C++ insertion refers to the process of adding elements to a container like a vector or a list, often using the `push_back` method for vectors or the `insert` method for lists.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(10); // Inserting an element at the end
numbers.push_back(20);
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Insertion in C++
Importance of Insertion in Programming
C++ insertion is a fundamental concept in programming. Insertion allows us to add new data into data structures, which is crucial for dynamic applications where information is frequently updated or changed. Understanding how to efficiently insert data can significantly affect the performance of an application, making it vital for any programmer, especially when managing large datasets or implementing complex algorithms.
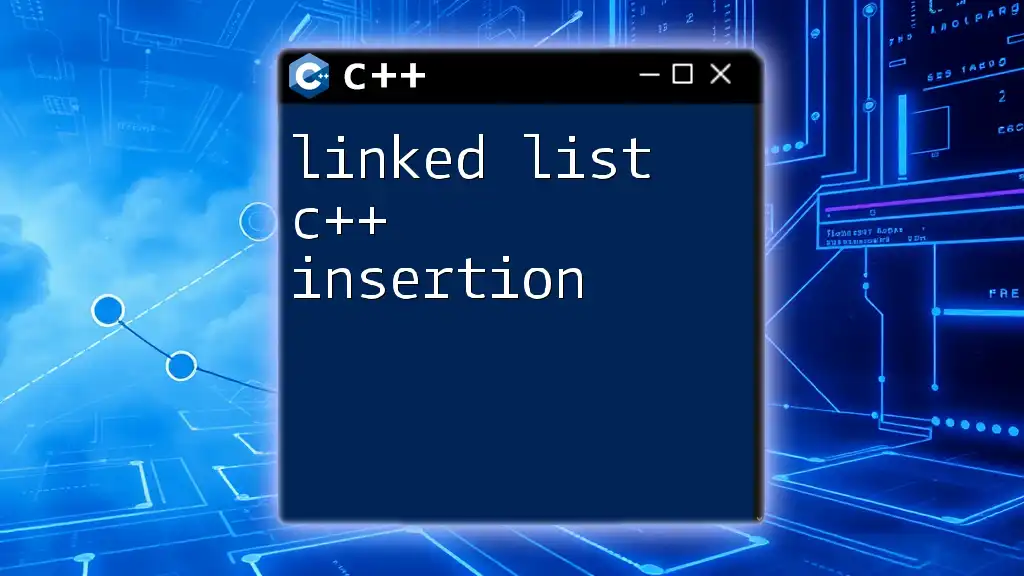
Types of Insertion in C++
Insertion in Arrays
Overview of Array Insertion
Arrays are a basic data structure that holds a fixed-size sequential collection of elements of the same type. One of the limitations of arrays is their inflexibility when it comes to insertion. Since arrays have a predefined size, inserting an element requires shifting other elements to accommodate the new entry, which can be inefficient for larger datasets.
Example: Inserting an Element into an Array
To illustrate how insertion works in an array, consider the following code snippet:
void insertIntoArray(int arr[], int &size, int element, int position) {
for (int i = size; i > position; i--) {
arr[i] = arr[i - 1];
}
arr[position] = element;
size++;
}
In this code, the function `insertIntoArray` takes in an array `arr`, its current size, the element to insert, and the position at which to insert the element. The for loop shifts existing elements to the right from the position to make space for the new element. This method showcases the linear time complexity of array insertion, which is O(n), where n is the number of elements in the array.
Insertion in Vectors
Understanding C++ Vectors
Vectors in C++ are dynamic arrays that can grow and shrink in size. They are part of the Standard Template Library (STL), providing a convenient way to handle dynamic data more efficiently than traditional fixed-size arrays. The flexibility of vectors makes insertion operations easier and more efficient.
Example: Using `push_back` to Insert Elements
Here is how you can insert elements into a vector:
#include <vector>
std::vector<int> myVector;
myVector.push_back(10); // Adding an element to the end
Using the `push_back` method, you can add elements to the end of the vector without worrying about size limitations. Vectors automatically resize themselves, making this insertion operation average O(1) in time complexity, vastly improving efficiency compared to arrays.
Insertion in Linked Lists
Overview of Linked Lists
A linked list is a data structure consisting of nodes, where each node contains data and a pointer to the next node in the sequence. Unlike arrays, linked lists provide more flexibility for insertion and deletion, allowing elements to be added or removed without shifting other elements.
Example: Inserting a Node in a Linked List
Here's how you can insert a new node at the head of a linked list:
struct Node {
int data;
Node* next;
};
void insertAtHead(Node*& head, int data) {
Node* newNode = new Node();
newNode->data = data;
newNode->next = head;
head = newNode;
}
This code creates a new node and assigns its `data` and `next` pointer. By inserting at the head, this operation achieves O(1) time complexity, making it a constant time insertion. It shows just how effective linked lists can be for inserting elements dynamically.
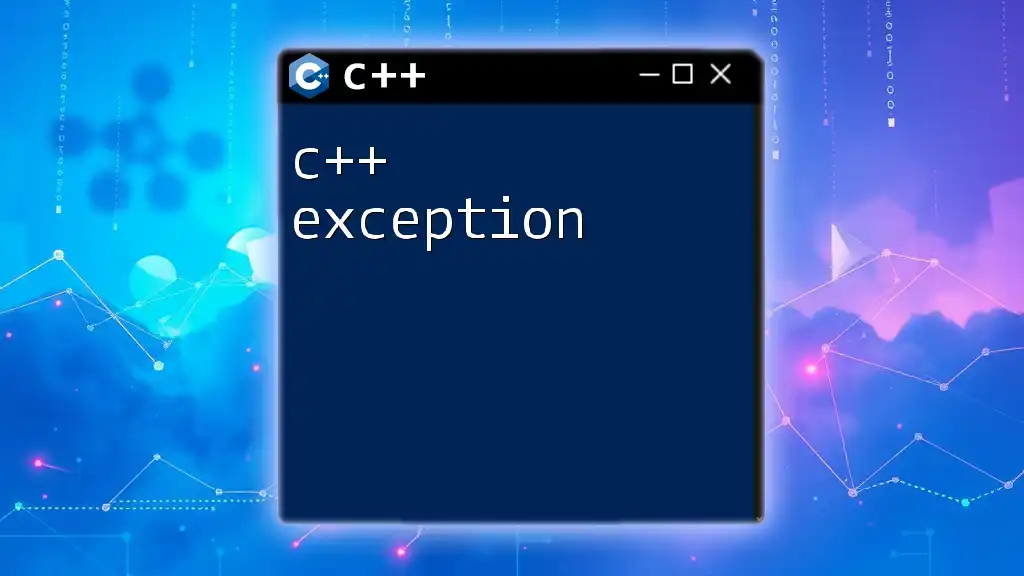
Advanced Insertion Techniques
Insertion in Trees
Introduction to Tree Structures
Trees are hierarchical data structures that consist of nodes connected by edges. A binary search tree (BST) is a type of tree that maintains sorted order, allowing for efficient insertion, deletion, and search operations.
Example: Inserting a Node in a Binary Search Tree
The following code demonstrates how to insert a node into a binary search tree:
struct TreeNode {
int data;
TreeNode* left;
TreeNode* right;
};
TreeNode* insertNode(TreeNode* root, int data) {
if (!root) {
return new TreeNode{data, nullptr, nullptr};
}
if (data < root->data) {
root->left = insertNode(root->left, data);
} else {
root->right = insertNode(root->right, data);
}
return root;
}
In this recursive function, if the `root` is null, a new node is created. Depending on the comparison between `data` and the current node’s data, the function navigates left or right. The time complexity for this operation is O(log n) in a balanced tree, making it a highly efficient method for data insertion.
Insertion in Sets
The Concept of Sets in C++
Sets in C++ are part of the STL and allow for storing unique elements. When we try to insert a duplicate value, the set automatically ignores it. This feature makes sets particularly useful in scenarios where uniqueness is a requirement.
Example: Inserting Elements in a Set
Here’s how you can insert elements into a set:
#include <set>
std::set<int> mySet;
mySet.insert(5);
mySet.insert(10);
mySet.insert(5); // Will not add duplicate
The `insert` function prevents duplicates and has an average time complexity of O(log n), owing to the underlying balanced tree structure used for implementation.
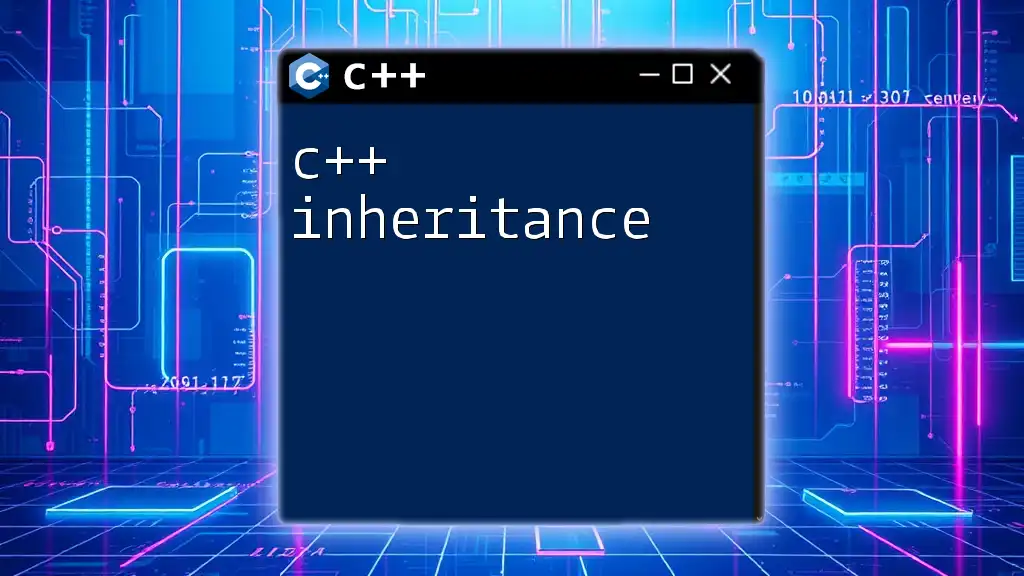
Optimizing Insertion Operations
Best Practices for Efficient Insertion
To optimize insertion performance, select the appropriate data structure based on the specific use case. For example, if frequent insertions and deletions occur, linked lists or deques may be appropriate. On the other hand, when most operations are read, but infrequent insertions are needed, vectors or arrays could enhance performance.
Case Study: Performance Analysis
When analyzing the time complexity of various insertion methods, understand that each data structure serves different scenarios. Arrays have a longer insertion time due to shifting elements, whereas linked lists and trees offer more efficient options for insertion operations. Understanding these trade-offs will help you choose the right data structure tailored to your requirements.
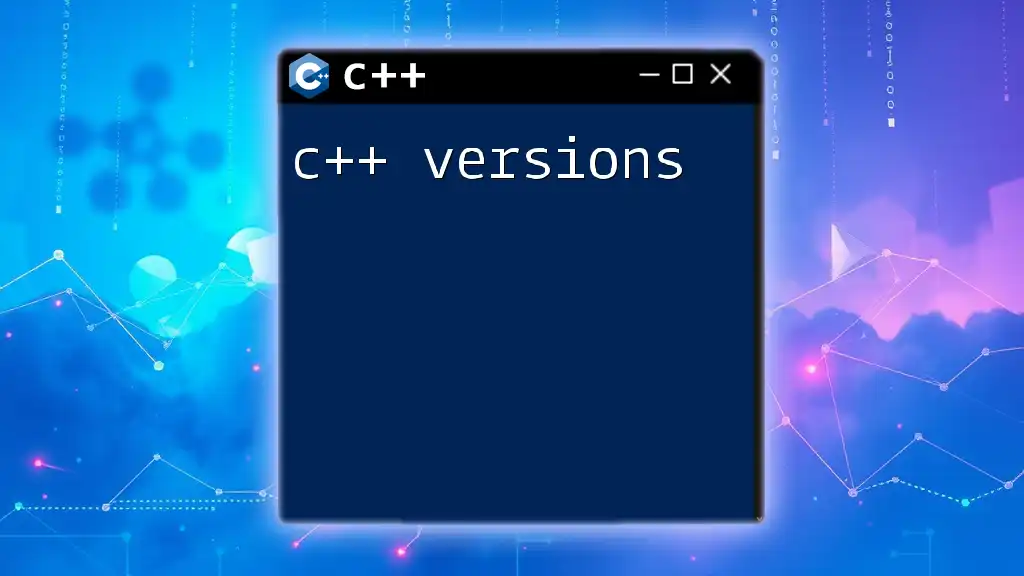
Common Pitfalls and How to Avoid Them
Mistakes in Insertion Logic
Common errors in insertion logic can lead to performance degradation or even program crashes. Errors such as memory leaks or off-by-one errors often arise when manipulating pointers or array indices. To avoid these pitfalls, always validate your input values, and consider utilizing tools like sanitizers or debuggers to identify and rectify logical flaws.
Conclusion
Summary of Key Takeaways
C++ insertion plays a critical role in how we manage and manipulate data within our programs. Understanding the various methods for inserting data across different data structures can significantly enhance the efficiency of algorithms and overall program performance.
Further Reading and Resources
For continued learning, consider checking advanced C++ literature, participating in programming forums, and exploring further resources that dive into data structures and algorithms for a deeper understanding of insertion techniques in C++.