A derived class in C++ is a class that inherits properties and behaviors (member variables and functions) from another class, known as the base class, enabling code reusability and the creation of hierarchies.
Here's a code snippet illustrating this concept:
class Base {
public:
void display() {
std::cout << "Base class display function." << std::endl;
}
};
class Derived : public Base {
public:
void show() {
std::cout << "Derived class show function." << std::endl;
}
};
int main() {
Derived obj;
obj.display(); // Call function from the base class
obj.show(); // Call function from the derived class
return 0;
}
What is a Derived Class in C++?
A derived class is a class that is based on another class, referred to as the base class. The main purpose of a derived class is to extend the functionality of the base class by inheriting its properties and methods. This is a fundamental aspect of inheritance in object-oriented programming (OOP), allowing you to create a hierarchy of classes that share code and behaviors.
Key Terminology:
- Base Class: The class from which properties and methods are inherited.
- Derived Class: The class that inherits from the base class.
- Inheritance: The mechanism through which one class acquires the properties of another.
- Access Specifiers: Keywords that determine the accessibility of classes and their members (e.g., public, protected, private).
Why Use Derived Classes?
Using derived classes comes with several significant benefits:
- Code Reuse: By inheriting methods and properties from a base class, you reduce code duplication, making your code base cleaner and easier to maintain.
- Polymorphism: Derived classes enhance the flexibility of your programs. Through polymorphism, a derived class can be treated as its base class, allowing for dynamic and varied behaviors at runtime.
- Extension of Existing Functionality: You can add or modify the functionality of a base class without altering its code, which is particularly useful for large projects or frameworks.
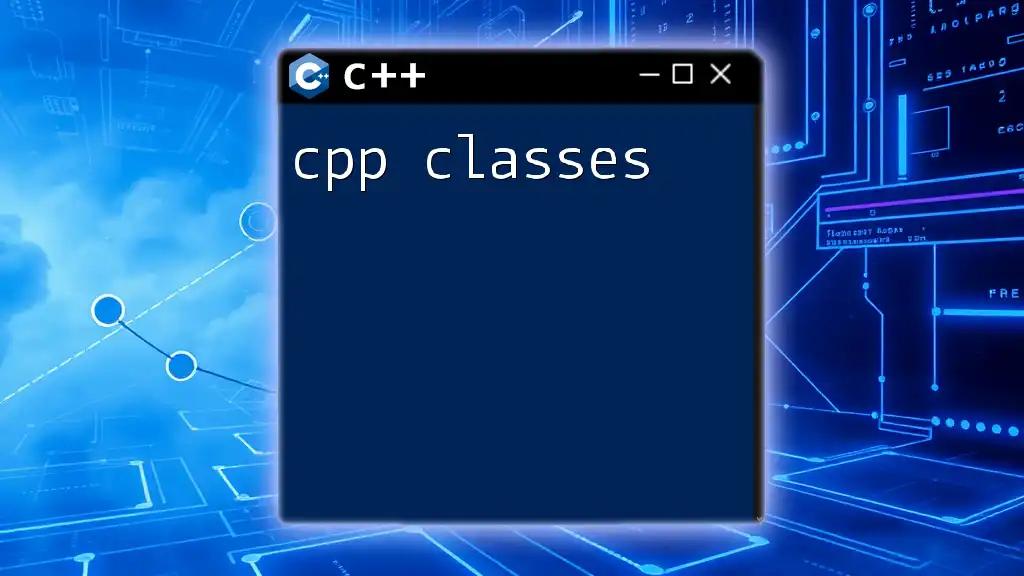
Creating a Derived Class in C++
Syntax for Declaring a Derived Class
Creating a derived class involves using a specific syntax to define the relationship between the base and derived classes. Here’s the basic syntax:
class BaseClass {
public:
void baseFunction() {
// Base class function
}
};
class DerivedClass : public BaseClass {
public:
void derivedFunction() {
// Derived class function
}
};
In this example, `DerivedClass` inherits from `BaseClass` using public inheritance, meaning that all public members of `BaseClass` will be accessible from `DerivedClass`.
Types of Inheritance
C++ supports various types of inheritance, which influence how base class members are accessed in derived classes.
Public Inheritance
In public inheritance, the public and protected members of the base class remain public and protected in the derived class.
Example:
class Base {
public:
void show() { std::cout << "Base Class" << std::endl; }
};
class Derived : public Base {
public:
void display() { std::cout << "Derived Class" << std::endl; }
};
Here, `display()` in `Derived` can access `show()` directly, as it is public.
Protected Inheritance
In protected inheritance, the public and protected members of the base class become protected members in the derived class. This means they cannot be accessed from the outside world, only by derived classes.
Code Example:
class Base {
protected:
int protectedValue;
};
class Derived : protected Base {
public:
void setValue(int val) { protectedValue = val; }
};
In this example, `setValue()` can modify `protectedValue`, but no external code can access it directly.
Private Inheritance
In private inheritance, both public and protected members of the base class become private members in the derived class, limiting access.
Example:
class Base {
public:
void show() { std::cout << "Base Class" << std::endl; }
};
class Derived : private Base {
public:
void display() { Base::show(); } // Accessing base class method
};
Here, `display()` can utilize `show()`, but `show()` is not accessible outside `Derived`.

Member Functions in Derived Classes
Overriding Member Functions
Derived classes can override base class member functions, allowing for more specific implementations. This feature is central to polymorphism in C++.
Code Snippet:
class Base {
public:
virtual void display() { std::cout << "Base Display" << std::endl; }
};
class Derived : public Base {
public:
void display() override { std::cout << "Derived Display" << std::endl; }
};
In this code, `Derived` provides its own implementation of `display()`. The `override` keyword ensures that you are in fact overriding a base class method, preventing potential mistakes.
Accessing Base Class Methods
You can still access base class methods from a derived class, even if they are overridden.
Example:
class Base {
public:
void baseFunction() { std::cout << "Base Function" << std::endl; }
};
class Derived : public Base {
public:
void callBaseFunction() {
baseFunction(); // Accessing base class method
}
};
This ensures you can utilize base class functionalities even when extending or modifying behaviors in derived classes.

Utilizing Constructors and Destructors in Derived Classes
Constructors in Derived Classes
Constructors in derived classes can call constructors of their base classes. This ensures that the base part of the object is initialized properly.
Code Snippet:
class Base {
public:
Base() { std::cout << "Base Constructor" << std::endl; }
};
class Derived : public Base {
public:
Derived() { std::cout << "Derived Constructor" << std::endl; }
};
The output will indicate the order of constructor calls. When `Derived` is instantiated, `Base` will be constructed first.
Destructors in Derived Classes
Destructors are also important in the derived classes, particularly to manage resources effectively. When a derived object is destroyed, the destructor of the base class is automatically called after the derived class's destructor completes.
Example:
class Base {
public:
~Base() { std::cout << "Base Destructor" << std::endl; }
};
class Derived : public Base {
public:
~Derived() { std::cout << "Derived Destructor" << std::endl; }
};
This ensures proper cleanup of resources allocated in both the base and derived classes.
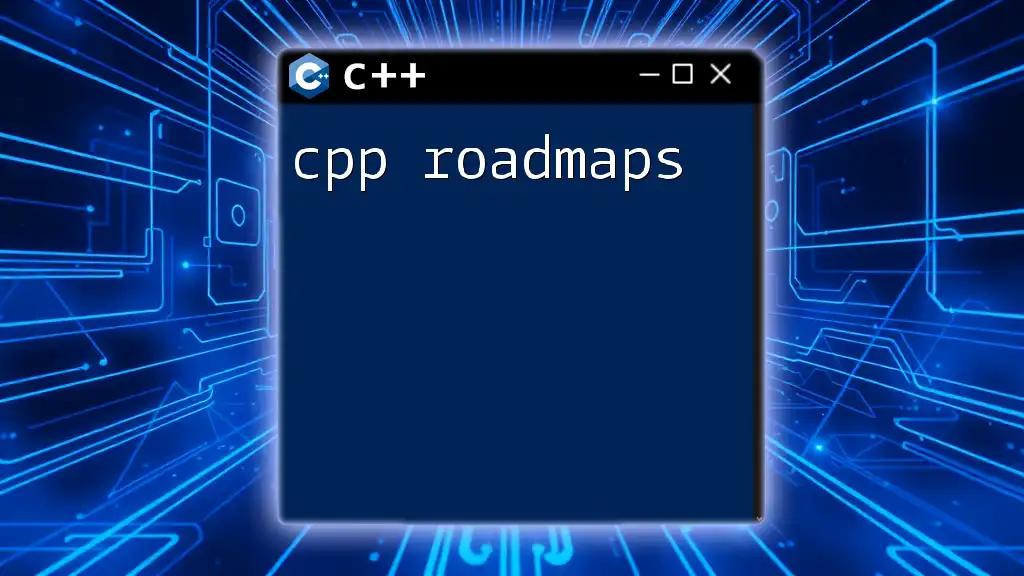
The Role of Access Specifiers in Derived Classes
Public, Protected, and Private Inherited Members
Understanding how access specifiers affect members in derived classes is crucial. Public and protected members from the base class will maintain their visibility based on the inheritance type, whereas private members will remain inaccessible.
Examples:
If a member is public in the base class and derived publicly, it remains public:
class Base {
public:
int publicValue;
};
class Derived : public Base {};
In `Derived`, `publicValue` is still public. Changing the inheritance to protected or private alters its accessibility.
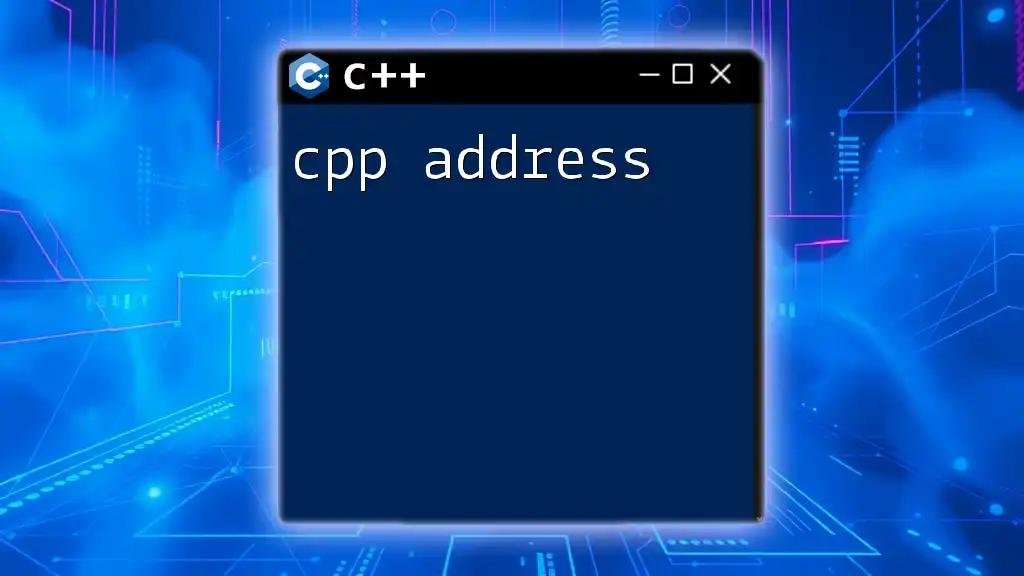
Virtual Classes and Abstract Base Classes
Understanding Virtual Functions
Virtual functions allow the derived class to provide a specific implementation that is called at runtime instead of compile time. This is crucial for achieving polymorphism.
Example:
class Base {
public:
virtual void show() = 0; // Pure virtual function
};
class Derived : public Base {
public:
void show() override { std::cout << "Derived implementation" << std::endl; }
};
In this case, `Base` declares a pure virtual function, making it an abstract class that cannot be instantiated directly. Only derived classes can provide implementations and thus become concrete types.
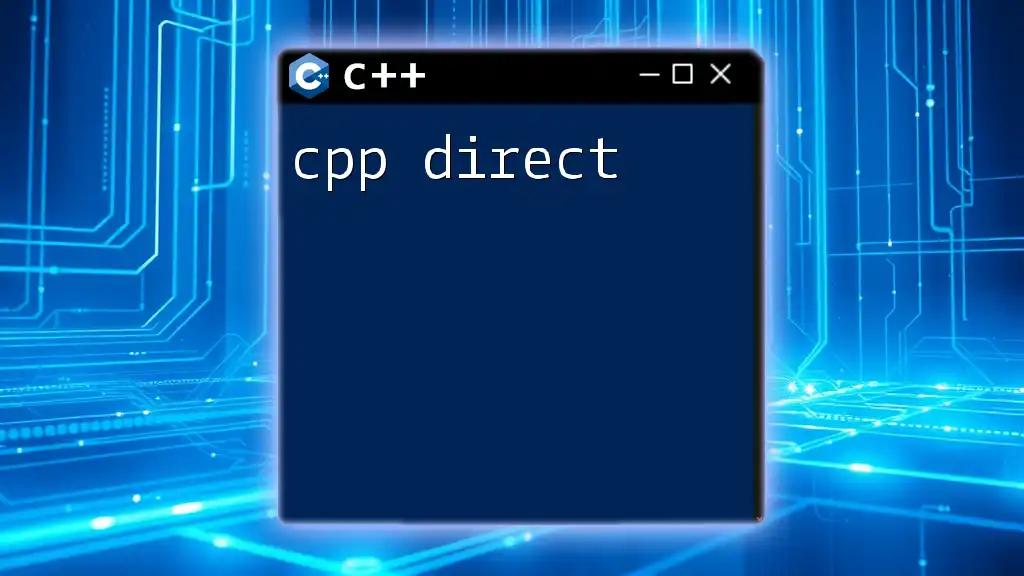
Use Cases and Best Practices for Derived Classes in C++
Real-World Examples of Derived Classes
Derived classes find applications in various domains, such as:
- In game development, where you might have a `Character` base class with `Player` and `Enemy` derived classes, allowing specific properties and methods relevant to each.
- In graphical applications, you could have a `Shape` base class with derived classes like `Circle`, `Square`, and `Triangle`, each implementing their specific draw method.
Best Practices
To effectively use derived classes in C++, consider the following best practices:
- Use meaningful names for your base and derived classes to convey their responsibilities.
- Favor composition over inheritance when appropriate, as it may lead to more flexible and maintainable designs without rigid class hierarchies.
- Keep the base classes focused and cohesive. They should represent a clear abstraction to reduce complexity in derived classes.
- Utilize virtual destructors in base classes when you intend to use polymorphism, ensuring proper resource cleanup.

Conclusion
Understanding the concept of cpp derived classes is essential for harnessing the full capabilities of object-oriented programming in C++. Derived classes enhance code reuse, enable polymorphism, and allow for the extension of functionalities, all of which contribute to cleaner and more maintainable code. By mastering these concepts, you can build more robust and efficient applications in C++.
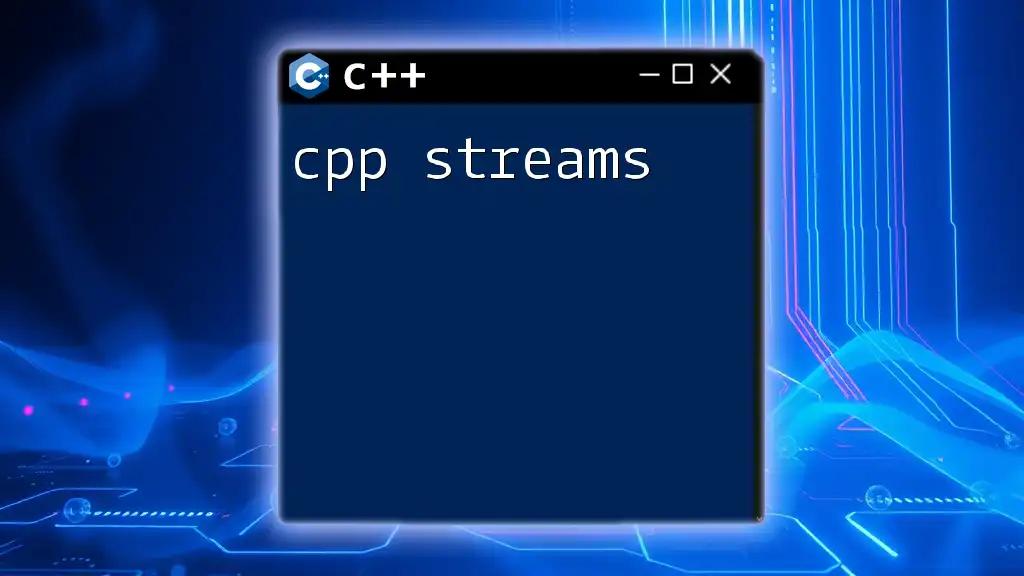
Additional Resources
To further your understanding, consider exploring recommended books, online courses, and community forums that focus on C++ and advanced programming concepts. Engaging with these resources will help solidify your knowledge and foster ongoing learning in the field of software development.