C++ is a powerful, high-level programming language that combines the features of both procedural and object-oriented programming, making it suitable for system/software development as well as game programming.
Here's a simple code example demonstrating a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a high-level, versatile programming language that is widely used in system and application software development. Originally developed by Bjarne Stroustrup in the early 1980s, C++ evolved from the C programming language and is recognized for enabling both procedural and object-oriented programming.
Its significance in the software development landscape cannot be overstated. C++ combines the efficiency and control of lower-level languages with the abstraction necessary for higher-level programming, making it a favorite amongst system programmers, game developers, and application architects.
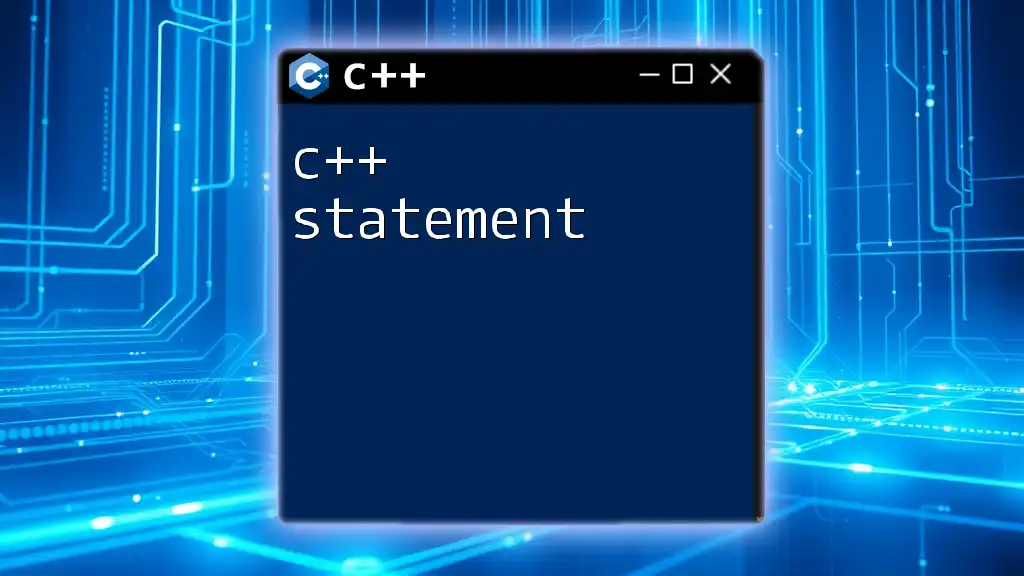
Background of C++
History of C++
C++ has a rich history that dates back to its inception in 1979 when Stroustrup began working on "C with Classes." The language gained traction quickly, with the first commercial release occurring in 1985. Key milestones include:
- 1989: The introduction of multiple inheritance.
- 1998: The first official standard, known as C++98, was ratified.
- 2011: C++11 brought significant features such as auto keyword, range-based for loops, and smart pointers.
Importance of C++ in the Programming Landscape
C++ plays a pivotal role in various domains due to its performance and versatility. It is extensively used in:
- Systems programming for operating systems and embedded systems.
- Game development where real-time performance is critical.
- High-performance applications like financial trading systems or simulations.
When compared with languages such as Python and Java, C++ offers greater control over system resources, making it a preferred choice for memory-critical applications.
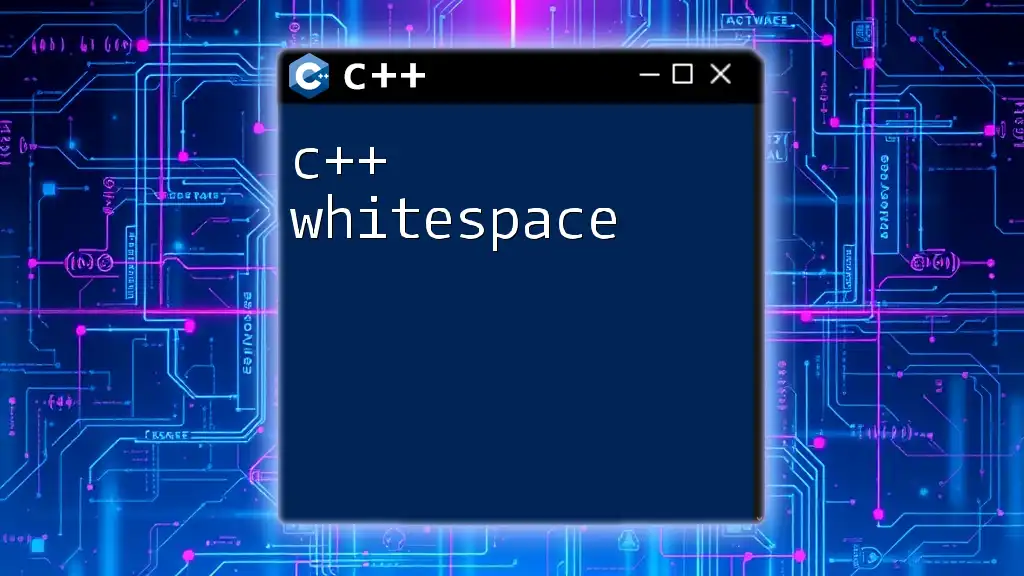
Understanding C++ Syntax
Basic Syntax and Structure
Every C++ program begins with including necessary header files and defining the `main()` function, which serves as the entry point. Here's a simple example of a complete C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this example:
- `#include <iostream>` includes the input-output stream library.
- `using namespace std;` allows the use of standard functions without prefixing them.
- `cout` is used for output, and `endl` creates a new line.
Understanding the basic structure is essential for writing C++ programs.
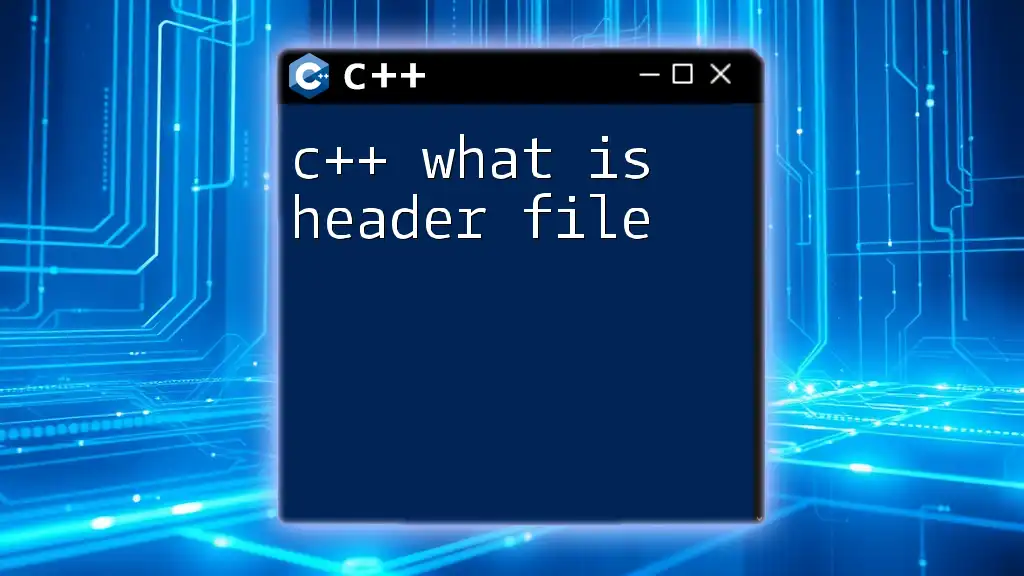
Primary Features of C++
Object-Oriented Programming (OOP) Concepts
C++ is often celebrated for its support for Object-Oriented Programming (OOP), which enhances code organization and reusability. Here are its core concepts:
Classes and Objects
A class is a blueprint for creating objects. Objects are instances of classes that encapsulate data and functions. Here's a simple example of defining a class in C++:
class Car {
public:
string brand;
void honk() {
cout << "Honk! Honk!" << endl;
}
};
In this example, `Car` is a class with a public member variable `brand` and a public method `honk()`. Creating an object of this class allows you to use its attributes and methods.
Encapsulation, Inheritance, and Polymorphism
- Encapsulation is the bundling of data and methods that operate on the data.
- Inheritance allows new classes to acquire the properties of existing ones, promoting code reusability.
- Polymorphism enables one interface to be used for different data types, allowing functions to process objects differently based on their class.
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful feature of C++ that provides data structures and algorithms. The advantages of using STL include:
- Efficiency: Reusable code saves time and minimizes errors.
- Flexibility: Algorithms can operate on any compatible container.
Common STL containers include `vector`, `list`, and `map`. Here's an example of using a vector:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
The above code initializes a vector with integers and prints them. Utilizing STL can significantly enhance your coding efficiency in C++.
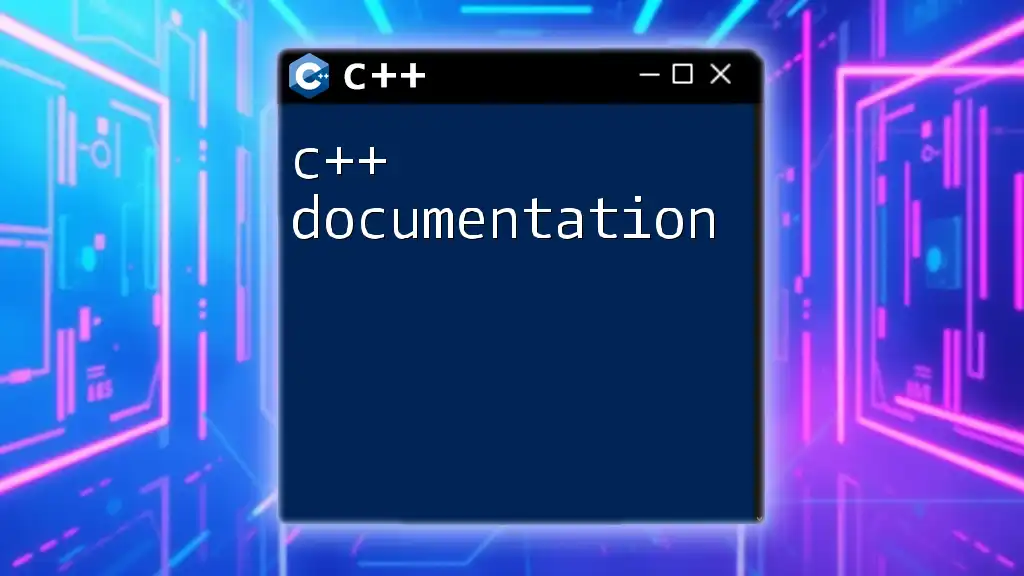
Key Concepts in C++
Memory Management
One of the notable features of C++ is memory management. It provides both automatic and dynamic memory management capabilities. Understanding pointers and dynamic memory allocation is crucial for efficient resource use.
Consider this example:
int* ptr = new int(5);
cout << *ptr; // Outputs: 5
delete ptr;
In this code:
- `new` allocates memory for an integer and returns its address.
- `*ptr` dereferences the pointer to access the stored value.
- `delete` frees the allocated memory, preventing memory leaks.
Error Handling
Error handling in C++ is primarily achieved through the use of exceptions. This mechanism allows developers to manage runtime errors and maintain program stability. Here's how exception handling works:
try {
throw std::runtime_error("Error occurred");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
In this example, a runtime error is thrown and caught, enabling the program to handle the error gracefully while providing useful debug information.
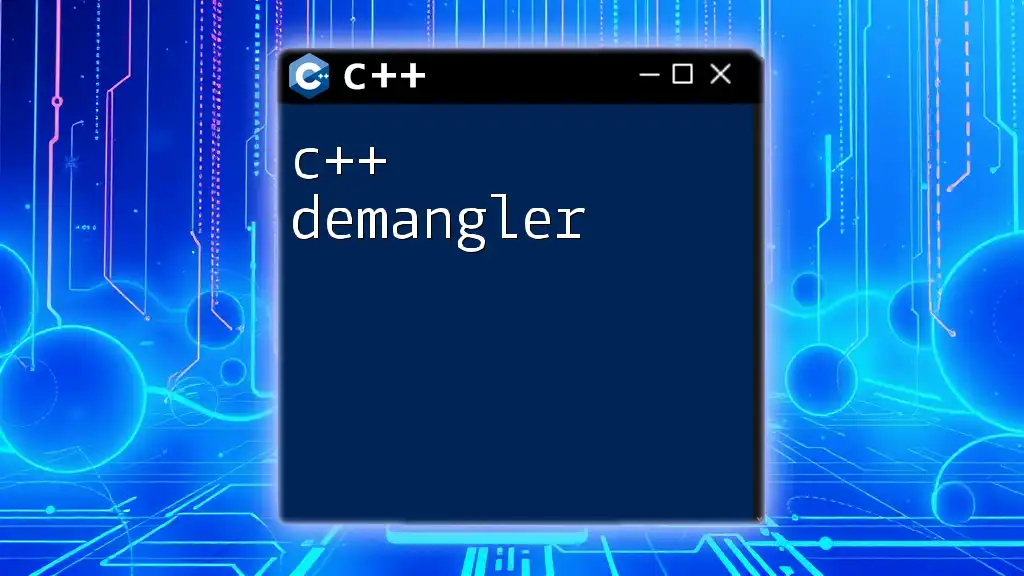
The C++ Ecosystem
Compilers and Development Environments
C++ development typically involves using compilers and integrated development environments (IDEs). Popular compilers include GCC, Clang, and Microsoft Visual Studio. Each comes with unique features and optimizations for C++ code compilation.
While choosing an IDE, consider options like:
- Code::Blocks: A free, open-source IDE that supports multiple compilers.
- Eclipse: Offers a C/C++ development environment and extensive plugins.
C++ Communities and Resources
C++ has a vibrant community and vast resources available for learners and developers:
- Online Forums: Platforms like Stack Overflow and CPlusPlus.com are invaluable for asking questions and sharing knowledge.
- Recommended Books: Titles such as "C++ Primer" and "The C++ Programming Language" provide comprehensive insights into both basic and advanced C++ concepts.
- Documentation: The official C++ documentation is an essential resource for understanding the language's intricacies.
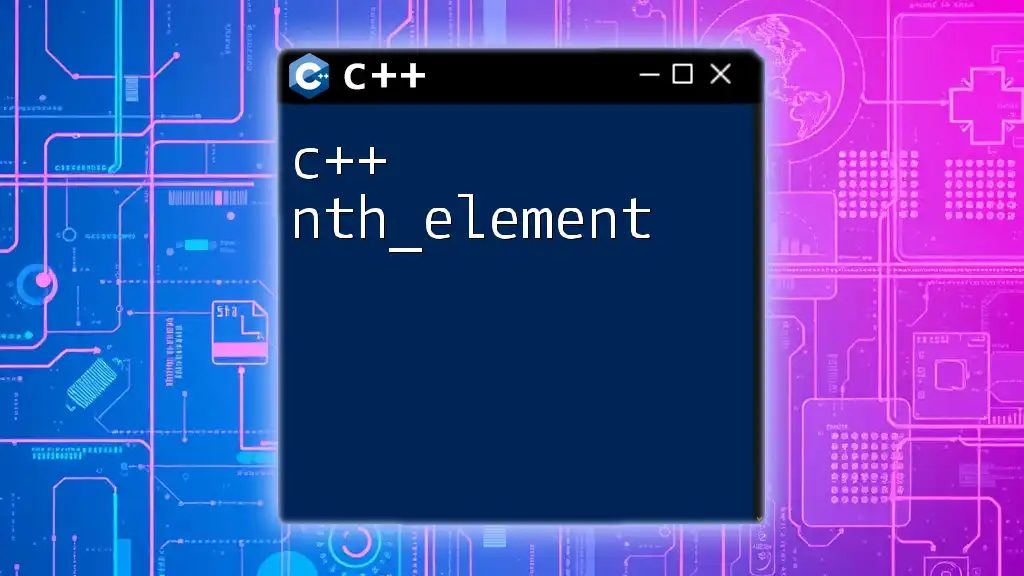
Conclusion
The Future of C++
C++ continues to evolve, maintaining its relevance in modern programming. As new standards emerge (e.g., C++20), further improvements in performance, usability, and language features are expected. The continual evolution signifies that C++ will remain a critical language for developers.
Call to Action
If you're intrigued by programming and want to learn C++ effectively, consider joining our training program. We offer concise resources and hands-on experiences that empower you to master C++. Code is best learned through practice and experimentation—embrace this journey!