The `setprecision` function in C++ is used to control the number of significant digits displayed for floating-point values, allowing for more precise output formatting.
#include <iostream>
#include <iomanip>
int main() {
double value = 3.14159265359;
std::cout << std::fixed << std::setprecision(3) << value << std::endl; // Output: 3.142
return 0;
}
Understanding Precision in C++
What is Precision in C++?
Precision in programming refers to the accuracy with which numbers are represented and displayed. It is particularly important in C++ when dealing with floating-point numbers, as minor differences can lead to significant discrepancies in calculations. Having control over numerical output helps ensure that results are both legible and reliable, which is vital for tasks that require high degrees of accuracy, such as scientific calculations and financial applications.
The Role of the iostream Library
The `iostream` library is central to input and output operations in C++. It provides classes and functionalities for reading from and writing to standard input and output streams. Among its capabilities, it allows developers to manipulate how data is presented, which is where the `setprecision` command comes into play. By including `iomanip`, a header that defines various manipulators, you can manage numerical output formatting with ease.
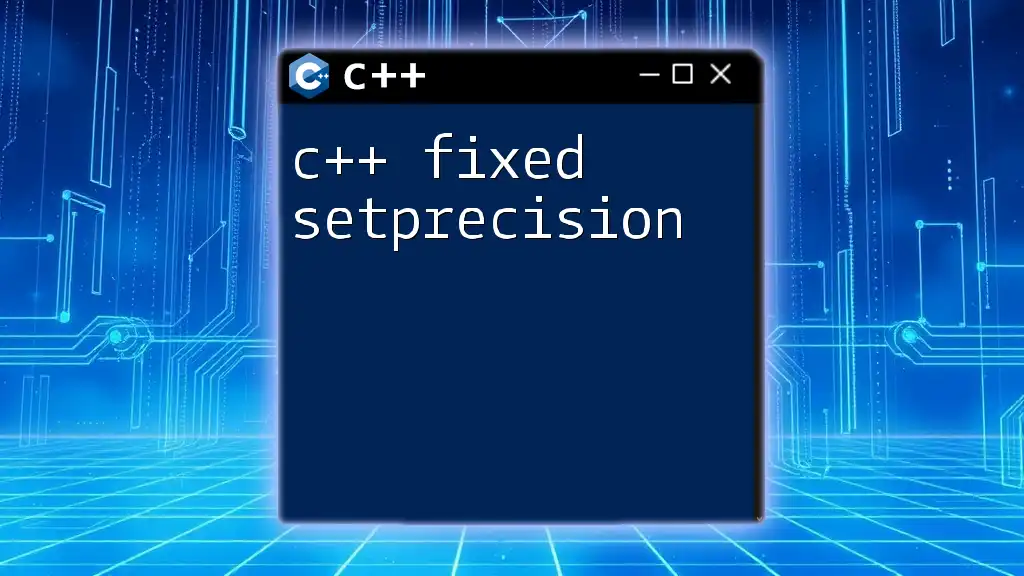
Introduction to cpp setprecision
What is `setprecision`?
`setprecision` is a manipulator defined in the `<iomanip>` header of C++. Its primary purpose is to specify the number of digits displayed for floating-point output. By controlling this aspect of output formatting, programmers can enhance readability and maintain accuracy across various applications.
Why Use `setprecision`?
Using `setprecision` is crucial for several reasons:
- It ensures that numerical outputs meet specific formatting requirements, which can be vital in reports, logging, or user interfaces.
- It can help avoid confusion that arises from overly long decimal representations, making output easier to understand.
- In scenarios like scientific data presentation, controlling the precision can conform to field standards.
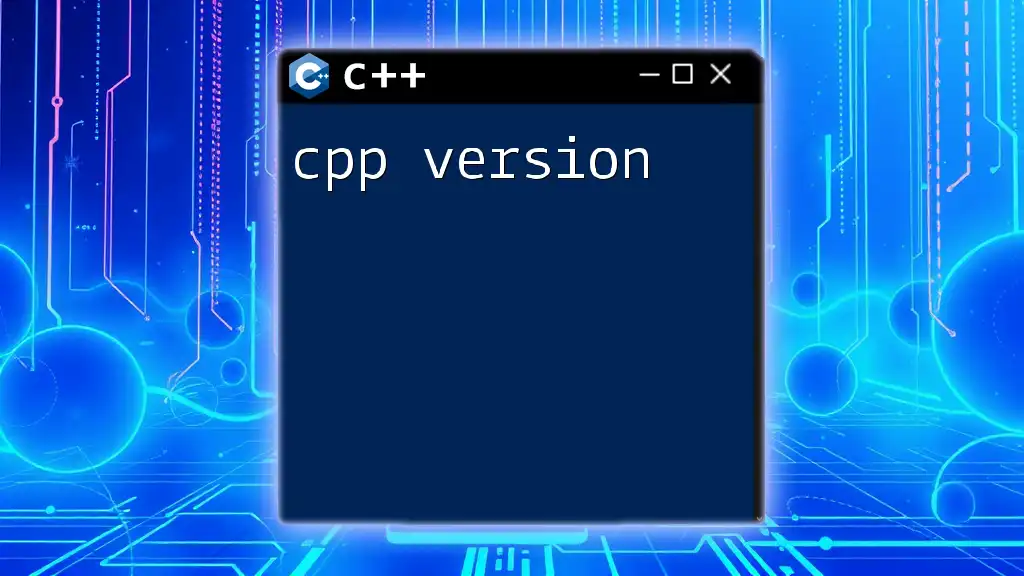
How to Use `setprecision` in C++
Basic Syntax of setprecision in C++
The basic syntax of `setprecision` is straightforward:
std::setprecision(n);
In this syntax, `n` represents the total number of digits to display. This count includes all digits, both before and after the decimal point. Below is an example illustrating its use:
#include <iostream>
#include <iomanip>
int main() {
double num = 123.456789;
std::cout << std::setprecision(5) << num << std::endl; // Output: 123.46
return 0;
}
Different Modes of `setprecision`
Default Mode
By default, C++ outputs floating-point numbers with six digits of precision. This behavior can produce lengthy, sometimes cumbersome output. Consider this example:
#include <iostream>
int main() {
double num = 123.456789;
std::cout << num << std::endl; // Output: 123.457
return 0;
}
Fixed vs Scientific Notation
Using `setprecision`, you can switch between fixed and scientific notation, each serving its own purpose.
Fixed Precision
To display numbers in fixed-point notation, use `std::fixed` along with `setprecision`. This setting shows a specified number of digits after the decimal point.
Example:
#include <iostream>
#include <iomanip>
int main() {
double num = 123.456789;
std::cout << std::fixed << std::setprecision(2) << num << std::endl; // Output: 123.46
return 0;
}
Scientific Notation
For very large or small numbers, scientific notation is often more readable. You can switch to this format using `std::scientific`.
Example:
#include <iostream>
#include <iomanip>
int main() {
double num = 123456789;
std::cout << std::scientific << std::setprecision(3) << num << std::endl; // Output: 1.235E+08
return 0;
}
How to Use setprecision Effectively
Precision with Integer Types
It is essential to note that `setprecision` affects only floating-point numbers. As a result, applying it to integers yields no change in output. For instance:
#include <iostream>
#include <iomanip>
int main() {
int num = 1234;
std::cout << std::setprecision(5) << num << std::endl; // Output: 1234
return 0;
}
Chaining with Other Formatters
`setprecision` can be combined with other formatting manipulators, such as `std::fixed`, to achieve desired output styles. For example, a combination might look like this:
#include <iostream>
#include <iomanip>
int main() {
double num = 3.14159;
std::cout << std::fixed << std::setprecision(4) << num << std::endl; // Output: 3.1416
return 0;
}
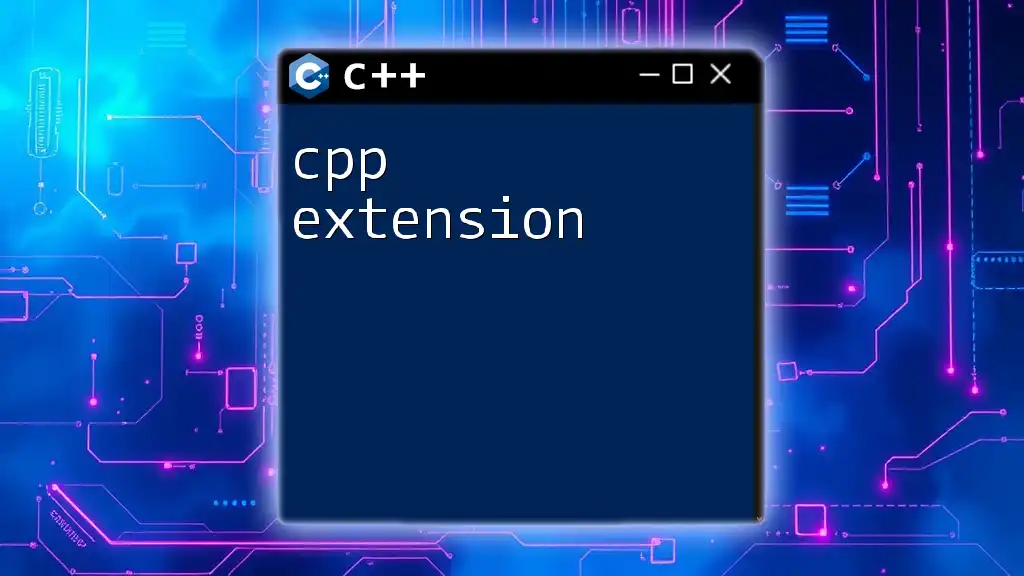
Advanced Concepts with `setprecision`
Setting Precision Globally
If you want to set precision in a way that affects multiple outputs, you can use `std::cout` in combination with `setprecision`. Keep in mind that this can lead to precision issues with subsequent outputs if not reset appropriately.
Example:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setprecision(3);
std::cout << 123.456 << std::endl; // Output: 123.456
std::cout << 3.14159 << std::endl; // Output: 3.142
return 0;
}
Impact on Performance
While `setprecision` provides formatting control, it is essential to consider its potential impact on performance. Excessive use of formatting operations can slow down output, especially in performance-critical applications. Therefore, using `setprecision` judiciously is recommended to avoid unnecessary overhead.
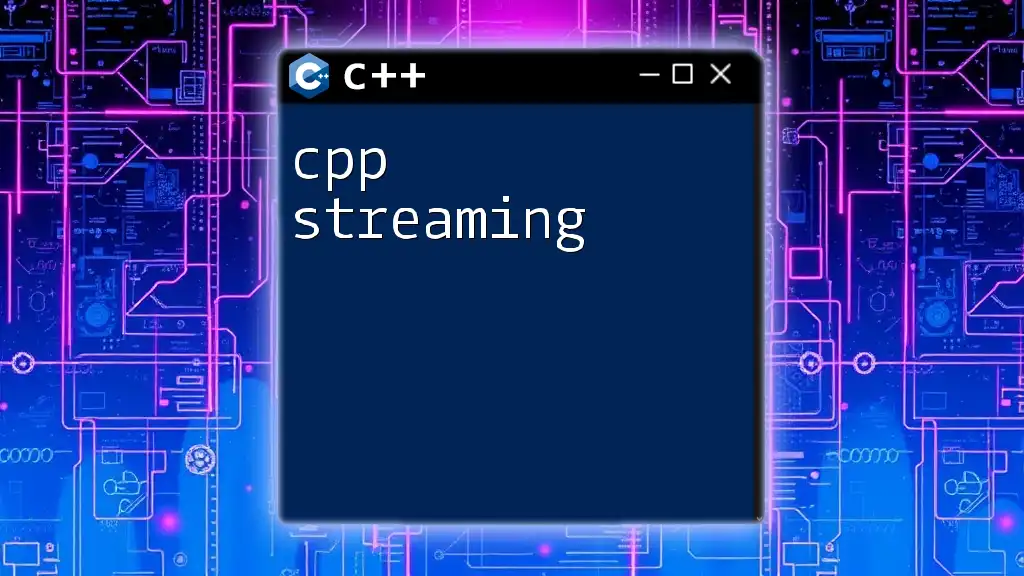
Summary
In summary, `cpp setprecision` is a powerful tool for managing numerical output in C++. It allows programmers to control how many digits are displayed for floating-point numbers, with options to output in fixed or scientific notation. Understanding how to use `setprecision` effectively can enhance program readability and compliance with specific formatting standards.
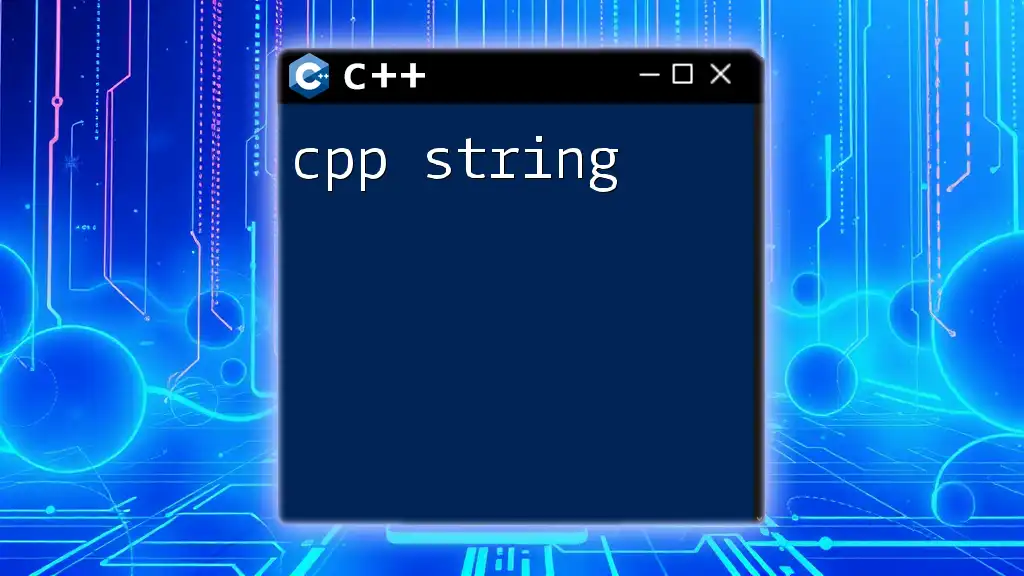
Additional Resources
For deeper insights into `setprecision` and related formatting functions, consider checking the official C++ documentation on `iostream` and manipulators. Additionally, books or online courses focused on advanced C++ topics can provide further techniques and best practices for mastering output formatting.
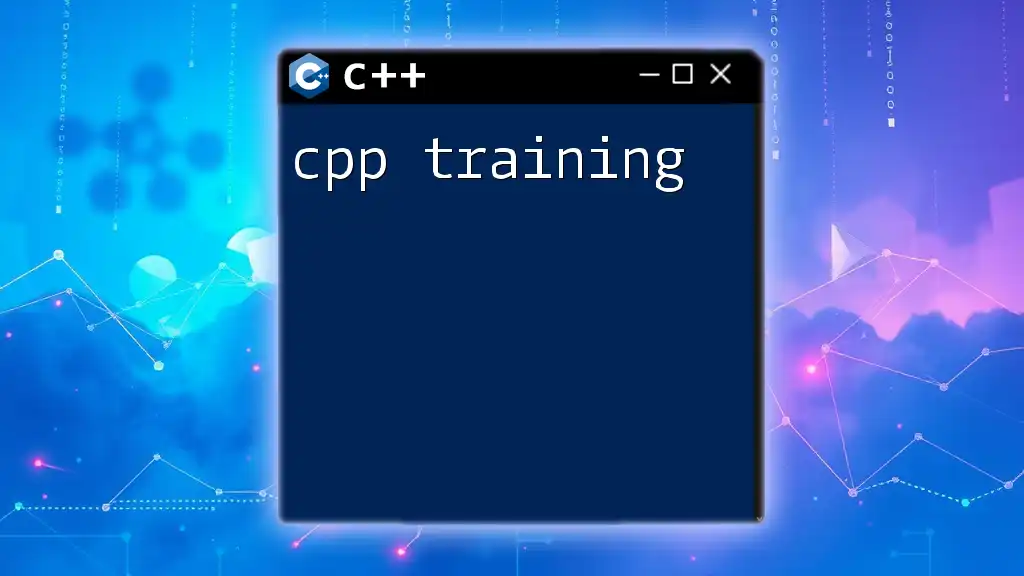
Conclusion
Mastering `cpp setprecision` not only improves the clarity of your numerical outputs but also contributes to the overall quality of your C++ programs. I encourage you to experiment with different precision settings in your projects and share your insights and questions about using `setprecision` effectively!