A C++ package manager is a tool that simplifies the installation, version management, and configuration of C++ libraries and dependencies for your projects.
Here's a code snippet demonstrating how to use `vcpkg`, a popular C++ package manager, to install the `boost` library:
vcpkg install boost
Understanding the Concept of a Package Manager
What is a Package Manager?
A package manager is a crucial tool in software development that automates the process of installing, upgrading, configuring, and removing software packages. It acts as a bridge between developers and libraries or frameworks, ensuring that all required dependencies are properly managed. Package managers help mitigate common issues related to global installations, such as version conflicts and dependency trees.
The Role of Package Managers in C++
In the context of C++, package managers play a vital role in simplifying and streamlining the development workflow. C++ projects often depend on various third-party libraries, and managing these dependencies manually can be cumbersome and error-prone. C++ package managers help by allowing developers to:
- Easily fetch and install libraries.
- Manage different versions of libraries efficiently.
- Share and distribute packages within a community.
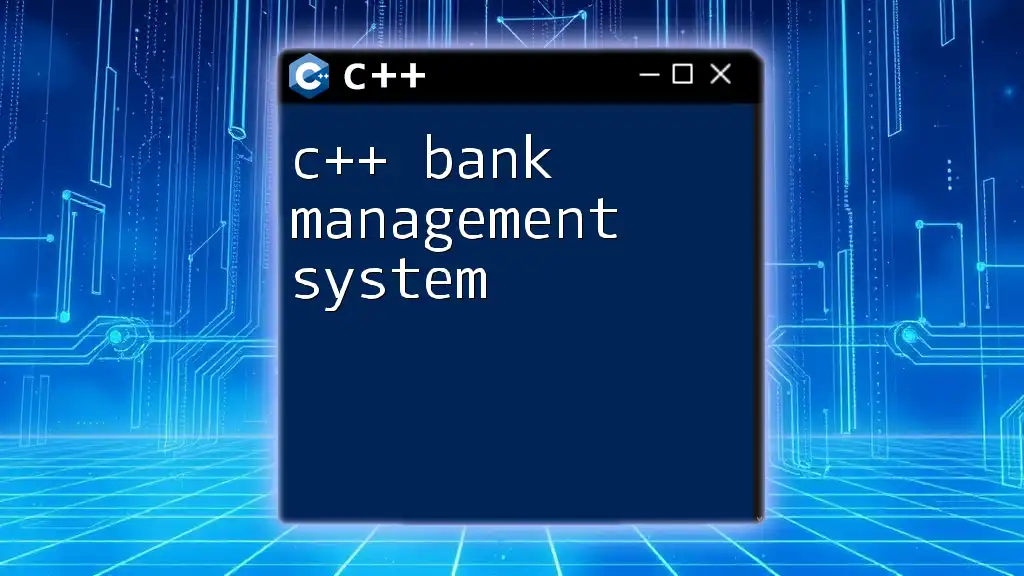
Popular C++ Package Managers
Overview of C++ Package Managers
Several C++ package managers have gained popularity among developers, each with its unique strengths and features. Notable ones include:
- Conan: Originally designed for C++, it has grown into a versatile package manager supporting many platforms.
- vcpkg: Specifically designed to work seamlessly with Visual Studio and the Microsoft ecosystem.
- Hunter: Integrates with CMake, allowing it to pull dependencies for any CMake project easily.
Conan: The C++ Package Manager
What is Conan?
Conan is an open-source package manager specifically crafted for C++ projects. It is widely adopted due to its versatility and extensive community support. Conan helps developers automate the management of library dependencies seamlessly, allowing a smooth integration process within any C++ project.
Installing Conan
To get started with Conan, you first need to have Python installed on your system. Then, you can easily install it using pip. Here’s how:
pip install conan
Once installed, you can verify the installation by executing:
conan --version
Basic Commands in Conan
Some fundamental commands in Conan that new users should know include:
- Creating a package: This command generates a basic package structure.
conan new <package-name>/<version>@user/channel
- Installing a package: This fetches a specific package along with its dependencies.
conan install <package-name>/<version>@user/channel
Conan also allows you to create and manage profiles, making it easier to customize build configurations.
vcpkg: The Visual C++ Package Manager
What is vcpkg?
vcpkg is another popular package manager designed specifically for Windows development and seamlessly integrates with Visual Studio. It supports numerous libraries and frameworks, designed to make dependency management straightforward for developers working within the Microsoft ecosystem.
Installing vcpkg
Installing vcpkg requires Git and CMake on your system. To install, run the following commands:
git clone https://github.com/microsoft/vcpkg.git
cd vcpkg
.\bootstrap-vcpkg.bat
Once installed, to check if it works correctly, you can run:
vcpkg help
Basic Commands in vcpkg
Some useful commands for managing packages with vcpkg include:
- Adding packages: To install a library:
vcpkg install <package-name>
- Integrating with Visual Studio: vcpkg provides integration with Visual Studio to manage dependencies directly within your projects. This can be done using:
vcpkg integrate install
Hunter: The C++ Package Manager
Introduction to Hunter
Hunter is a C++ package manager that integrates directly with CMake. It eliminates the need for manual downloading and building of dependencies, providing a streamlined experience.
Basic Setup of Hunter
To use Hunter, you will need to edit your `CMakeLists.txt` for configuring dependencies. Here’s an example setup:
include("path/to/hunter/hunter.cmake")
hunter_add_package(Boost)
find_package(Boost REQUIRED)
This setup integrates the Boost library into your project without manually handling dependencies, demonstrating Hunter's strengths in CMake project environments.
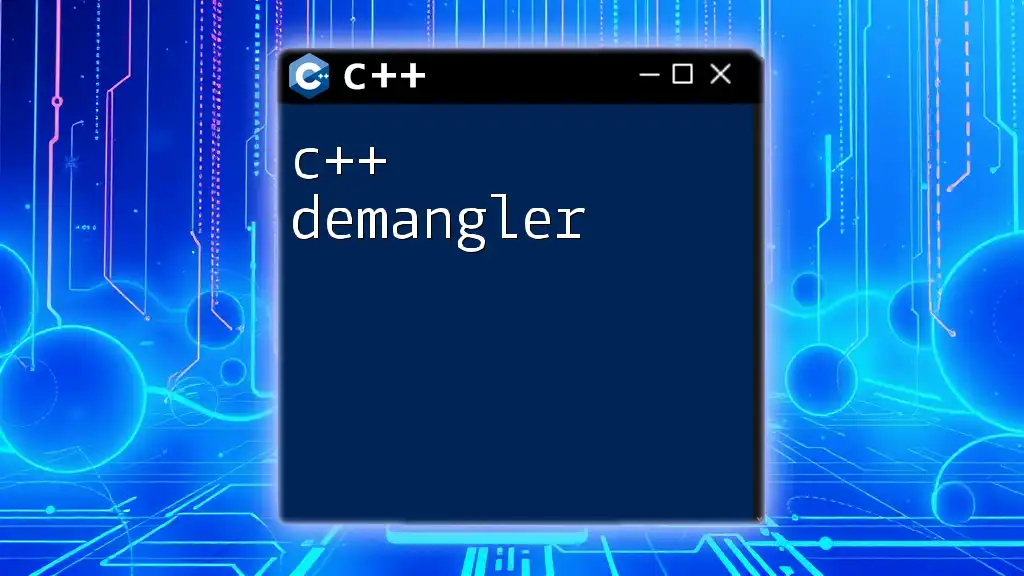
Choosing the Right Package Manager for Your Project
Criteria for Selection
When selecting a C++ package manager, consider various criteria such as:
- Community Support: Look for an active user base and contributions.
- Ease of Use: The tool should be intuitive and easy to integrate into your workflow.
- Feature Set: Evaluate if it meets your specific project needs in terms of library support and functionality.
Use-Case Scenarios
Different projects may require different tools, and understanding these scenarios can help inform your choice. For instance:
- Small projects might benefit from Conan's straightforward CLI and flexibility.
- Large-scale applications may require vcpkg's robustness and close integration with Visual Studio.
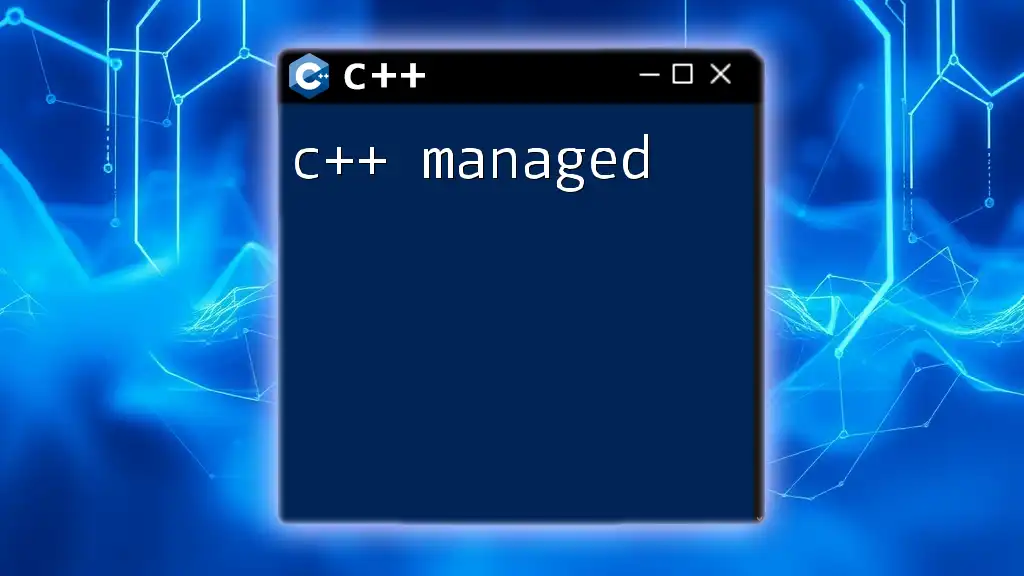
Working with C++ Package Managers
Best Practices for Using Package Managers
To ensure efficient use of C++ package managers, adhere to the following best practices:
- Organize Dependencies: Maintain a clear structure in your project to manage dependencies efficiently.
- Regularly Update Packages: Staying updated with the latest package versions can prevent security vulnerabilities and bugs.
Common Challenges and Solutions
Working with package managers can come with its complexities. Some common challenges include:
- Dependency Conflicts: If two libraries require different versions of the same dependency, use tools provided by the package manager to resolve these conflicts.
- Managing Outdated Packages: Routinely check for updates and take steps to migrate to newer versions when necessary.
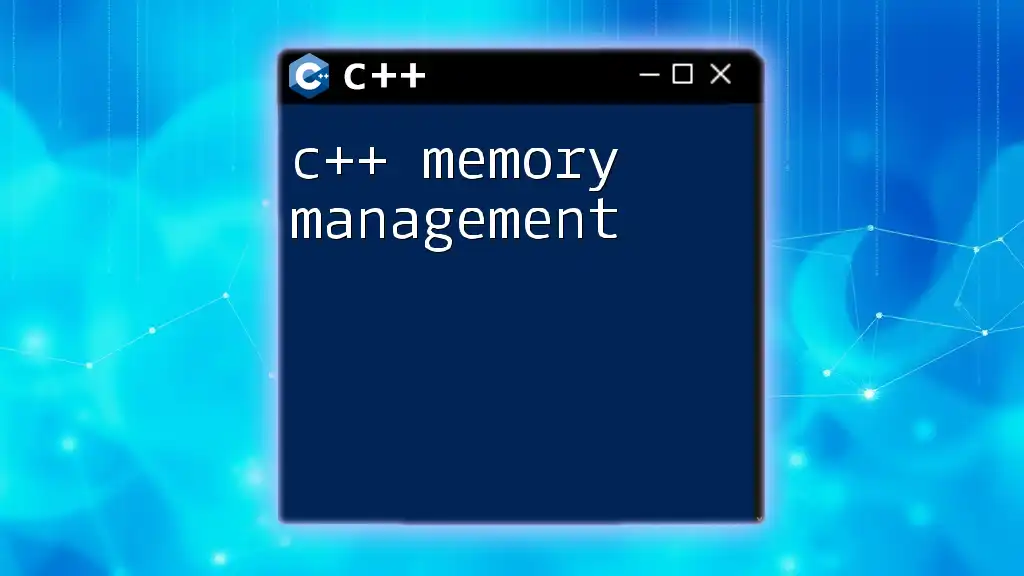
Conclusion
The importance of a C++ package manager cannot be overstated in today's development landscape. From simplifying dependency management to enabling seamless integration with existing projects, choosing the right tool can enormously enhance productivity.
We encourage you to delve into using C++ package managers in your own projects, exploring the vast ecosystem of libraries they provide, and joining our community to share tips and insights.
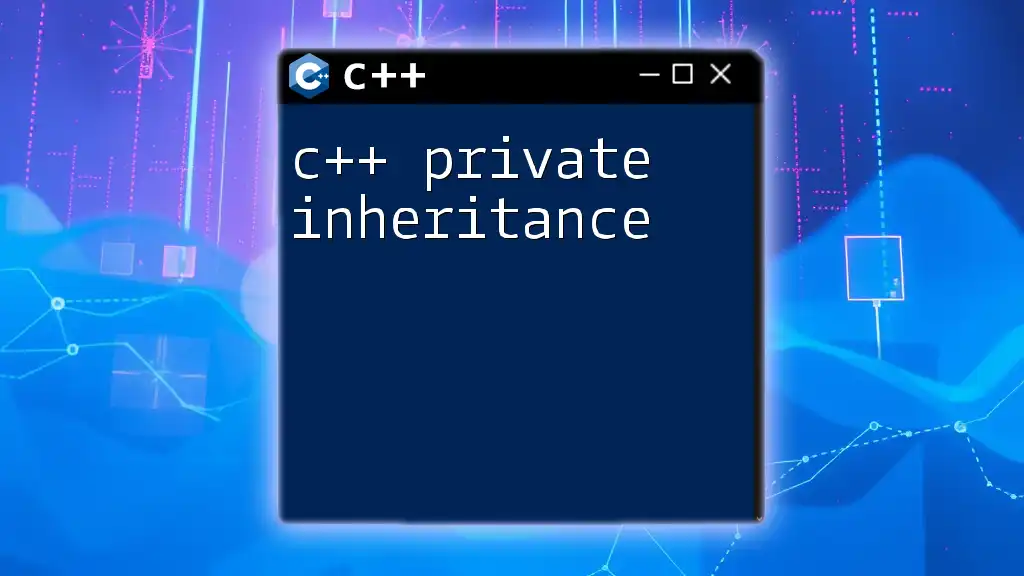
Additional Resources
Useful Links
For further exploration, refer to the official documentation for:
- Conan: [Conan Documentation](https://docs.conan.io/)
- vcpkg: [vcpkg Documentation](https://github.com/microsoft/vcpkg)
- Hunter: [Hunter Documentation](https://github.com/ruslo/hunter)
Recommended Tools for C++ Development
While package managers are crucial, combining them with powerful IDEs like Visual Studio, CLion, or VS Code can enrich your development experience, providing you with powerful debugging and compiling capabilities.
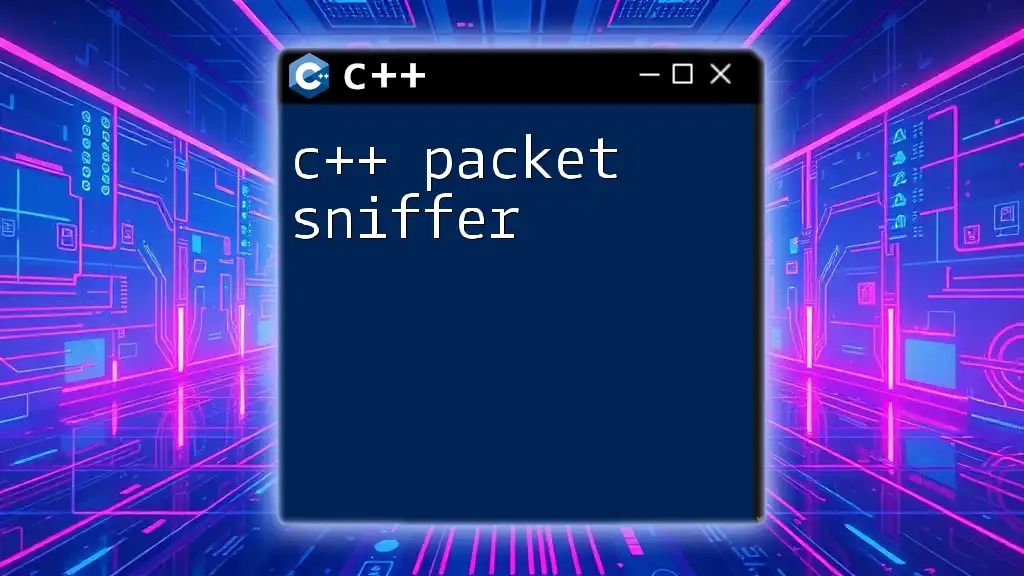
FAQs
What is the best package manager for C++?
The best package manager depends on your specific project requirements and the ecosystems you’re working with. Conan is great for flexibility, while vcpkg is excellent for Visual Studio users.
Can I use multiple package managers in one project?
Using multiple package managers in a single project can lead to complications. It's recommended to stick to one to avoid version conflicts.
How do I contribute to a package manager?
Many package managers have community contribution guidelines on their official websites. You can participate by submitting packages, reporting issues, or contributing to documentation.
By understanding and utilizing a C++ package manager effectively, you’ll greatly enhance your development productivity and simplify managing repositories and dependencies within your C++ projects.