Raylib is a simple and easy-to-use library for C++ that allows developers to create 2D and 3D games and graphics with minimal effort.
Here's a simple example to create a window using raylib:
#include "raylib.h"
int main() {
InitWindow(800, 600, "Hello Raylib!");
SetTargetFPS(60);
while (!WindowShouldClose()) {
BeginDrawing();
ClearBackground(RAYWHITE);
DrawText("Welcome to Raylib!", 190, 200, 20, LIGHTGRAY);
EndDrawing();
}
CloseWindow();
return 0;
}
Introduction to Raylib
What is Raylib?
Raylib is a simple and easy-to-use C library designed for game development. It provides a range of modules for handling graphics, input, audio, and much more. Its origin lies in creating a user-friendly experience, allowing developers to focus on making their games rather than wrestling with complex code.
Key features and benefits of using Raylib
- Ease of use: Raylib is aimed at beginners, which makes it a great choice for those new to game development.
- Modular design: The library is divided into multiple modules, enabling developers to include only what they need for their projects.
- Performance: Raylib is designed to be efficient, functioning well even with limited resources, making it suitable for both simple and complex games.
- Cross-platform: It supports many operating systems including Windows, Linux, and macOS, allowing for wide accessibility.
Why choose C++ for game development with Raylib?
Using C++ with Raylib grants developers access to object-oriented programming features, allowing for better organization within large projects. Additionally, C++ offers enhanced performance optimizations when compared to other languages, which is crucial in game development where speed is often a priority.
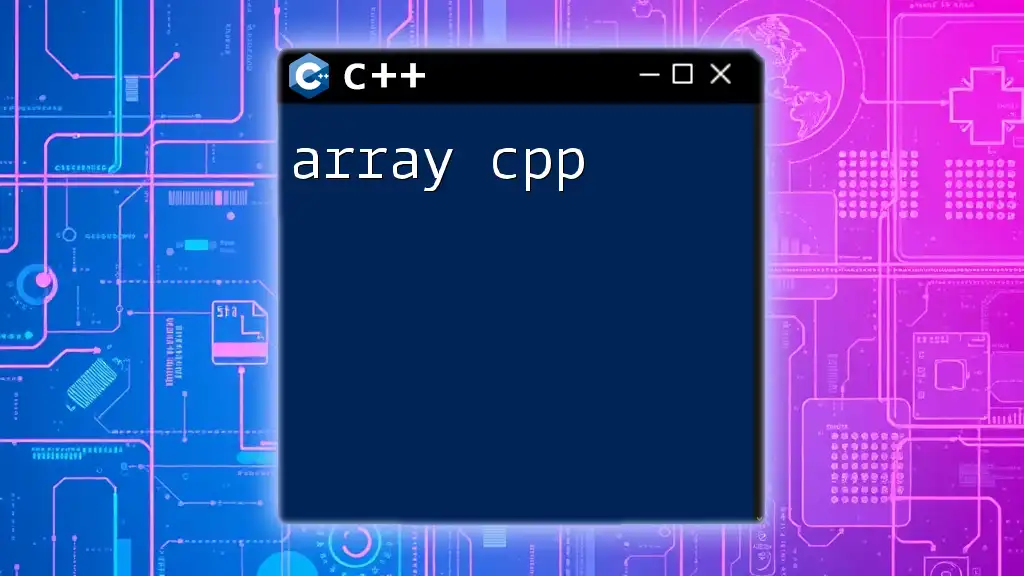
Setting Up Raylib with C++
Installation Requirements
Before delving into Raylib, your system should meet certain requirements based on your operating system:
- Windows: Visual Studio or MinGW.
- macOS: Xcode.
- Linux: GCC and relevant libraries.
Installation Steps
Setting up Raylib can often be done through package managers.
On Windows
Using vcpkg:
vcpkg install raylib
Alternatively, for MSYS2:
pacman -S mingw-w64-x86_64-raylib
On macOS
Using Homebrew:
brew install raylib
On Linux
For Debian-based systems:
sudo apt-get install libraylib-dev
Alternatively, on Fedora:
sudo dnf install raylib raylib-devel
Configuring Your IDE
To ensure that Raylib works seamlessly within your development environment, configuring your IDE correctly is crucial. For example, in Visual Studio, you'd create a new project, link the Raylib library in your project settings, and add the necessary include directories.
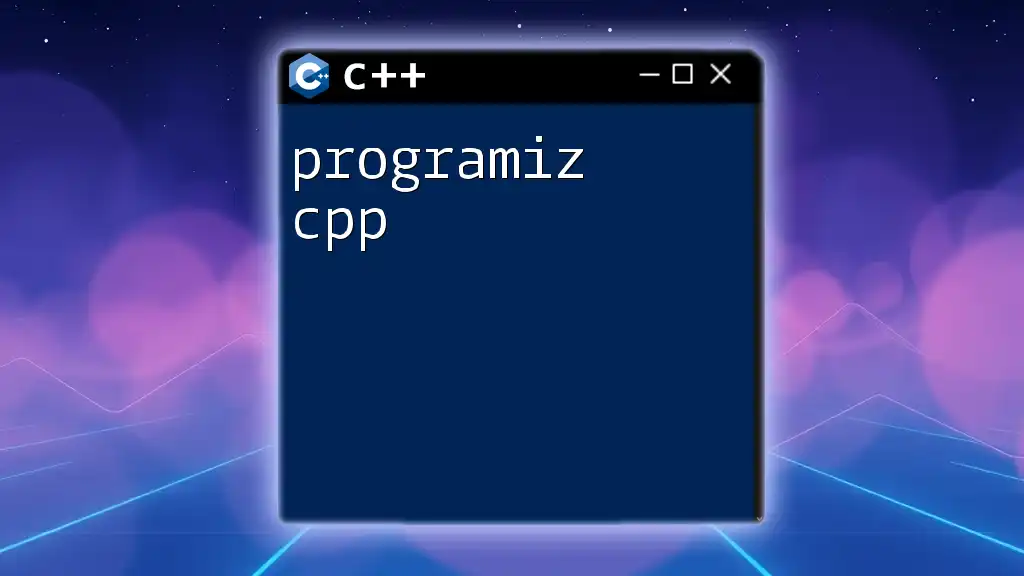
Basic Concepts in Raylib
Understanding 2D/3D graphics
Raylib excels in both 2D and 3D graphics rendering. Understanding the difference between the two is important:
- 2D graphics: Focused on rendering flat surfaces and colors, operations typically involve modifying bitmap images.
- 3D graphics: Involves dealing with vertices, lighting, and more complex transformations.
Raylib Structure
Raylib functions and objects are intuitively defined. At the core of any Raylib program lies the main game loop. Below is a basic skeleton of a Raylib program:
#include "raylib.h"
int main(void)
{
// Initialization
InitWindow(800, 600, "Raylib C++ Example");
// Game loop
while (!WindowShouldClose())
{
BeginDrawing();
ClearBackground(RAYWHITE);
DrawText("Welcome to Raylib!", 190, 200, 20, LIGHTGRAY);
EndDrawing();
}
// De-Initialization
CloseWindow();
return 0;
}
This example showcases the essential structure where you initialize the window, enter a game loop for rendering, and finally clean up before exiting.
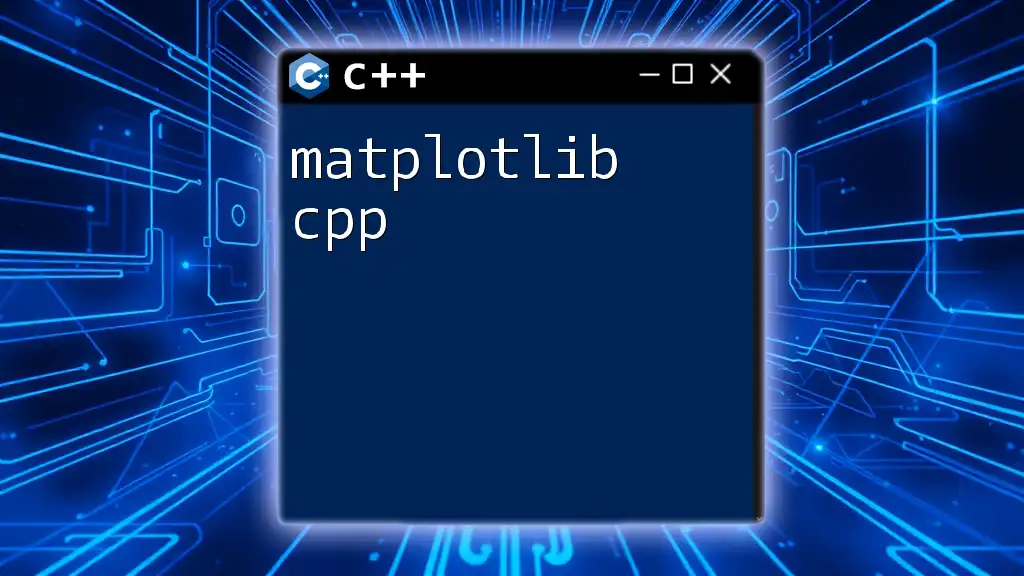
Drawing Shapes and Text
Drawing 2D Shapes
Raylib comes with a variety of functions to draw basic shapes. For instance:
- Rectangles can be drawn using `DrawRectangle()`.
- Circles can be rendered with `DrawCircle()`.
Here’s a simple example of drawing shapes in Raylib:
BeginDrawing();
ClearBackground(RAYWHITE);
DrawRectangle(200, 150, 200, 100, BLUE);
DrawCircle(400, 300, 50, RED);
EndDrawing();
Displaying Text
Displaying text effectively enhances the user experience. The function `DrawText()` allows you to render text on the screen with chosen formatting options:
DrawText("Hello, Raylib!", 350, 250, 20, DARKGRAY);
This will display a text string at coordinates (350, 250) with a font size of 20 in gray.
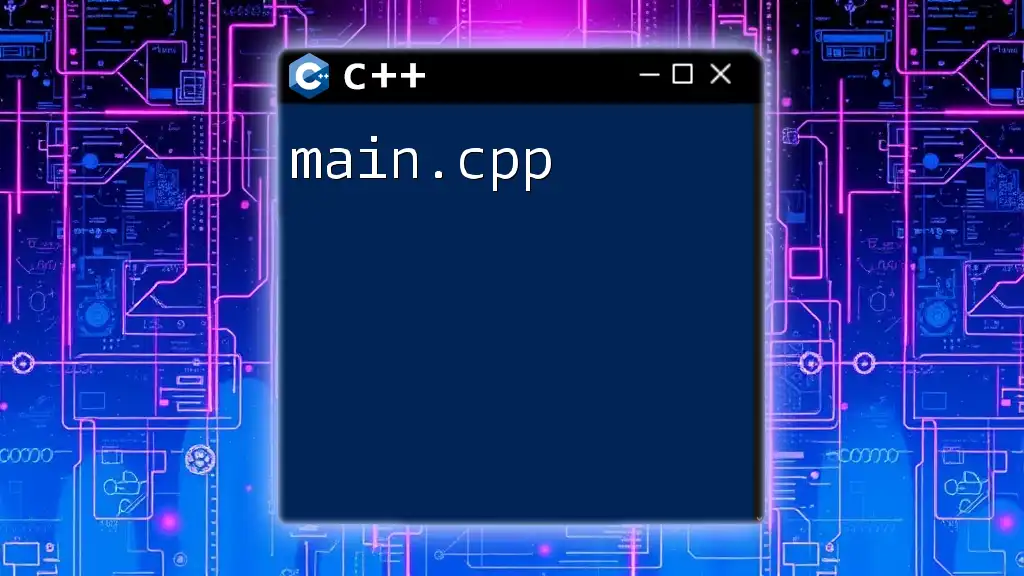
Working with Images and Textures
Loading Images
Images can be easily loaded into a Raylib application using the `LoadImage()` and `LoadTexture()` functions. Here’s how you can load and display an image:
Image image = LoadImage("my_image.png");
Texture2D texture = LoadTextureFromImage(image);
UnloadImage(image);
BeginDrawing();
ClearBackground(RAYWHITE);
DrawTexture(texture, 0, 0, WHITE);
EndDrawing();
UnloadTexture(texture);
Ensure your image is in a compatible format like PNG or JPG.
Texture Manipulation
Textures can undergo transformations during rendering. To draw a `Texture` at a specific position:
DrawTexture(texture, xPosition, yPosition, WHITE);
For sprite animations, you can adjust the texture rectangle parameters, effectively displaying only a portion of the texture.
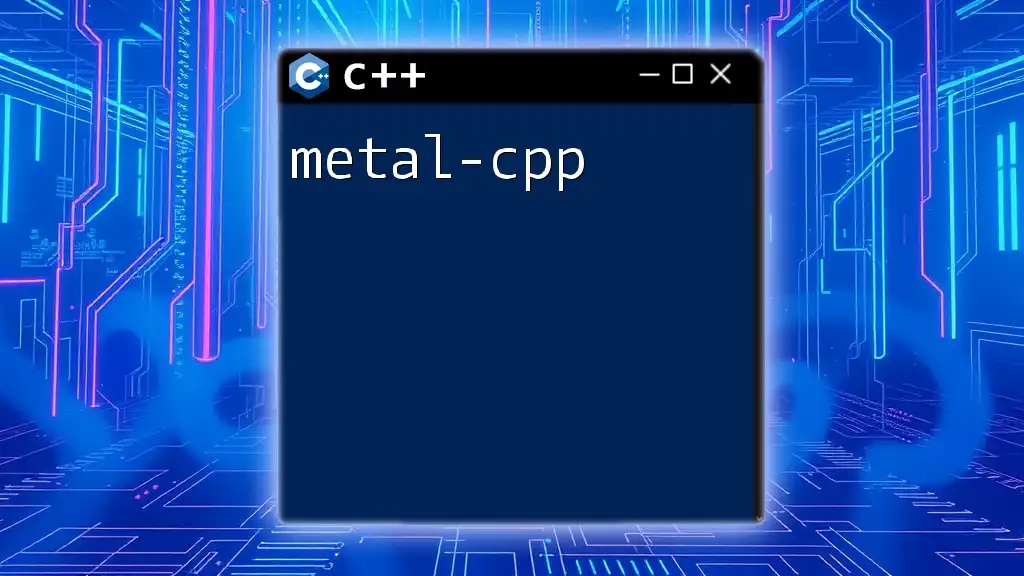
Implementing User Input
Handling Keyboard and Mouse Input
Responding to user input is critical in gaming. Raylib provides functions to capture keyboard and mouse events. For example:
if (IsKeyPressed(KEY_SPACE))
{
// Code for what happens when the space key is pressed
}
To handle mouse input:
if (IsMouseButtonDown(MOUSE_LEFT_BUTTON))
{
// Code for what happens when left mouse is clicked
}
Creating Interactive Objects
Here’s a simple WASD control example for moving a character on-screen:
Vector2 position = { 400, 300 };
if (IsKeyDown(KEY_W)) position.y -= 2;
if (IsKeyDown(KEY_S)) position.y += 2;
if (IsKeyDown(KEY_A)) position.x -= 2;
if (IsKeyDown(KEY_D)) position.x += 2;
DrawTexture(character, position.x, position.y, WHITE);
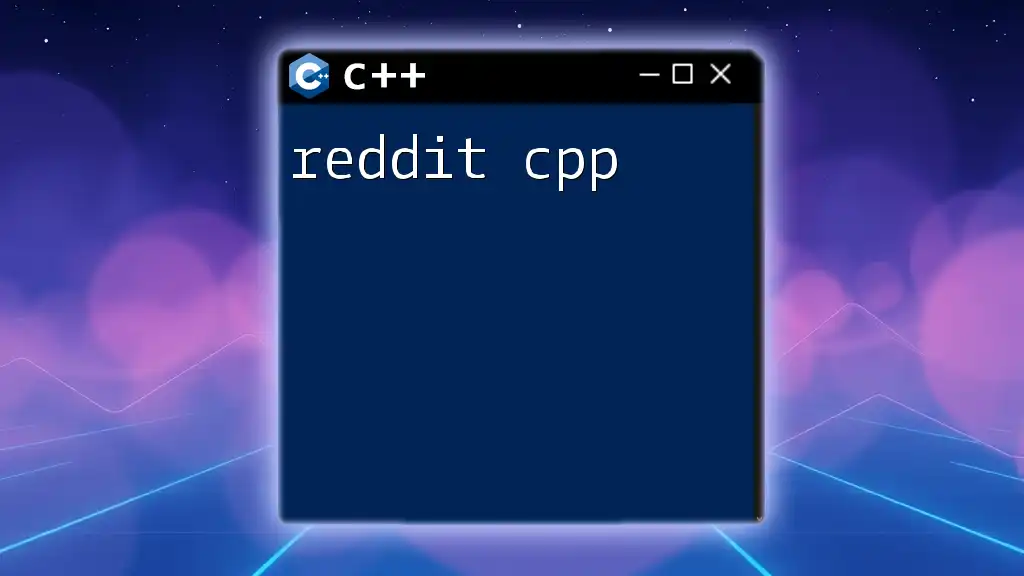
Adding Music and Sound Effects
Loading Audio Files
Raylib supports various audio formats. Load sound with `LoadSound()`, followed by playing it with:
Sound sound = LoadSound("sound_effect.wav");
PlaySound(sound);
Remember that managing audio formats is important for compatibility.
Managing Music Playback
For background music, utilize the `LoadMusicStream()` function:
Music music = LoadMusicStream("background_music.mp3");
PlayMusicStream(music);
In the game loop, call `UpdateMusicStream(music);` to ensure smooth playback.
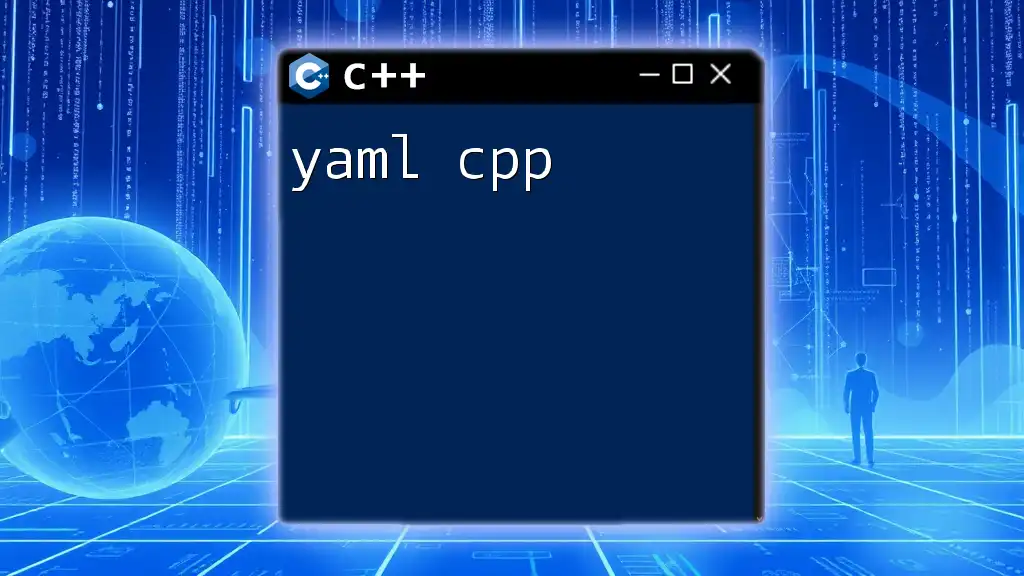
Advanced Topics
Handling Game State
A game often needs different states for menus, gameplay, or game over scenarios. You can manage state by using an enumerated type:
enum GameState {MENU, PLAYING, GAME_OVER};
GameState currentState = MENU;
Switch based on state to render appropriate components.
Using 3D Models
Loading 3D models is straightforward. Raylib supports formats like OBJ. Use the following code snippet to render a model:
Model model = LoadModel("model.obj");
while (!WindowShouldClose())
{
BeginDrawing();
ClearBackground(RAYWHITE);
DrawModel(model, (Vector3){0, 0, 0}, 1.0f, WHITE);
EndDrawing();
}
UnloadModel(model);
Matrix transformations allow rotating or translating models effectively.
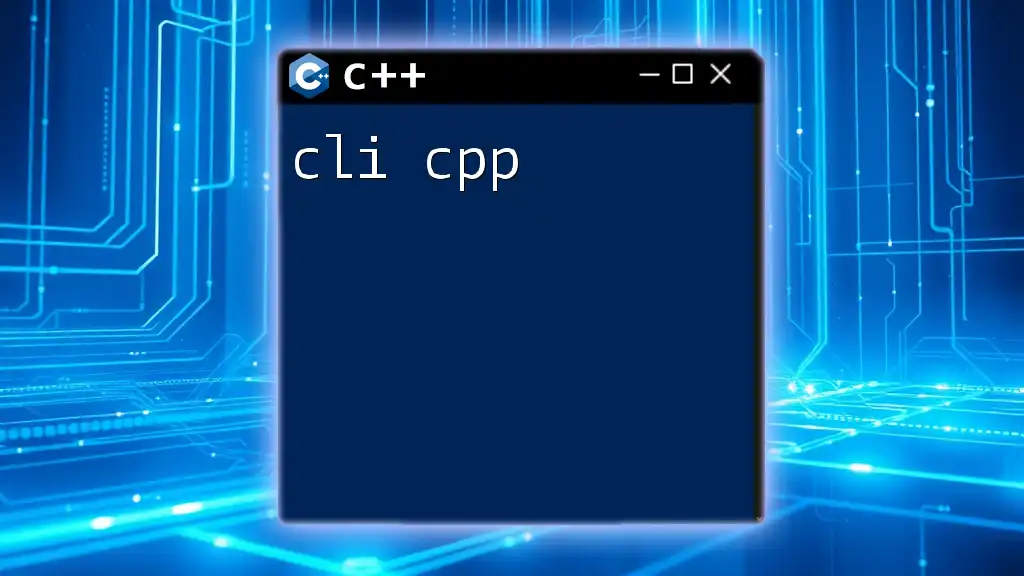
Raylib Resources and Community
Official Documentation
The [Raylib official website](https://raylib.com) is a treasure trove of information where you can find extensive documentation, tutorials, and source code examples. This resource is invaluable for understanding Raylib's full capabilities.
Community and Learning Resources
Getting involved with the community can enhance your learning experience exponentially. Raylib has an active user base and forums where you can ask questions. Joining Discord channels or browsing GitHub repositories dedicated to Raylib can provide inspiration and guidance.
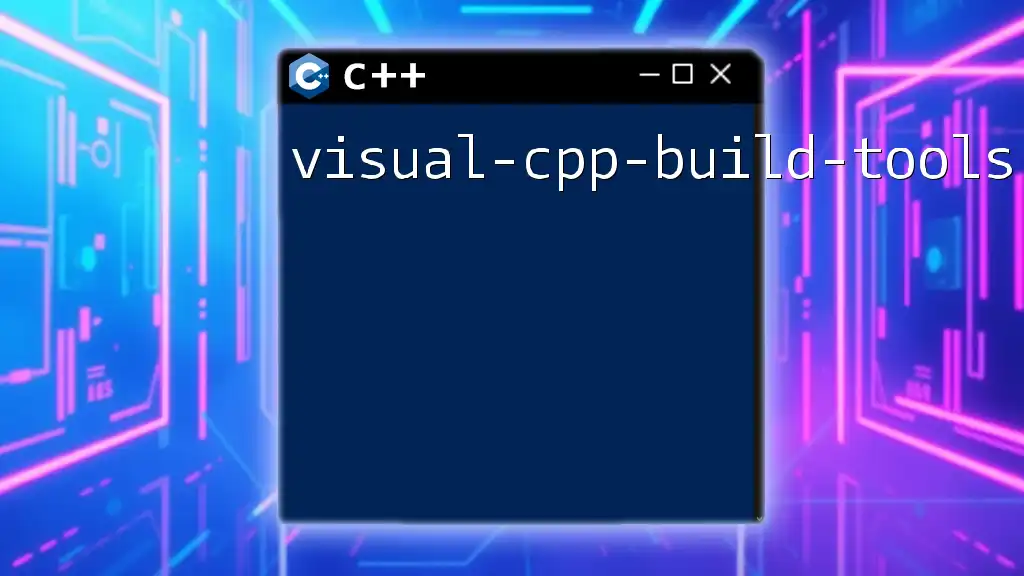
Conclusion
Raylib C++ offers a rich and simple toolkit for creating engaging games. By following this guide, developers can quickly become familiar with the fundamental strategies involved in using Raylib effectively.
Experimentation and creativity are key in your journey, and don’t hesitate to share your progress with the community as you embark on building your games. Happy coding!
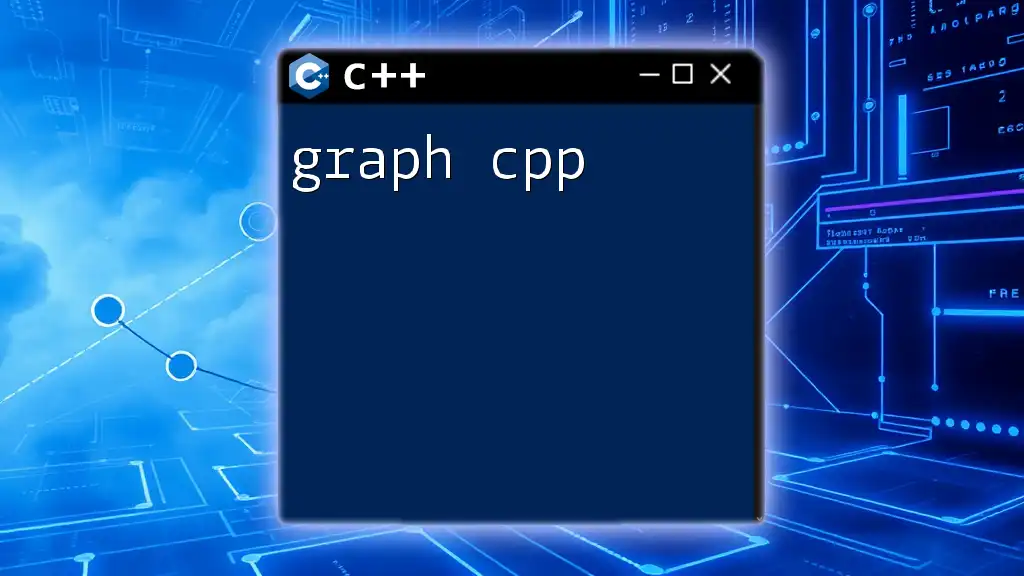
Appendix
A Glossary of Common Terms
Familiarizing yourself with common game development terms like sprites, texturing, and game loops will enhance your understanding and capability in using Raylib effectively.
Additional Example Projects
For inspiration, consider exploring various projects shared within the Raylib community that showcase its versatility, including platformers, puzzle games, and simulations. Links to these resources will be beneficial for further learning.
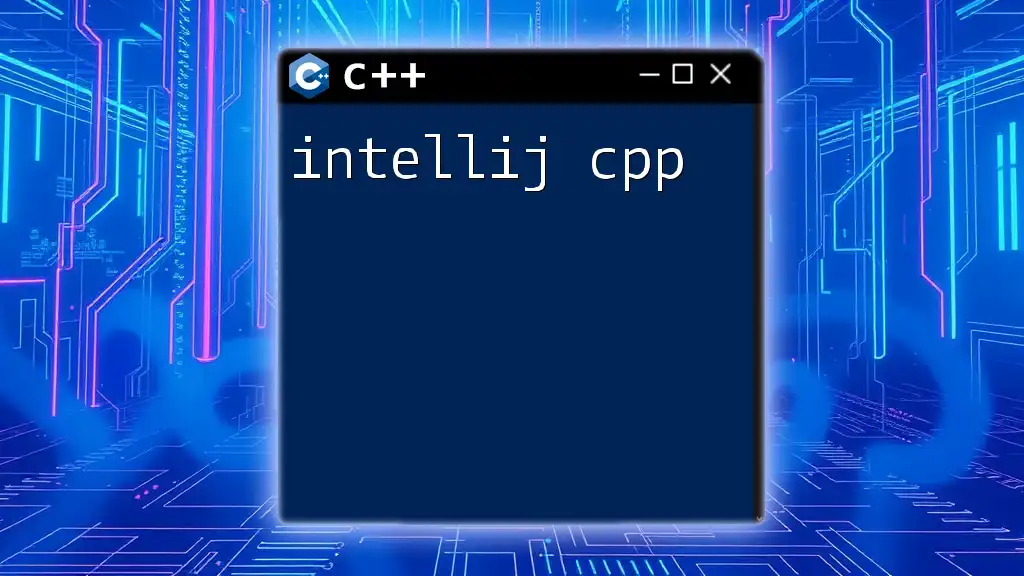
Final Thoughts
Continued learning and practice are the best ways to mastery. As you explore Raylib, leverage both its power and simplicity to let your ideas flourish. Don’t hesitate to reach out to the community for feedback or guidance.