A header file in C++ is a file with a `.h` extension that contains declarations for functions, classes, and variables, allowing them to be shared across multiple source files.
// example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
void greet();
#endif // EXAMPLE_H
What is a C++ Header File?
A C++ header file is a file containing C++ declarations and macro definitions to be shared among several source files. These files are crucial in creating modular and maintainable code, allowing programmers to use functions, classes, and variables defined in one source file across multiple files. Header files streamline the code-writing process, making it easier to manage and modify.
Characteristics of a C++ Header File
-
File Extension: Header files typically use the `.h` or `.hpp` file extensions. While `.h` is the traditional extension used widely within C and C++, `.hpp` is often used to indicate headers that include C++ code and features.
-
Content: A C++ header file can contain various elements, including:
- Function prototypes
- Class definitions
- Macros
- Inline functions
Why Use Header Files?
The use of header files offers several benefits, including:
-
Modularity: By separating declarations into header files, the code becomes better organized. Each file can focus on a specific functionality, facilitating easier understanding and management.
-
Reusability: Header files enable the sharing of common functionalities across multiple programs without the need to rewrite code. This is particularly useful when you have libraries that need to be integrated into different projects.
-
Maintainability: With header files, updates become simpler. If a function or class definition changes, you only need to update the header file and its implementations rather than revisiting all the source files that utilize it.
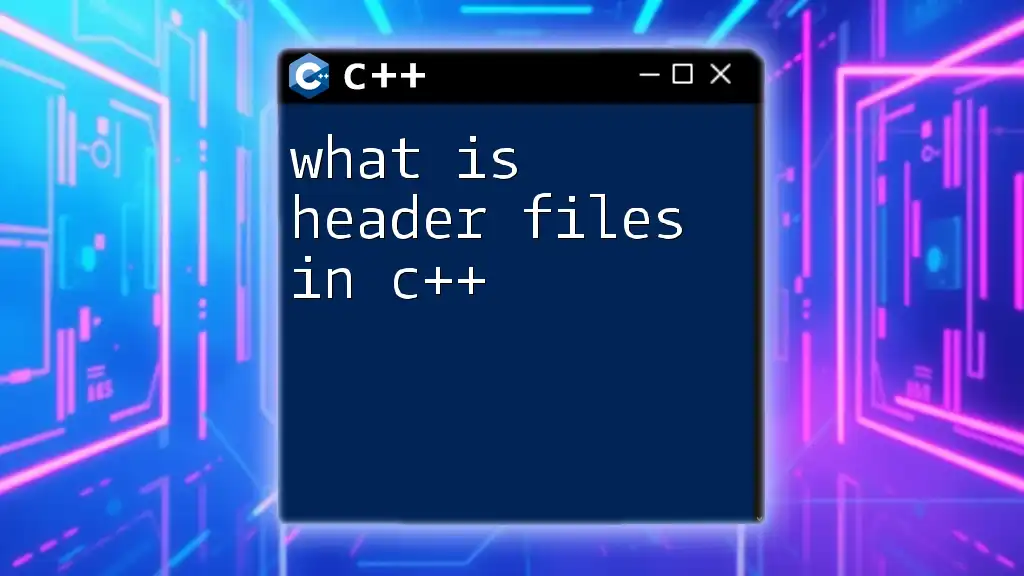
What Are C++ Header Files?
C++ header files can contain several primary components that facilitate a structured approach to programming.
Common Components
-
Function Prototypes: These act as declarations for functions defined in other files. They inform the compiler about the function's name, return type, and parameters. For example:
// Function Prototype int add(int a, int b);
-
Class Definitions: Header files often declare classes that can be used across multiple source files. This promotes code organization and encapsulation. An example class definition might look like:
class MyClass { public: void myFunction(); };
-
Macros and Constants: Preprocessor directives like `#define` are commonly used in header files to create constants or macros that can be reused throughout the code. For instance:
#define PI 3.14159
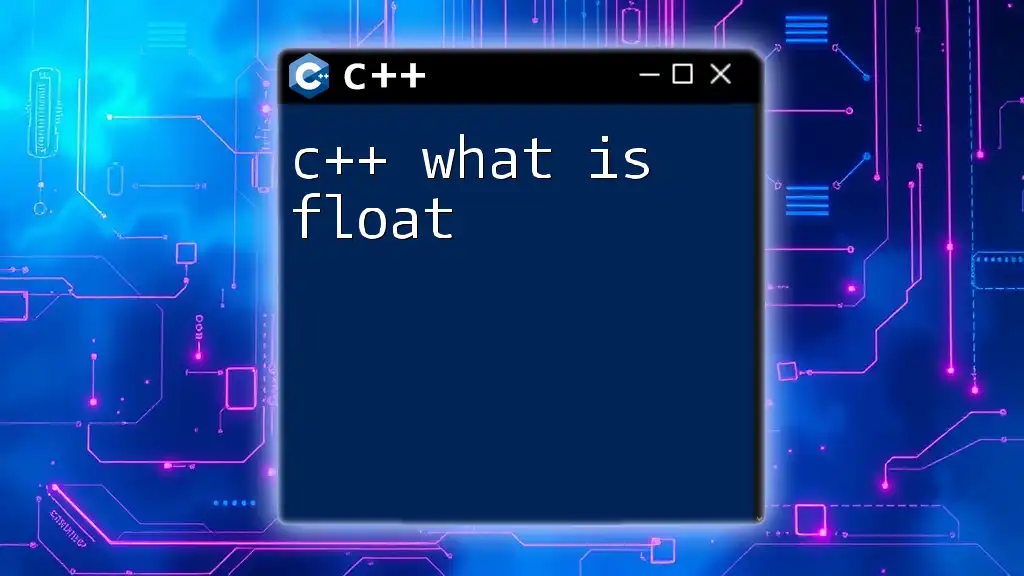
What Are Header Files in C++?
When discussing header files in C++, it’s essential to differentiate between standard header files provided by the C++ library and user-defined header files that programmers create.
Standard Header Files
C++ has a comprehensive set of standard library header files. These headers contain declarations of functions and classes used to perform input/output operations, data manipulation, and algorithms. Some common examples include:
- `<iostream>`: Used for input and output stream operations.
- `<vector>`: Contains the standard template library (STL) vector class.
User-Defined Header Files
User-defined header files are created by programmers to encapsulate their custom functions and classes. This practice enhances code reusability and organization. A simple example of a user-defined header file could look like:
// MyHeader.h
void greet();
In a corresponding source file, you could implement the function:
#include "MyHeader.h"
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
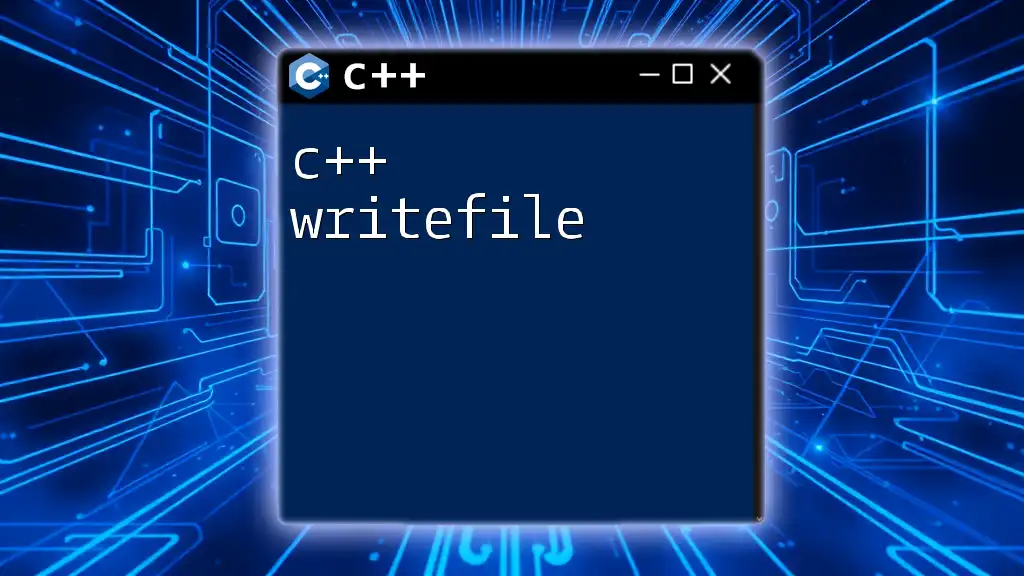
How to Include Header Files in C++
To utilize the declarations in a header file, you need to include it in your source files using the `#include` directive. Understanding the syntax is vital for proper code structure.
Syntax of Including Header Files
-
Using angle brackets (`<filename>`): This method is used for standard library headers. The compiler looks for the header in the standard library paths.
#include <iostream> // Including a standard library header
-
Using quotes (`"filename"`): This method is used for user-defined headers. The compiler first looks for the header file in the same directory as the source file.
#include "MyHeader.h" // Including a user-defined header
Example Code Snippet
Combining the inclusion of both standard and user-defined headers would look like this:
#include <iostream> // Standard library header
#include "MyHeader.h" // User-defined header
int main() {
greet(); // Calling the function from MyHeader.h
return 0;
}
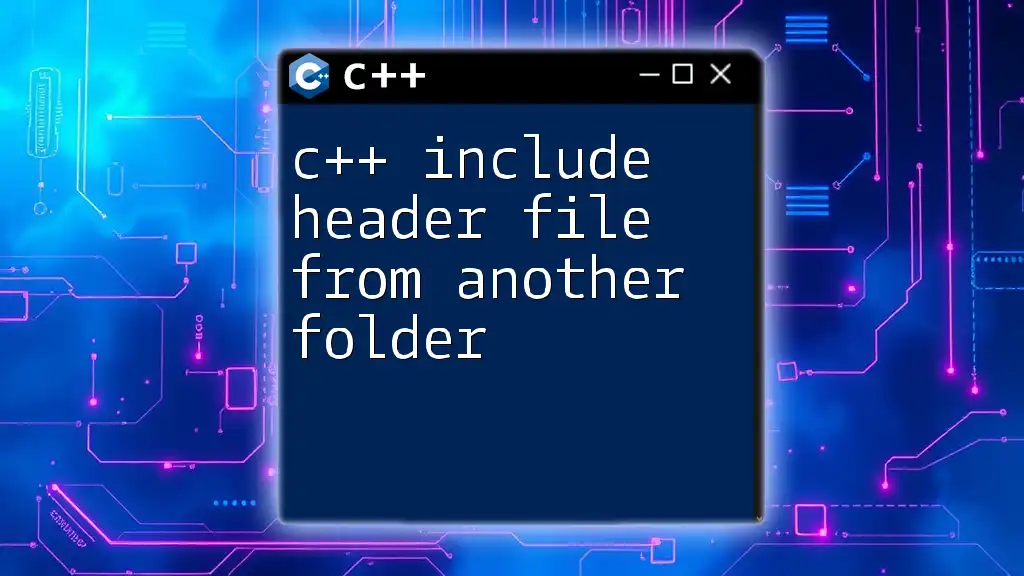
Best Practices for Using Header Files
To optimize the usage of header files, developers should adhere to several best practices.
Avoiding Common Pitfalls
-
Duplicate Definitions: To prevent accidental multiple definitions, you should implement header guards. These guards ensure that the contents of the header file are included only once per compilation unit:
#ifndef MYHEADER_H #define MYHEADER_H // Header content here #endif
-
Separate Implementation from Declaration: It is advisable to keep header files containing declarations separate from their implementations. Typically, the implementation should reside in `.cpp` files.
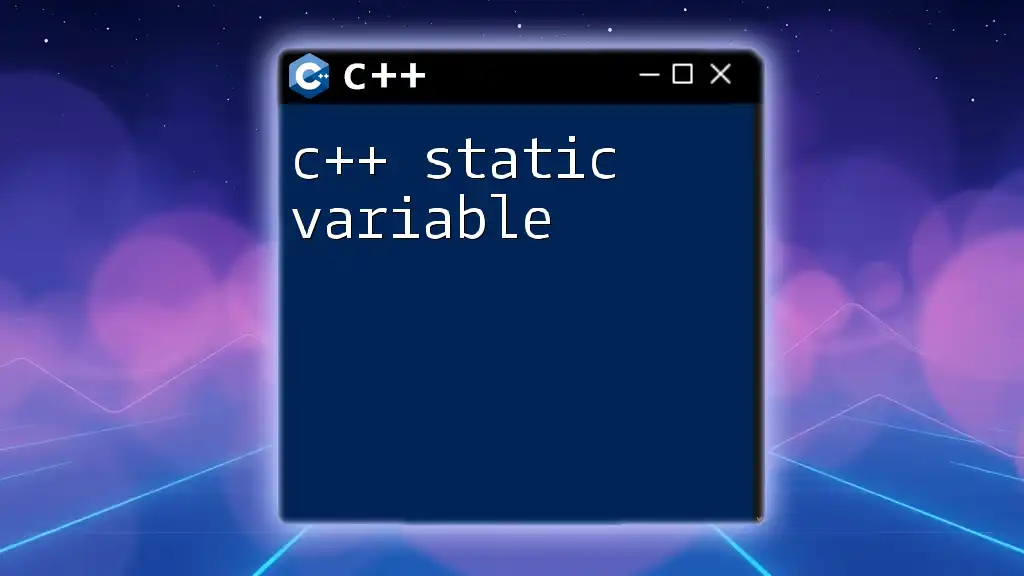
Summary
In summary, understanding C++ header files is a significant step towards mastering C++ programming. They promote modularity, reusability, and maintainability within your code, making the programming process more efficient and organized.
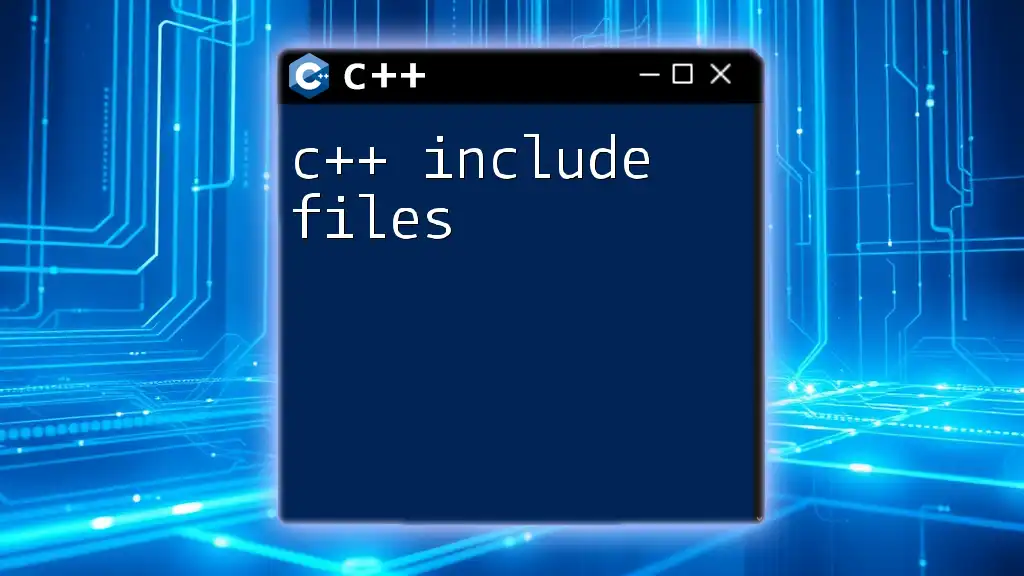
Conclusion
Incorporating header files in your C++ projects will not only improve code clarity but also enhance your coding skills for future programming endeavors. As you practice and experiment with creating custom header files, you’ll appreciate their importance in professional software development.
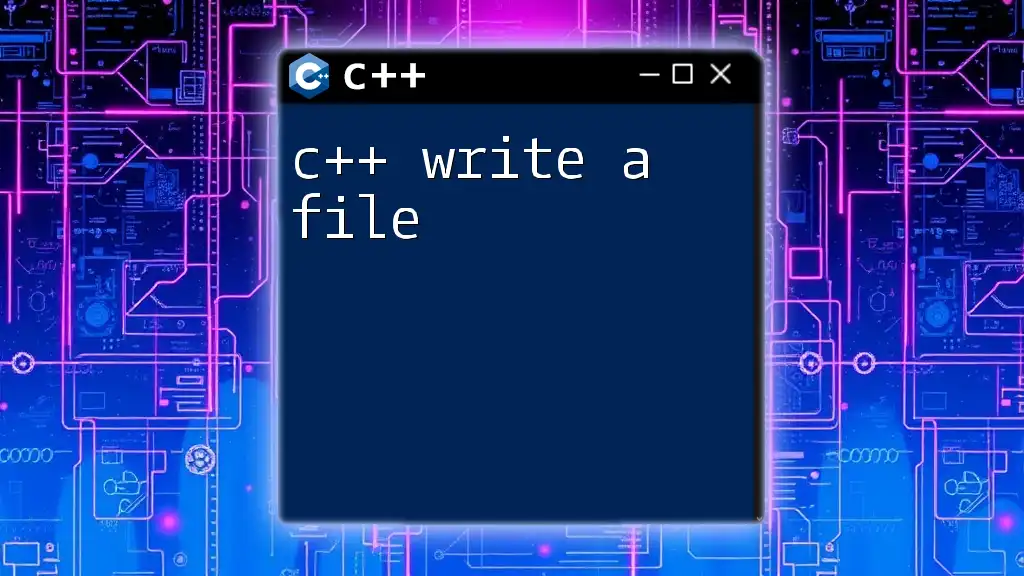
Additional Resources
For further reading, consider exploring the official C++ documentation, online tutorials, and coding communities that discuss advanced concepts and best practices related to header file usage in C++.