An inventory management system in C++ is a program that helps track and manage stock levels, orders, and sales through structured data representation and user interactions.
Here’s a concise code snippet demonstrating a simple inventory management system in C++:
#include <iostream>
#include <vector>
#include <string>
struct Product {
std::string name;
int quantity;
};
class Inventory {
std::vector<Product> items;
public:
void addProduct(const std::string& name, int quantity) {
items.push_back({name, quantity});
}
void displayInventory() {
for (const auto& item : items) {
std::cout << "Product: " << item.name << ", Quantity: " << item.quantity << std::endl;
}
}
};
int main() {
Inventory inv;
inv.addProduct("Laptop", 5);
inv.addProduct("Mouse", 20);
inv.displayInventory();
return 0;
}
Understanding Inventory Management
Inventory management is crucial for any business that sells products, serving as the backbone for effective stock control, order processing, and database management. An inventory management system provides businesses with a structured way to track the stock of goods, ensuring that inventory levels are optimized while minimizing costs. The importance of effective inventory management cannot be understated, as it directly impacts customer satisfaction and operational efficiency.
Key Concepts in Inventory Management
Effective inventory management involves several key concepts, including:
- Stock Control: Understanding how to monitor items in stock, ensuring supply meets demand without overstocking.
- Order Processing: Managing the flow of goods from suppliers to customers, including tracking orders and shipments.
- Database Management: Storing and retrieving product data efficiently for easy access and updates.
Types of Inventory Management System
There are primarily two types of inventory management systems:
- Manual vs. Automated Systems: Manual systems rely on spreadsheets and paper, while automated systems leverage software to streamline processes.
- Cloud-based vs. Local Systems: Cloud-based systems offer remote access and collaboration, whereas local systems may provide faster performance but limited accessibility.
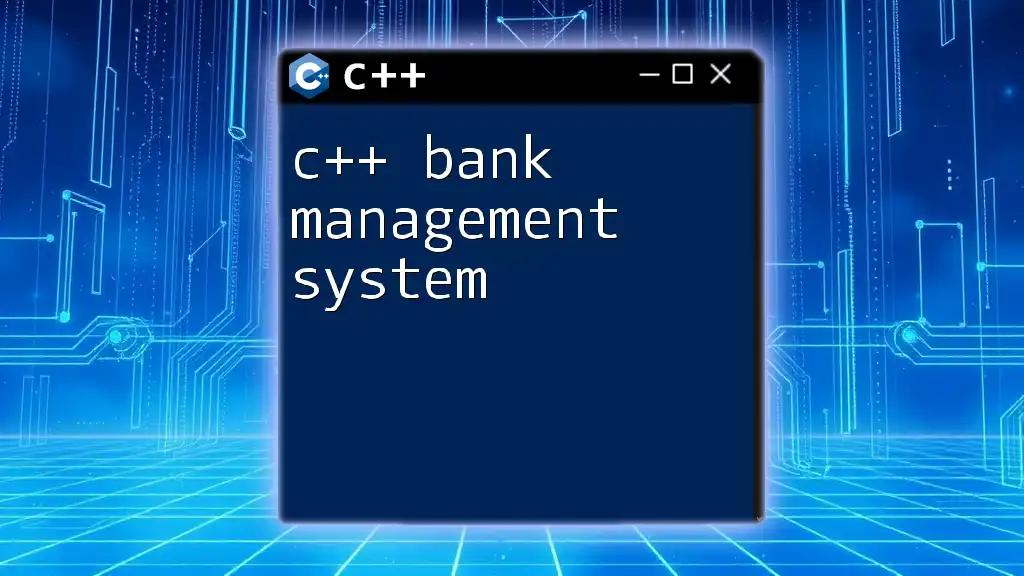
Setting Up the Development Environment
Before diving into coding, setting up the right tools and software is essential for developing an inventory management system in C++. You will need a C++ compiler such as GCC or MinGW and an Integrated Development Environment (IDE) like Code::Blocks or Visual Studio.
Creating the Project Structure
A well-organized project directory can enhance maintainability. You should consider the following directory structure:
inventory_management/
|-- src/ # Source code files
|-- include/ # Header files
|-- bin/ # Compiled binaries
|-- data/ # Data files, if any

Designing the Inventory Class
Defining the Inventory Class
The cornerstone of our system will be the InventoryItem class, which will encompass important attributes and methods to manage inventory items effectively. The class structure might look like this:
class InventoryItem {
public:
int productID;
std::string name;
std::string description;
int quantity;
double price;
void addItem(int id, std::string itemName, std::string itemDesc, int qty, double cost);
void removeItem(int id);
void updateItem(int id, int newQty, double newPrice);
void displayItems();
};
Explanation of the Class Components
- Attributes: They represent the properties of each item, such as product ID, name, description, quantity, and price.
- Methods: These functions will handle adding, removing, updating, and displaying inventory items.

Implementing Basic Inventory Functions
Adding Items to Inventory
To add items to our inventory, we'll implement the `addItem()` method. Here’s how it looks:
void InventoryItem::addItem(int id, std::string itemName, std::string itemDesc, int qty, double cost) {
productID = id;
name = itemName;
description = itemDesc;
quantity = qty;
price = cost;
}
This function efficiently captures and stores product details. For example, to add a new Laptop, you might call:
InventoryItem laptop;
laptop.addItem(101, "Laptop", "High performance laptop", 10, 999.99);
Removing Items from Inventory
Removing items is straightforward with the `removeItem()` method, which cleans up our inventory when items are sold out.
void InventoryItem::removeItem(int id) {
if (this->productID == id) {
// Logic to remove item
std::cout << "Item removed: " << name << std::endl;
}
}
For instance, if a product with ID `101` is sold out, we call `laptop.removeItem(101);`.
Updating Item Details
To ensure our stock levels and prices are always accurate, we’ll implement `updateItem()`:
void InventoryItem::updateItem(int id, int newQty, double newPrice) {
if (this->productID == id) {
this->quantity = newQty;
this->price = newPrice;
}
}
This method allows us to update an item’s quantity or price, reflecting current market conditions.
Displaying Inventory Items
Our final basic function, `displayItems()`, will present users with current inventory data:
void InventoryItem::displayItems() {
std::cout << "ID: " << productID << ", Name: " << name << ", Quantity: " << quantity << ", Price: " << price << std::endl;
}
This command outputs item details clearly, enabling quick assessments of available stock.

User Input and Interaction
Creating a user-friendly interface is vital for managing our inventory system. We could implement console-based interaction that is straightforward and functional.
Implementing a Simple Menu
To facilitate navigation, create a menu system:
void displayMenu() {
std::cout << "1. Add Item\n";
std::cout << "2. Remove Item\n";
std::cout << "3. Update Item\n";
std::cout << "4. Display All Items\n";
std::cout << "5. Exit\n";
}
Pairing this menu with user input allows seamless management of inventory functions.

Managing Inventory Data
Storing Inventory in a File
A key aspect of any inventory management system is data persistence. To achieve this, you can store inventory in a file, commonly in CSV or JSON formats. This allows you to save and retrieve your data easily.
Code Snippet for File Handling
The implementation might involve file streams:
# include <fstream>
void saveToFile(const std::vector<InventoryItem>& items) {
std::ofstream outFile("inventory.txt");
for (const auto& item : items) {
outFile << item.productID << "," << item.name << "," << item.description << ","
<< item.quantity << "," << item.price << "\n";
}
outFile.close();
}
Discuss Serialization of Objects
Serialization is a technique that converts an object into a format that can be easily stored and reconstructed later. It is important in inventory systems for safely saving and loading complex objects.
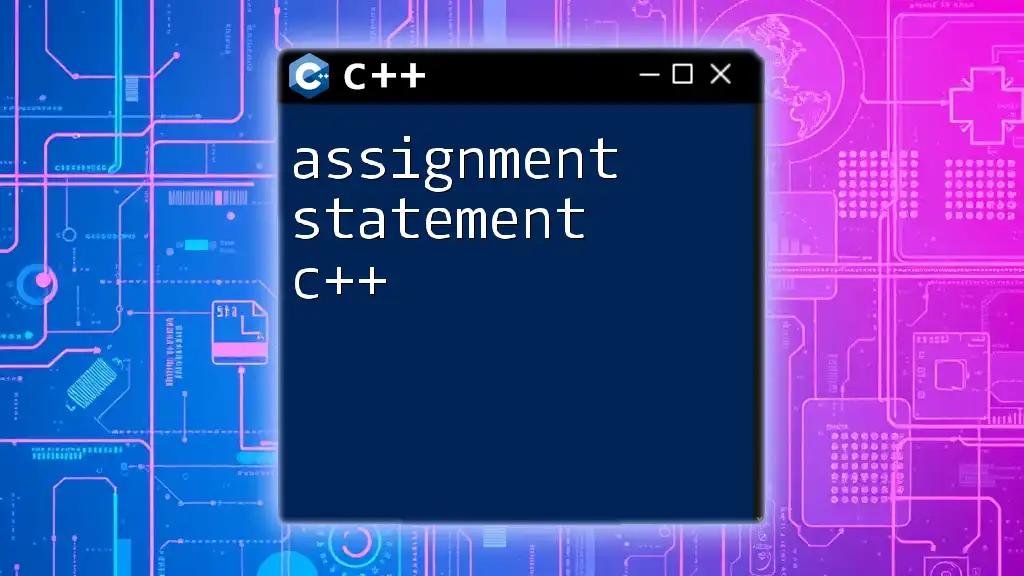
Advanced Features
Implementing Search Functionality
An effective inventory management system should allow users to quickly find items. You can implement a search function to locate an item by its name or ID. Example code for searching might look like:
InventoryItem* searchItem(int id, std::vector<InventoryItem>& items) {
for (auto& item : items) {
if (item.productID == id) {
return &item;
}
}
return nullptr;
}
Handling Low Stock Notifications
Low stock notifications can significantly impact customer satisfaction. Implement logic that checks inventory levels and notifies you when they fall below a designated threshold.
Multi-User Capabilities (Optional)
If scalability is a goal, consider implementing multi-user access. This involves threading and synchronization concepts to handle simultaneous operations, ensuring data integrity.

Conclusion
Throughout this guide, we explored the critical components involved in creating an inventory management system in C++. From setting up your environment to designing essential functions for managing inventory, you have the tools necessary to implement a robust system. As you continue your learning journey, consider expanding into databases or GUI applications to create a more sophisticated solution. Engage with fellow learners and fellow developers, and never hesitate to ask questions. This community is here to support your growth and success in C++ programming.