A C++ bank management system is a console application that allows users to perform basic banking operations like creating accounts, depositing, withdrawing, and checking balance using structured programming concepts.
Here's a simple code snippet demonstrating basic account operations in C++:
#include <iostream>
#include <string>
using namespace std;
class BankAccount {
public:
string name;
double balance;
BankAccount(string accName) {
name = accName;
balance = 0.0;
}
void deposit(double amount) {
balance += amount;
cout << "Deposited: " << amount << endl;
}
void withdraw(double amount) {
if (amount <= balance) {
balance -= amount;
cout << "Withdrew: " << amount << endl;
} else {
cout << "Insufficient balance!" << endl;
}
}
void checkBalance() {
cout << "Balance: " << balance << endl;
}
};
int main() {
BankAccount account("John Doe");
account.deposit(1000);
account.withdraw(500);
account.checkBalance();
return 0;
}
Understanding the Bank Management System
What is a Bank Management System?
A Bank Management System (BMS) is a software solution that automates the basic functions of a bank, facilitating efficient management of its operations and helping to enhance customer experience. It performs various tasks such as user registration, account management, transaction processing, and reporting. The digital transformation of banking through such software not only increases operational efficiency but also mitigates the risk of human errors.
Key Features of a Bank Management System
A robust C++ bank management system encompasses several critical features:
- User Registration and Authentication: The system securely stores user information and ensures that only authorized individuals can access sensitive data by implementing authentication protocols.
- Account Management: Users can create, modify, and delete their accounts with ease, enhancing user experience.
- Transaction Management: The system can handle deposits, withdrawals, and transfers between accounts, ensuring seamless financial operations.
- Balance Inquiry: Users can quickly check their account balances, promoting transparency and trust.
- Reporting and Data Analytics: The system generates essential reports that fulfill regulatory requirements and provide insights into financial performance.
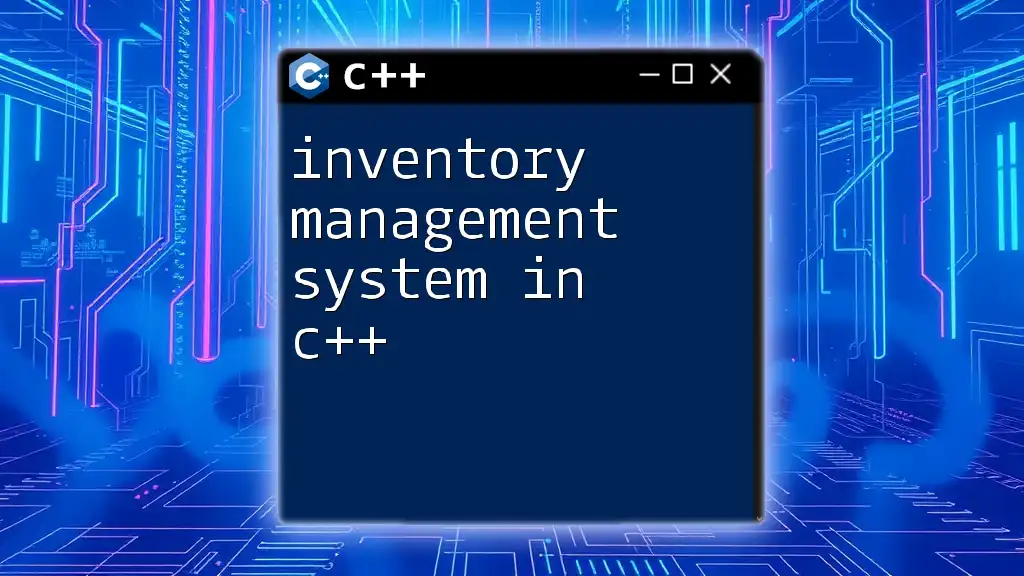
Setting Up the Development Environment
Required Tools and Software
To develop a C++ bank management system, you need several tools:
- Compiler: Popular options include GCC and Visual Studio. These compilers translate C++ code into machine-readable format.
- IDE: Choose an Integrated Development Environment like Code::Blocks or Eclipse, which provides a user-friendly way to write, debug, and compile C++ code.
- Libraries: Depending on the complexity of your system, libraries like Boost or SQLite can be beneficial for added functionalities, such as database management.
Installing and Configuring Your Environment
- Installing a Compiler: Choose and download a suitable compiler for your operating system. For Windows, you might consider installing MinGW, while Linux users can easily set up GCC via the package manager.
- Setting Up an IDE: After installing a compiler, download your preferred IDE. Ensure that it integrates seamlessly with the compiler for efficient code management.
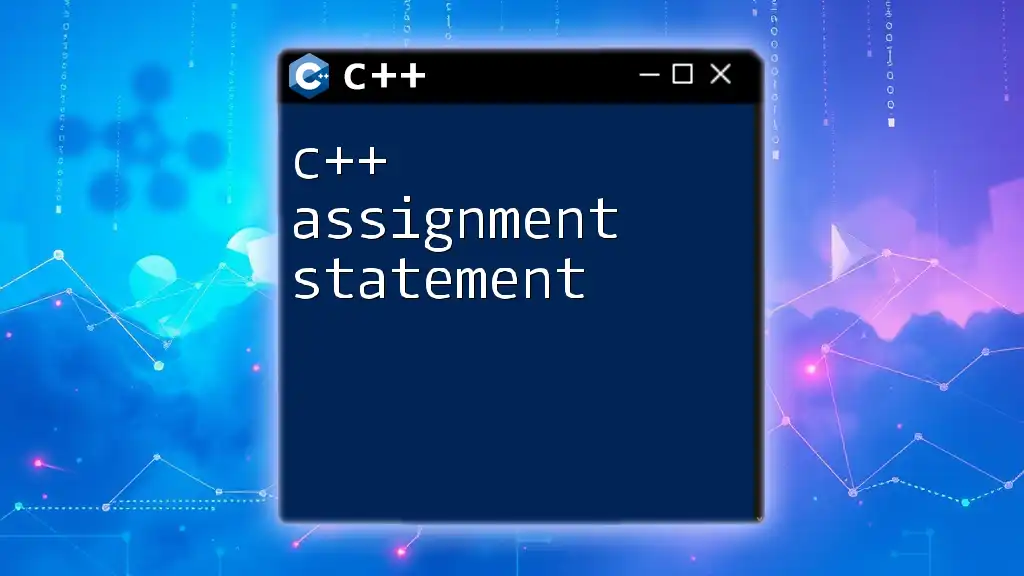
Building the Bank Management System
Project Structure
A well-organized project structure is crucial for the maintainability of the code. You might want to create separate folders for header files, source codes, and resources like documentation. This organization allows for easy navigation and enhances collaboration if more developers join.
Core Classes and Their Responsibilities
The heart of a C++ bank management system lies in its core classes that encapsulate various functionalities:
User Class
The User class manages the users of the banking system. It contains attributes to store user-specific data such as user ID, name, and password.
class User {
public:
void registerUser();
bool loginUser(std::string password);
private:
int userID;
std::string name;
std::string password;
};
Account Class
Next, the Account class focuses on account-related operations, including the ability to create, delete, or retrieve account details.
class Account {
public:
void createAccount();
double getBalance();
private:
int accountNumber;
double balance;
};
Transaction Class
Transactions are a fundamental aspect of any bank management system, which the Transaction class handles with operations for deposits, withdrawals, and transfers.
class Transaction {
public:
void deposit(double amount);
void withdraw(double amount);
private:
int transactionID;
double amount;
};
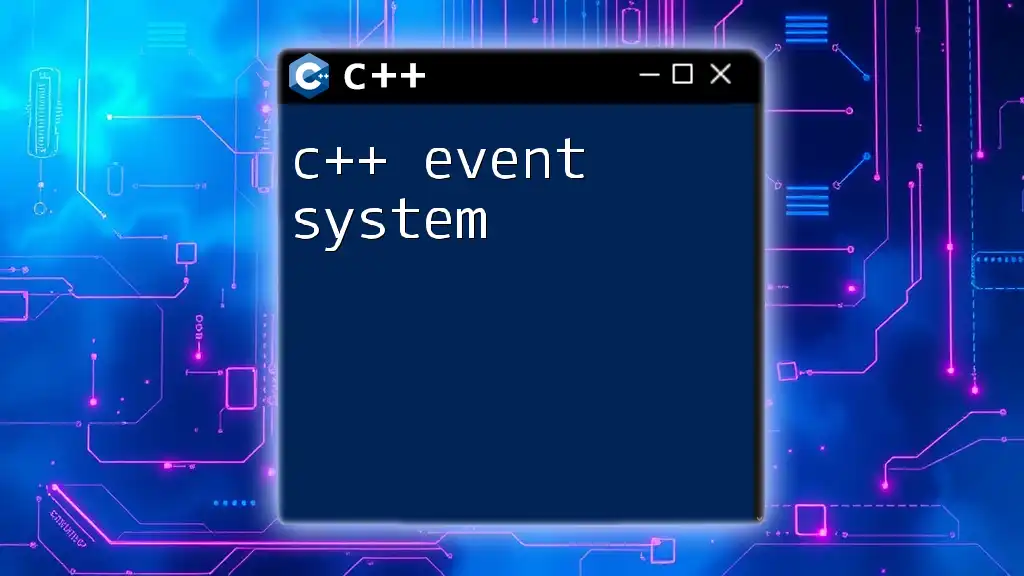
Implementing User Registration and Authentication
User Registration Flow
The user registration process is essential for initializing new users in the system. It collects user details, such as name and password, and stores them securely.
void User::registerUser() {
// Sample implementation
// Collect user details and save them in a database/file
}
User Login Process
Logging in is the next step after registration. It verifies user credentials and grants access to the bank management features.
bool User::loginUser(std::string password) {
// Validate password and return status
}
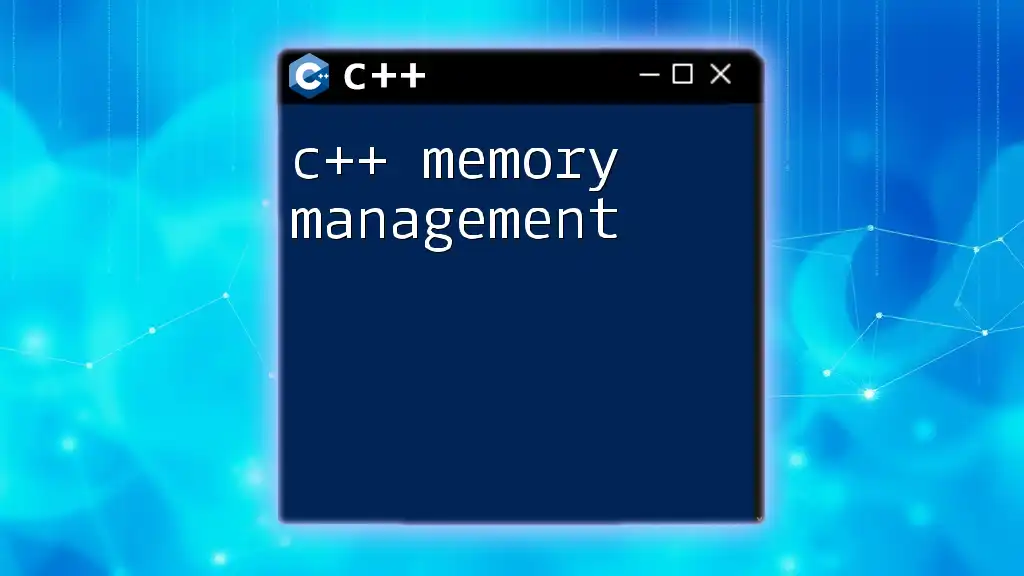
Account Management
Creating a New Account
Users should be able to create accounts effortlessly. The createAccount method should handle user inputs and account settings.
void Account::createAccount() {
// Implementation logic for creating an account
}
Deleting an Account
Account deletion should be straightforward while ensuring that it adheres to banking regulations and user authorization.
void Account::deleteAccount() {
// Implementation for account deletion
}
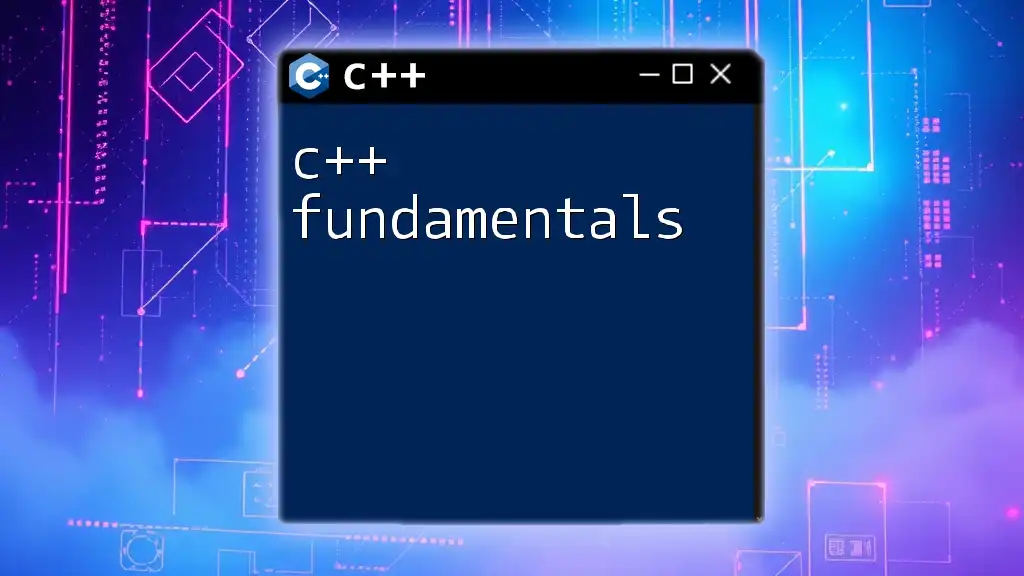
Transaction Management
Deposits
Deposits are critical for banking operations. The deposit feature allows users to add money to their accounts.
void Transaction::deposit(double amount) {
// Add the amount to the balance
}
Withdrawals
Users can also withdraw funds from their account. The withdrawal method ensures that the process checks for sufficient balance.
void Transaction::withdraw(double amount) {
// Deduct amount from the balance
}
Transfers
The transfer function must also be integrated to facilitate moving funds between accounts securely.
void Transaction::transfer(Account& toAccount, double amount) {
// Transfer mechanism
}
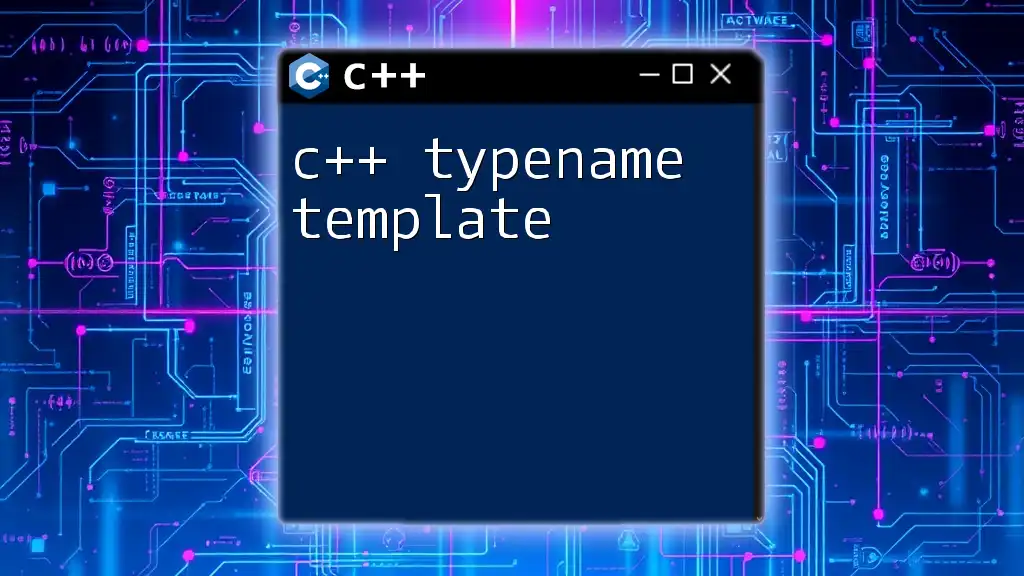
User Interface Design
Console vs. GUI-Based Interface
Choosing between a console-based or GUI-based interface often depends on your target audience. Console applications are easier and quicker to develop, whereas GUI applications can provide a more engaging user experience.
Basic Console Interface Example
For a simpler approach to interaction, a console menu can be implemented. Below is a basic implementation showcasing how users can interact with the system.
void displayMenu() {
std::cout << "1. Register\n";
std::cout << "2. Login\n";
std::cout << "3. Exit\n";
}
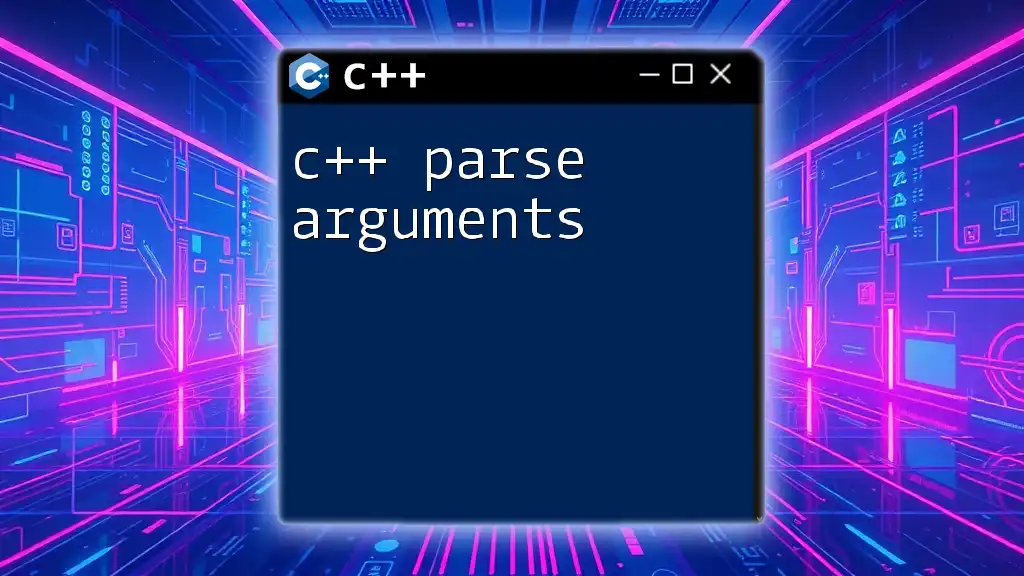
Conclusion
A C++ bank management system serves as a pivotal tool for both banking institutions and their customers. By providing secure user authentication, effective account management, and streamlined transaction processing, banks can deliver improved services. Developers are encouraged to expand upon this system, perhaps introducing additional features such as online banking or mobile access, to enhance user experience and engagement.
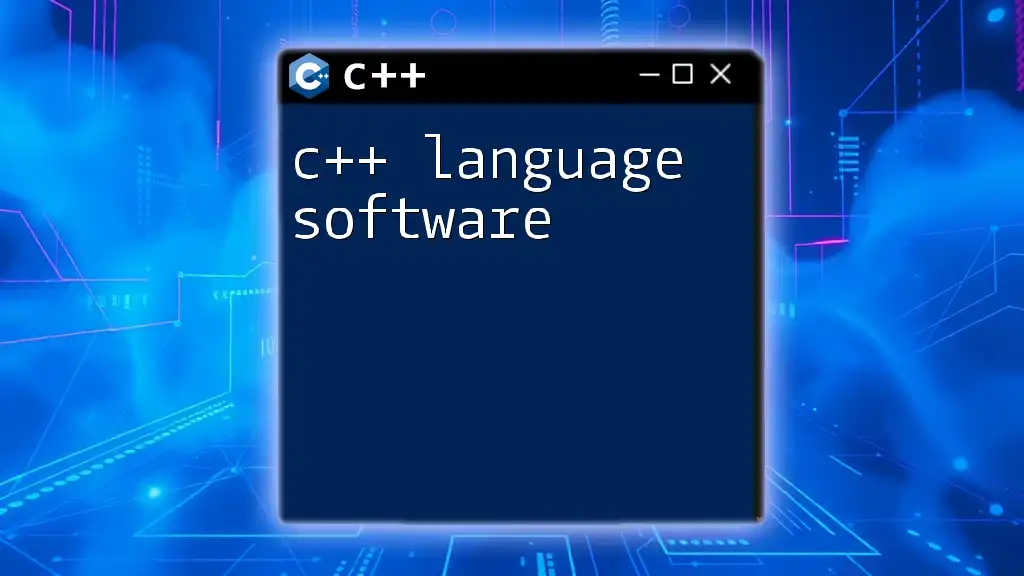
Additional Resources
Learning Materials
To deepen your understanding, consider referring to C++ programming books, taking online courses focused on system design, or exploring video tutorials that demonstrate practical implementations of banking software.
Community and Support
Engaging with communities on platforms like Stack Overflow or GitHub can be useful for seeking guidance from fellow developers. These resources often host discussions and provide feedback that can enhance your learning experience and improve your project.