Primitive data types in C++ are the basic building blocks of data manipulation and include types such as `int`, `char`, `float`, and `double`. Here's a simple code snippet demonstrating each type:
#include <iostream>
using namespace std;
int main() {
int age = 25; // integer type
char initial = 'A'; // character type
float height = 5.9; // floating-point type
double pi = 3.14159; // double-precision floating-point type
cout << "Age: " << age << ", Initial: " << initial << ", Height: " << height << ", Pi: " << pi << endl;
return 0;
}
Understanding Data Types in C++ Language
Data types play a critical role in C++ programming, acting as a conduit between your code and the data it manipulates. They define the kind of data a variable can hold and determine how much space it consumes in memory. Understanding these data types is essential for writing efficient, effective C++ code.
What are Data Types?
A data type is a classification that specifies which type of value a variable can hold, what operations can be performed on it, and how much memory is allocated for it. In C++, these are primarily divided into two categories:
- Primitive (or built-in) data types: These are the most basic data types provided directly by the language, such as integers and characters.
- User-defined data types: These include structures, unions, classes, and enumerations created by developers to better suit specific applications.
Why Choose Primitive Data Types?
Primitive data types are fundamental to C++ programming because they are simple and efficient. They are generally faster due to their smaller memory footprint, and they allow the compiler to optimize performance.
Additionally, primitive data types are inherent to the language's syntax, providing a direct and intuitive method for representing basic values. Understanding these types is crucial for anyone looking to become proficient in C++.
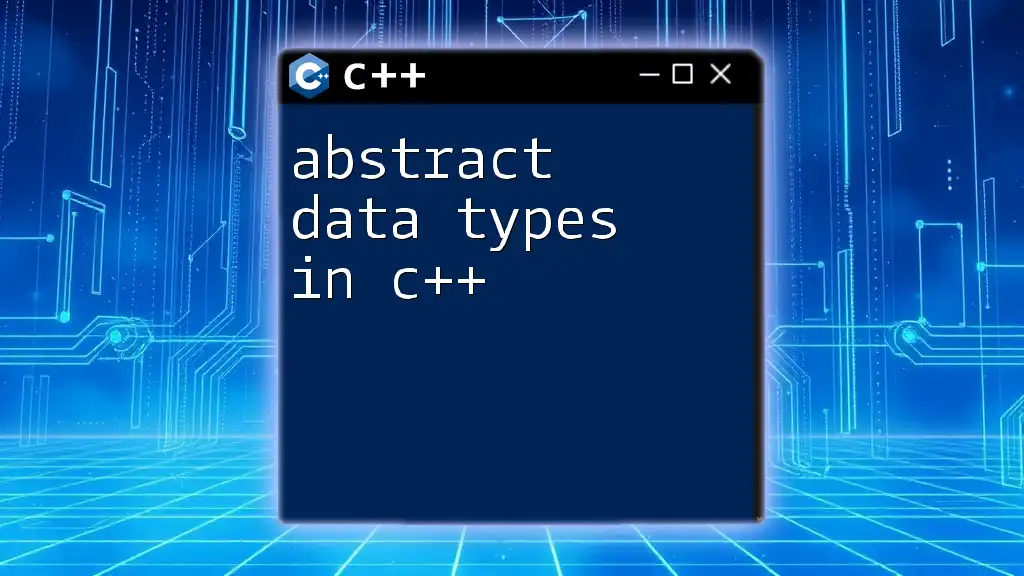
The Different Primitive Data Types in C++
C++ includes several primitive data types, each serving a unique function. Let’s delve into each type to better understand them.
Integer Data Types
Overview of Integers
Integer data types are used to store whole numbers, both positive and negative. C++ provides four variations of integers:
- int: Standard integer, typically 4 bytes.
- short: Smaller integer, generally 2 bytes.
- long: Larger integer, often 4 or 8 bytes depending on the system.
- long long: Guaranteed to be at least 8 bytes.
Memory allocation and the range of values they can hold can be system dependent, so it’s always good practice to check the limits based on the specific compiler used.
Code Example
#include <iostream>
using namespace std;
int main() {
int a = 10; // Standard integer
long b = 100000L; // Long integer
short c = 5; // Short integer
long long d = 10000000000LL; // Long long integer
cout << "Integer: " << a << ", Long: " << b << ", Short: " << c << ", Long Long: " << d << endl;
return 0;
}
Here, the different integer types allow for varying sizes and values, making them flexible for a range of applications.
Floating Point Data Types
Overview of Floating Point Types
For representing real numbers, floating point data types are suitable. C++ supports three types:
- float: Single precision floating point, usually 4 bytes.
- double: Double precision floating point, generally 8 bytes. Offers more precision than float.
- long double: Extended precision floating point, typically more than 8 bytes.
The floating point numbers can represent fractional values, which is useful in scenarios where precision is crucial, such as scientific calculations.
Code Example
#include <iostream>
using namespace std;
int main() {
float x = 5.75f; // Single precision
double y = 19.99; // Double precision
long double z = 12345.6789L; // Extended precision
cout << "Float: " << x << ", Double: " << y << ", Long Double: " << z << endl;
return 0;
}
By choosing the appropriate floating point type, you can optimize both memory use and computational efficiency.
Character Data Types
Understanding Characters
Character data types are used for representing individual characters, such as letters and symbols. The primary character type in C++ is:
- char: Represents a single character and usually occupies 1 byte.
C++ also provides variations to support internationalization:
- wchar_t: Represents wide characters, often used for languages with larger alphabets like Chinese or Japanese.
- char16_t and char32_t: Represent UTF-16 and UTF-32 encoded characters, respectively.
Code Example
#include <iostream>
using namespace std;
int main() {
char ch = 'A'; // Standard character
wchar_t wch = L'あ'; // Wide character
cout << "Character: " << ch << ", Wide Character: " << wch << endl;
return 0;
}
Using character types makes it easy to handle text, ensuring your applications can manage language and character set variations.
Boolean Data Type
Understanding Boolean Values
The boolean data type is used to represent truth values - true and false. In C++, it is defined using the keyword `bool`. Boolean types are significantly utilized in decision-making and control structures like loops and conditionals.
Code Example
#include <iostream>
using namespace std;
int main() {
bool isCoding = true;
if(isCoding) {
cout << "Keep coding!" << endl;
} else {
cout << "Take a break!" << endl;
}
return 0;
}
The boolean type is crucial for implementing logic in your applications, allowing for clear and concise conditional statements.
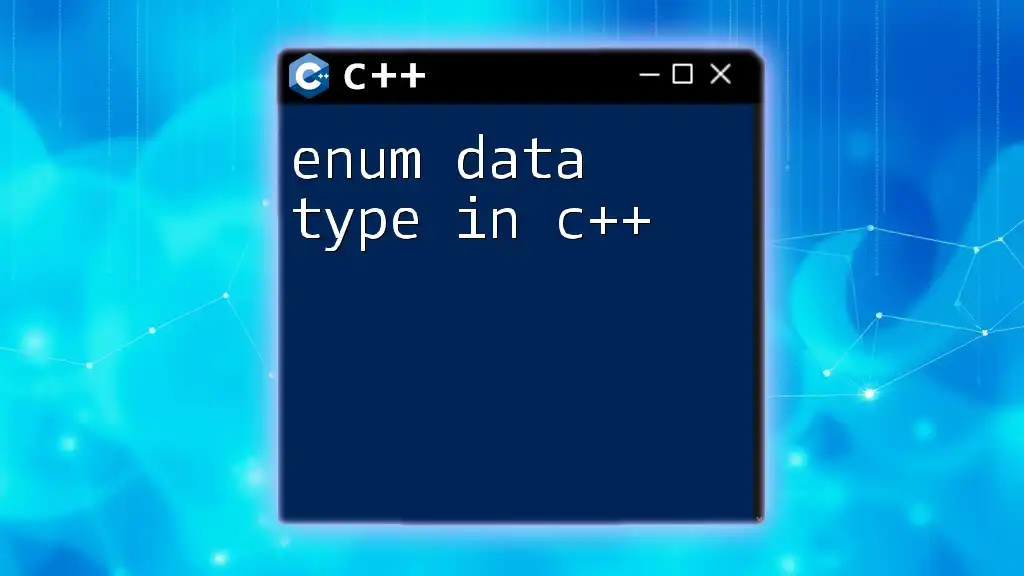
Type Modifiers and Their Impact
Overview of Type Modifiers
C++ allows modification of its primitive data types through type modifiers, which can alter the size and sign of a data type. The primary type modifiers include:
- signed: Indicates that the variable can hold both positive and negative values.
- unsigned: Indicates that the variable can only hold non-negative values.
- short: Modifies the integer types to become smaller.
- long: Modifies the integer types to become larger.
Combining Modifiers with Primitive Data Types
By combining these modifiers with primitive data types, developers can optimize their programs based on requirements, memory constraints, and expected data ranges.
Examples of Type Modifiers
#include <iostream>
using namespace std;
int main() {
unsigned int positiveNum = 42; // Non-negative number
signed int negativeNum = -42; // Can hold negative values
cout << "Unsigned Integer: " << positiveNum << ", Signed Integer: " << negativeNum << endl;
return 0;
}
Using type modifiers effectively can enhance performance and ensure that your application uses memory appropriately.
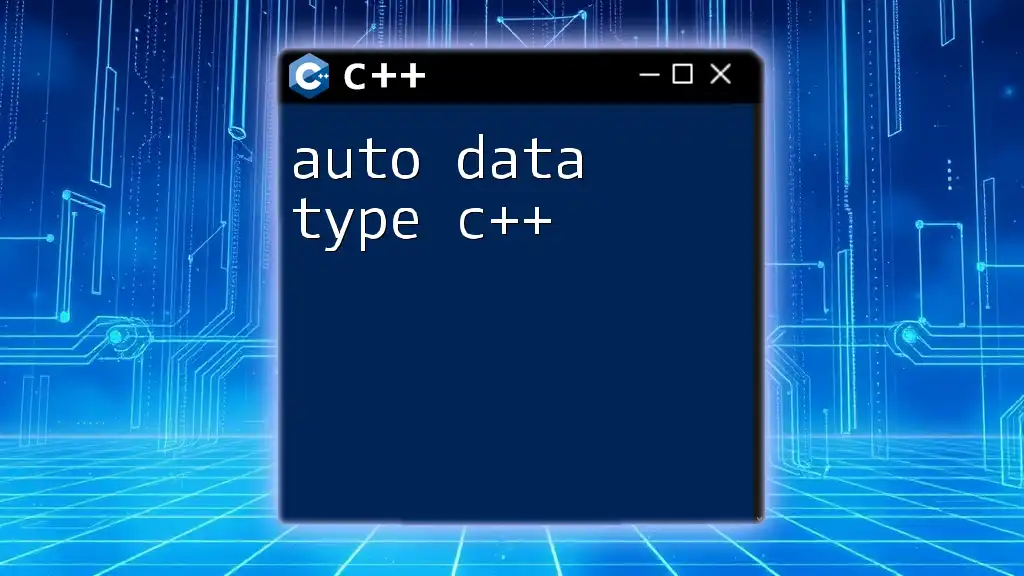
Summary of Primitive Data Types
To summarize, understanding primitive data types in C++ is fundamental for any programmer. With integer, floating point, character, and boolean types, you have ample tools to manipulate and represent data accurately. Each data type has specific properties that influence how your code performs and interacts with memory.
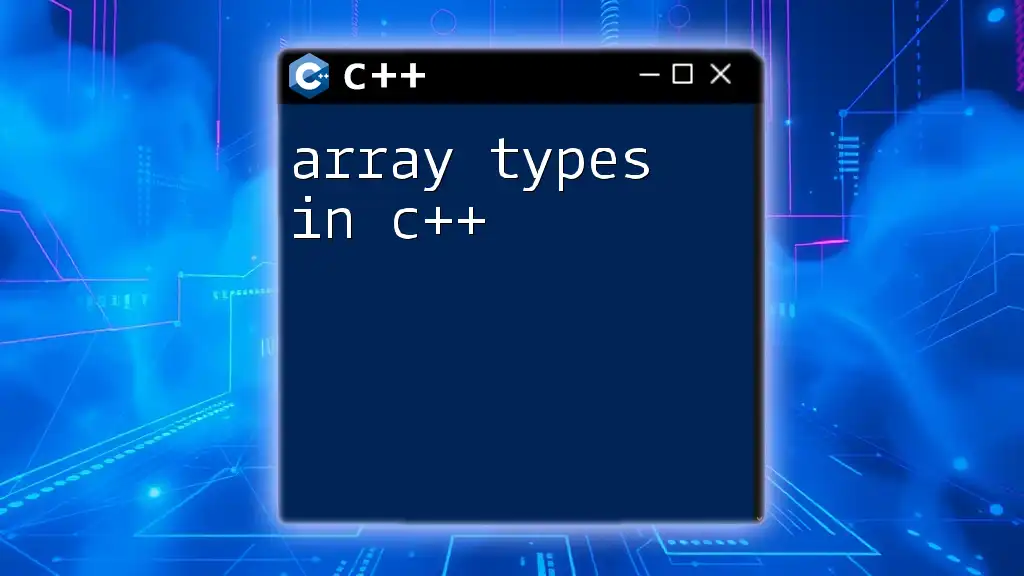
Conclusion
Having a solid grasp of primitive data types in C++ empowers you to choose the right type for your variables effectively. This not only improves the performance of your code but also enhances your programming skills overall. As you develop your projects, remember to leverage these data types to ensure efficient use of resources.
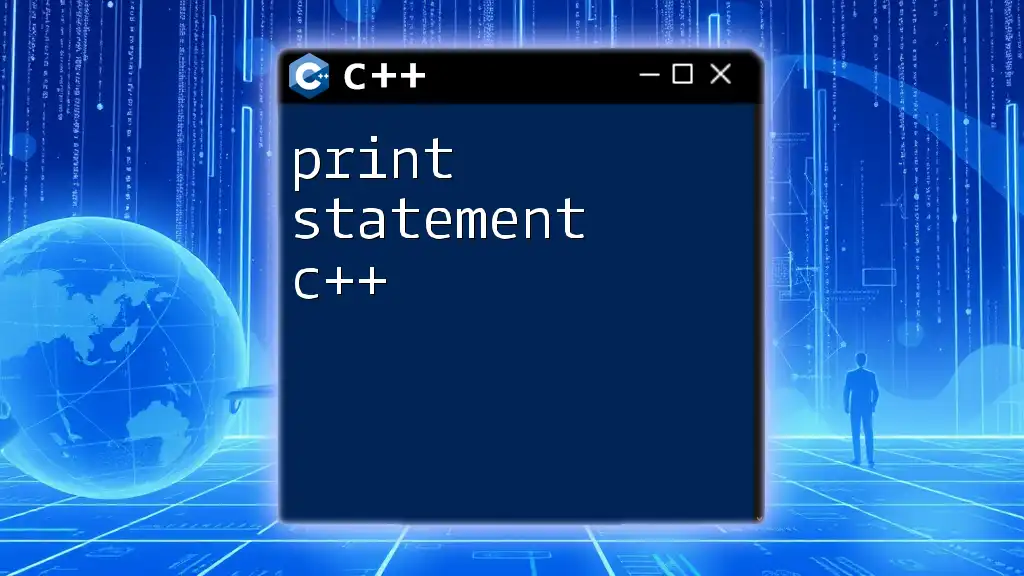
Additional Resources
To deepen your understanding of primitive data types in C++, consult the official C++ documentation as well as books and online courses targeted toward beginner and intermediate programmers. These resources will provide further insights into the nuances of C++ and its powerful capabilities.
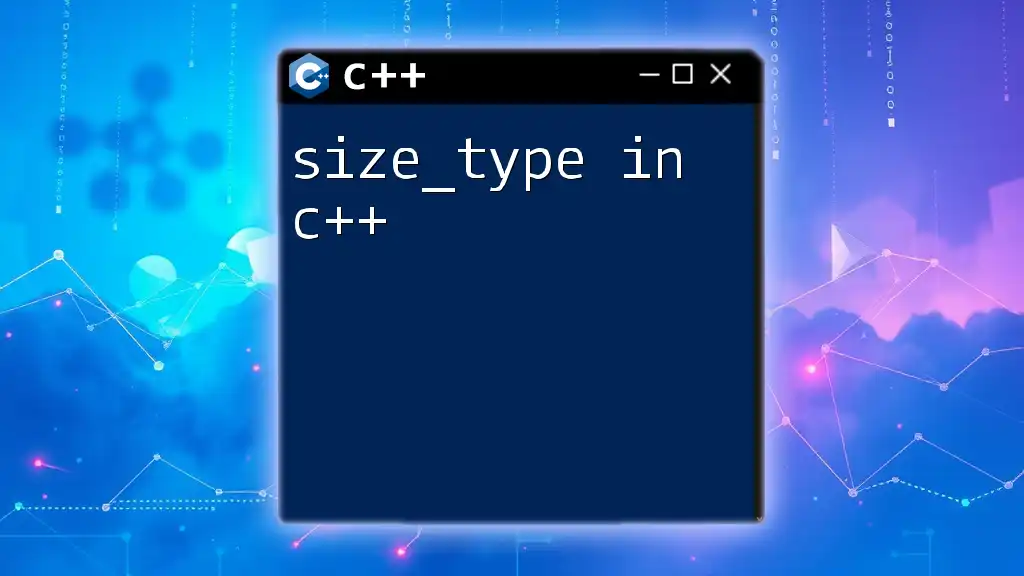
FAQ Section
What are the most common primitive data types in C++?
The common primitive data types in C++ include `int`, `float`, `double`, `char`, and `bool`.
How do I choose the right data type for my variables?
Consider the nature of your data (whole numbers vs. real numbers), the required precision, and the memory constraints of your application.
Can I create my own data types in C++? How does this relate to primitive types?
Yes, C++ supports user-defined data types through structures, classes, and unions, which can build upon primitive data types to create more complex data representations.