Here's a simple C++ code snippet that displays the current date using the `<chrono>` and `<iomanip>` libraries:
#include <iostream>
#include <chrono>
#include <iomanip>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t now_c = std::chrono::system_clock::to_time_t(now);
std::cout << "Current date: " << std::put_time(std::localtime(&now_c), "%Y-%m-%d") << std::endl;
return 0;
}
Getting Started with Calendar CPP
Setting Up Your Development Environment
To efficiently work with Calendar CPP, it's essential to set up your development environment correctly. Consider using widely recommended IDEs such as Visual Studio, Code::Blocks, or CLion. Once you have your IDE up and running, make sure you have access to the necessary libraries like <chrono> and <iomanip> for handling date and time.
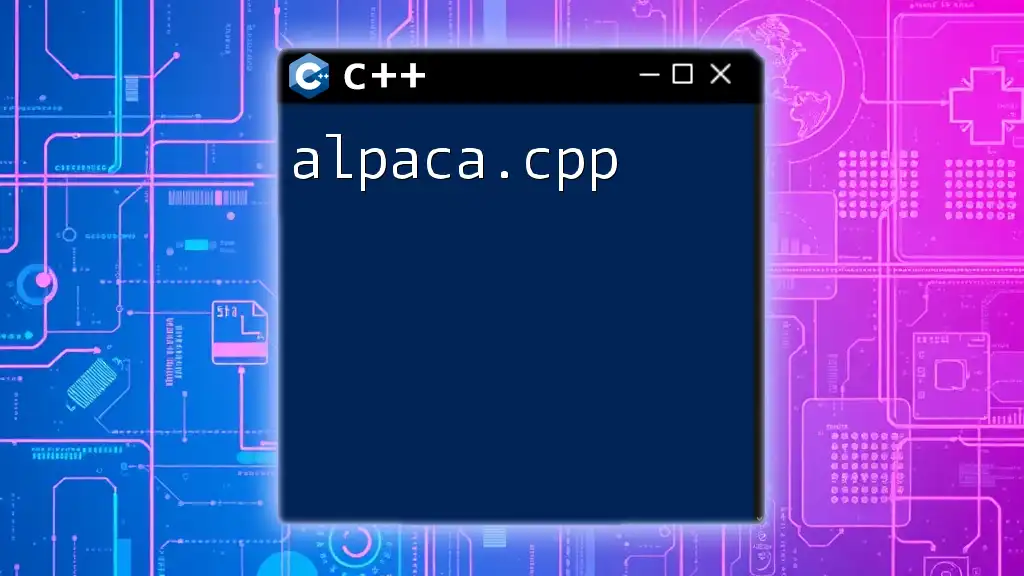
Key Concepts in Calendar CPP
Understanding Date and Time
Grasping the fundamental concepts of date and time is vital when working with Calendar CPP. Key aspects include time zones (the geographical regions with the same standard time), UTC (Coordinated Universal Time, which is the primary time standard), and local time (time adjusted for your specific location). Distinguishing between timepoints (specific points in time) and durations (the length of time between two timepoints) will aid you in utilizing date-related functions effectively.
The `chrono` Library in C++
The chrono library is a powerful tool in C++ for handling time. It offers various features to represent time points, durations, and clocks. Understanding this library is crucial for performing complex date manipulations needed in calendar functionalities.
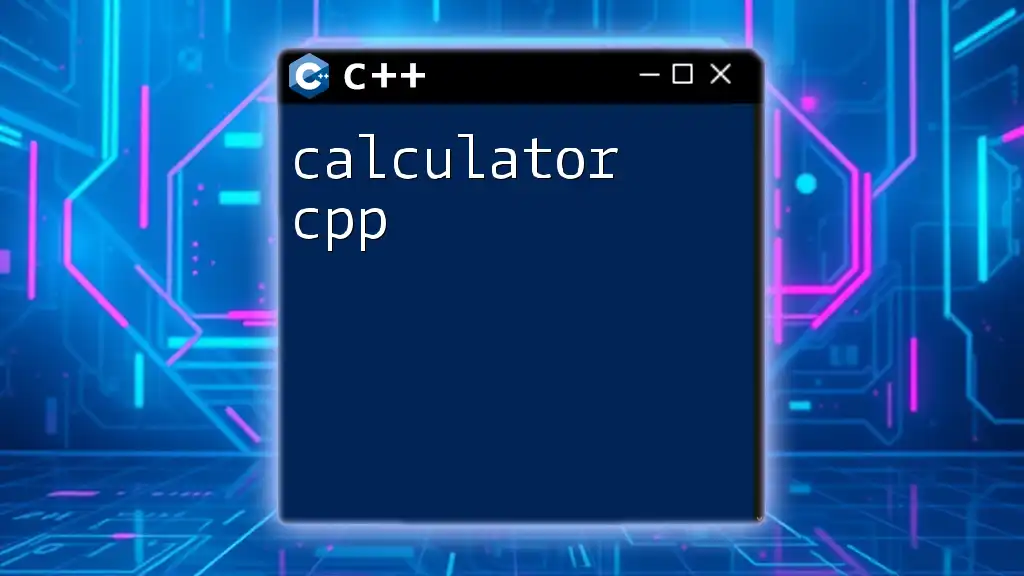
Basic Calendar Operations
Creating a Date
To create a date, you can use the following code snippet to get the current date and time:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t now_c = std::chrono::system_clock::to_time_t(now);
std::cout << "Current date and time: " << std::ctime(&now_c);
return 0;
}
This code utilizes `std::chrono::system_clock::now()` to acquire the current date and time. It converts it into a `time_t` object before printing, providing a readable format.
Formatting Dates
Formatting dates to human-readable strings is essential. You can manipulate the output format using `std::put_time`. Here’s an example:
#include <iomanip>
#include <iostream>
#include <chrono>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t now_c = std::chrono::system_clock::to_time_t(now);
std::cout << "Formatted date and time: "
<< std::put_time(std::localtime(&now_c), "%Y-%m-%d %H:%M:%S") << std::endl;
return 0;
}
This code formats the date and time as YYYY-MM-DD HH:MM:SS. Understand how each part of the format string affects the output, as this is crucial for tailoring date displays to user needs.
Calculating Date Differences
Understanding how to calculate the difference between two dates can be extremely beneficial. Use the following code snippet:
#include <iostream>
#include <chrono>
int main() {
std::chrono::system_clock::time_point start = std::chrono::system_clock::now();
// Simulate some process
std::this_thread::sleep_for(std::chrono::seconds(2)); // Sleep for 2 seconds
std::chrono::system_clock::time_point end = std::chrono::system_clock::now();
std::chrono::duration<double> elapsed_seconds = end - start;
std::cout << "Elapsed time: " << elapsed_seconds.count() << " seconds" << std::endl;
return 0;
}
This example subtracts the start time from the end time to compute the elapsed seconds. It demonstrates how to measure performance and timing in programs effectively.
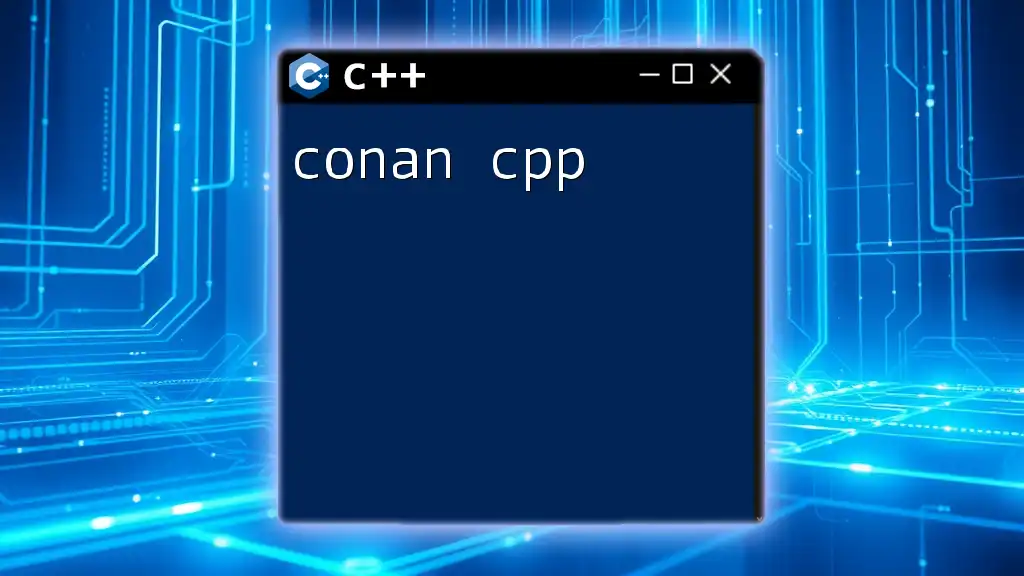
Advanced Calendar Operations
Date Arithmetic
Building on basic operations, date arithmetic allows you to add or subtract days, months, or years. The following code snippet illustrates adding days:
#include <iostream>
#include <chrono>
int main() {
auto today = std::chrono::system_clock::now();
auto tomorrow = today + std::chrono::hours(24); // Add 24 hours for next day
std::time_t tomorrow_c = std::chrono::system_clock::to_time_t(tomorrow);
std::cout << "Tomorrow's date: " << std::put_time(std::localtime(&tomorrow_c), "%Y-%m-%d %H:%M:%S") << std::endl;
return 0;
}
This code snippet adds 24 hours to the current date, yielding the date for tomorrow. However, keep in mind the complexities involved in adding months or years due to varying month lengths and leap years.
Creating Custom Date Structures
If your application requires specific functionality, it may be beneficial to create custom date structures. Here is an example:
#include <iostream>
struct CustomDate {
int day;
int month;
int year;
bool isValid() {
// Simple validation (you can expand upon this)
return (day > 0 && day <= 31 && month > 0 && month <= 12);
}
};
int main() {
CustomDate myDate = {29, 2, 2020};
if (myDate.isValid()) {
std::cout << "Valid date: " << myDate.day << "/" << myDate.month << "/" << myDate.year << std::endl;
} else {
std::cout << "Invalid date!" << std::endl;
}
return 0;
}
The above code defines a CustomDate struct with a validation method, helping manage specific date formats and conditions.
Handling Leap Years and Special Cases
Leap years, occurring every four years (except for century years not divisible by 400), can complicate date calculations. You can identify leap years using the following code:
#include <iostream>
bool isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int main() {
int year = 2024; // Example year
if (isLeapYear(year)) {
std::cout << year << " is a leap year." << std::endl;
} else {
std::cout << year << " is not a leap year." << std::endl;
}
return 0;
}
This function checks whether the provided year is a leap year, an essential check when calculating dates in February.
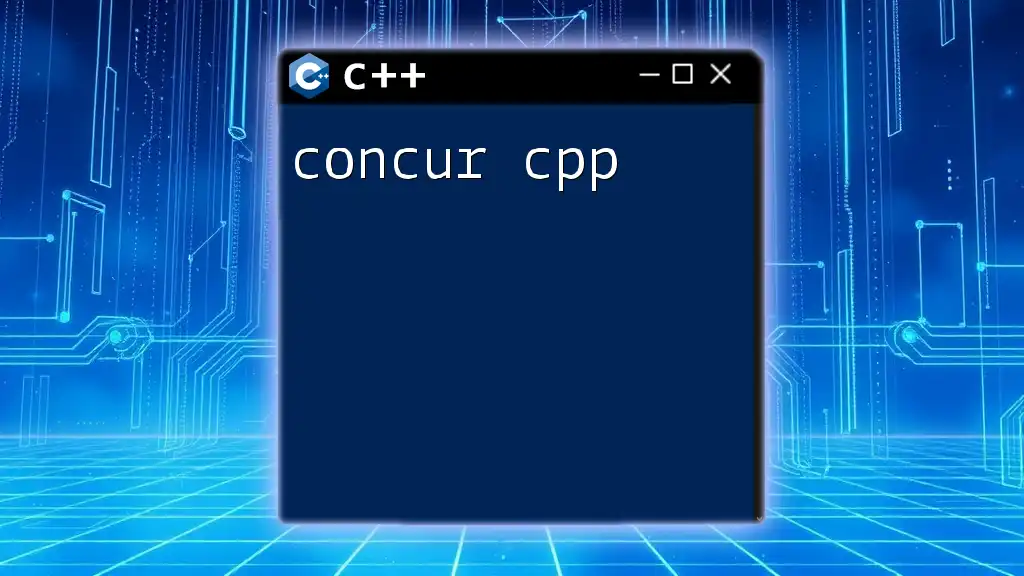
Scheduling and Events Management
Building a Simple Event Scheduler
Using the principles learned, you can develop a simple event scheduler. Below is an example of an event class managing start and end times:
#include <iostream>
#include <chrono>
#include <string>
class Event {
public:
std::chrono::system_clock::time_point start;
std::chrono::system_clock::time_point end;
std::string name;
Event(const std::string& eventName, const std::chrono::system_clock::time_point& startTime, const std::chrono::system_clock::time_point& endTime)
: name(eventName), start(startTime), end(endTime) {}
void displayEvent() {
std::time_t start_c = std::chrono::system_clock::to_time_t(start);
std::time_t end_c = std::chrono::system_clock::to_time_t(end);
std::cout << "Event: " << name << "\nStart: " << std::ctime(&start_c) << "End: " << std::ctime(&end_c) << std::endl;
}
};
int main() {
Event myEvent("Birthday Party", std::chrono::system_clock::now(), std::chrono::system_clock::now() + std::chrono::hours(2));
myEvent.displayEvent();
return 0;
}
This `Event` class stores a name and the start and end time of the event, displaying them neatly. When developing applications like calendars or reminders, consider managing overlapping events as a complex but necessary feature.
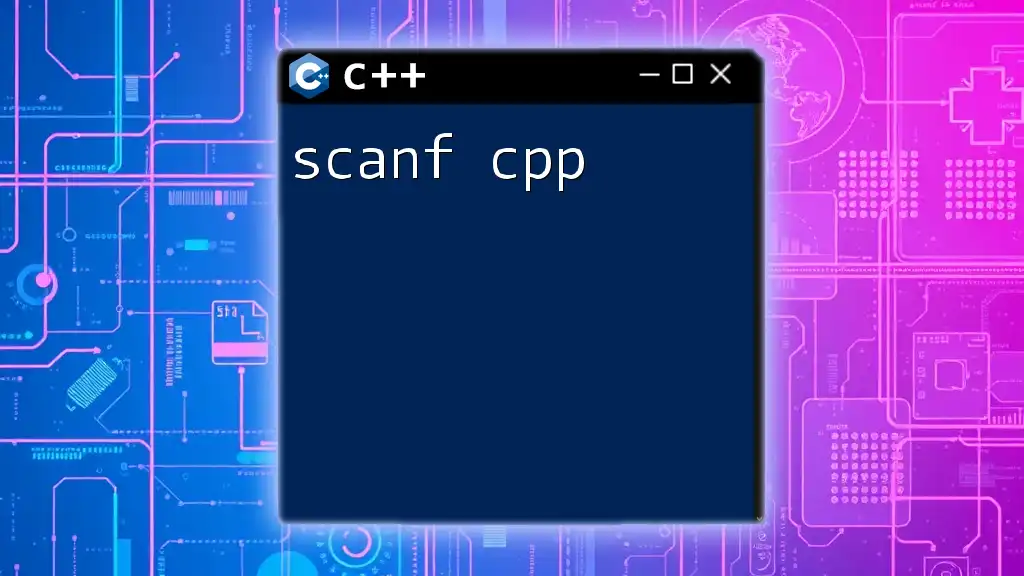
Best Practices
Error Handling in Date Functions
When working with dates, handling errors can significantly affect your application's reliability. Implementing exception handling can safeguard your application from crashes due to invalid dates:
#include <stdexcept>
// Previous includes...
int main() {
try {
// Code that could throw an exception (like invalid date creation)
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid argument encountered: " << e.what() << std::endl;
}
return 0;
}
This snippet prepares your application for handling exceptions effectively, ensuring robustness and reliability.
Performance Considerations
In applications requiring real-time date calculations, it’s crucial to optimize performance. Use efficient algorithms for date calculations, and consider reducing overhead by optimizing data structures, such as using arrays over more complex data types when appropriate.
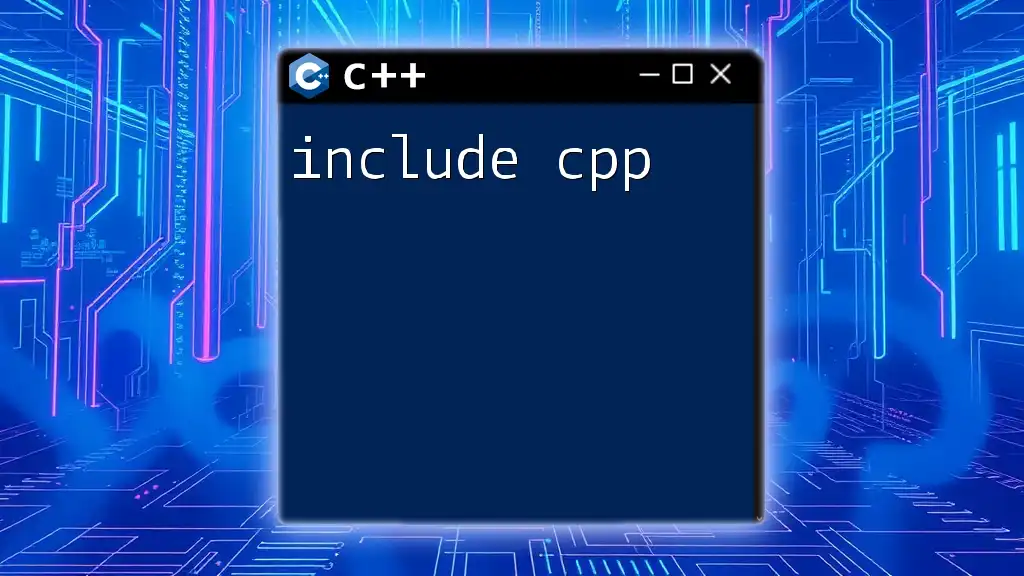
Conclusion
Summary of Key Takeaways
Understanding calendar-related functionalities in C++ significantly enhances your ability to manage dates within software applications. Mastering the chrono library and the nuances of date operations can lead to more efficient and understandable code.
Further Learning Resources
For those looking to delve deeper, consider exploring advanced C++ books, online C++ courses, or libraries like Boost.Date_Time, which offer extensive functionalities for date and time manipulations.
Call to Action
Encourage experimentation with the code examples presented in this article. Compile them, modify them, and share your results. The best way to learn is through practice, and your insights could lead to even better implementations!
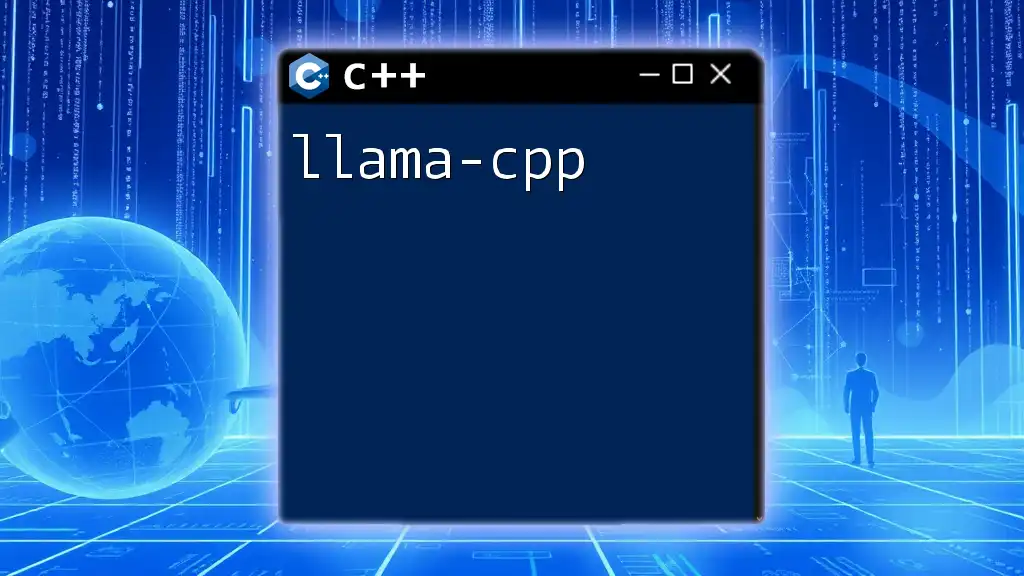
Code Appendix
Complete Code Examples
Here, you can consolidate key examples from the article for straightforward reference. This section could contain all the snippets provided along with a short description of each.
Glossary of Terms
- Timepoint: A particular point in time.
- Duration: The elapsed time between two time-points.
- UTC: Coordinated Universal Time, a time standard often used in computing.
This comprehensive guide serves to familiarize you with the core concepts and functionalities needed to effectively use Calendar CPP in your applications. Happy coding!