The `#include` directive in C++ allows you to include the contents of a file or library into your program, enabling the use of functions and classes defined outside your main source file.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is `#include`?
The `#include` directive in C++ is used to facilitate the inclusion of header files within your program. Header files are essential as they typically contain declarations for functions, constants, and data types, enabling code reuse and organization. By using `#include`, you essentially tell the compiler to include the contents of a specified file into your program at that point, streamlining your code and reducing redundancy.
Syntax of `#include`
The syntax of the `#include` directive is straightforward, consisting of the keyword `#include` followed by either angle brackets or quotes. The choice depends on the file’s origin:
#include <header_file>
- Angle brackets are used for standard library headers, telling the compiler to look in system directories. For example:
#include <iostream>
- Quotes are used for custom header files, indicating that the compiler should look in the current project directory first. For example:
#include "my_header.h"
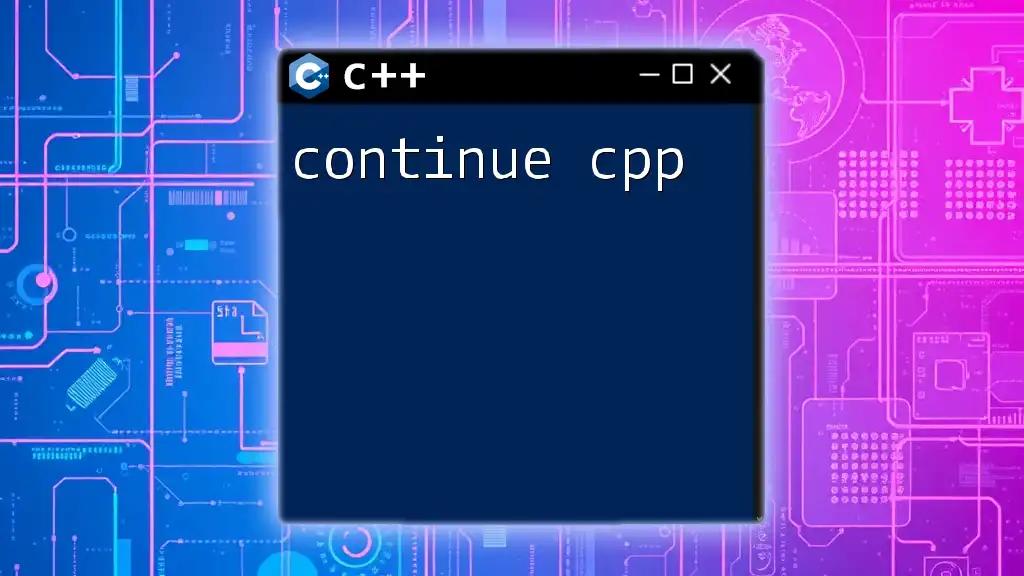
Why Use `#include`?
Advantages of Using Header Files
Using header files with the `#include` directive comes with several significant advantages:
-
Code Reusability: By organizing your code into reusable components, you can include the same header file in multiple source files without rewriting identical code.
-
Improved Code Organization: As projects grow in size, header files help maintain a clear structure. They separate interface from implementation, making your code cleaner and easier to navigate.
-
Easier Collaboration and Maintenance: When working in a team environment, having well-defined header files allows team members to collaborate effectively, focusing on different modules without interference.
Common Standard Library Headers
C++ provides numerous standard library headers that come in handy for various tasks. Here are a few commonly used headers along with their purposes:
-
`<iostream>`: This header is essential for input and output operations. It defines the standard input/output stream objects like `std::cin` and `std::cout`.
-
`<vector>`: This header provides facilities for the dynamic array, known as vectors, which can grow or shrink in size during runtime.
-
`<string>`: This header includes capabilities for string manipulation, offering enhanced features over traditional character arrays.
Example Section: Including Standard Libraries
To illustrate the use of `#include`, consider this simple program that utilizes the `<iostream>` header:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Here, the `#include <iostream>` directive allows us to use `std::cout`, which enables output to the console. When compiled, this program displays "Hello, World!" on the screen, showcasing the indispensable role of the `#include` directive.

Creating and Using Custom Header Files
Structuring Your Project with Custom Headers
For larger projects, custom header files become increasingly important, helping to modularize code. A well-organized directory structure might look something like this:
/MyProject
│
├── main.cpp
├── my_functions.h
└── my_functions.cpp
In this structure, `my_functions.h` contains function declarations, while `my_functions.cpp` implements those functions. The main program file `main.cpp` can then include the header as needed.
Creating a Custom Header File
Creating a custom header file involves defining function declarations and possibly constants or class interfaces. Here’s a simple example of a header file named `my_functions.h`:
#ifndef MY_FUNCTIONS_H
#define MY_FUNCTIONS_H
int add(int a, int b) {
return a + b;
}
#endif // MY_FUNCTIONS_H
This code snippet demonstrates how to create a header file. The `#ifndef`, `#define`, and `#endif` directives form a header guard, preventing multiple inclusions that could lead to redefinition errors.
Including Custom Header Files
To utilize your custom header file in a source file, incorporate it with the `#include` directive, as shown below in `main.cpp`:
#include "my_functions.h"
int main() {
int result = add(5, 7);
std::cout << "The sum is: " << result << std::endl;
return 0;
}
In this example, the `add` function is called from `my_functions.h`, showcasing how to incorporate custom functionality seamlessly into your program.
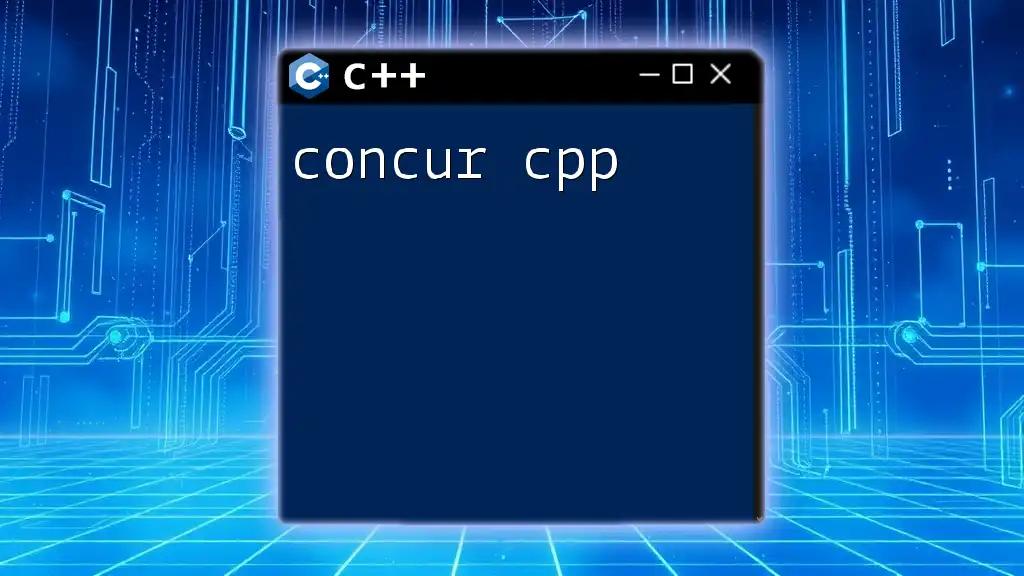
Best Practices for Using `#include`
Organizing Includes
A recommended best practice is to place all `#include` directives at the top of your source files. This helps in quickly identifying dependencies and improves code readability.
Avoiding circular dependencies is another crucial point. Circular dependencies occur when two files include each other, leading to compilation errors. Consider using forward declarations to mitigate this.
Use of Forward Declarations
Forward declarations declare a function or class without providing its implementation. This technique can often circumvent the need for `#include`, allowing for increased flexibility in your code structure.
For example:
class MyClass; // Forward declaration
void functionUsingMyClass(MyClass obj);
Avoiding Unnecessary Includes
Minimizing the number of included files can enhance compile times significantly. Whenever possible, try to avoid including headers that are not directly needed for declarations.
Using precompiled headers can also accelerate compile times in larger projects, allowing frequently used headers to be compiled only once.

Common Errors with `#include`
Understanding Compile Time Errors
Several compile time errors can arise from misusing the `#include` directive:
-
Unresolved Identifiers: This can occur if the header file containing the declaration is not included.
-
Missing Files: The compiler throws an error if it cannot find the specified header file, often due to incorrect paths or typographical errors in the filename.
Debugging Tips
To troubleshoot issues related to `#include`, start by checking for typographical errors in your filenames and paths. Ensure that the necessary files exist within the specified directories. Using IDEs with debugging tools can also help identify problematic includes efficiently.

Conclusion
The `#include` directive is a fundamental aspect of C++ programming, enabling modular code development and efficient function reuse. By mastering its usage—both with standard libraries and custom headers—you pave the way for cleaner, more maintainable code. Now that you understand the importance of the `#include` directive, we encourage you to practice creating your own headers and incorporating them into your projects. Stay tuned for more tutorials that explore C++ commands in-depth!

Additional Resources
For further exploration of the `#include` directive and C++ programming, consider visiting the official C++ documentation. Engaging with tutorials and reading recommended literature will deepen your understanding and proficiency in C++.