To include a header file from another folder in C++, you can use the relative or absolute path in the `#include` directive like this:
#include "../folder_name/header_file.h"
Replace `"../folder_name/header_file.h"` with the actual path to your header file.
Understanding Header Files
What are Header Files?
Header files in C++ serve as a crucial component in the development process. They typically contain declarations of classes, functions, and variables, allowing for better organization and modularization of code. By separating the interface from the implementation, header files enable code reusability and clarity.
Why Organize Header Files?
Organizing header files into separate folders not only enhances code readability but also contributes to maintaining a clean project structure. As projects grow in complexity, having a well-structured hierarchy of header files makes navigating the codebase easier and minimizes the chance of errors.
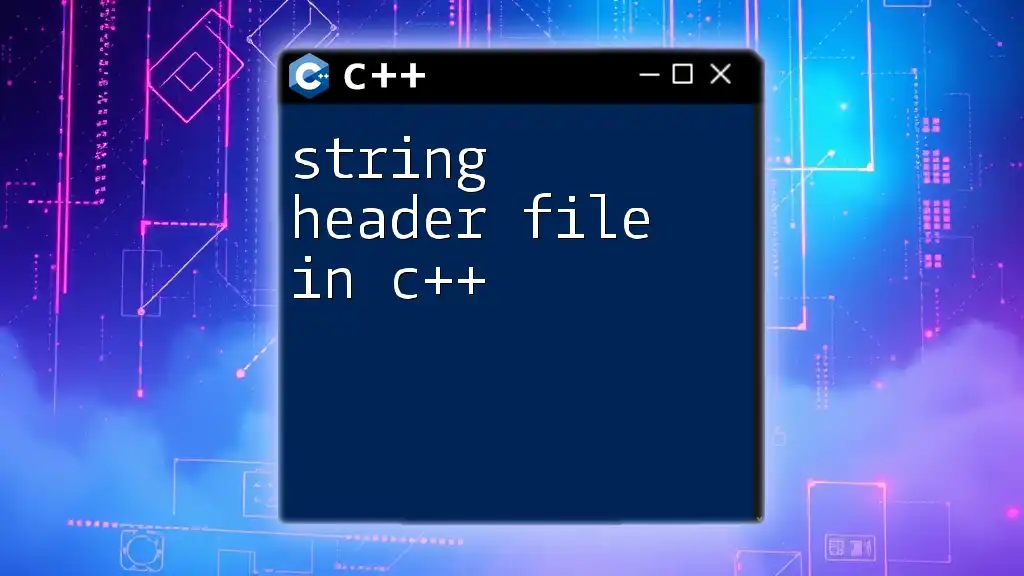
Basic Syntax of Including Header Files
Standard Include Syntax
In C++, headers can be included using two main formats:
- `#include <header_file>` is utilized for importing system libraries and built-in headers.
- `#include "header_file"` is preferred for user-defined headers.
The choice between these two formats depends on how the compiler is instructed to search for the specified files. Understanding the distinction between system libraries and user-defined headers is vital for effective coding.
Including User-Defined Header Files
User-defined header files are generally located within the project’s directory structure. To include such headers, the syntax `#include "my_header.h"` is used. For proper inclusion, ensure that the header file resides in the designated folder where the compiler can find it.
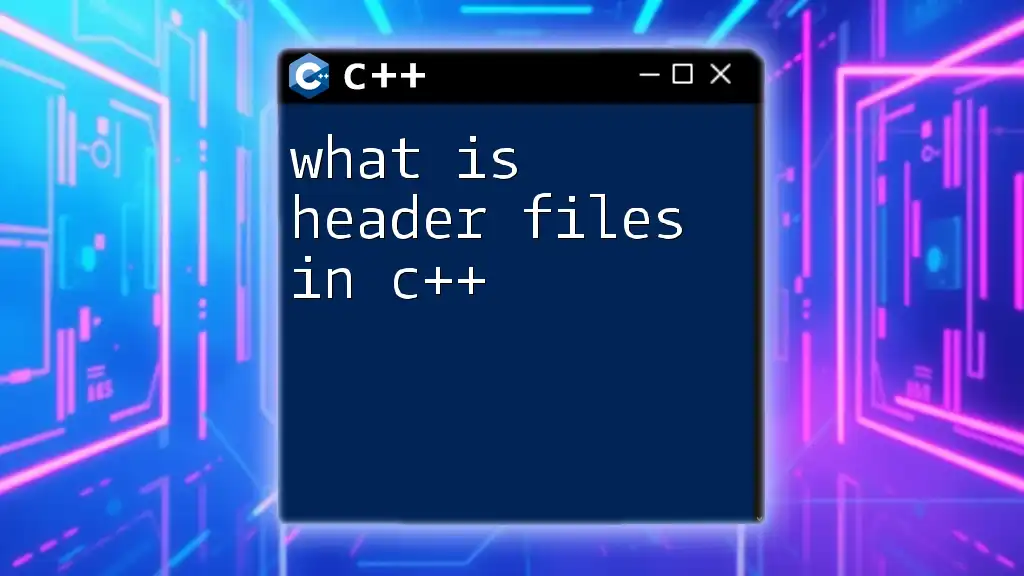
Including Header Files from Another Folder
Setting Up Your Project Structure
Establishing a clear directory structure for your C++ project is an initial step. A typical project may look like this:
project_root/
├── src/
│ └── main.cpp
└── include/
└── my_header.h
In this setup, `main.cpp` is the source file that will include the header file located in another folder. A well-organized structure not only simplifies file management but also supports scalability and team collaboration.
Using Relative Paths
In C++, you can include a header file from another folder using a relative path. This method specifies the location of the file in relation to the file that is including it.
For example, to include `my_header.h` from `main.cpp`, the syntax would be:
#include "../include/my_header.h"
Here, `..` indicates moving one directory up from the current directory, facilitating access to the header file.
Pros of using relative paths include:
- Simplified navigation when the project structure is maintained.
Cons may involve:
- Increased complexity when files are deeply nested or moved, leading to broken paths.
Using Absolute Paths
An absolute path specifies the complete location from the root directory down to the header file. This can be useful, especially in larger projects where files might be deeply nested. An example of including a header with an absolute path is:
#include "/path/to/project/include/my_header.h"
This approach removes ambiguity but can result in poor portability, as absolute paths may vary between different machines or settings.
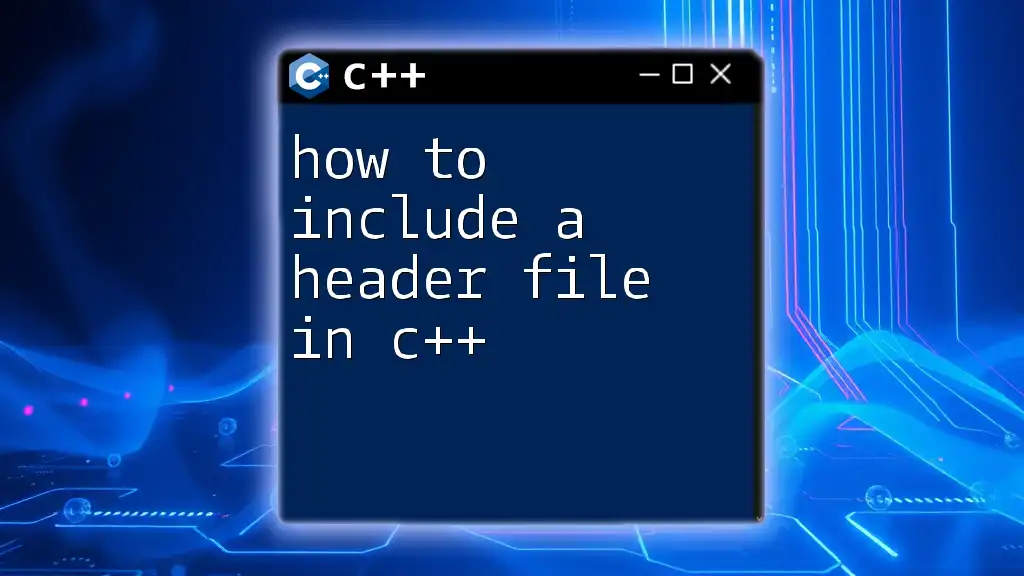
Configuring Compiler Options
Using Compiler Flags
To streamline the inclusion of header files, you can use compiler flags that specify the directory locations. This is commonly done using the `-I` option with popular compilers like g++ or clang++. For example:
g++ -I/path/to/project/include src/main.cpp -o output
This command informs the compiler to look for header files in the specified directory, promoting a clean code structure and reducing the need for modifying your code every time you change folder structure.
Modifying IDE Settings
If you're working within an Integrated Development Environment (IDE), you can often specify include directories in the project settings. For instance, in Visual Studio, navigate to the project properties, locate the C/C++ section, and append the path under "Additional Include Directories". This ensures that your IDE correctly recognizes your include paths without requiring habitual changes in your code.
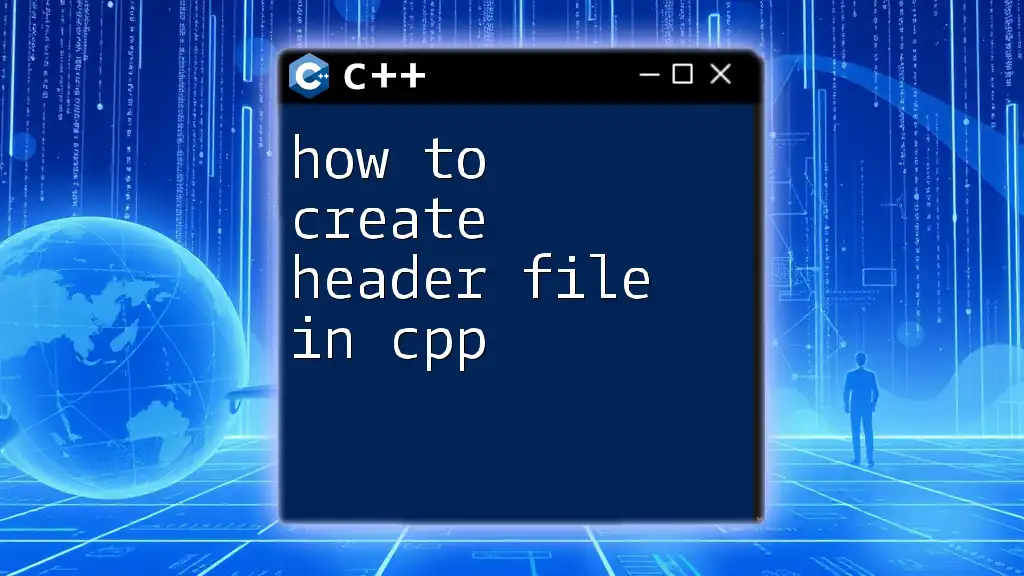
Best Practices
Consistent Directory Structure
Maintaining a consistent directory structure is paramount when developing multiple C++ projects. It allows quick navigation and minimizes errors, fostering better collaboration among team members who may be unfamiliar with the project layout.
Naming Conventions
Clear and meaningful naming conventions for header files and folders enhance readability. For instance, using a prefix like `MyLib_` can identify proprietary or specialized libraries, making it easier to recognize their purpose at a glance.
Documentation
Never underestimate the importance of documentation. Proper comments within header files improve maintainability. When you declare a function or class, ensure you provide a brief explanation about its purpose and usage. This practice benefits both current and future developers working on the code.
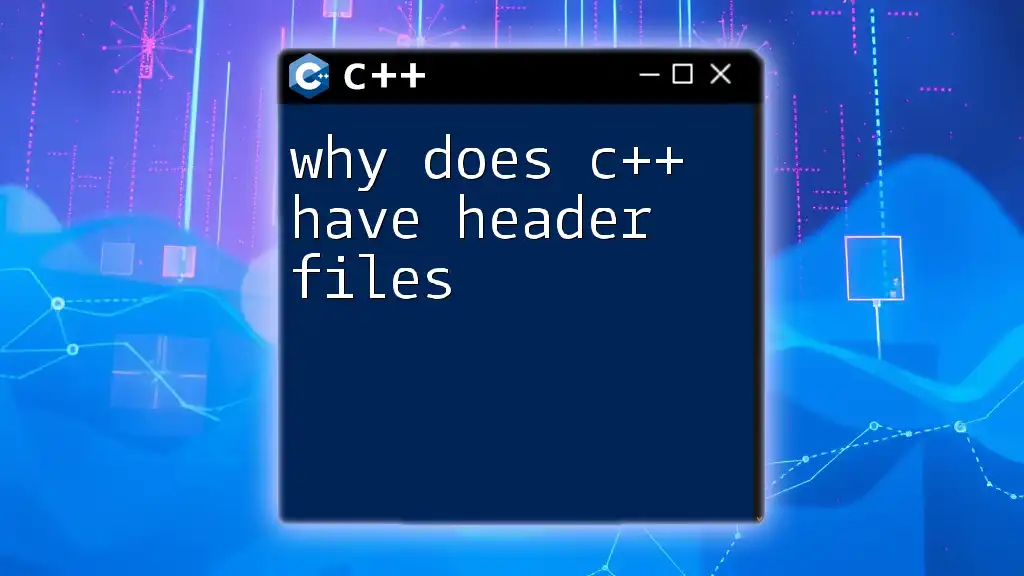
Troubleshooting Common Issues
Common Errors When Including Header Files
As you explore including header files from another folder, you may encounter some common errors, such as:
-
File Not Found Error: This usually occurs when the specified path is incorrect. Verify your include path and ensure that the header file exists in the specified location.
-
Multiple Definition Error: This manifests when a function or variable is defined in multiple places. Ensure you use header guards in your header files to prevent multiple inclusions.
Compiler Warnings and How to Fix Them
Warnings can also arise, typically related to unused variables or functions declared but not defined. Addressing these warnings improves your program's efficiency and clarity. Resolve them by ensuring that all declared elements are utilized or by removing unnecessary code.
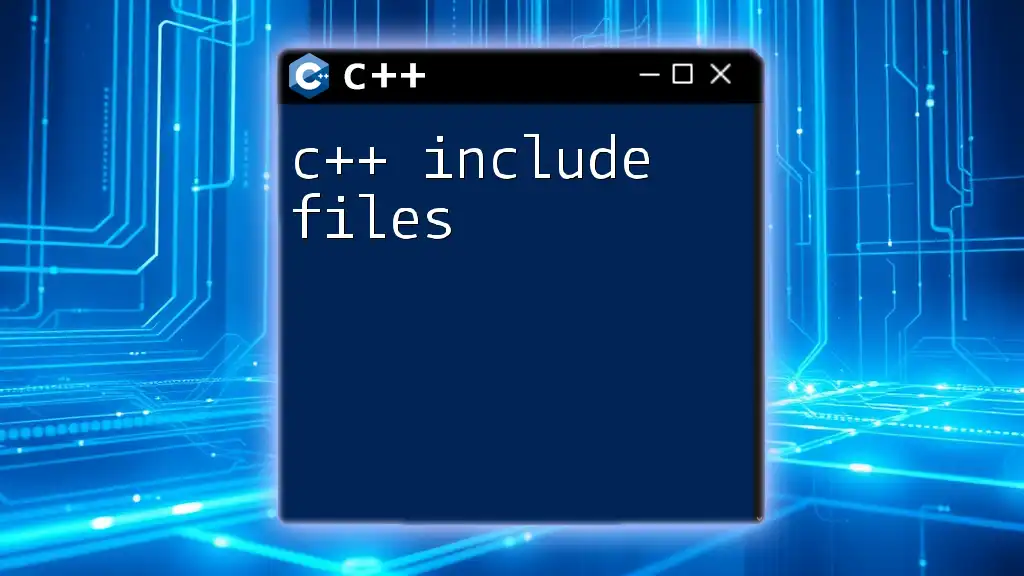
Conclusion
Incorporating header files from another folder in C++ is a fundamental yet powerful practice that enhances organization and maintainability within your projects. By following the guidelines and best practices outlined in this article, you can effectively manage your project structure and streamline your development process. Embrace these techniques to optimize your C++ coding experience today.
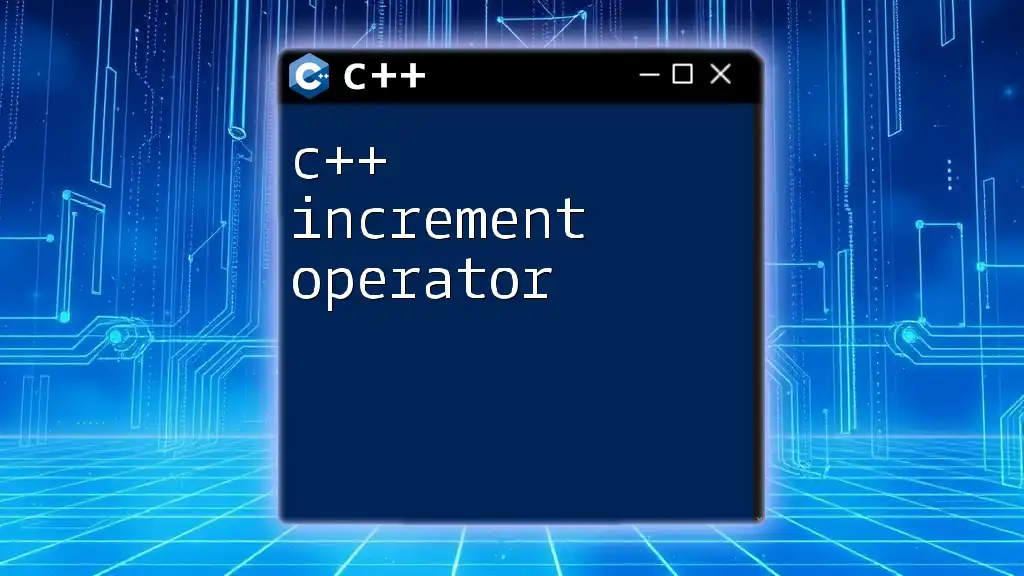
Additional Resources
For further learning, consult reputable C++ documentation, progressively dive into tutorials, and engage with community resources online. Continuous education in C++ matters, particularly as coding standards evolve.