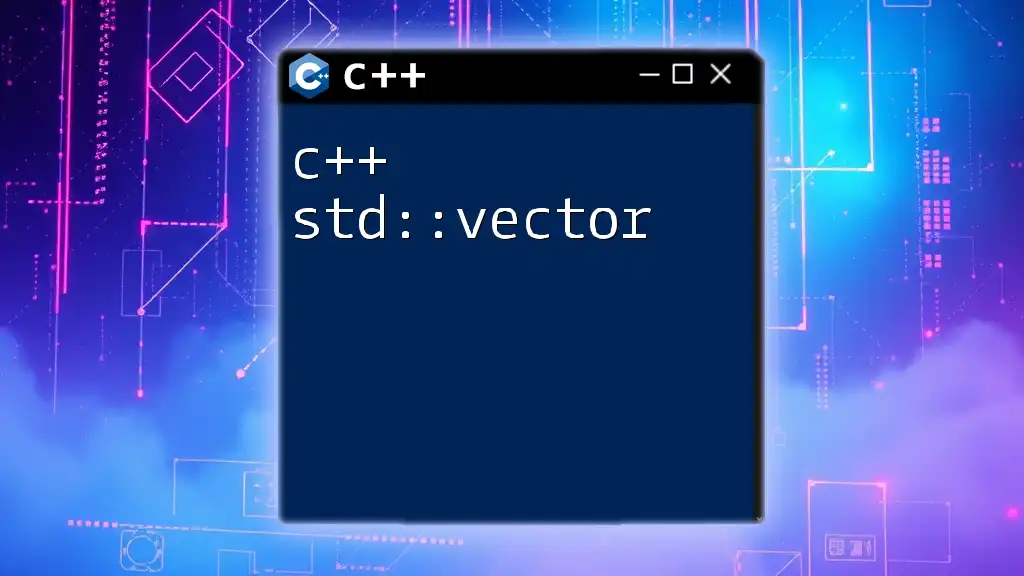
C++ and Object-Oriented Features
Introduction to Object-Oriented Concepts
C++ stands out primarily due to its incorporation of OOP principles. Fundamental OOP concepts include:
- Encapsulation: Combining data and the functions that operate on that data into a single unit, often called a class.
- Inheritance: Allowing one class to inherit properties and behaviors from another, promoting code reuse.
- Polymorphism: Enabling objects to be treated as instances of their parent class, providing flexibility in interfaces.
Examples of OOP in C++
These principles facilitate the creation of robust and scalable systems. For example, consider a simple representation of animals using classes:
class Animal {
public:
virtual void makeSound() { std::cout << "Animal sound"; }
};
class Dog : public Animal {
public:
void makeSound() override { std::cout << "Bark"; }
};
In this example, `Animal` is a base class, and `Dog` inherits from `Animal`. The `makeSound` method is overridden to provide specific functionality for dogs, demonstrating polymorphism.
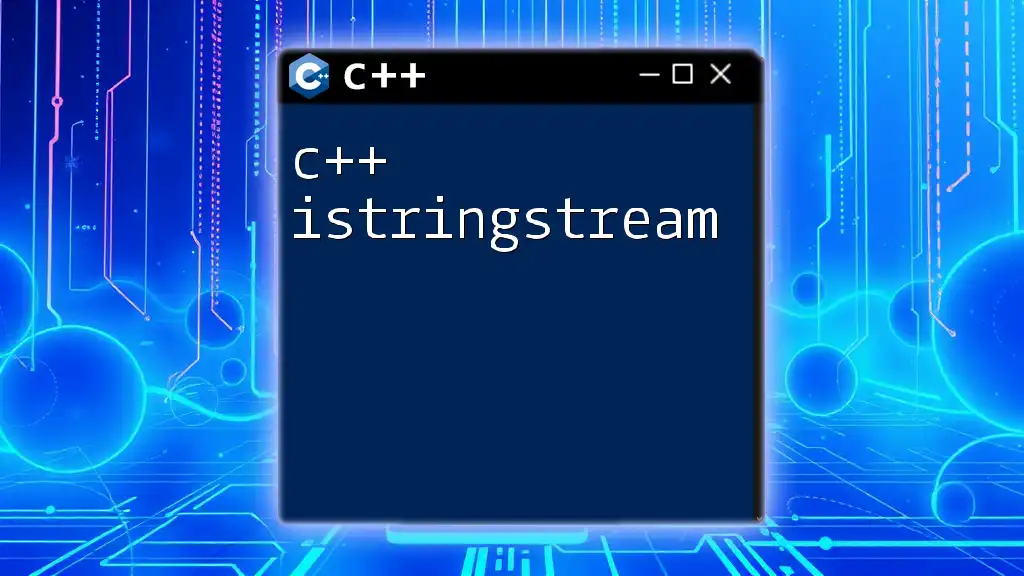
C++: A Combination of High-Level and Low-Level Language Features
High-Level Features
C++ is equipped with a rich set of high-level features that make programming more intuitive. One significant advantage is its Standard Template Library (STL), which offers a collection of algorithms and data structures, promoting code efficiency and clarity. Exception handling provides a way to manage run-time errors robustly.
An example of using the STL with vectors is as follows:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Here, `std::vector` allows dynamic array management, demonstrating C++’s adaptability and ease of use.
Low-Level Features
At the same time, C++ retains low-level capabilities such as manual memory management and bit manipulation. Direct access to memory through pointers allows for performance optimization that high-level languages often abstract away. This dual nature empowers programmers to choose the level of abstraction that best suits their needs.
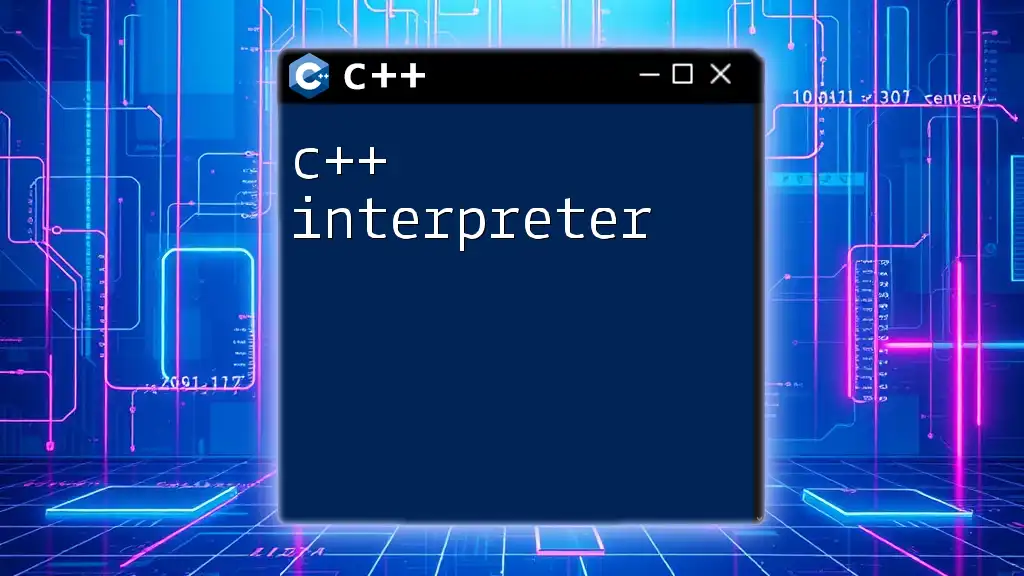
Other Influences on C++
The Role of Other Languages
C++ was influenced by various programming languages that preceded it, notably Simula, which introduced OOP concepts, and Ada, known for strong type checking. These influences helped shape C++’s structure and philosophy.
Comparison with Other Languages
When comparing C++ with modern languages like Java and Python, we see distinct philosophies:
- C++: Blends procedural and object-oriented programming with low-level access to memory; highly performant.
- Java: Aimed at portability and abstraction, removing direct memory management; inherently slower but easier to learn.
- Python: Prioritizes readability and simplicity, sacrificing some performance and control.
Each language has its strengths and weaknesses, and the choice often depends on the specific application requirements.
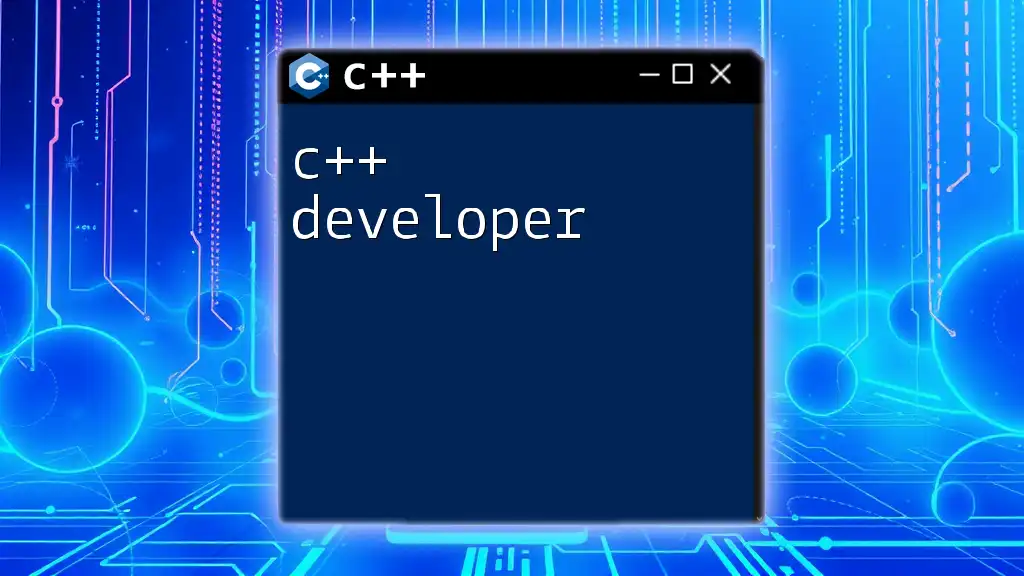
The Future of C++
Current Developments
C++ continues to evolve, with recent standards like C++20 introducing new features such as concepts and coroutines, which offer greater expressiveness and efficiency. The community actively develops the language, ensuring that it remains relevant in a rapidly changing technological landscape.
C++ in Modern Applications
C++ remains indispensable in various fields, including gaming, finance, and high-performance computing. Its speed and efficiency make it a preferred choice for tasks demanding heavy computation, such as graphics rendering and large-scale simulations.
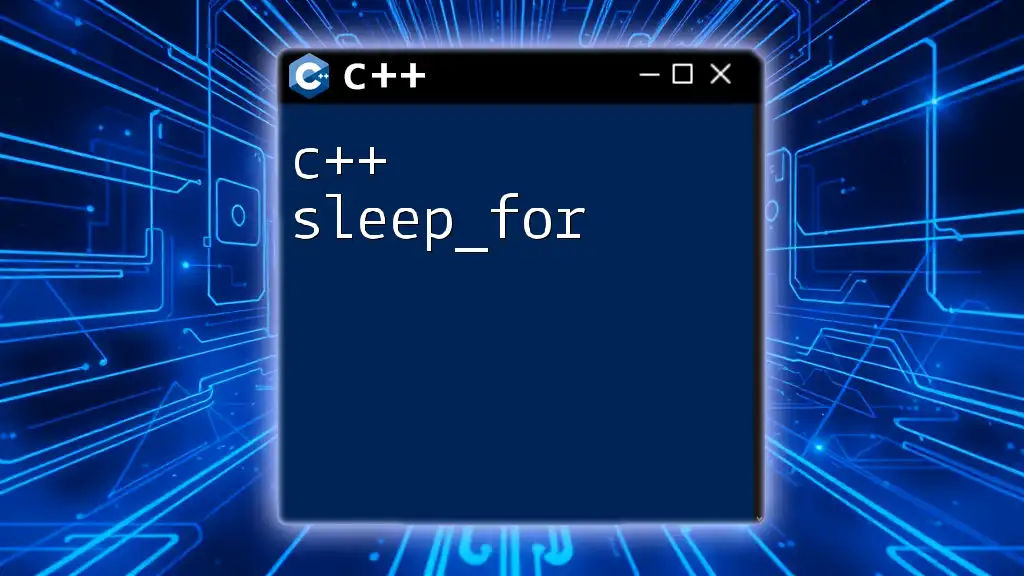
Conclusion
Understanding where C++ originates from enhances our appreciation for its design and execution. C++ is derived from C and reflects a merger of object-oriented principles with low-level performance capabilities. This powerful combination enables developers to write efficient, maintainable, and modular code, making C++ a cornerstone in the programming community.
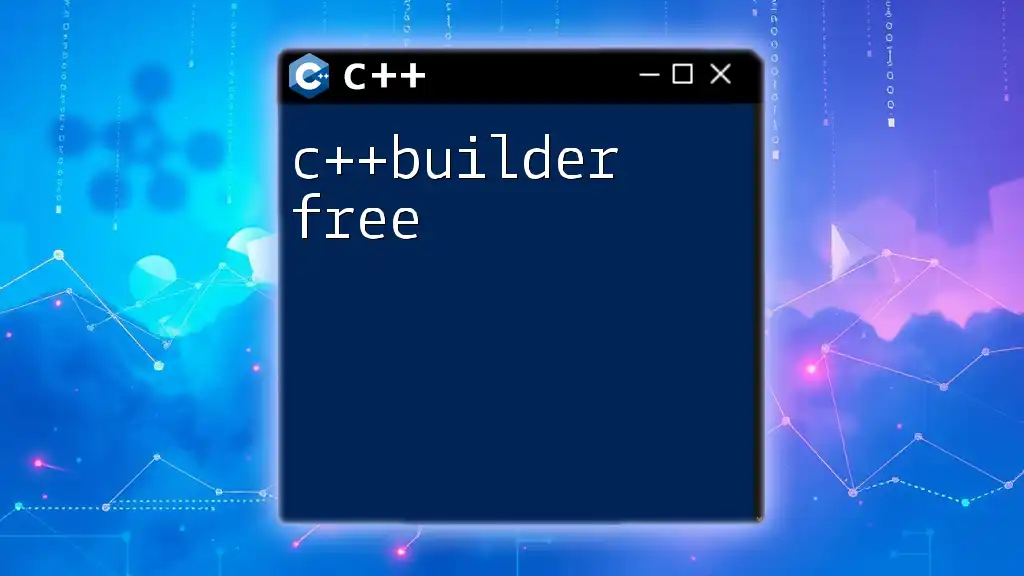
Call to Action
For those eager to explore the world of C++, join our courses and start learning the essential commands and practices today. Dive deeper into C++ programming with our resources and transform your coding skills!