"Reddit cpp" refers to the community and discussions surrounding the use of C++ commands shared and learned on Reddit, often featuring quick tips and concise code snippets for efficient programming.
#include <iostream>
int main() {
std::cout << "Hello, Reddit CPP Community!" << std::endl;
return 0;
}
Understanding C++ Commands
What Are C++ Commands?
C++ commands refer to the various instructions that can be executed in a C++ program to perform specific operations. Mastering these commands is crucial for writing effective and efficient code. They encompass everything from basic input and output operations to complex algorithms that manipulate data structures.
Key Features of C++
C++ is known for its versatility and power, driven by several key features:
- Object-Oriented Programming (OOP): This paradigm promotes code reusability and modularity through concepts like classes and objects.
- Standard Template Library (STL): STL provides ready-to-use classes and functions that drastically reduce programming time.
- Memory Management: Awareness of dynamic memory allocation and deallocation is critical when working in C++.
For example, the following snippet illustrates the use of classes in C++, a hallmark of object-oriented programming:
#include <iostream>
class Animal {
public:
void speak() {
std::cout << "Animal speaks!" << std::endl;
}
};
int main() {
Animal a;
a.speak();
return 0;
}
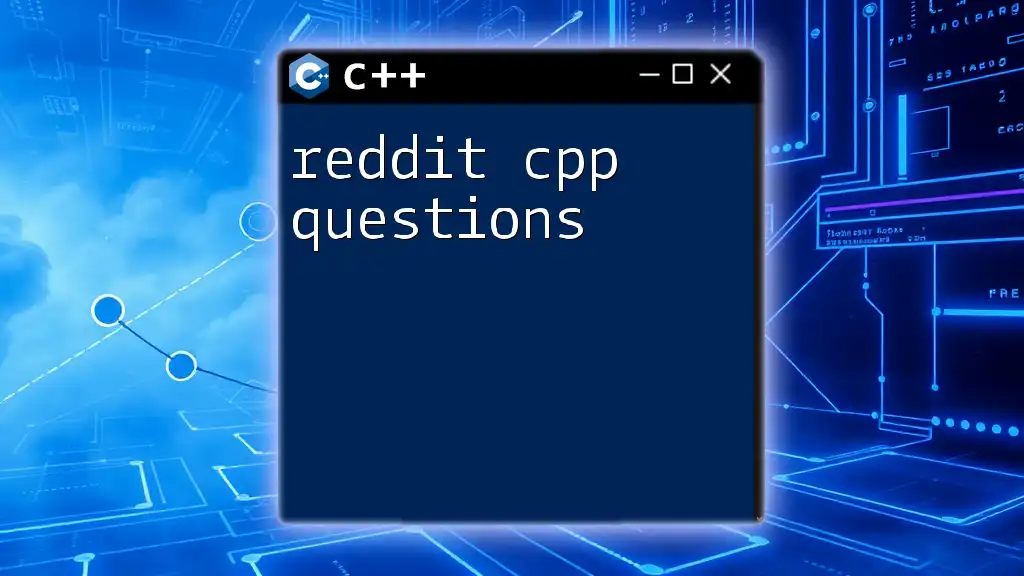
Reddit as a Learning Platform
The Power of Reddit for Programmers
Reddit has evolved into a dynamic space for technology discussions, especially for programmers. It offers a unique platform where developers can ask questions, share knowledge, and learn from community experiences. The ability to connect with others facing similar challenges is invaluable.
Must-Visit Subreddits for C++ Enthusiasts
-
r/CPlusPlus: This is the premier subreddit for discussions focused on C++. Users post anything from questions about syntax to project showcases. An example thread might explore the pros and cons of using `std::map` versus `std::unordered_map`.
-
r/learncpp: Tailored for beginners, this subreddit focuses on learning resources and tutorials. It offers a wealth of user-generated content, including step-by-step guides to mastering C++.
-
r/programming: Though broader in scope, this subreddit often features discussions about various programming languages, including C++. Engaging here can provide insights into how C++ compares to other languages and frameworks.
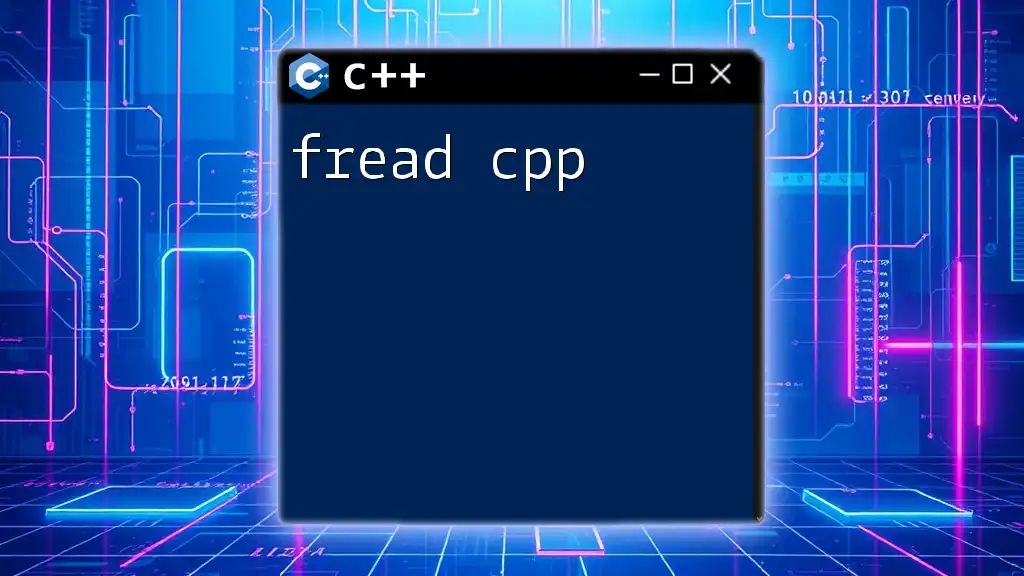
Engaging with the Community
Best Practices for Participating in Reddit Discussions
When engaging on Reddit, asking effective questions is essential. A well-crafted question often garners more detailed responses. Start by providing context, outlining what you’ve tried, and specifying the areas where you require assistance. Additionally, show appreciation for advice given; this fosters a positive community atmosphere.
Contributing to C++ Discussions
One of the best ways to enhance your skills is by sharing your knowledge. If you've solved a common issue, consider writing a post detailing your process or explaining a tricky C++ concept. Popular posts often include code snippets and explanations that allow others to learn from your experiences. For instance, if you found a unique solution to a problem using recursion, share it with an example to inspire fellow programmers.
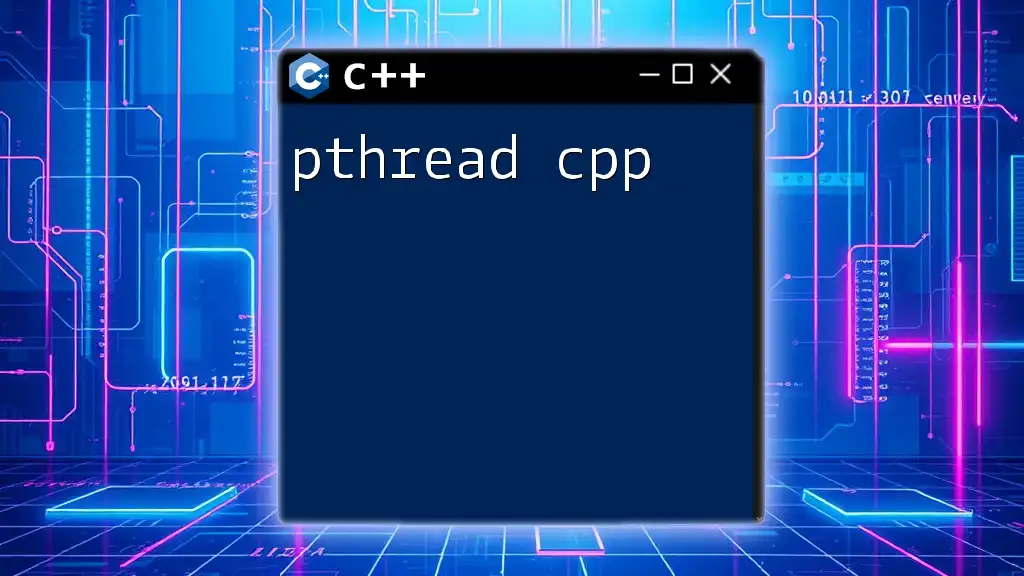
Resources for Learning C++ on Reddit
Recommended Learning Threads
Reddit is rich with standout threads focusing on C++. For example, there are discussions around common pitfalls when starting with pointers or the differences between `malloc` and `new`. Curating these links can help structure your learning and guide you through your programming journey.
Tutorials and External Links Shared on Reddit
Users frequently share valuable tutorials on Reddit. Threads showcasing resources such as Codecademy, Udacity, and Coursera provide a starting point for learners. A post detailing a beginner-friendly C++ tutorial that covers basic syntax might read as follows:
- Resource: Codecademy's C++ Course
- Overview: Offers an interactive environment to learn fundamental C++ syntax.
- Link: (Insert actual link)
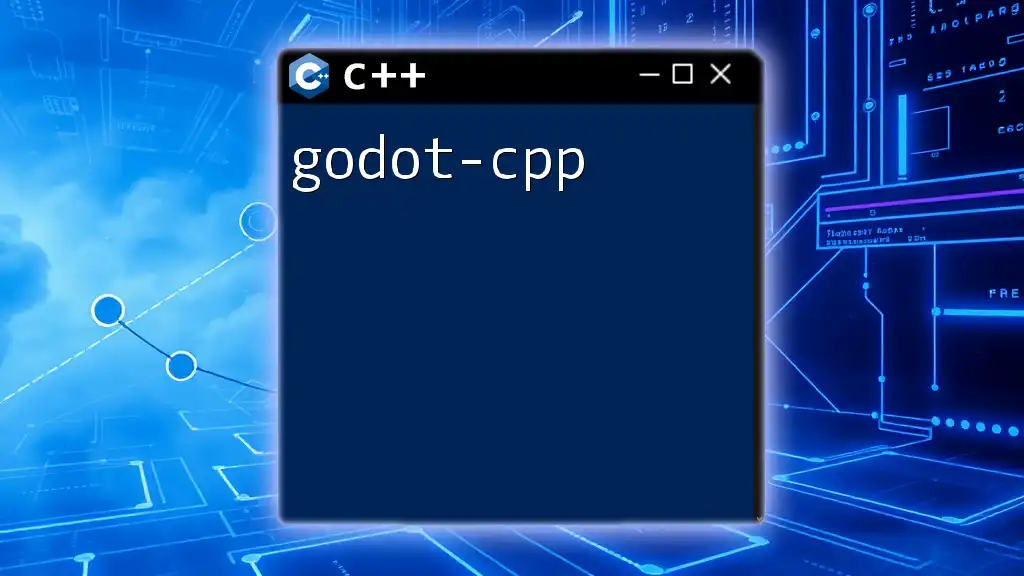
Common C++ Commands and Their Use Cases
Essential C++ Commands for Beginners
For those just starting with C++, some of the fundamental commands include:
- Output Command (`cout`): Output strings or variables to the console.
Example usage:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Intermediate C++ Commands to Explore
As you advance, it's important to familiarize yourself with command structures like `std::vector` and `std::map`. These templates from the STL help streamline your code and handle complex data tasks more efficiently.
Consider `std::vector`, which allows you to work with dynamically-sized arrays. Here’s an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
for(int num : nums) {
std::cout << num << " ";
}
return 0;
}
Advanced C++ Commands for Expert Coders
For those looking to delve into more complex areas of C++, you might explore operator overloading. This feature enhances the functionality of operators for user-defined types. Below is an example that showcases how to overload the addition operator:
class Complex {
public:
double real, imag;
Complex operator+(const Complex& other) {
return Complex{real + other.real, imag + other.imag};
}
};
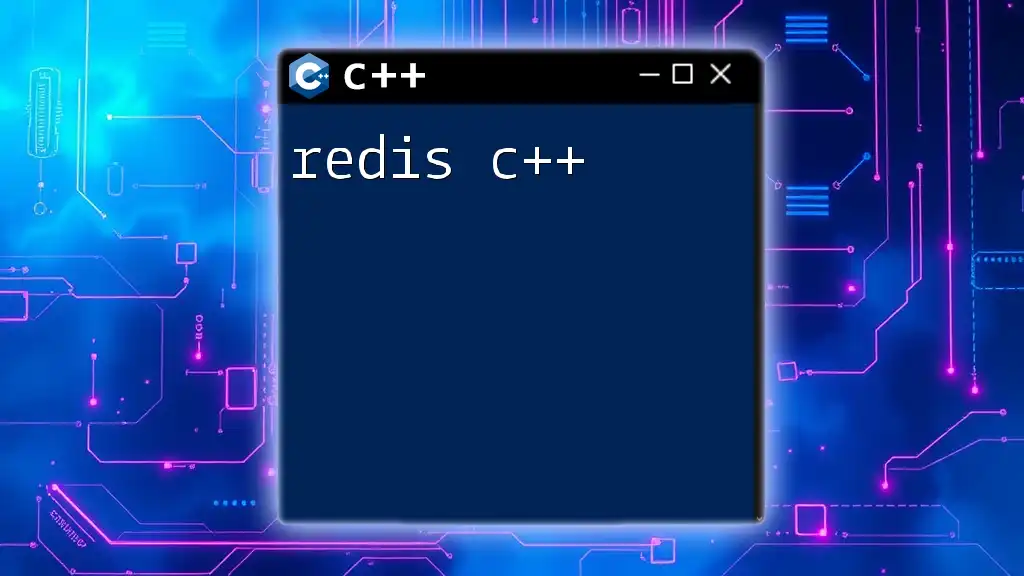
Finding Inspiration on Reddit
Highlighting Unique Projects
On Reddit, users often share innovative projects that can spark inspiration. By exploring these projects, you not only gain insight into real-world applications of C++ but also learn techniques and best practices you can apply to your projects.
Staying Updated with C++ Developments
Reddit is an excellent resource for staying informed about the latest trends in C++. New libraries, tools, and community initiatives often get significant attention on relevant subreddits, keeping you in the loop with the C++ ecosystem.
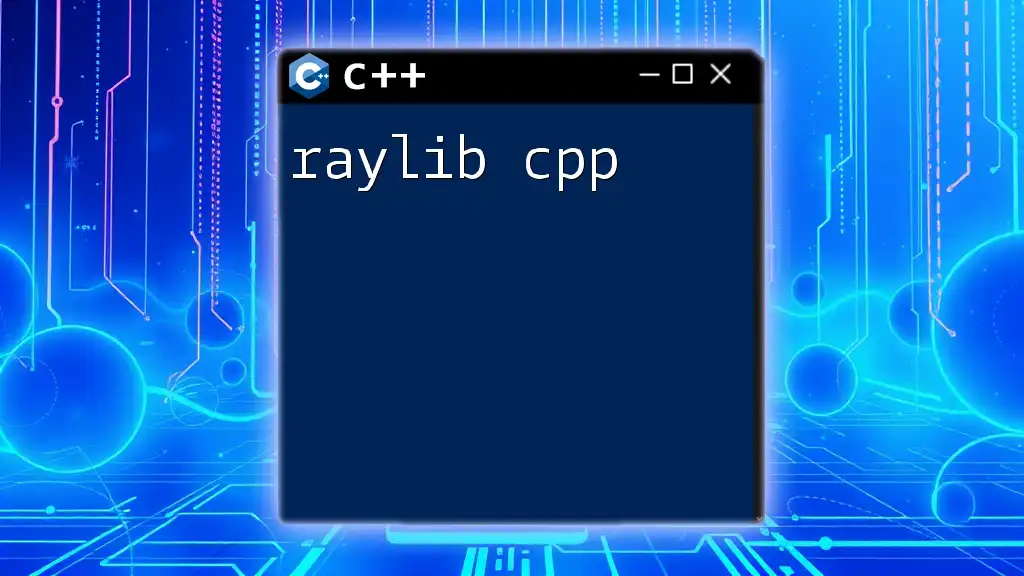
Conclusion
Utilizing Reddit for your C++ learning journey can yield tremendous benefits. By engaging with the community, sharing your own insights, and consistently exploring valuable resources, you will enhance your C++ skills in no time. Don’t hesitate to interact, ask questions, and contribute—the C++ community on Reddit thrives on collaboration and shared knowledge.
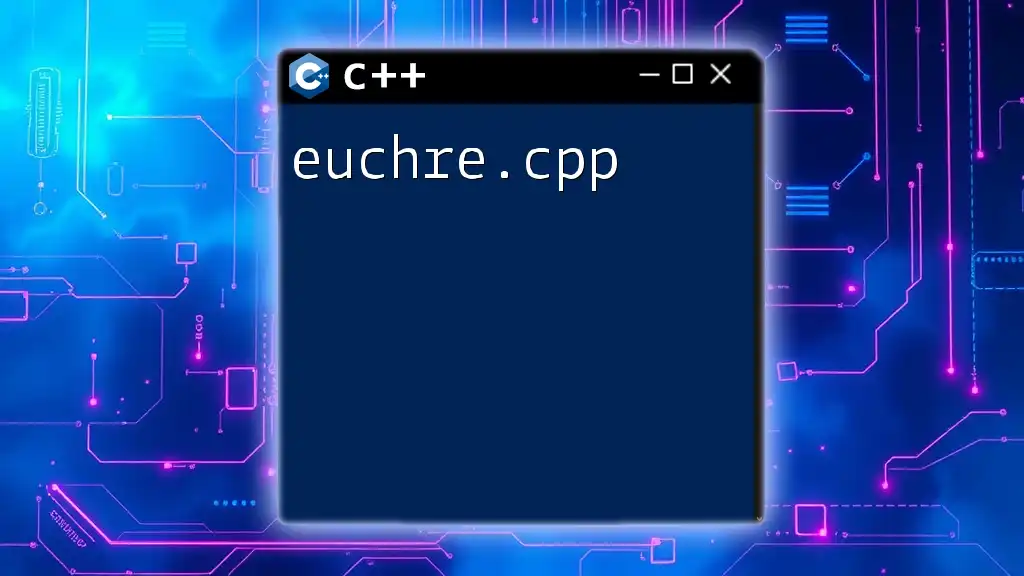
Call to Action
Join the relevant C++ subreddits, get involved in discussions, and don't forget to share your learning journey. Reddit is a powerful support system, ready to help you on your path to mastering C++ commands!
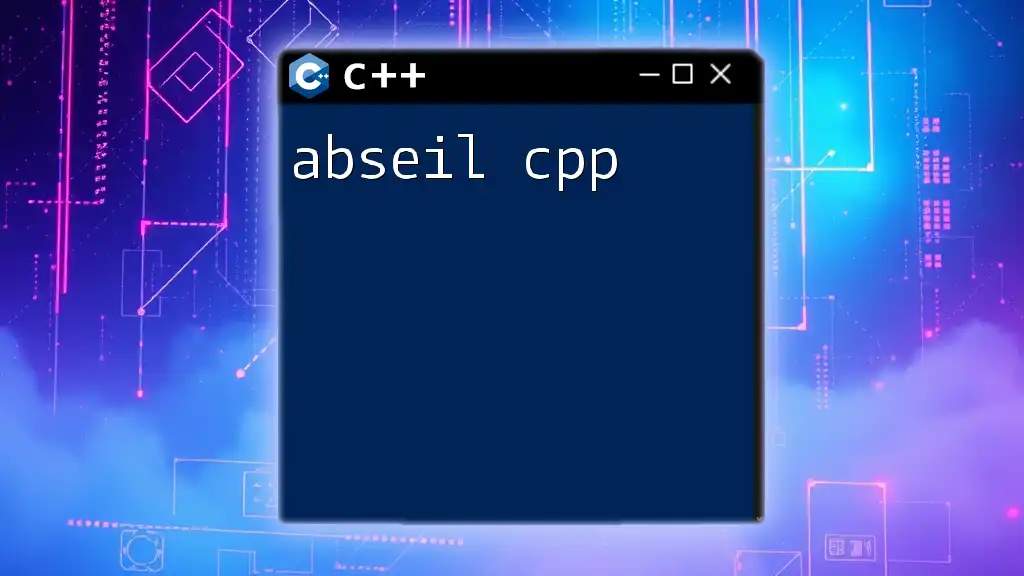
Additional Resources
To further enhance your knowledge, check out the recommended Reddit threads mentioned throughout this guide and consider exploring related articles or books focused on C++ programming.