Abstract data types (ADTs) in C++ are user-defined types that encapsulate data and operations, allowing them to be manipulated without exposing the underlying implementation details.
Here's a simple example of an abstract data type in C++ using a stack:
#include <iostream>
#include <vector>
class Stack {
private:
std::vector<int> data;
public:
void push(int value) { data.push_back(value); }
void pop() { if (!data.empty()) data.pop_back(); }
int top() const { return data.empty() ? -1 : data.back(); }
bool isEmpty() const { return data.empty(); }
};
What is an Abstract Data Type?
An Abstract Data Type (ADT) is a mathematical model that provides a logical description of a data type and the operations that can be performed on it, without defining how these operations will be implemented. This separation between interface and implementation is crucial because it allows developers to use a data type without needing to understand the details involved in managing that data.
In programming, the concept of ADTs is fundamental as it promotes code clarity, reusability, and maintenance. By using abstract data types, you can design software that clearly separates the abstract behavior of data structures from their implementation.
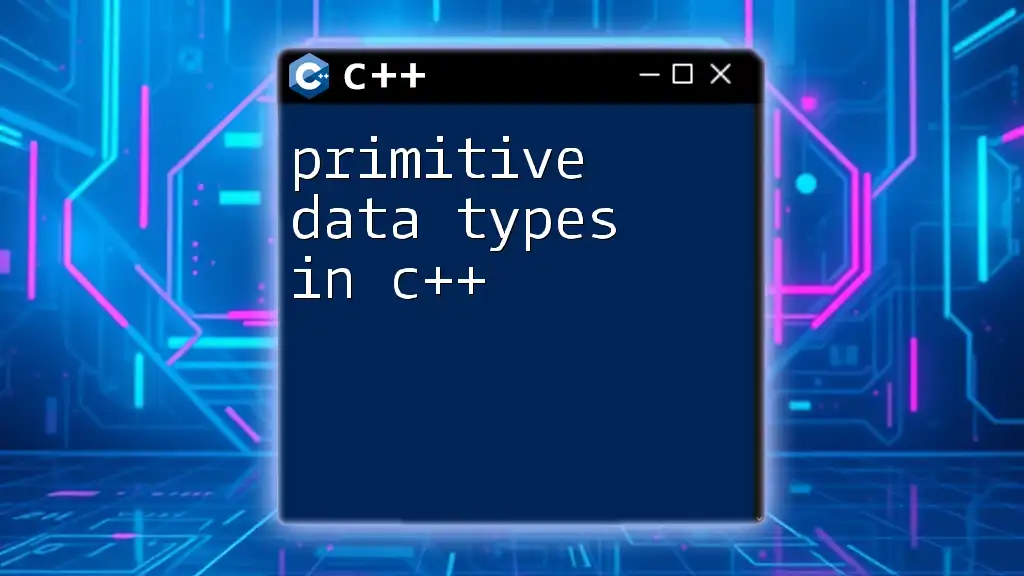
Why Use Abstract Data Types in C++?
Using abstract data types in C++ offers a multitude of benefits, including:
- Encapsulation: The implementation details of the data structure can be hidden from the user, allowing for a cleaner interface.
- Data Hiding: Internal data cannot be accessed directly; this protects data integrity and reduces potential side effects.
- Modularity: Enables developers to break down complex systems into manageable parts, enhancing maintainability.
- Ease of Use: Once defined, users can focus on the operations provided by the ADT rather than the underlying implementation.
When comparing ADTs to primitive data types in C++, it becomes clear that ADTs provide a much richer set of functionality and behavior, facilitating the development of complex software systems.
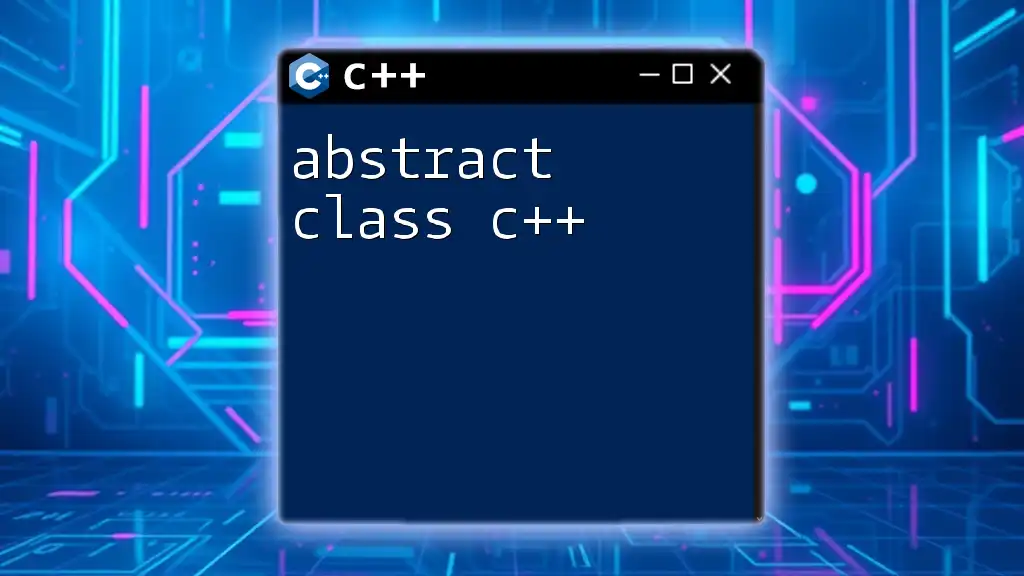
Understanding the Concept of ADTs in C++
Theoretical Foundation of ADTs
An abstract data type encompasses both the data and the operations that are allowed on that data. This encompasses not just the data itself but also the methods or functions that act on that data.
For example, consider a `List` ADT. This abstract representation includes operations such as `add`, `remove`, and `find`, without delving into whether a list is implemented as an array, a linked list, or another structure.
Key Characteristics of Abstract Data Types
The key characteristics of abstract data types include:
- Encapsulation: Internal details are hidden, exposing only the necessary operations to the user.
- Operations: ADTs define a set of operations, promoting a clear and understandable interface.
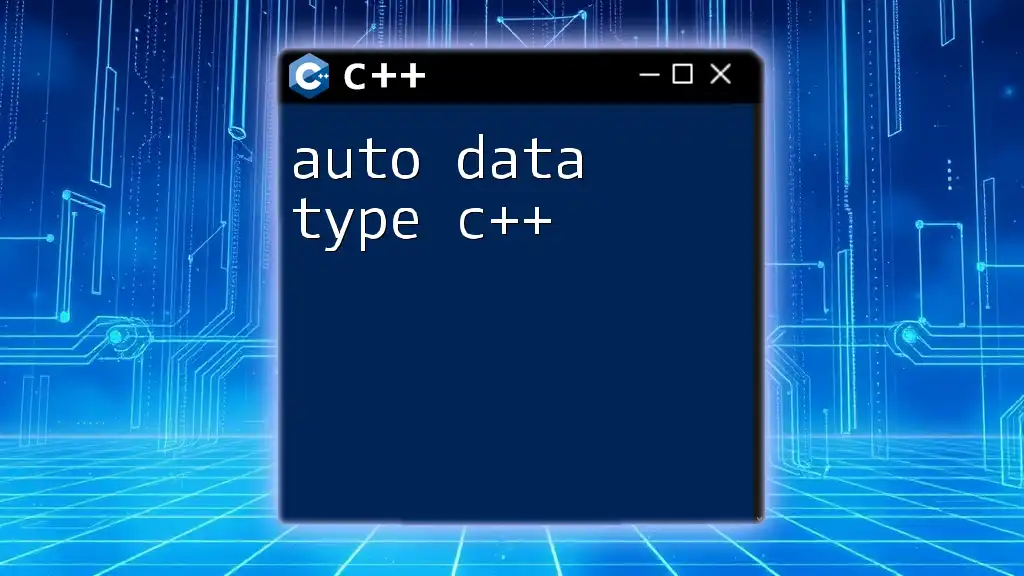
C++ ADT: The Building Blocks
Defining an ADT in C++
Defining an ADT in C++ typically involves creating a class that encapsulates its data and methods. Here’s an example of a Stack ADT:
class Stack {
private:
std::vector<int> elements; // The underlying container to store stack elements
public:
void push(int value); // Adds an element to the top of the stack
int pop(); // Removes and returns the top element
bool isEmpty() const; // Checks if the stack is empty
};
In this example, the `Stack` class encapsulates its data (`elements`) and provides three public methods to interact with that data.
Implementing Operations on ADTs
Let’s implement the core operations for the `Stack` ADT:
void Stack::push(int value) {
elements.push_back(value); // Adds the value to the end of the vector
}
int Stack::pop() {
if (isEmpty()) throw std::out_of_range("Stack is empty"); // Error handling
int value = elements.back(); // Retrieve the last element
elements.pop_back(); // Remove the last element
return value; // Return the popped value
}
bool Stack::isEmpty() const {
return elements.empty(); // Checks if there are elements in the stack
}
These methods allow users to push new elements onto the stack, pop elements off, and check if the stack is empty.
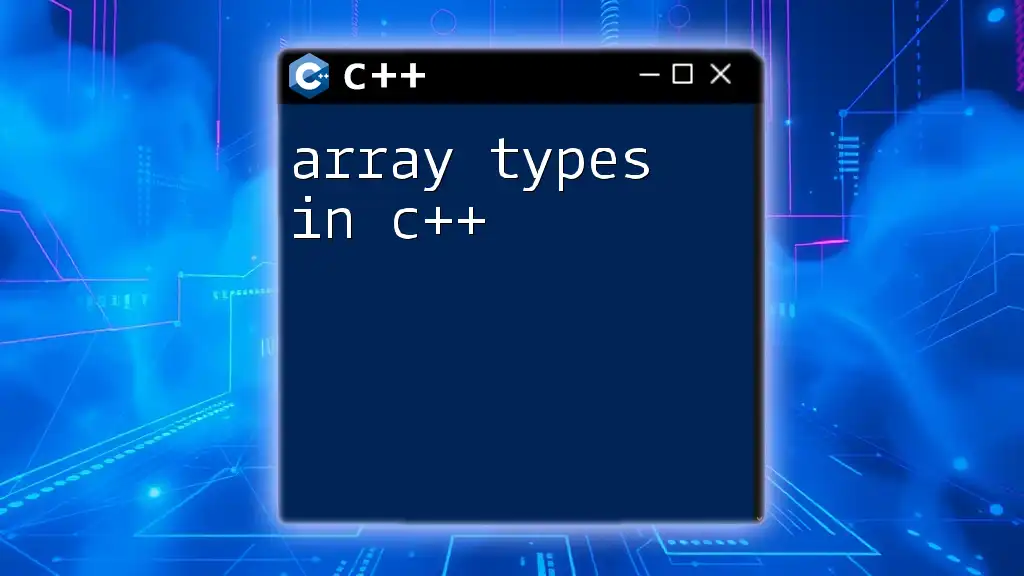
Popular Abstract Data Types in C++
List ADT in C++
The List ADT allows for dynamic collections of items. It can be implemented using different underlying structures, such as linked lists or dynamic arrays. A simple Linked List can be defined as follows:
class Node {
public:
int data; // Data stored in this node
Node* next; // Pointer to the next node
};
class LinkedList {
private:
Node* head; // Pointer to the first node in the list
public:
void insert(int value); // Inserts a new node with the given value
void remove(int value); // Removes the first node with the given value
// Additional functions could include traversal or search
};
Queue ADT Implementation
A Queue ADT operates in a first-in, first-out (FIFO) manner. Let’s look at an implementation for a Circular Queue:
class CircularQueue {
private:
int* queue; // Dynamic array to hold queue elements
int front; // Index of the front element
int rear; // Index of the rear element
int size; // Total size of the queue
public:
CircularQueue(int s) : size(s), front(0), rear(-1) {
queue = new int[size]; // Memory allocation for the queue
}
void enqueue(int value); // Adds an element to the queue
int dequeue(); // Removes an element from the queue
// Additional methods can include isEmpty and isFull
};
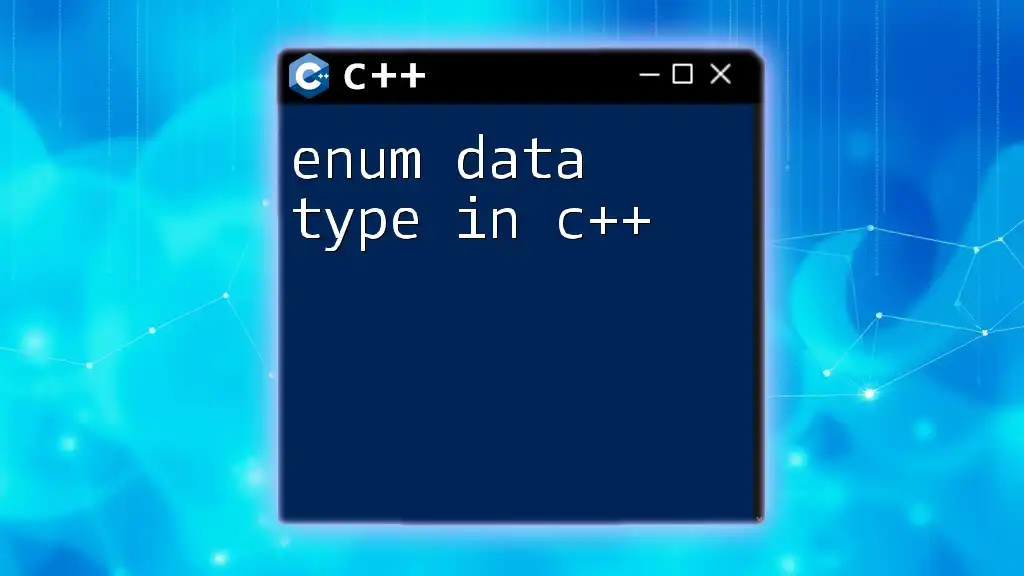
Abstract Data Types and Object-Oriented Programming
Integrating ADTs with OOP Principles
Abstract Data Types are deeply integrated with Object-Oriented Programming principles in C++. The use of encapsulation, inheritance, and polymorphism allows developers to model real-world entities effectively through abstraction.
Using Abstract Classes and Interfaces in C++
In C++, you can create abstract classes using pure virtual functions, allowing you to define interfaces. Here's an example of an abstract shape class:
class AbstractShape {
public:
virtual double area() = 0; // Pure virtual function, making this class abstract
};
class Rectangle : public AbstractShape {
private:
double width;
double height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double area() override {
return width * height; // Calculate area for rectangle
}
};
In this example, any derived class must implement the `area()` method, providing flexibility while ensuring that the concepts remain abstract.
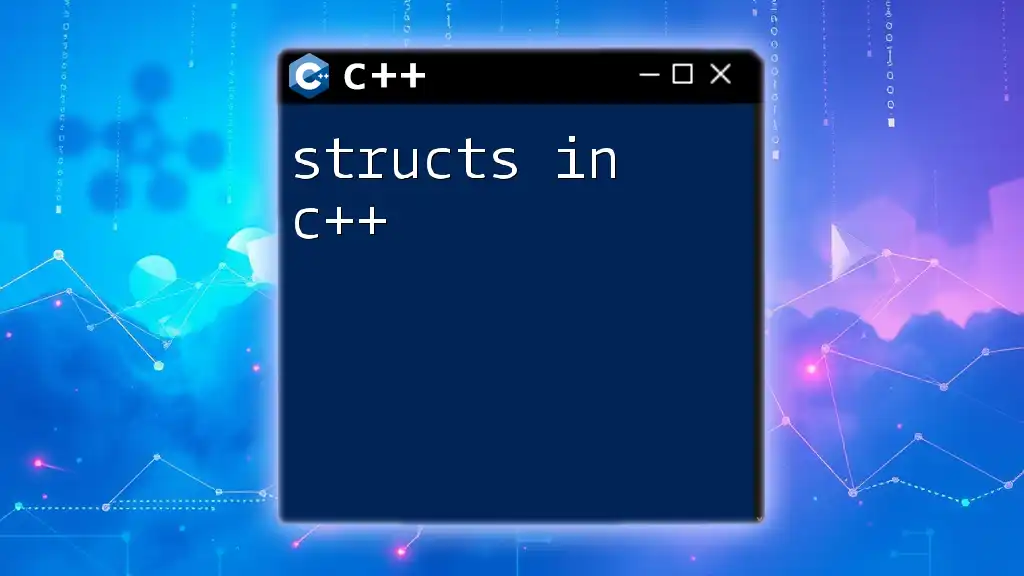
Common Use Cases for Abstract Data Types in C++
Real-World Applications of ADTs in Software Development
Abstract Data Types are utilized in numerous applications, from database management systems (using lists and trees) to graphics rendering (using shapes and polygons). By abstracting the data structure, developers can implement complex behaviors efficiently and reliably.
Performance Considerations
When to use ADTs is often a matter of balancing performance needs and abstraction benefits. While abstracting data types can lead to clearer code, sometimes direct manipulation of a specific data structure can yield better performance in critical applications. Hence, it is essential to analyze the pros and cons based on item access, insertion, and deletion speeds.
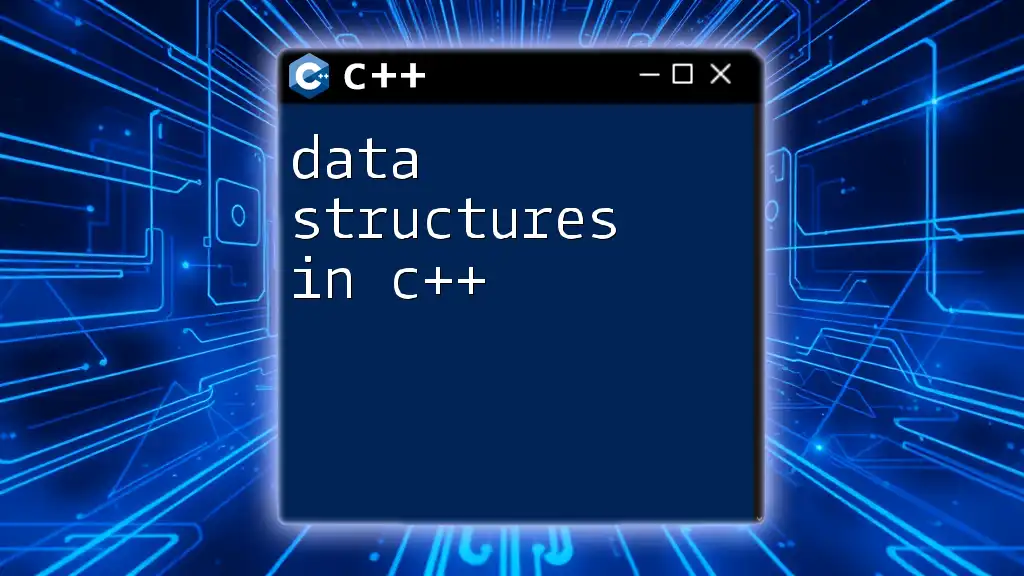
Conclusion
Abstract Data Types in C++ play a crucial role in programming, significantly improving code readability, maintainability, and modularity. Understanding how to define and implement ADTs is essential for software development and is a fundamental skill for proficient C++ programmers. The separation of interface and implementation allows developers to focus on solving complex problems without getting bogged down by unnecessary details.
Encouragement for Continued Learning
To delve deeper into abstract data types and C++, consider exploring various online resources, books, and courses designed to enhance your understanding and skills. Engaging with the community can also provide additional insights and support on your learning journey.