In C++, `std` is a namespace that contains all the features of the C++ Standard Library, including input/output, containers, algorithms, and more, allowing you to avoid naming conflicts.
Here’s a code snippet demonstrating its use with the `cout` function from the Standard Library:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is `std` in C++?
In C++, `std` is a namespace that stands for Standard. This namespace encompasses the entire C++ Standard Library, which provides a rich collection of classes and functions for common programming tasks. Understanding `std` is crucial for developers because it defines a standard set of functionalities designed to promote code clarity and efficiency.
Namespaces in C++ help avoid naming conflicts. Without namespaces, two different libraries might contain functions or classes with the same name, causing ambiguity in your code. By using `std`, you encapsulate all Standard Library features under a single name, helping to ensure uniqueness and clarity.
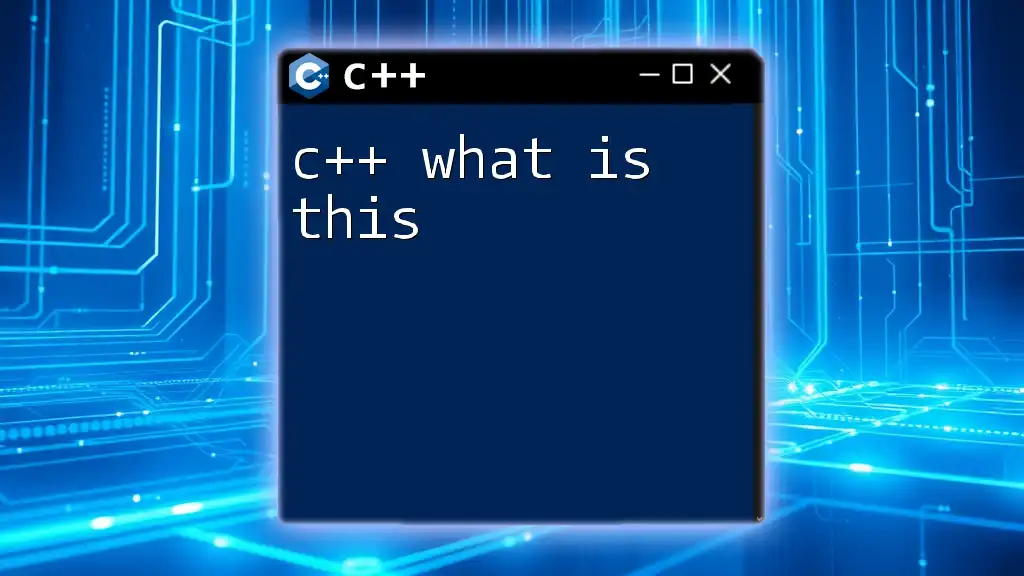
Understanding the C++ Standard Library
What is the C++ Standard Library?
The C++ Standard Library is a powerful resource in C++ programming that includes predefined classes and functions to perform various operations. It covers a wide range of functionalities, including data structures, algorithms, input/output handling, and more.
Key functionalities provided by the Standard Library include:
- Containers: Data structures like vectors, lists, and maps.
- Algorithms: Predefined methods for sorting, searching, and manipulating data.
- Streams: Tools for handling input and output.
- Strings: A robust class for managing strings dynamically.
Using the Standard Library not only speeds up the development process but also enhances code readability and maintainability. It allows programmers to leverage tried and tested implementations of common programming tasks.
The Role of `std::`
In C++, many features in the Standard Library require prefixing with `std::`. This prefix indicates that the function or object belongs to the standard namespace.
The necessity of `std::` arises mainly due to:
- Clarity: It makes it explicit where a function or class comes from, promoting better code documentation.
- Avoiding Conflicts: If your code or third-party libraries define a function with the same name as one in the Standard Library, using `std::` helps distinguish between them.
Here's an example where `std::` is used:
#include <iostream>
int main() {
std::cout << "Hello, C++!" << std::endl; // Here, std:: indicates that cout is from the Standard Library.
return 0;
}
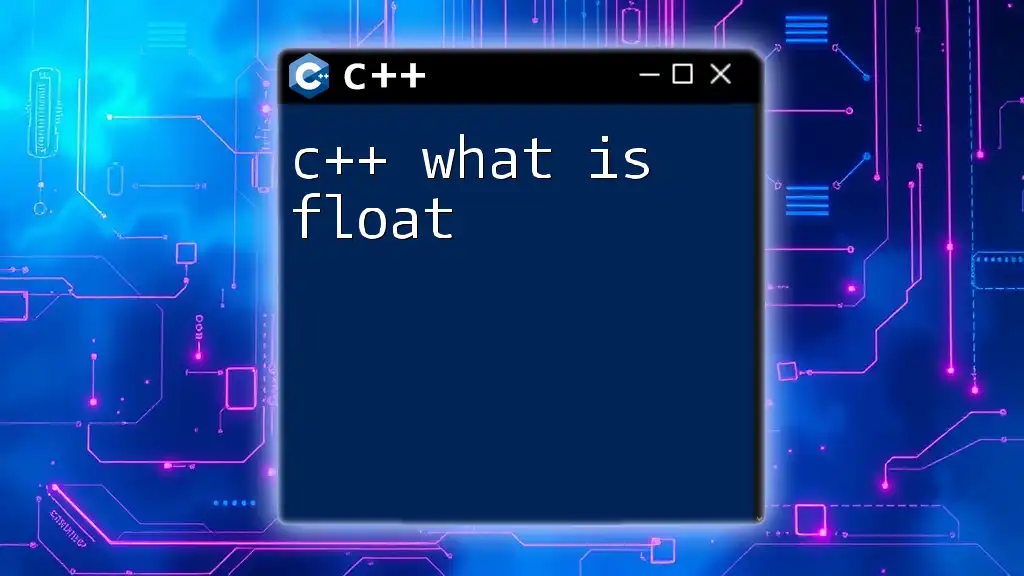
Components of the C++ Standard Library
Containers
Containers in C++ are essential structures that allow developers to manage collections of objects. The Standard Library provides several container types, each optimized for different tasks.
Common types of containers include:
- `std::vector`: A dynamic array that allows fast access and modification.
- `std::list`: A doubly linked list that facilitates fast insertion and deletion.
- `std::map`: A key-value pair collection that stores unique keys for efficient retrieval.
Here's a simple example demonstrating the use of a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5
}
return 0;
}
Algorithms
The C++ Standard Library also includes a variety of algorithms that operate on these containers. These algorithms save time and effort since they are optimized and well-tested.
Common algorithms include:
- Sort: Rearranges elements in a specified order.
- Find: Searches for an element in a container.
- Accumulate: Computes the cumulative sum of elements.
Here’s an example of using the `std::sort` algorithm:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {5, 3, 4, 1, 2};
std::sort(nums.begin(), nums.end());
for (const auto& num : nums) {
std::cout << num << " "; // Output: 1 2 3 4 5
}
return 0;
}
Input/Output Streams
C++ offers a sophisticated input/output system for handling data. The Standard Library facilitates this through several components, most notably `std::cin`, `std::cout`, and `std::cerr`.
- `std::cout` is used for outputting data to the console.
- `std::cin` reads input from the user.
- `std::cerr` is used for error output, enabling separation of error messages from regular output.
Here’s a basic example demonstrating both input and output:
#include <iostream>
int main() {
std::cout << "Please enter a number: ";
int num;
std::cin >> num;
std::cout << "You entered: " << num << std::endl; // Output: You entered: <user_input>
return 0;
}
Strings
Handling textual data in C++ is made easier with the `std::string` class. This class provides dynamic memory management and a rich set of member functions for string manipulation.
Using `std::string` allows developers to:
- Concatenate strings easily.
- Compare strings.
- Access individual characters and substrings.
A simple demonstration is as follows:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl; // Output: Hello, World!
return 0;
}
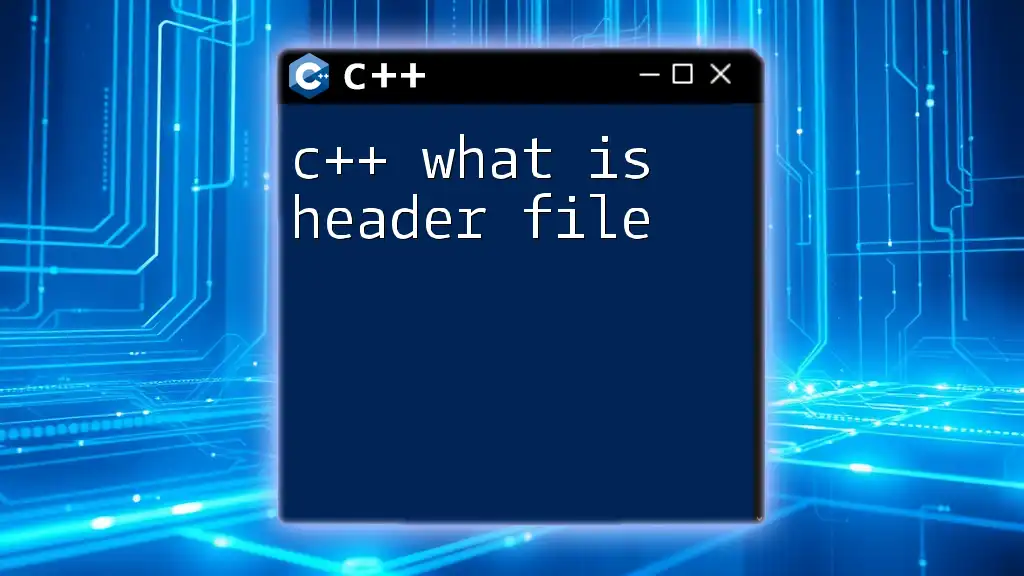
Common Mistakes with `std`
Forgetting the `std::` Prefix
One of the most common issues faced by new C++ programmers is neglecting to use the `std::` prefix for standard library components. This can lead to compilation errors and confusion when the compiler cannot locate the specified functions or classes.
To illustrate, if you attempt to use `cout` without the prefix, like so:
#include <iostream>
int main() {
cout << "Error: Missing std:: prefix!" << std::endl; // Compilation Error
return 0;
}
To make the code cleaner, you may use the using directive:
using namespace std; // However, be cautious with this in larger projects
Misworking with Namespace
While using `std::` is recommended, it’s also vital to manage namespaces effectively. Using `using namespace std;` can be convenient during small tasks or examples, but it may lead to naming conflicts in larger projects, where multiple libraries might use the same names for functions or variables.
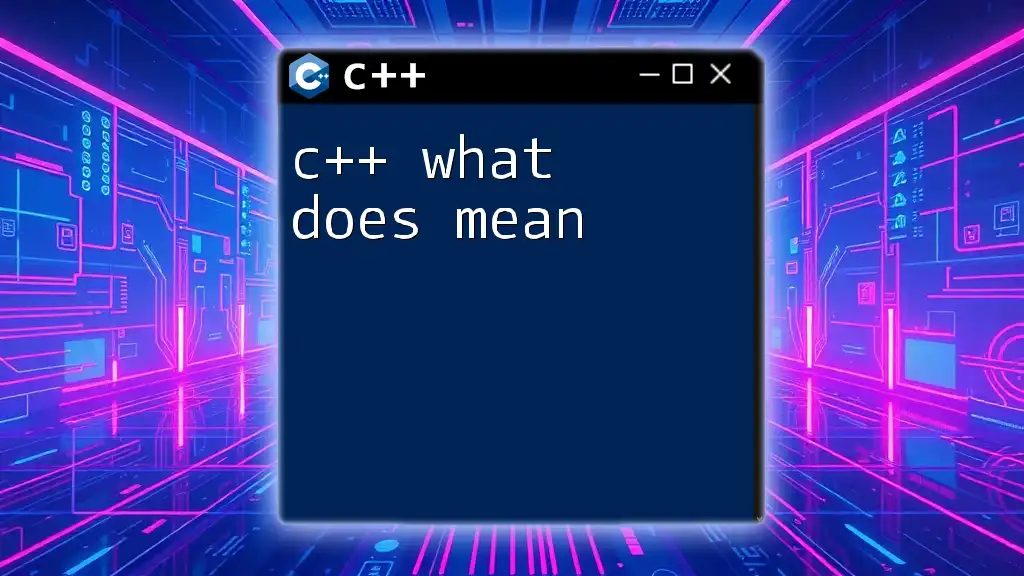
Conclusion
Understanding `std` and the C++ Standard Library is a vital step for any serious C++ programmer. The benefits of using the Standard Library—such as increased productivity, improved code quality, and maintainability—are significant. By adopting the `std::` prefix and utilizing the components offered by the Standard Library, developers can create robust and efficient applications.
As you dive deeper into the language, remember that practice is key. Embrace the tools available to you through `std`, and you'll find your programming capabilities expanding in a myriad of exciting ways.
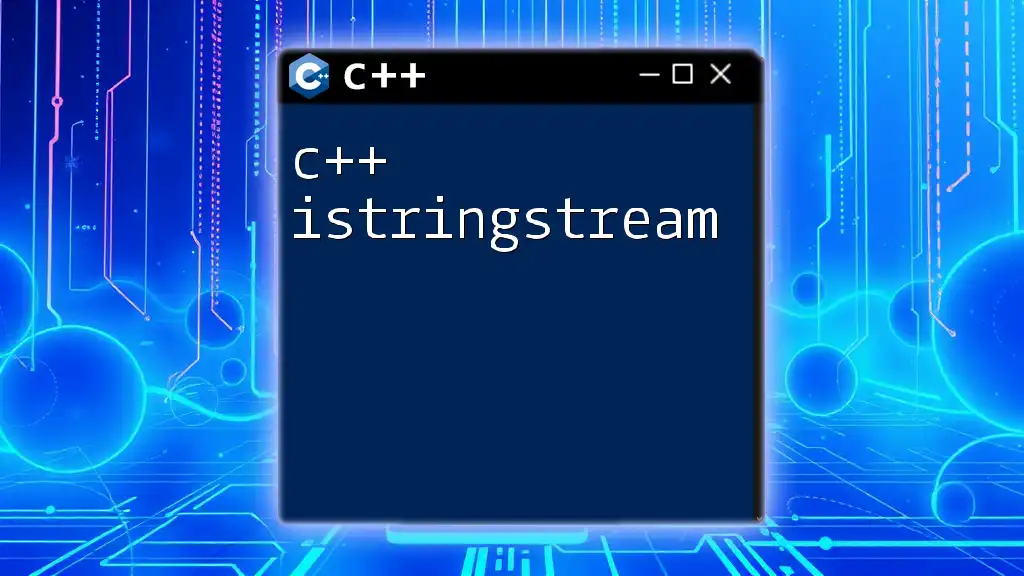
Call to Action
We invite you to share your experiences with `std` and the Standard Library in your C++ projects. What challenges have you faced, or what tips do you have for others? Additionally, check out our courses and resources for deeper learning!