In C++, a linked list can be updated by inserting a new node at a specified position using a simple structure that defines the node and a function to handle the insertion.
struct Node {
int data;
Node* next;
};
void insert(Node** head_ref, int new_data, int position) {
Node* new_node = new Node();
new_node->data = new_data;
new_node->next = nullptr;
if (position == 0) {
new_node->next = *head_ref;
*head_ref = new_node;
return;
}
Node* temp = *head_ref;
for (int i = 0; temp != nullptr && i < position - 1; i++)
temp = temp->next;
if (temp == nullptr) return;
new_node->next = temp->next;
temp->next = new_node;
}
Linked List Insertion in C++
What is a Linked List?
A linked list is a linear data structure where elements, known as nodes, are stored in a non-contiguous manner. Each node contains two parts: the data itself and a pointer to the next node in the list. Unlike arrays, linked lists do not rely on continuous memory locations, allowing for efficient dynamic memory allocation. This flexibility in memory usage is a significant advantage, especially when dealing with unpredictable data sizes.
Types of Linked Lists
There are several types of linked lists, each serving specific requirements:
-
Singly Linked List: This type consists of nodes that link to the next node only, making traversal in one direction possible.
-
Doubly Linked List: Nodes in a doubly linked list contain pointers to both the next and previous nodes, allowing for bidirectional traversal.
-
Circular Linked List: In this configuration, the last node points back to the first node, creating a circular structure that can be singly or doubly linked.
Structure of a Node
In C++, a node can be expressed using a structure. The typical definition includes an integer for the data and a pointer to the next node.
struct Node {
int data;
Node* next;
};
This simple structure allows linked lists to maintain a cohesive series of nodes, facilitating a variety of operations, including insertion, deletion, and traversal.
Memory Management in Linked Lists
One pivotal aspect of linked lists is their reliance on dynamic memory allocation. With the `new` operator, nodes can be allocated on-the-fly, which allows the list to grow or shrink according to the needs of the application. Proper use of `delete` is essential to avoid memory leaks when nodes are no longer needed.
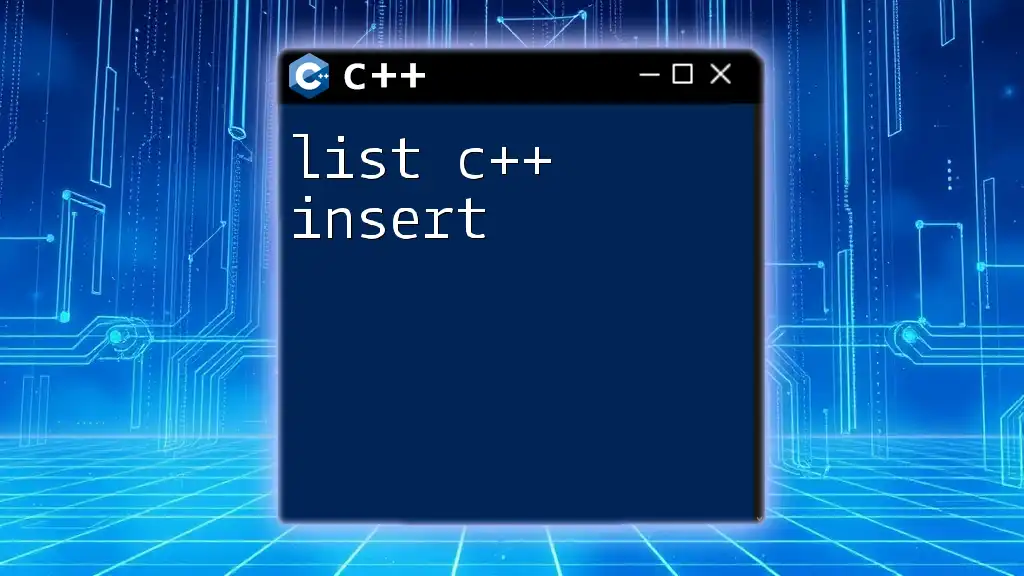
Insertion Operations in a Linked List
Overview of Insertion
Insertion is one of the fundamental operations performed on linked lists. It involves adding a new node to the list, which can occur in several locations: at the beginning, at the end, after a specific node, or at a given position. Each insertion scenario requires a distinct approach.
Inserting at the Beginning
To insert a new node at the start of the list, the process is quite straightforward.
- Create a new node.
- Point the new node’s next to the current head.
- Update the head to the new node.
Here’s how that looks in code:
void insertAtBeginning(Node** head_ref, int new_data) {
Node* new_node = new Node();
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
This function effectively places the new node at the front, making it the new head of the list.
Inserting at the End
Inserting at the end of a linked list requires traversing the entire list to find the last node and then linking the new node to it. The steps involved are:
- Create a new node.
- Check if the list is empty. If so, make the new node the head.
- Traverse to the end of the list, then set the last node’s next to the new node.
This can be implemented as follows:
void insertAtEnd(Node** head_ref, int new_data) {
Node* new_node = new Node();
Node* last = *head_ref;
new_node->data = new_data;
new_node->next = nullptr;
if (*head_ref == nullptr) {
*head_ref = new_node;
return;
}
while (last->next != nullptr) {
last = last->next;
}
last->next = new_node;
}
Inserting After a Specific Node
To place a new node after a particular existing node, the following steps are essential:
- Ensure the previous node isn’t null.
- Create a new node and set its next pointer to the previous node’s next.
- Update the previous node’s next to the new node.
Here’s the code for inserting after a specific node:
void insertAfter(Node* prev_node, int new_data) {
if (prev_node == nullptr) {
std::cout << "The given previous node cannot be NULL.";
return;
}
Node* new_node = new Node();
new_node->data = new_data;
new_node->next = prev_node->next;
prev_node->next = new_node;
}
Inserting at a Specific Position
Inserting a node at a specific index requires a little more logic:
- Validate the position. If it's less than zero, ignore it.
- Create a new node.
- If the position is zero, use the “insert at the beginning” technique.
- Traverse the list to the desired position and insert the new node.
The implementation looks like this:
void insertAtPosition(Node** head_ref, int position, int new_data) {
if (position < 0) return;
Node* new_node = new Node();
new_node->data = new_data;
if (position == 0) {
new_node->next = *head_ref;
*head_ref = new_node;
return;
}
Node* current = *head_ref;
for (int i = 0; current != nullptr && i < position - 1; i++)
current = current->next;
if (current == nullptr) return;
new_node->next = current->next;
current->next = new_node;
}
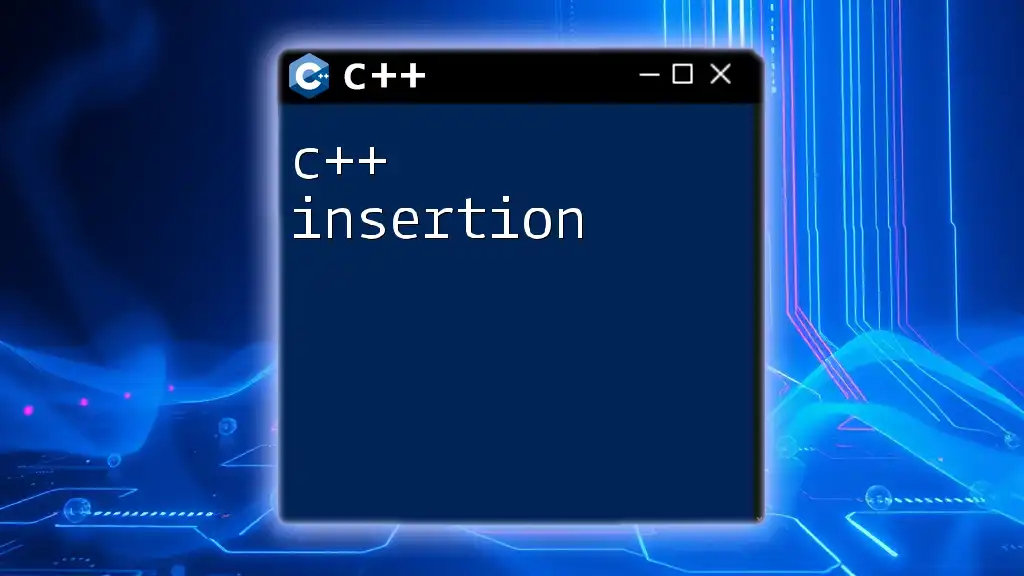
Best Practices for Linked List Insertion
Error Handling
It's crucial to implement checks for null pointers and ensure memory allocation is successful. Failing to address potential issues can lead to program crashes.
Memory Leaks
To avoid memory leaks, ensure every allocated node is properly deleted when no longer needed. Use `delete` to free memory, and always maintain a pointer to the head node to prevent losing track of the list.
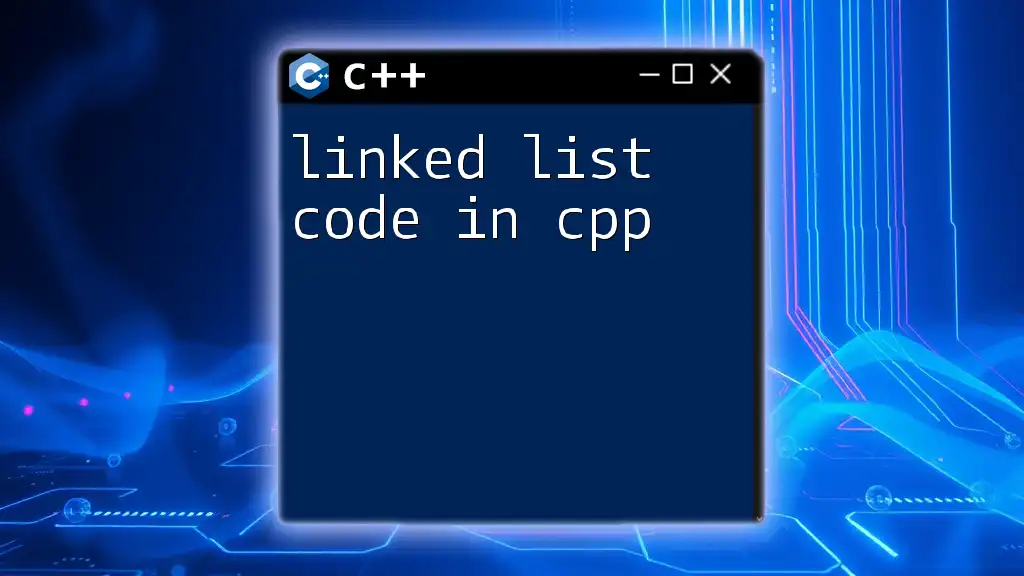
Common Mistakes to Avoid
Inserting with Null Pointers
Always check if the previous node or head is null before proceeding with insertion to avoid dereferencing null pointers that may lead to undefined behavior.
Forgetting to Update Head
When inserting at the beginning or in an empty list, remember to update the head pointer. Neglecting this can result in data loss or inaccessible nodes.
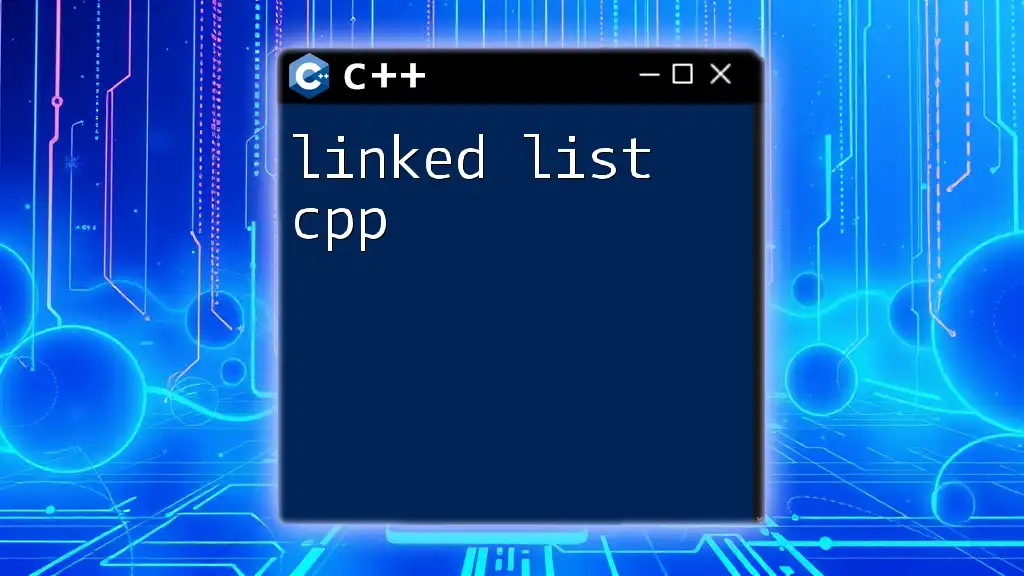
Conclusion
Inserting nodes into a linked list is a fundamental operation that requires careful consideration of various scenarios. Each insertion method covered provides the versatility needed for efficient linked list management. To master linked list c++ insertion, practice implementing these functions while paying attention to error handling and memory management. By doing so, you'll strengthen your understanding and build a robust foundation in linked list operations.
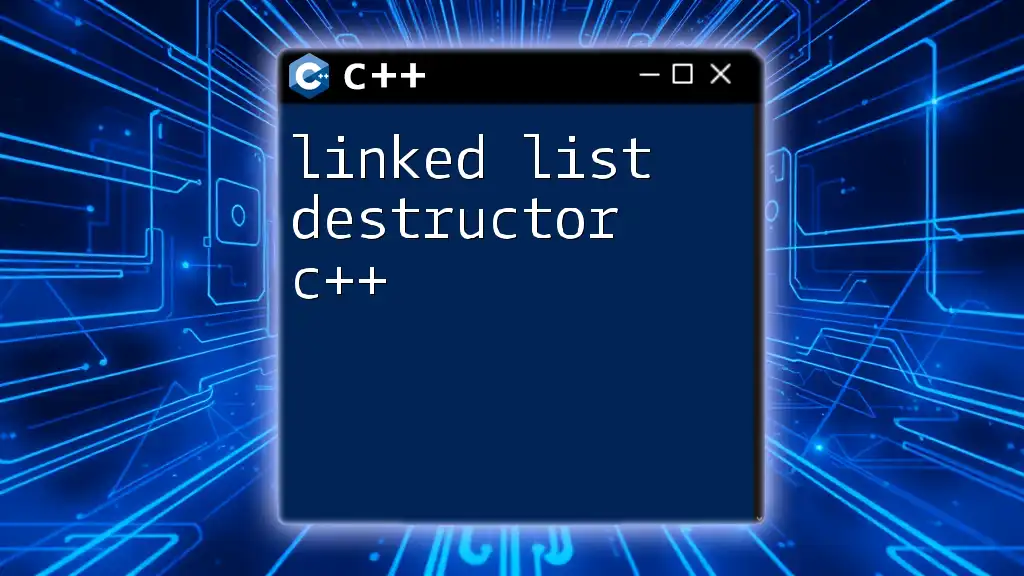
Additional Resources
For further learning, explore recommended textbooks on data structures and algorithms, as well as online courses that dive into linked lists. Engaging with practical exercises will also solidify your skills. Consider solving linked list challenges to test your knowledge and enhance your coding abilities in C++.