In C++, the directive `#include <iostream>` is used to include the standard input-output stream library, which allows you to use features like `std::cout` and `std::cin` for console input and output operations.
Here's a code snippet demonstrating its use:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Include Directives in C++
What is an Include Directive?
An include directive in C++ is a preprocessor command that allows you to include the contents of one file within another. This is essential for modular programming, where you can separate functionality into different files and include them as needed. By using include directives, you can create a well-structured codebase and promote code reuse.
The Structure of an Include Directive
The basic format of an include directive is:
#include <library>
In this syntax, the angle brackets indicate that the library is part of the standard library. However, if you are including your own header file, you would use quotes:
#include "my_header.h"
Using angle brackets tells the preprocessor to search for the header file in the system directories, while quotes make it look in the local directory first.
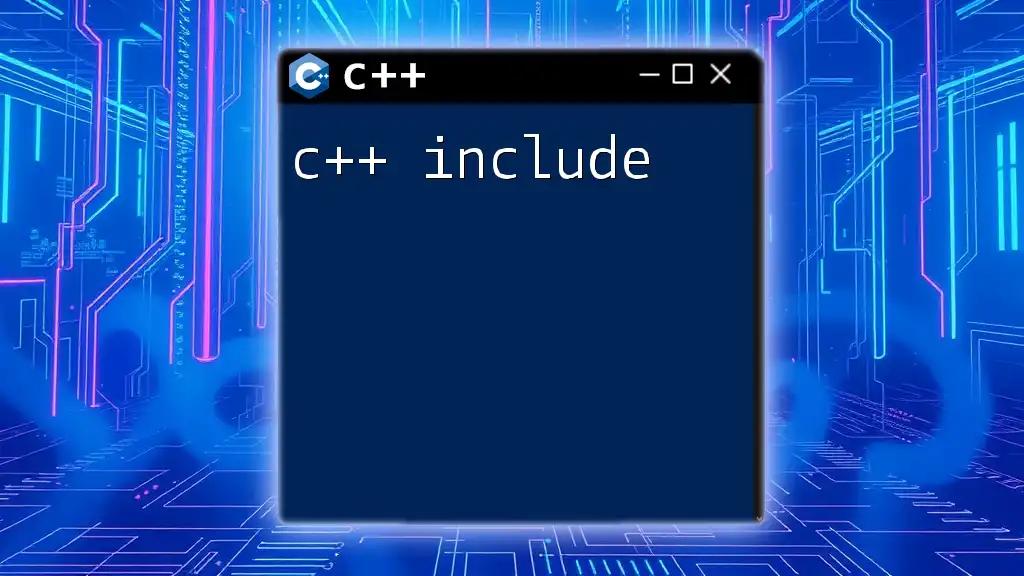
Delving into the `std` Namespace
What is the std Namespace?
The `std` namespace is a crucial part of the C++ Standard Library. Namespaces are used in C++ to provide a way to organize code and prevent naming conflicts between different parts of a program or between libraries. By enclosing standard components like functions and classes within the `std` namespace, C++ maintains clarity and modularity.
Why Use `std`?
Using the `std` namespace is important to avoid naming conflicts, especially in larger projects that may incorporate multiple libraries. By fully qualifying an identifier with `std::`, you make it clear that you are referring to a standard library component. For instance, using `std::cout` explicitly indicates that you're using the output stream mechanism from the standard library.

Basic Syntax for Including Standard Libraries
Commonly Used Standard Libraries
iostream
The `#include <iostream>` directive is one of the most commonly used in C++. It includes the header necessary for input-output operations. This allows you to perform operations like reading from the keyboard and writing to the console.
Code Example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this example, `<iostream>` enables the use of `cout`, and the line outputs "Hello, World!" to the console.
vector
The `#include <vector>` directive enables you to use the `vector` container, which is part of the Standard Template Library (STL). Vectors are dynamic arrays that can grow as needed.
Code Example:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
In this code snippet, we create a vector of integers and use a range-based for loop to print each number.
string
The `#include <string>` directive allows you to utilize the `string` class for string manipulation. This includes various built-in functions to handle string data efficiently.
Code Example:
#include <string>
#include <iostream>
int main() {
std::string welcome = "Welcome to C++";
std::cout << welcome << std::endl;
return 0;
}
This example demonstrates a simple usage of the `string` class to create a welcome message and print it out.
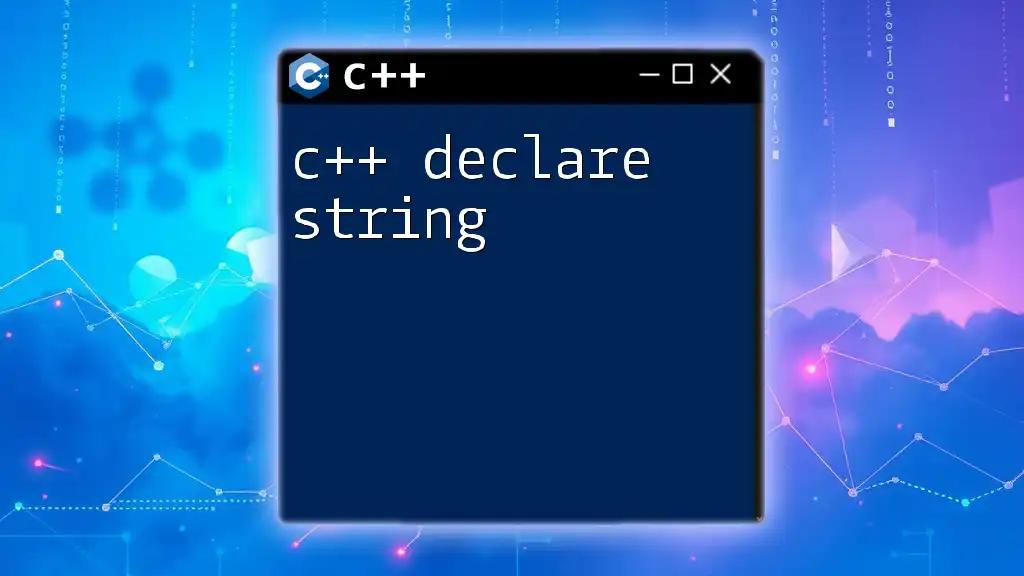
Advanced Concepts in `#include <std>`
Creating Custom Headers
Creating custom header files is an excellent way to encapsulate functionality in reusable modules. A custom header might include function declarations, macros, or even class definitions. Ensure that you protect your header files from being included multiple times by using include guards:
#ifndef MY_HEADER_H
#define MY_HEADER_H
// Declarations or definitions go here
#endif // MY_HEADER_H
Best Practices for Including Libraries
It’s a good practice to minimize dependencies on includes. Here are some best practices:
- Include only what you need: This keeps compile times down and the code cleaner.
- Use forward declarations whenever possible: If you only need to declare a class, you can do so without including its header file, thereby reducing compilation dependencies.

Common Mistakes to Avoid
Forgetting the `std::` Prefix
One of the most frequent issues developers encounter is neglecting to use the `std::` prefix for standard library components. Here's a common mistake:
#include <iostream>
// This will cause an error if std:: is not used
int main() {
cout << "Hello World!"; // Error: 'cout' was not declared in this scope
return 0;
}
By forgetting to prefix `cout` with `std::`, the compiler cannot identify the identifier, leading to confusion and errors.
Mishandling Include Directives
Another common issue is creating circular dependencies with include directives. If two files include each other, it can result in compilation errors. Similarly, be mindful of the difference between using angle brackets and quotes, as Misusing them can lead to failures in locating header files.
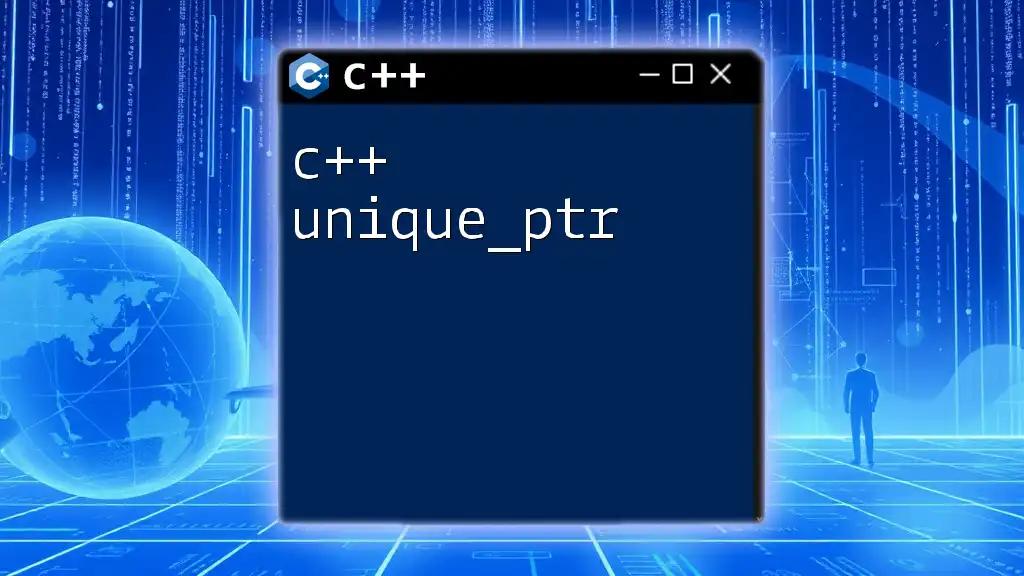
Conclusion
Incorporating `#include <std>` and understanding its underlying principles is vital for effective C++ programming. Mastery over the `std` namespace and the various standard libraries elevates your coding practices, allowing for cleaner, more efficient, and less error-prone code. Practice includes writing simple programs utilizing different standard libraries and become comfortable with standard directives as well as your own custom headers.
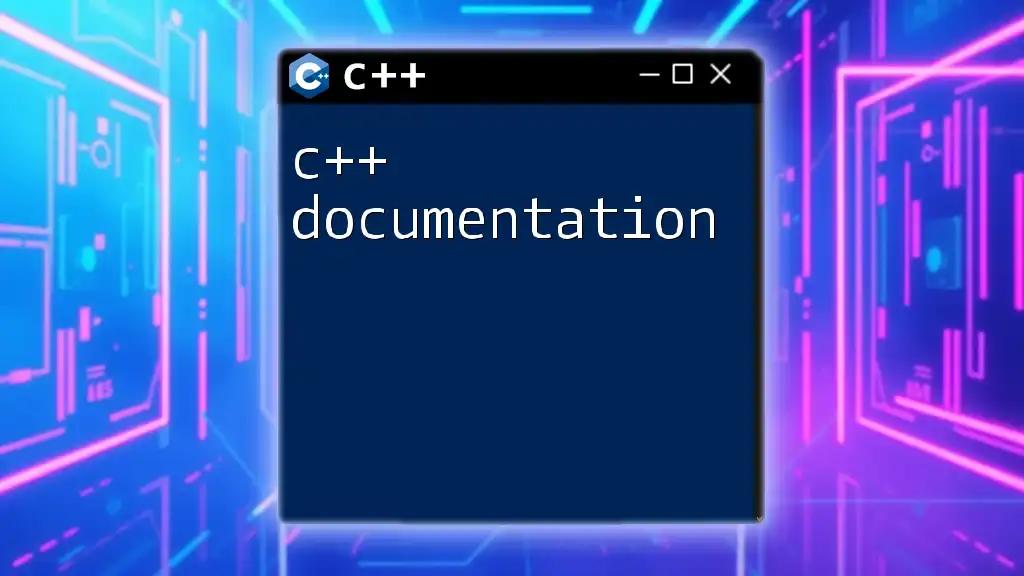
Additional Resources
To further hone your understanding of C++ libraries and constructs, explore additional resources such as tutorial websites, documentation for the C++ standard library, and community forums where you can engage with other developers. By continually learning, you will deepen your knowledge and skill in using `c++ include std` and beyond.