In C++, the `fixed` and `setprecision` manipulators are used together to control the display of floating-point numbers, ensuring that a specific number of digits appear after the decimal point.
Here’s a code snippet demonstrating their use:
#include <iostream>
#include <iomanip>
int main() {
double num = 3.14159265358979;
std::cout << std::fixed << std::setprecision(2) << num << std::endl; // Output: 3.14
return 0;
}
Understanding Precision in C++
What is Precision in C++?
Precision in C++ refers to the degree of accuracy with which a floating-point number is represented and displayed. It is crucial for numerical computations where the outcome is sensitive to the precision of its inputs. Precision becomes particularly important in applications such as scientific calculations or graphical algorithms, where even the slightest inaccuracies can lead to significant errors.
Why Use Fixed Precision?
When dealing with numerical outputs, you may need to maintain a consistent formatting style, which is where fixed precision comes into play. Fixed precision means that the program will always output the same number of digits after the decimal point, regardless of the value represented. This is essential in scenarios like **financial calculations", where rounding can significantly affect results, or in scientific contexts where uniformity in data presentation is needed.
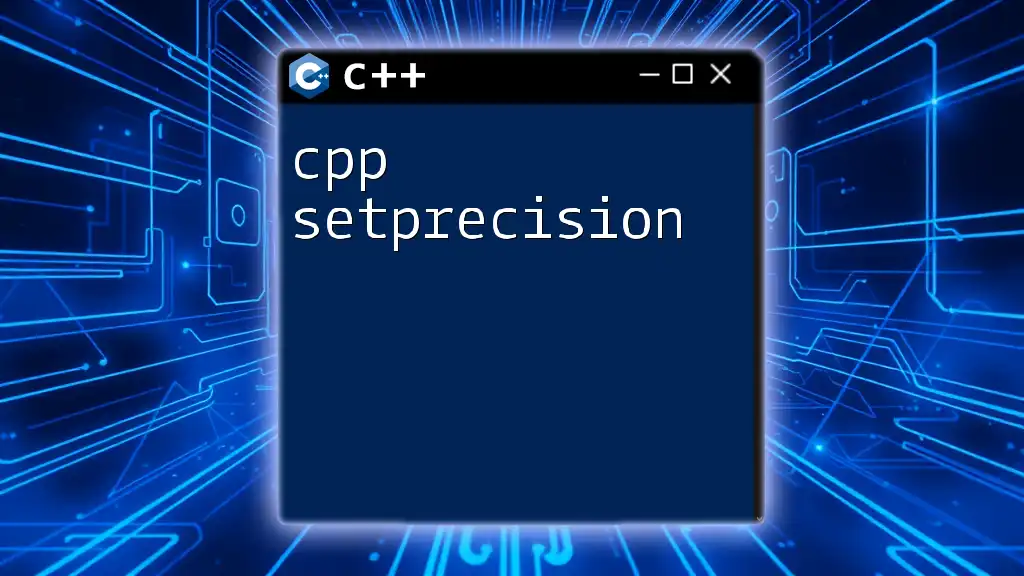
The `setprecision` Function in C++
Overview of `setprecision`
The `setprecision` function provides programmers a way to control how many digits are displayed from a floating-point number. By default, C++ will display floating-point numbers with a varying number of decimal places based on the number's value. With `setprecision`, you can specify exactly how many digits should be printed.
Syntax of `setprecision`
The syntax for this function is relatively straightforward, often used in conjunction with I/O streams. To use `setprecision`, you include it in your output statement:
std::cout << std::setprecision(n);
Here, `n` denotes the total number of digits displayed. Note that this number includes both the digits before and after the decimal point.
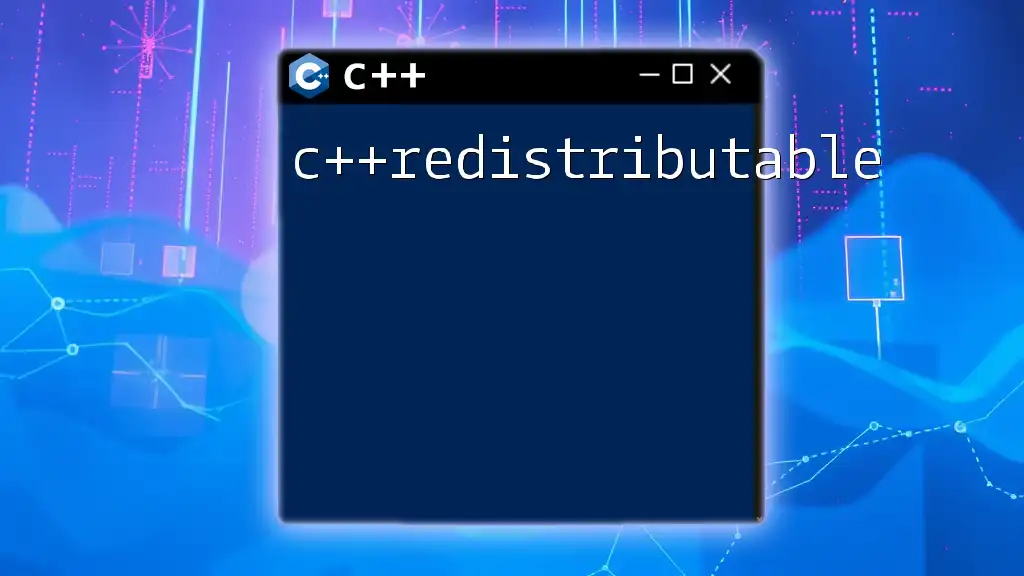
Fixed Precision with `setprecision`
How to Use Fixed with `setprecision`
To enforce fixed precision, you utilize the `std::fixed` manipulator alongside `setprecision`. This ensures that the output is consistently formatted to a specified number of decimal places.
Before you can use `setprecision` effectively, ensure to include the `<iomanip>` header:
#include <iostream>
#include <iomanip>
Let’s see an example:
int main() {
double num = 3.14159265358979;
std::cout << std::fixed << std::setprecision(2) << num << std::endl; // Outputs: 3.14
return 0;
}
In the example above, we set the precision to two decimal places and used `std::fixed` to ensure that the output is strictly in fixed format.
Using `fixed` and `setprecision` Together
The functions `std::fixed` and `std::setprecision` are often used together for clarity and precision in numerical output. When you set a specific precision level, you can manipulate the output for better readability and consistency across your application's output.
Consider the following code, which sets different levels of precision:
#include <iostream>
#include <iomanip>
int main() {
double num = 3.14159265358979;
std::cout << std::fixed << std::setprecision(4) << num << std::endl; // Outputs: 3.1416
std::cout << std::fixed << std::setprecision(1) << num << std::endl; // Outputs: 3.1
return 0;
}
Each time we change the precision, the output changes accordingly, showcasing the function's versatility in formatting output.
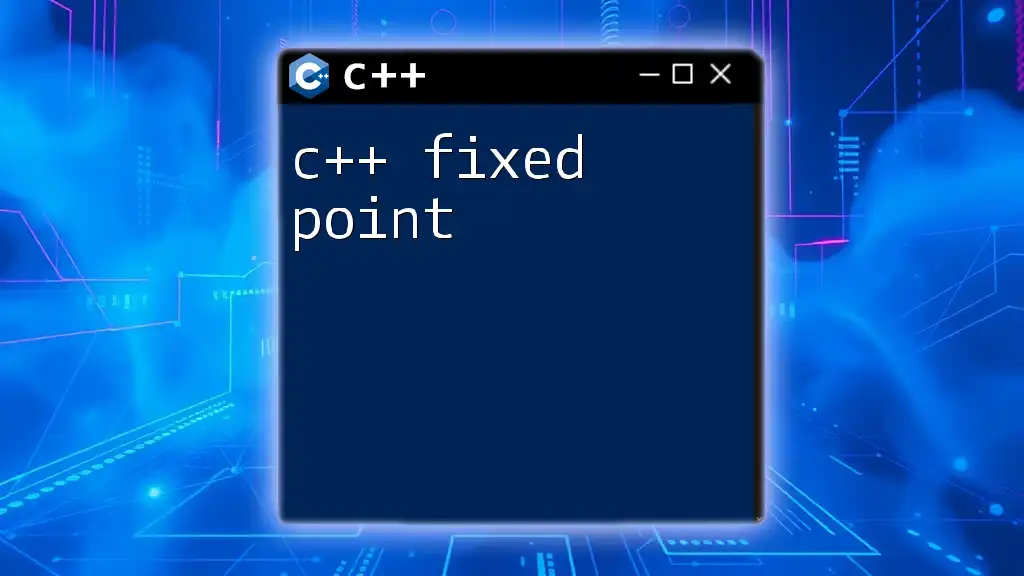
Real-world Examples of Fixed `setprecision` Usage
Financial Applications
Fixed precision is particularly useful in financial applications where transactions require high precision to prevent rounding errors. For example, in a banking application, you may want to ensure that balances are displayed correctly:
#include <iostream>
#include <iomanip>
int main() {
double balance = 12345.6789;
std::cout << std::fixed << std::setprecision(2) << balance << std::endl; // Outputs: 12345.68
return 0;
}
Using two decimal places is standard in financial applications, ensuring that users see a clear and accurate representation of currency values.
Scientific Calculations
In scientific computations, precision can dictate the reliability of results. Consider a situation where we are calculating Pi or Euler's number with high accuracy. Using fixed precision helps maintain a high degree of fidelity in displaying results:
#include <iostream>
#include <iomanip>
int main() {
const double PI = 3.141592653589793;
std::cout << std::fixed << std::setprecision(5) << PI << std::endl; // Outputs: 3.14159
return 0;
}
This ensures that you communicate results with the necessary degree of accuracy expected in scientific contexts.
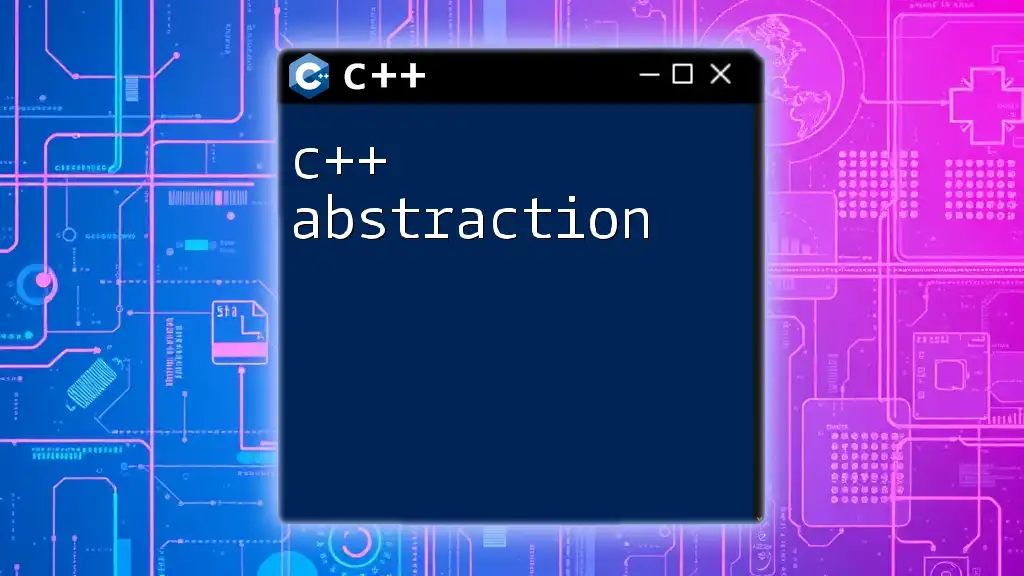
Special Considerations and Common Errors
Common Pitfalls with `setprecision`
One common mistake when using `setprecision` is neglecting to use `std::fixed`. If `std::fixed` is not applied, C++ may adjust the precision automatically based on the number, leading to unexpected results. Here's an example of how this oversight could affect your output:
#include <iostream>
#include <iomanip>
int main() {
double num = 2.5;
std::cout << std::setprecision(4) << num << std::endl; // Might output: 2.5
return 0;
}
In this instance, without `std::fixed`, the output does not show the precision you expect.
What Does Not Get Affected by `setprecision`?
`setprecision` is specifically designed to work with floating-point types. For integral types (like `int` or `long`), `setprecision` has no effect. They will always be printed as whole numbers, regardless of how `setprecision` is applied.
To understand this, consider:
int main() {
int value = 123;
std::cout << std::setprecision(4) << value << std::endl; // Outputs: 123
return 0;
}
As observed, the output remains unchanged since `setprecision` cannot modify integer types.
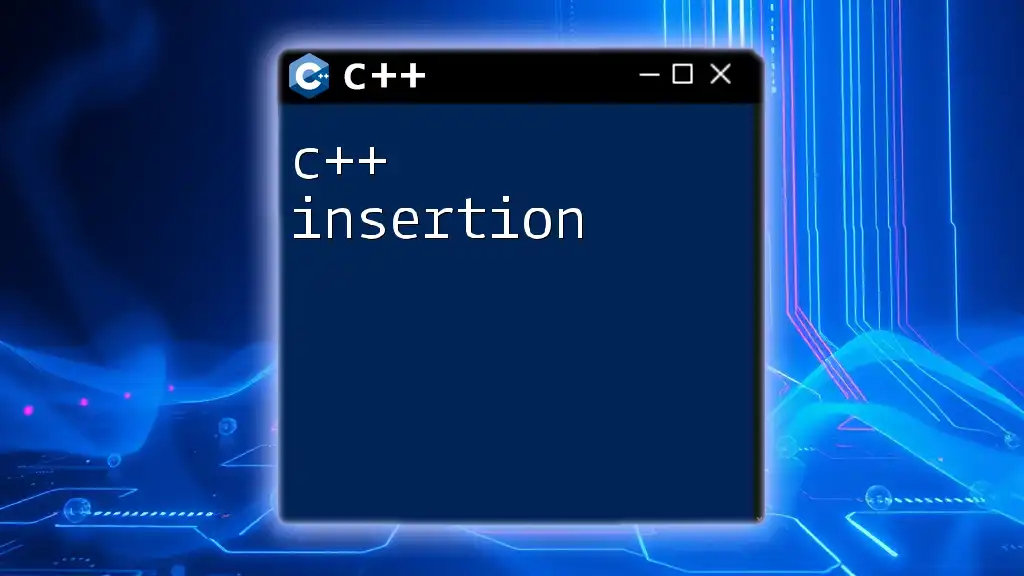
Conclusion
In summary, understanding C++ fixed setprecision is pivotal for controlling the output format of floating-point numbers effectively. By leveraging `std::fixed` and `setprecision`, you ensure your application's output remains consistent and clear. This is especially important in financial and scientific applications, where precision directly influences the results.
As you practice implementing these techniques, remember to run various code snippets to familiarize yourself with their behavior. The clearer your output, the more reliable your program will be, allowing you to present data in a manner that meets the expectations of your audience.
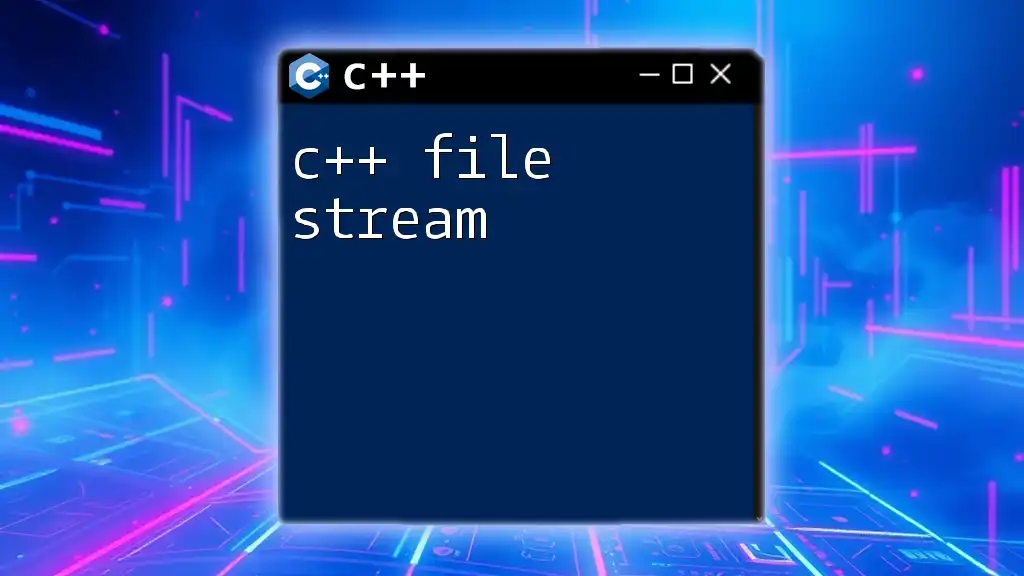
Additional Resources
For further reading and exploration of precision in C++, consider reviewing links to official C++ documentation and recommended tutorials that delve deeper into output formatting and handling floating-point arithmetic effectively.
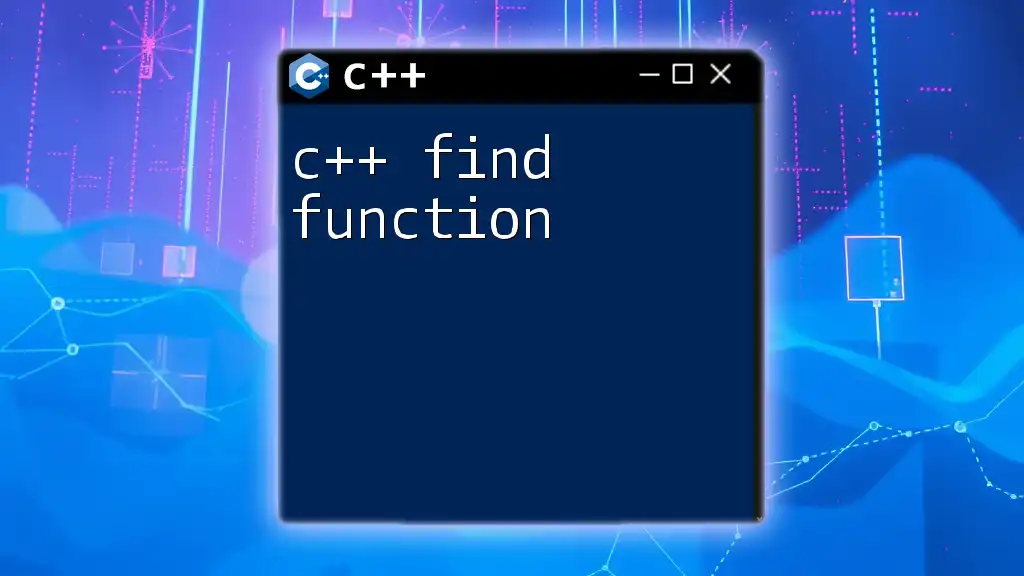
FAQ Section
What is the default precision in C++?
By default, C++ uses a precision value of six for floating-point numbers unless specified otherwise.
How does `setprecision` affect integer outputs?
`setprecision` does not change how integers are displayed; they are always printed as whole numbers.
Can I use `setprecision` without including the `<iomanip>` header?
No, you must include the `<iomanip>` header to use `setprecision` in your program effectively.