Reddit is a popular platform where users frequently seek advice and share knowledge about C++ programming, often asking concise questions that can be answered with practical code snippets.
Here’s an example of a simple C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Reddit as a Learning Tool
The Power of Community Learning
Community-driven platforms like Reddit serve as invaluable resources for those delving into C++ programming. Here, users can engage with a plethora of experienced programmers and novices alike to seek answers and share knowledge. Engaging with this community can bridge the learning gap, offering real-time solutions and insights into common problems that many encounter in the C++ landscape.
Top Subreddits for C++ Questions
The C++ community on Reddit features several subreddits tailored for specific needs:
- r/cpp: This is a general subreddit for discussions around C++, focusing on news, libraries, and language features.
- r/learncpp: Aimed at beginners, this subreddit offers tutorials, resources, and a supportive environment for those just getting started with C++.
Exploring these communities can lead you to diverse perspectives and effective solutions to your C++ queries.
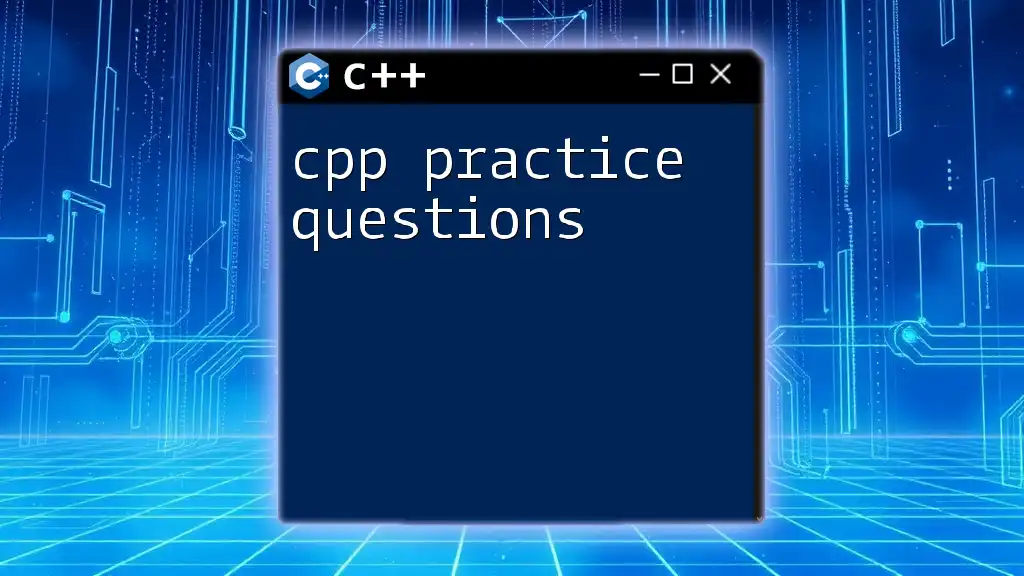
Common C++ Questions from Reddit
Basic Syntax and Structure Questions
What is the difference between `#include <header>` and `#include "header"`?
When including headers in C++, the syntax you choose has implications for the compiler’s search path:
- Using `#include <header>` tells the compiler to look in system directories where standard and third-party libraries reside.
- Conversely, `#include "header"` instructs the compiler to first look in the project directory before traversing system paths.
Here’s a code snippet that illustrates the different usages:
#include <iostream> // Using angle brackets for standard libraries
#include "my_header.h" // Using quotes for local header files
How does the main function work in C++?
The entry point of every C++ program is the `main` function. Defined as `int main()`, it signifies that the function will return an integer value to the operating system.
A basic structure looks like this:
int main() {
// Your code goes here
return 0; // Indicates successful completion
}
This structure is essential for defining program flow and understanding how execution starts.
Object-oriented Programming Questions
What is the difference between classes and structs?
In C++, both classes and structs are used to create user-defined data types, but they have key differences:
- Visibility: Members of a class are private by default, while members of a struct are public.
- Intended Use: Classes are typically used to encapsulate data and behaviors (methods), while structs are used primarily for data storage.
Here’s a simple comparison:
struct MyStruct {
int x; // Public member by default
};
class MyClass {
private:
int x; // Private member by default
public:
void setX(int val) { x = val; }
};
Both can hold data and methods, but the choice between them often depends on your design preferences and the complexity of the application.
How to implement inheritance in C++?
Inheritance allows one class to inherit the properties and methods of another. This is a fundamental principle of object-oriented programming that promotes code reusability.
Consider this code snippet demonstrating public inheritance:
class Base {
public:
void show() { std::cout << "Base Class" << std::endl; }
};
class Derived : public Base {
public:
void show() { std::cout << "Derived Class" << std::endl; }
};
In this example, `Derived` inherits from `Base`, enabling it to use the `show` method defined in `Base`. The derived class can also override inherited methods, as demonstrated, making it a pivotal concept in structuring C++ applications.
Advanced Concepts and Features
What are smart pointers and how do they help with memory management?
Smart pointers in C++ (available from C++11 onward) provide automatic memory management through RAII (Resource Acquisition Is Initialization). They help prevent memory leaks and dangling pointers. The three primary types of smart pointers are:
- `std::unique_ptr`: Manages a single resource and delivers exclusive ownership.
- `std::shared_ptr`: Allows multiple pointers to share ownership of a resource.
- `std::weak_ptr`: Provides a non-owning reference to a resource managed by `shared_ptr`.
Consider this example using `std::unique_ptr`:
#include <memory>
std::unique_ptr<int> p1(new int(5)); // Create a unique pointer
In this case, `p1` automatically deallocates its memory when it goes out of scope, making manual memory management unnecessary.
How to use lambda expressions in C++?
Lambda expressions are a feature introduced in C++11, enabling the creation of anonymous functions on-the-fly. They are especially useful for operations like sorting or filtering collections.
Here’s an example of using a lambda expression to sort a vector:
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {4, 1, 3, 2};
std::sort(numbers.begin(), numbers.end(), [](int a, int b) { return a < b; });
for (const auto& n : numbers) {
std::cout << n << " "; // Output sorted numbers
}
return 0;
}
In this code, the lambda function `[](int a, int b) { return a < b; }` defines a comparison operation that is passed directly to `std::sort`.
Common Errors and Debugging Questions
What does "undefined reference" mean in C++?
The "undefined reference" error occurs during the linking phase of compilation when the compiler cannot find the definition of a declared function or variable. This often arises due to either missing implementation files or mismatched function prototypes.
A common scenario leading to this error is when a function is declared but never defined:
void myFunction(); // Declaration
int main() {
myFunction(); // Call to an undefined reference
return 0;
}
To resolve this, ensure that all declared functions have their corresponding definitions provided.
How to use a debugger effectively in C++?
Debugging is crucial for finding and fixing errors in your code. Popular debugging tools include gdb and built-in debuggers in IDEs like Visual Studio or Code::Blocks.
Here are some tips for effective debugging:
- Set Breakpoints: Use breakpoints to pause execution at critical lines in your code.
- Inspect Variables: Monitor the values of variables to identify unexpected changes or errors in logic.
- Step Through Code: Utilize step-over and step-into functionalities to traverse through your code line by line.
Using these techniques can drastically shorten the time spent troubleshooting.
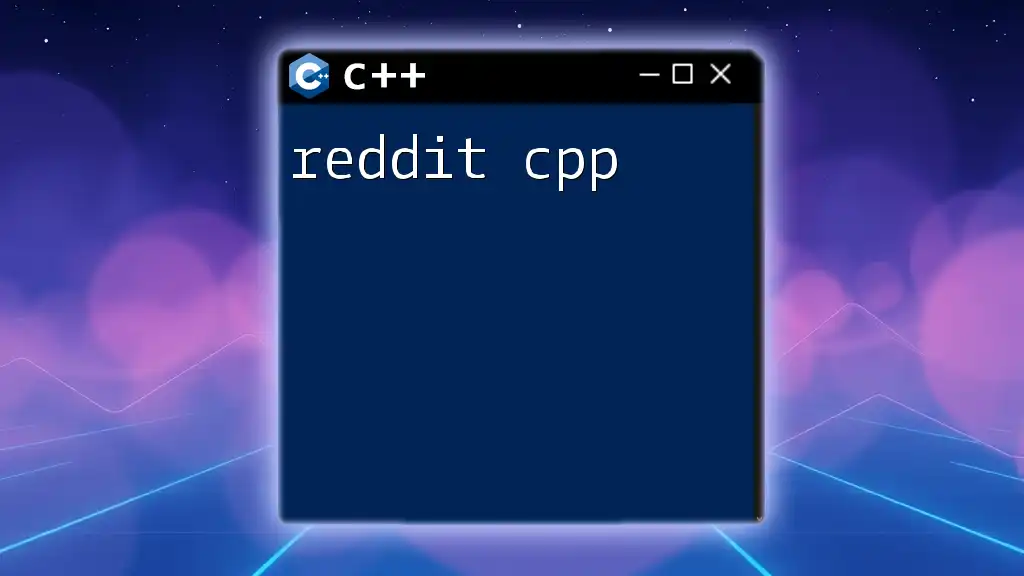
Resources for Continued Learning
Recommended Books and Online Courses
To further deepen your understanding of C++, consider these resources:
- Books:
- "The C++ Programming Language" by Bjarne Stroustrup
- "Effective Modern C++" by Scott Meyers
- Online Courses:
- Coursera: Courses by top universities focusing on C++.
- Udacity: Nanodegree programs specializing in C++ development.
Helpful Online Tools and Compilers
In addition to traditional IDEs, online compilers like Replit and Ideone offer convenient environments to test snippets of C++ code. These platforms are especially useful for sharing code quickly or collaborating with others without the need for intricate setup.
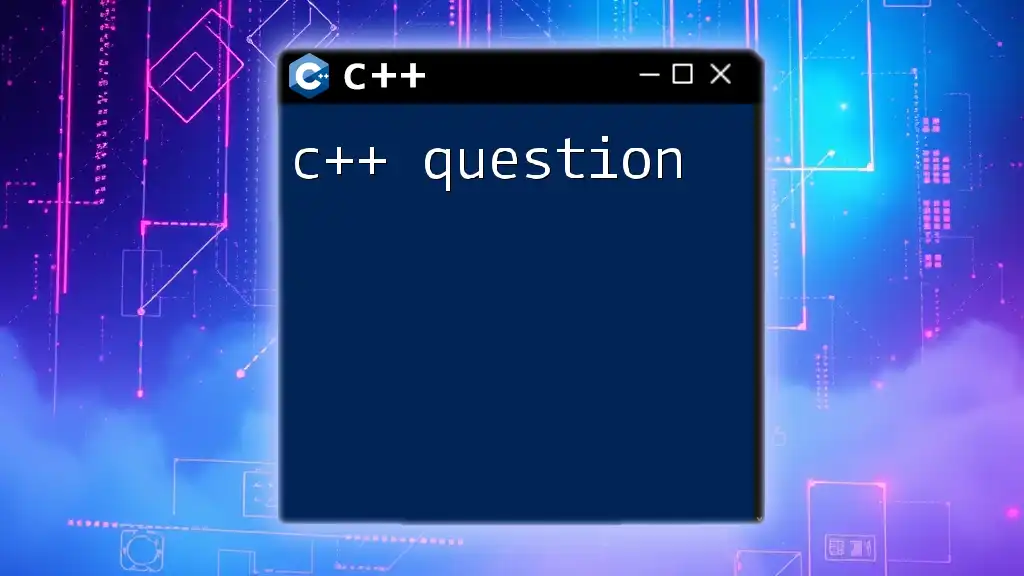
Conclusion
Engaging with reddit cpp questions not only enhances your learning but also connects you with a community of C++ enthusiasts ready to help. By actively participating in forums, you can gain diverse insights and solutions to the challenges you face in your programming journey. Remember to contribute your knowledge as well; community learning is a two-way street.
Consider joining our company’s upcoming courses and resources designed to teach C++ commands quickly and efficiently. Whether you're a beginner or an intermediate programmer, we’re here to help you advance your skills!