The Observer Pattern in C++ is a behavioral design pattern that allows a subject to notify a list of observers automatically of any state changes, promoting loose coupling between components.
Here’s a simple code snippet demonstrating the Observer Pattern in C++:
#include <iostream>
#include <vector>
#include <string>
class Observer {
public:
virtual void update(const std::string &message) = 0;
};
class Subject {
private:
std::vector<Observer*> observers;
public:
void addObserver(Observer* observer) {
observers.push_back(observer);
}
void notifyObservers(const std::string &message) {
for (Observer* observer : observers) {
observer->update(message);
}
}
};
class ConcreteObserver : public Observer {
public:
void update(const std::string &message) override {
std::cout << "Received update: " << message << std::endl;
}
};
int main() {
Subject subject;
ConcreteObserver observer1, observer2;
subject.addObserver(&observer1);
subject.addObserver(&observer2);
subject.notifyObservers("Hello Observers!");
return 0;
}
Observer Pattern in C++
What is the Observer Pattern?
The observer pattern is a behavioral design pattern that defines a one-to-many dependency between objects. When one object, known as the subject, changes state, all registered objects, referred to as observers, are notified and updated automatically. This pattern is instrumental in creating a system that can easily add or remove elements without significant changes to the core logic.
Importance of the Observer Pattern
Using the observer pattern provides several benefits in software development. Primarily, it facilitates a decoupled architecture, which enhances the maintainability of the code. Commonly used in scenarios like GUI frameworks, event handling systems, and real-time data monitors, this pattern provides a robust method for dynamic notifications across your application.
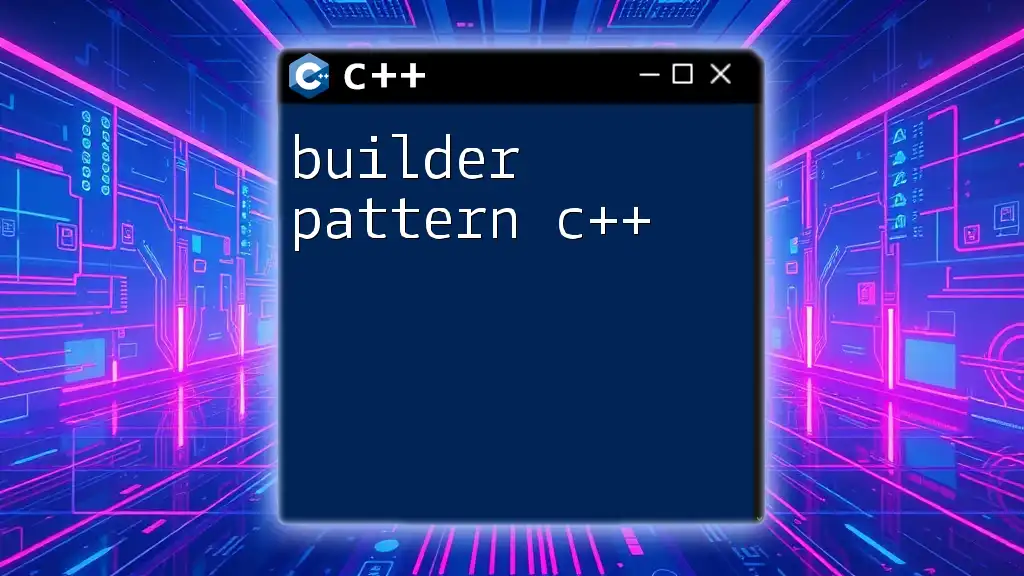
Understanding the Observer Design Pattern
Key Concepts
In the observer pattern, there are several key components:
- Observer: This represents the entity that needs to be updated whenever a change occurs in the subject.
- Subject: This is the core component that maintains a list of observers and notifies them of any updates.
- Subscription: The process through which an observer registers itself with a subject to receive notifications.
- Notification: The mechanism by which the subject informs the observers about changes.
Common Use Cases
The observer pattern finds its application across various domains:
- GUI Frameworks: UI elements often respond to user actions (like button clicks) which can be efficiently managed using the observer pattern.
- Weather Stations: Systems that monitor weather changes can notify multiple displays without tightly coupling the data source to the views.
- Real-Time Applications: Any application like stock market trackers or live comments systems where real-time updates are essential can benefit greatly from this pattern.
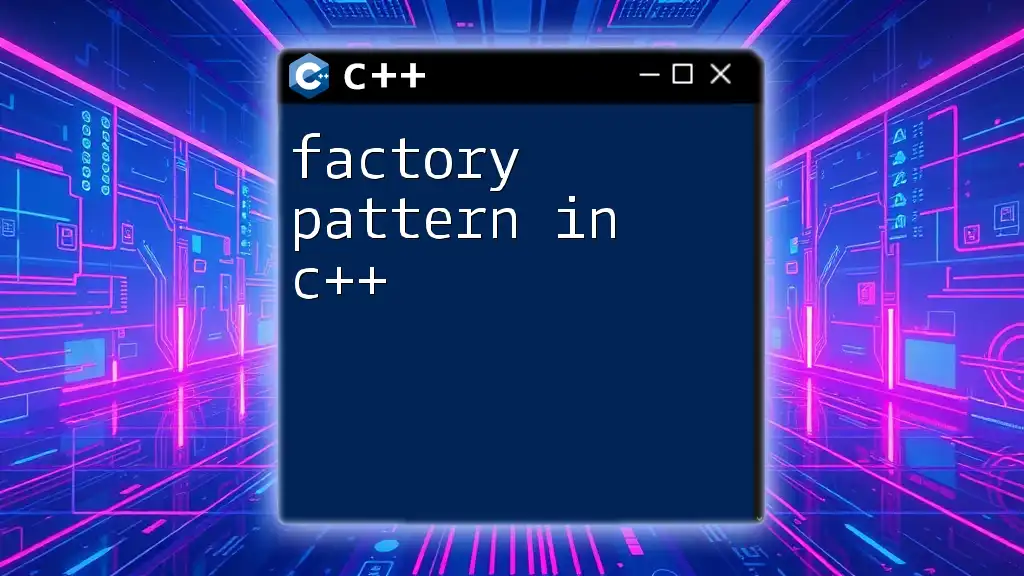
Implementing the Observer Pattern in C++
Defining the Subject Class
Subject Interface
The subject plays a central role in managing observers. It provides methods for attaching, detaching, and notifying observers. Here’s how you might define the Subject interface in C++:
class Subject {
public:
virtual void attach(Observer* observer) = 0;
virtual void detach(Observer* observer) = 0;
virtual void notify() = 0;
};
Defining the Observer Class
Observer Interface
The observer interface provides a blueprint for any class that wishes to receive updates from a subject. The typical method that an observer must implement is `update`. Here’s an example:
class Observer {
public:
virtual void update() = 0;
};
Concrete Subject Implementation
Implementing the Subject
Now, let’s implement a concrete subject. This specific subject will manage observers and notify them whenever its state changes, demonstrating the full functionality of the observer pattern:
#include <vector>
#include <algorithm>
class ConcreteSubject : public Subject {
std::vector<Observer*> observers;
// state of the subject
int state;
public:
void attach(Observer* observer) override {
observers.push_back(observer);
}
void detach(Observer* observer) override {
observers.erase(std::remove(observers.begin(), observers.end(), observer), observers.end());
}
void notify() override {
for (Observer* observer : observers) {
observer->update();
}
}
void setState(int newState) {
state = newState;
notify(); // state has changed, notify observers
}
int getState() const {
return state;
}
};
Concrete Observer Implementation
Implementing an Observer
Next, we create a concrete implementation of the observer. This observer will register with the subject and implement its response to state changes:
class ConcreteObserver : public Observer {
ConcreteSubject* subject;
public:
ConcreteObserver(ConcreteSubject* subj) : subject(subj) {
subject->attach(this);
}
void update() override {
// react to the subject's state change
std::cout << "Observer notified of state change! New state is: "
<< subject->getState() << std::endl;
}
};
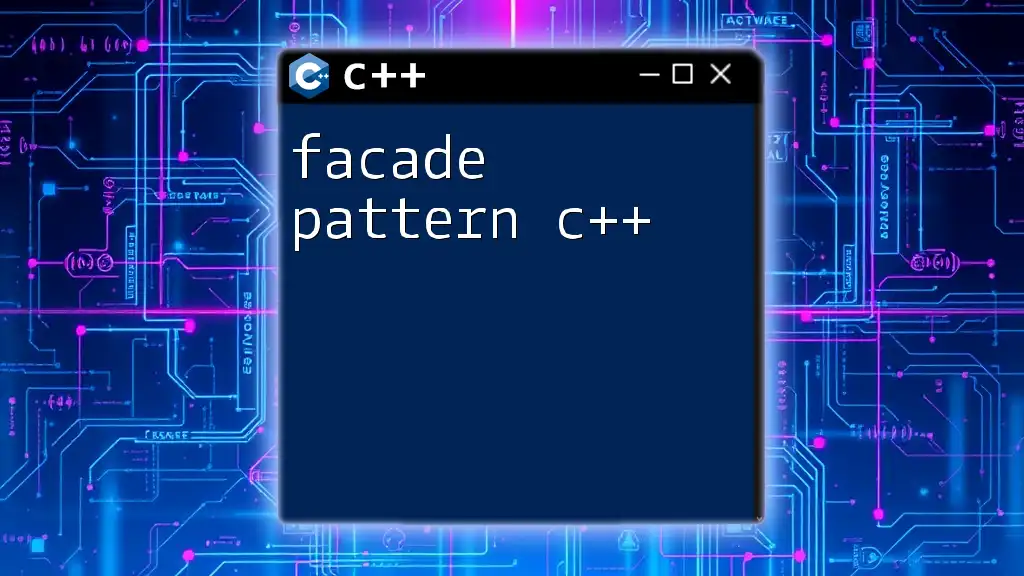
Benefits of the Observer Pattern in C++
Loose Coupling
One of the primary advantages of the observer pattern is the loose coupling it provides. Subjects and observers can evolve independently; the subject doesn’t need to know the details of its observers—it simply needs to know that they implement the observer interface. This separation simplifies testing and enhances flexibility.
Flexibility and Scalability
The observer pattern is especially beneficial in highly dynamic scenarios. You can add or remove observers at runtime without significant changes to your core logic. This is crucial for applications that may need to adapt or scale, like a notifications system that may add new listeners as needed.
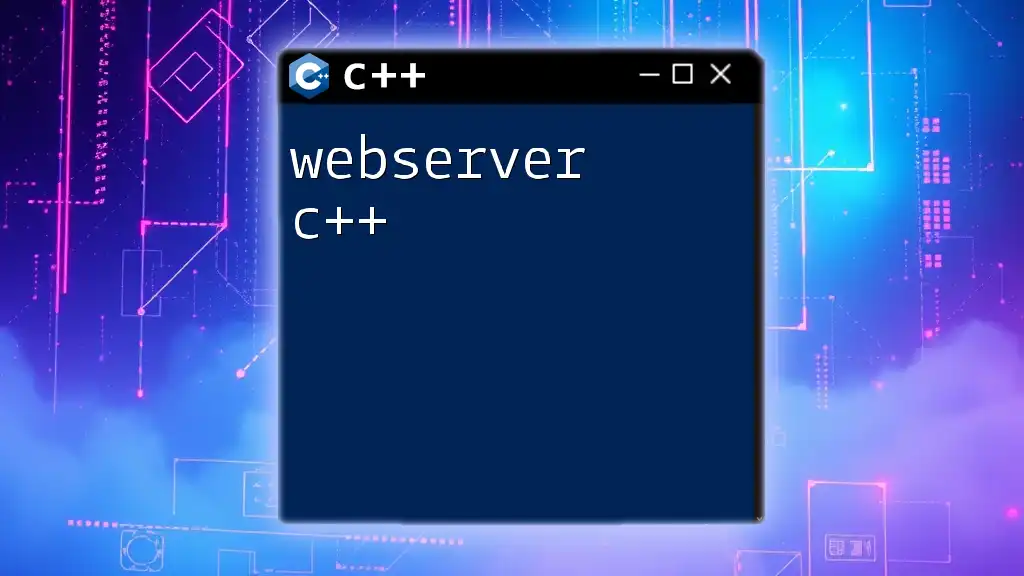
Challenges and Considerations
Memory Management
Memory management is a significant concern when using the observer pattern. If observers aren’t properly detached from the subject, you may encounter dangling pointers or memory leaks. To mitigate these risks, always ensure observers are detached when they no longer need updates.
Performance Overheads
When there are many observers, the overhead of notifying all observers can impact performance. It’s essential to balance the number of observers and the frequency of notifications to maintain system efficiency.
Event Ordering
In scenarios where multiple observers depend on the same subject, keeping track of the order of notifications may become problematic. It’s vital to consider this in designs where event ordering is critical.
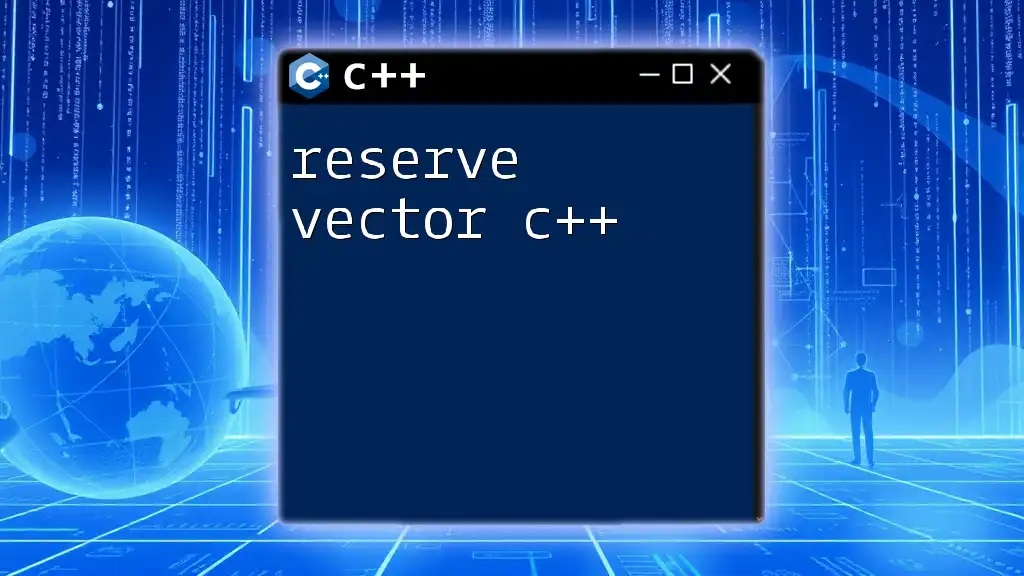
Best Practices for Using the Observer Pattern
Naming Conventions
Using clear and consistent naming conventions is critical for maintaining the readability of your code. Class names like `ConcreteSubject` and `ConcreteObserver` clarify intentions and responsibilities.
When to Use the Observer Pattern
Evaluate your design against common scenarios to determine if the observer pattern fits your needs. If your application requires dynamic subscriptions and decoupled components, this pattern is likely to be a suitable choice.
Alternatives to the Observer Pattern
While the observer pattern is remarkably effective, alternatives exist, such as the Mediator pattern. In situations where you need to centralize communication between different modules or components, the mediator may be more appropriate.
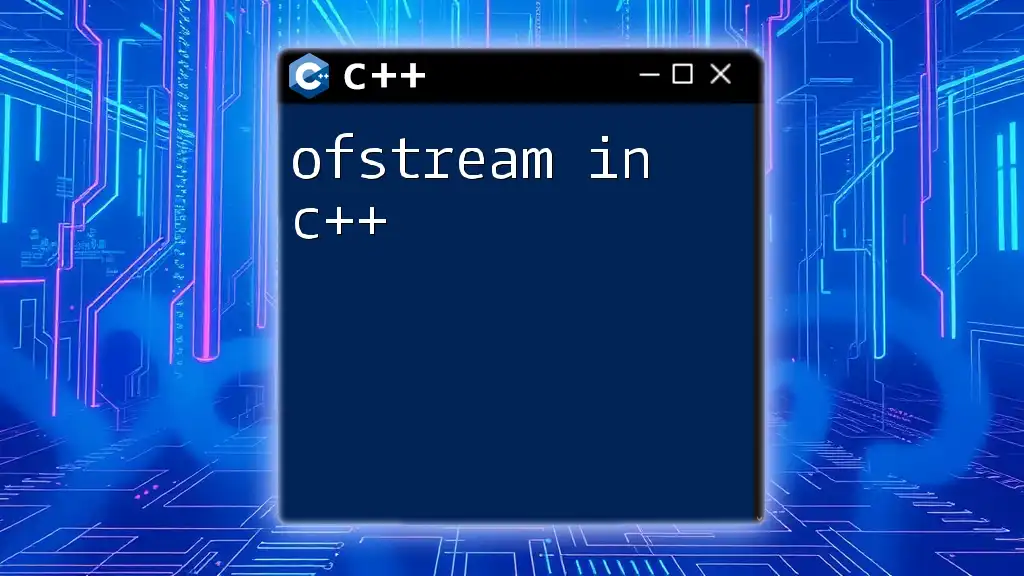
Conclusion
In summary, the observer pattern in C++ reinforces a flexible, scalable, and maintainable architecture by promoting loose coupling. By understanding its structure, benefits, and best practices, developers can leverage this powerful design pattern to enhance their applications. Through further exploration and experimentation, you can effectively implement the observer pattern to create responsive and robust systems.