In C++, you can create a new array using the array type with a specified size, such as in the example below:
int myArray[5]; // This declares an array of integers with 5 elements.
Understanding the New Operator in C++
What is the New Operator?
The `new` operator in C++ is a powerful tool for dynamic memory allocation, allowing you to create arrays or single variables whose size is determined at runtime. Unlike stack memory, where variables are allocated and deallocated automatically, memory allocated with the `new` operator resides in the heap until explicitly released. This gives you greater control over memory usage—an essential feature in high-performance or memory-constrained applications.
How New Works with Arrays
When dealing with arrays, the `new` operator enables us to allocate a block of memory large enough to hold multiple elements of a specified type. The syntax for allocating a new array involves identifying the data type and the desired number of elements.
The general syntax is as follows:
dataType *arrayName = new dataType[size];
Example: Allocating a new array
int *myArray = new int[10]; // Allocates an array of 10 integers
In this example, an array capable of holding ten integers is created on the heap, which is a good illustration of how `new array cpp` works in practice.
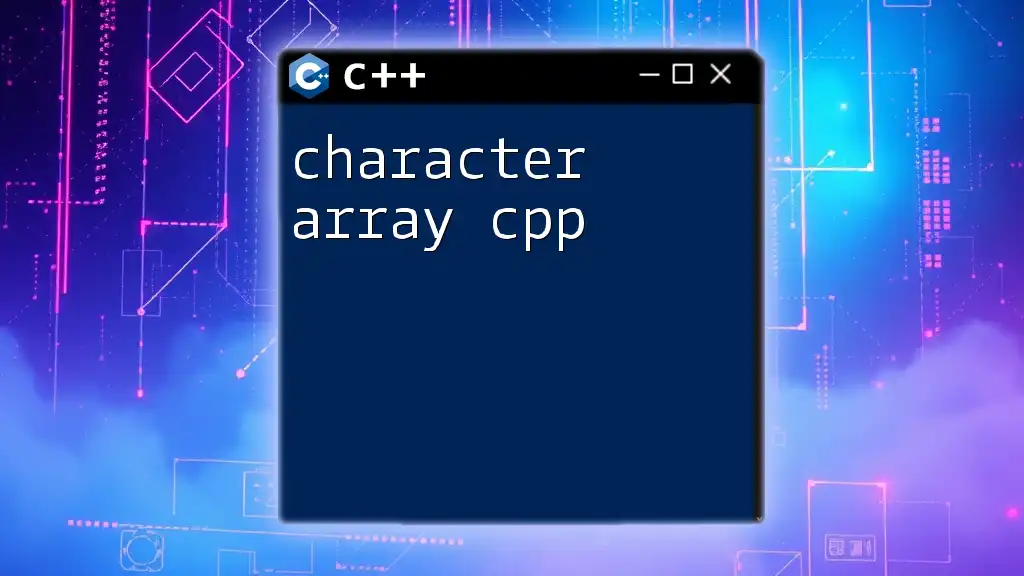
Creating a New Array using the New Operator
Basic Syntax for New Arrays
The basic syntax is straightforward. By defining the data type, we can declare an array name and specify its size.
double *scores = new double[5]; // Allocates an array of 5 doubles
Initializing New Arrays
Array elements can be initialized during allocation or afterward. If you do not initialize them, the values in the array will be undefined.
-
Default Initialization: If you do not specify initial values, garbage values will be present in the array.
int *defaultArray = new int[3]; // Contains garbage values
-
Custom Initialization: You can initialize the array elements using a loop after allocation.
int *initArray = new int[3]; for (int i = 0; i < 3; i++) { initArray[i] = i * 10; // Initializes values to 0, 10, and 20 }
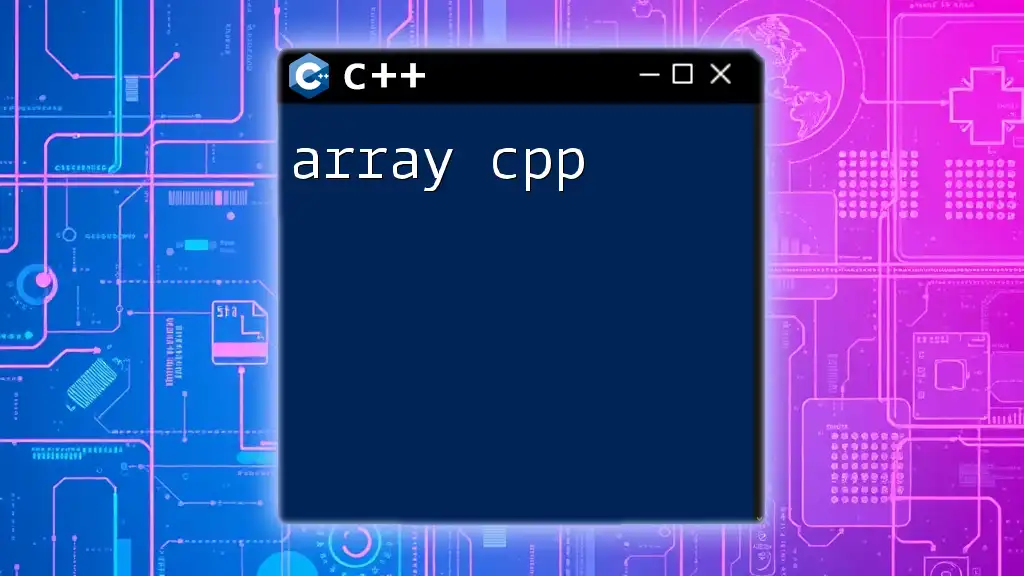
Memory Management with New Arrays
Releasing Memory using Delete
One of the critical aspects of working with dynamic memory in C++ is ensuring that you release it appropriately using the `delete` operator. Failing to do so can lead to memory leaks, where memory that is no longer in use remains allocated, consuming resources unnecessarily.
To release an array, use:
delete[] arrayName;
Example: Safe deallocation of an array
delete[] myArray; // Frees the memory allocated to myArray
Common Pitfalls
When using the `delete` operator, be cautious not to mistakenly delete the array with just `delete`, which is intended for single objects. Proper deletion is essential for preventing undefined behavior and memory leaks.
Memory leaks can occur if you forget to free memory that you no longer need. Therefore, remember to call `delete[]` for all arrays you create with `new`.
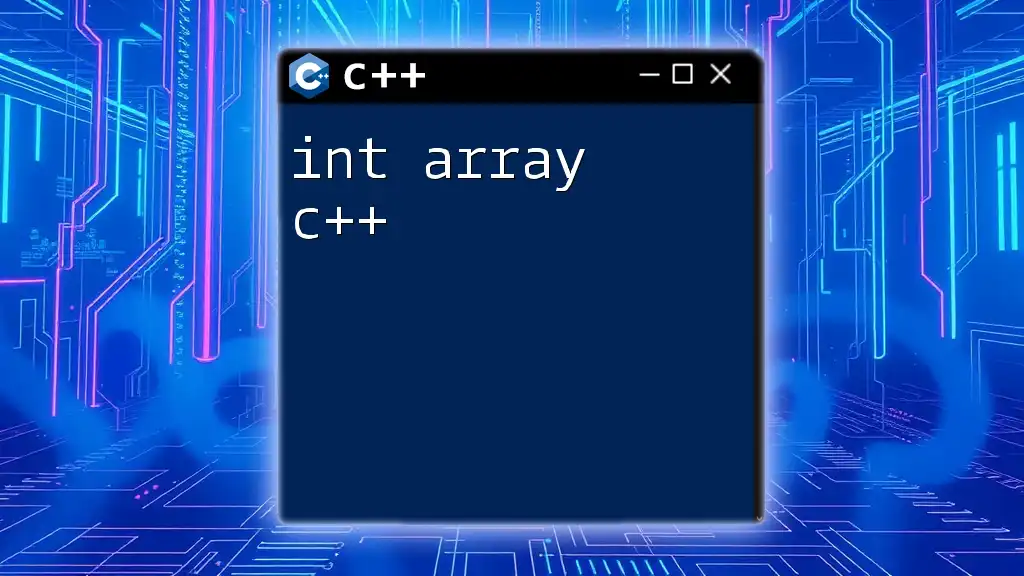
Accessing and Modifying Elements in New Arrays
Accessing Array Elements
Once an array has been allocated with the `new` operator, accessing its elements is as simple as using index notation. C++ arrays are zero-indexed, meaning that the first element is accessed with the index 0.
Example: Accessing and displaying elements in a new array
for (int i = 0; i < 5; i++) {
std::cout << scores[i] << std::endl; // Displays the values in the scores array
}
Modifying Array Elements
You can easily modify elements in a dynamic array using the same indexing.
Example: Modifying existing values and displaying changes
scores[0] = 10.5; // Sets the first element to 10.5
scores[1] = 20.0; // Sets the second element to 20.0
for (int i = 0; i < 2; i++) {
std::cout << scores[i] << std::endl; // Displays modified values
}
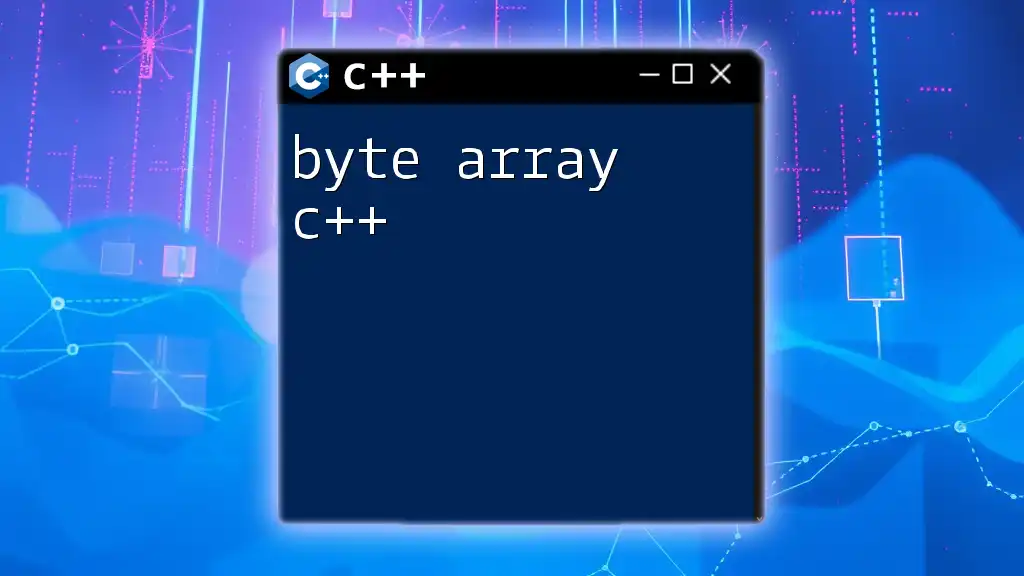
Best Practices for Using New Arrays in C++
When to Use New Arrays
Dynamic arrays with the `new` operator can be incredibly beneficial when dealing with variable-sized data structures, especially when the size is only known at runtime. Use new arrays when performance is critical, and you need greater flexibility in managing memory.
Alternatives to New Arrays
While new arrays serve a specific purpose, it's worth noting that modern C++ provides additional options like `std::vector`, which is part of the Standard Template Library (STL). Vectors handle memory management automatically, and they can dynamically resize as needed.
Pros of Using `std::vector`:
- Automatic memory management.
- Simplified syntax for addition and deletion.
- Enhanced safety with better bounds checking.
Cons:
- Slightly more overhead compared to raw pointers.
If simplicity and safety are a priority, consider using `std::vector` over raw dynamic arrays.
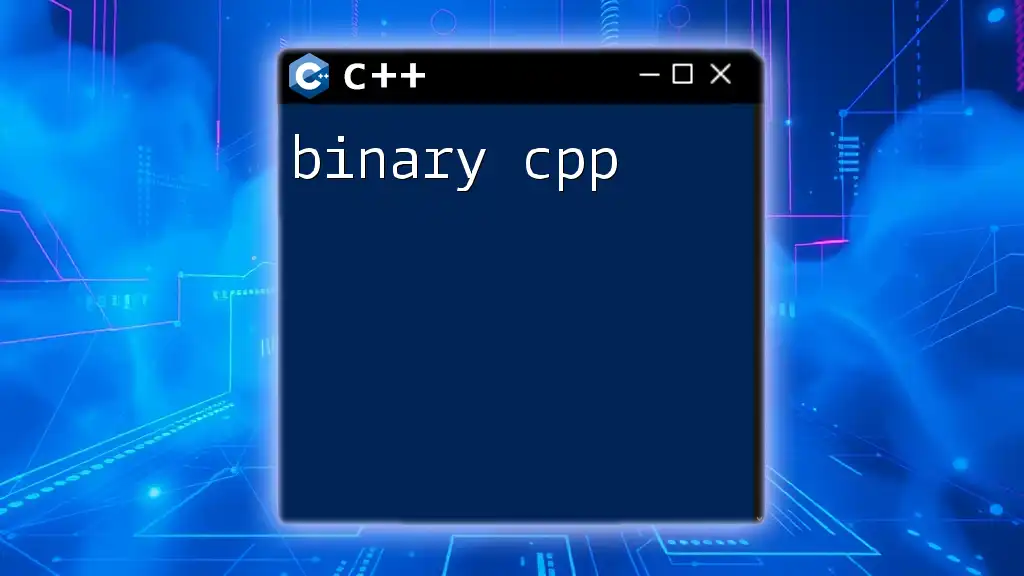
Common Errors and Troubleshooting
Common Mistakes with New Arrays
Some prevalent errors while working with new arrays in C++ include:
- Memory leaks: Forgetting to call `delete[]`.
- Accessing out of bounds: This can lead to undefined behavior.
- Incorrect deletion: Using `delete` instead of `delete[]` for arrays.
Debugging Tips
Utilizing tools like Valgrind or AddressSanitizer can help detect memory leaks and access errors. Learning how to properly use these tools can significantly improve your debugging process and memory management techniques in C++.
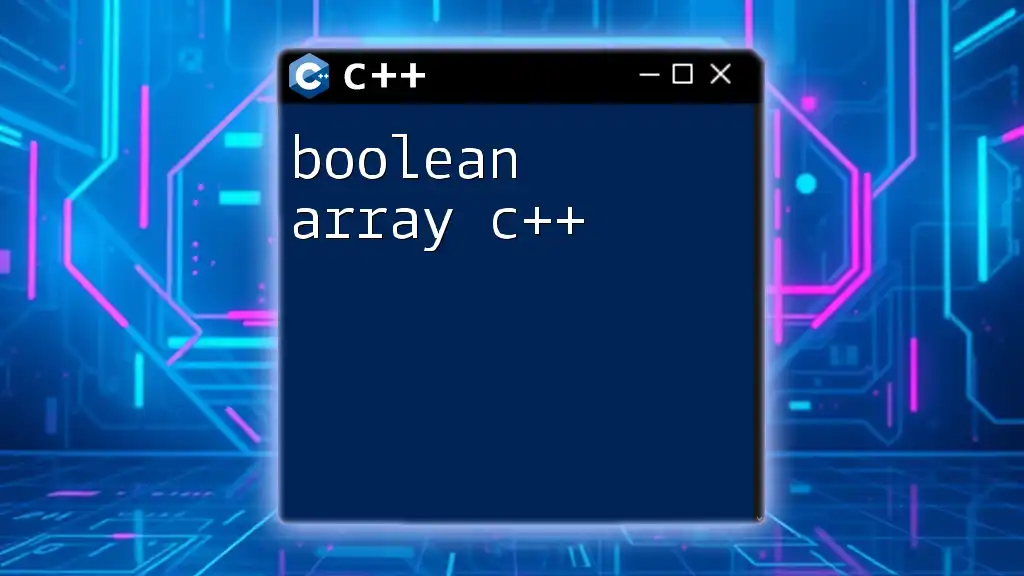
Conclusion
In summation, understanding how to effectively use dynamic arrays with the `new` operator is crucial in C++. By mastering the allocation, initialization, and management of new arrays, you will have a powerful tool at your disposal for handling collections of data. As you gain experience, consider transitioning to more advanced data structures like `std::vector`, which offer numerous benefits. Strive to practice regularly and implement what you’ve learned in projects to achieve mastery over C++ arrays.
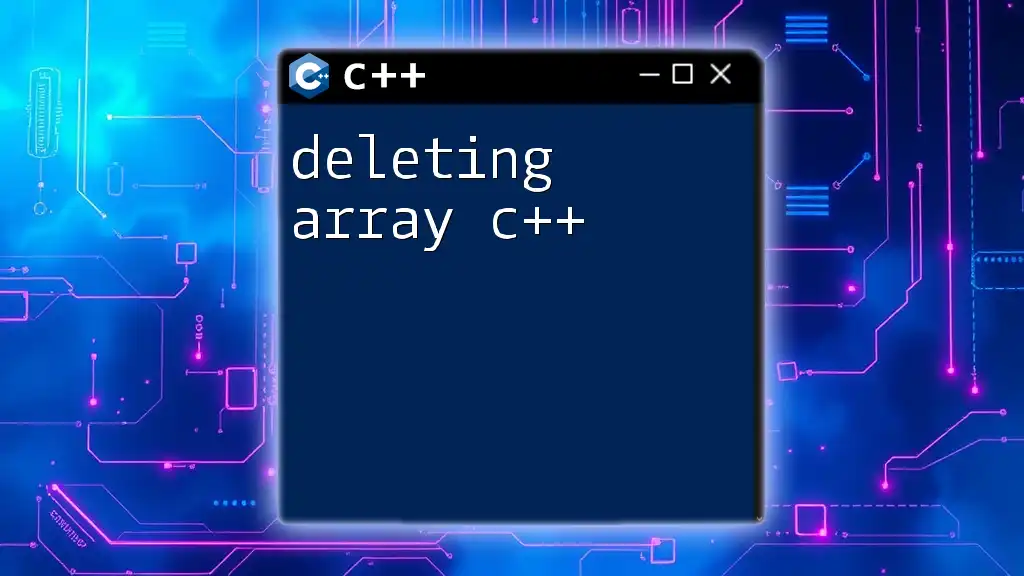
Additional Resources
To deepen your understanding, refer to recommended readings or explore online coding platforms where you can experiment with the code snippets provided in this guide. The world of C++ offers endless opportunities for growth and the development of your programming skills.