The Builder Pattern in C++ is a design pattern that separates the construction of a complex object from its representation, allowing for the same construction process to create various representations.
#include <iostream>
#include <string>
class Car {
public:
std::string engine;
std::string color;
void info() {
std::cout << "Car with engine: " << engine << " and color: " << color << std::endl;
}
};
class CarBuilder {
private:
Car car;
public:
CarBuilder& setEngine(const std::string& engine) {
car.engine = engine;
return *this;
}
CarBuilder& setColor(const std::string& color) {
car.color = color;
return *this;
}
Car build() {
return car;
}
};
int main() {
CarBuilder builder;
Car myCar = builder.setEngine("V8").setColor("Red").build();
myCar.info();
return 0;
}
What is the Builder Pattern?
The Builder Pattern is a creational design pattern that allows developers to construct complex objects step by step. Unlike traditional constructors, which can become cumbersome and confusing as they require multiple parameters, the builder pattern provides a more flexible approach. By separating the construction of a complex object from its representation, it allows for different representations to be created using the same construction process.
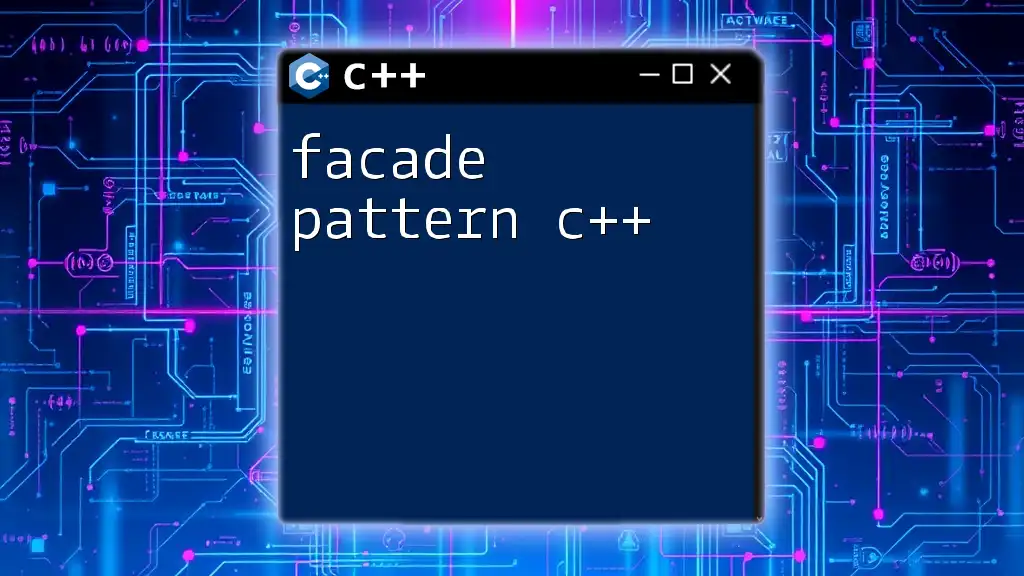
When to Use the Builder Pattern
The builder pattern is particularly useful in various scenarios, including but not limited to:
- When the object being created has many parameters, some of which may be optional.
- When constructing an object involves multiple steps and requires a clear construction process.
- When different representations of a product may be created using a common construction process.
It’s essential to understand that while the builder pattern is powerful, it should be compared against other design patterns, such as the Factory Pattern, to ensure the right choice is made for your specific use case.
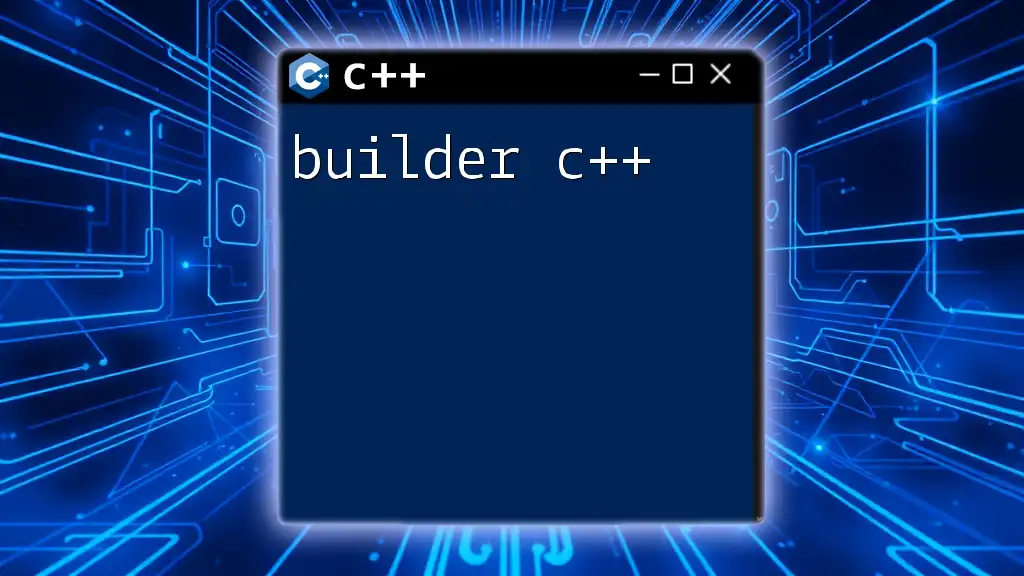
Understanding the Need for the Builder Pattern
Complex Object Construction
In many applications, objects can become complex due to numerous attributes and states. For example, consider a `House` object that may have attributes like its foundation, number of floors, and whether it has a garage. Traditional constructors can lead to constructor overloading or even a long parameter list, which decreases code readability and maintainability.
Benefits of Using the Builder Pattern
Utilizing the builder pattern offers numerous advantages:
- Improved Readability and Maintainability: Each step of the construction process is clearly defined, making it easier to comprehend the process at a glance.
- Flexibility: The builder can be modified to create various representations without altering the core construction logic, enhancing the design's adaptability.
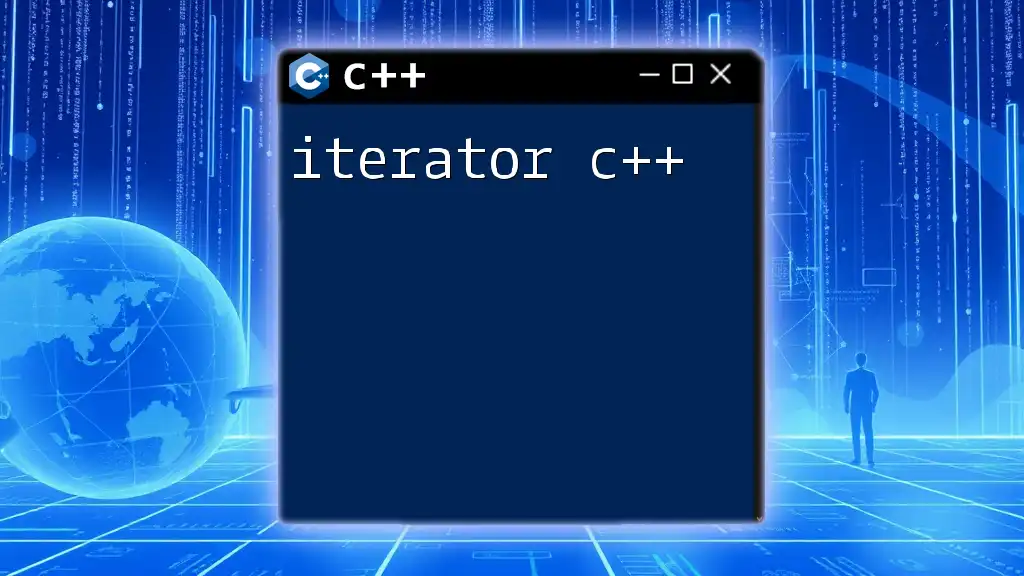
Components of the Builder Pattern in C++
The Product Class
The product class embodies the complex object being built. In our example, we will define a simple `House` class.
class House {
public:
std::string foundation;
int floors;
bool hasGarage;
void show() {
std::cout << "House with " << floors
<< " floors, foundation: " << foundation
<< ", garage: " << (hasGarage ? "yes" : "no") << std::endl;
}
};
The Builder Interface
The builder interface establishes a contract for creating different parts of the product. By defining an interface, you ensure that any concrete builder must implement the defined methods.
class HouseBuilder {
public:
virtual void buildFoundation() = 0;
virtual void buildFloors() = 0;
virtual void buildGarage() = 0;
virtual House* getHouse() = 0;
};
Concrete Builders
Concrete builders implement the builder interface. They contain methods defining how each part of the product is built.
class ConcreteHouseBuilder : public HouseBuilder {
private:
House* house;
public:
ConcreteHouseBuilder() { house = new House(); }
void buildFoundation() override { house->foundation = "Concrete"; }
void buildFloors() override { house->floors = 2; }
void buildGarage() override { house->hasGarage = true; }
House* getHouse() override { return house; }
};
Director Class
The director class orchestrates the building process. It utilizes the builder to construct the product, ensuring a controlled and coherent building sequence.
class ConstructionDirector {
public:
void construct(HouseBuilder* builder) {
builder->buildFoundation();
builder->buildFloors();
builder->buildGarage();
}
};
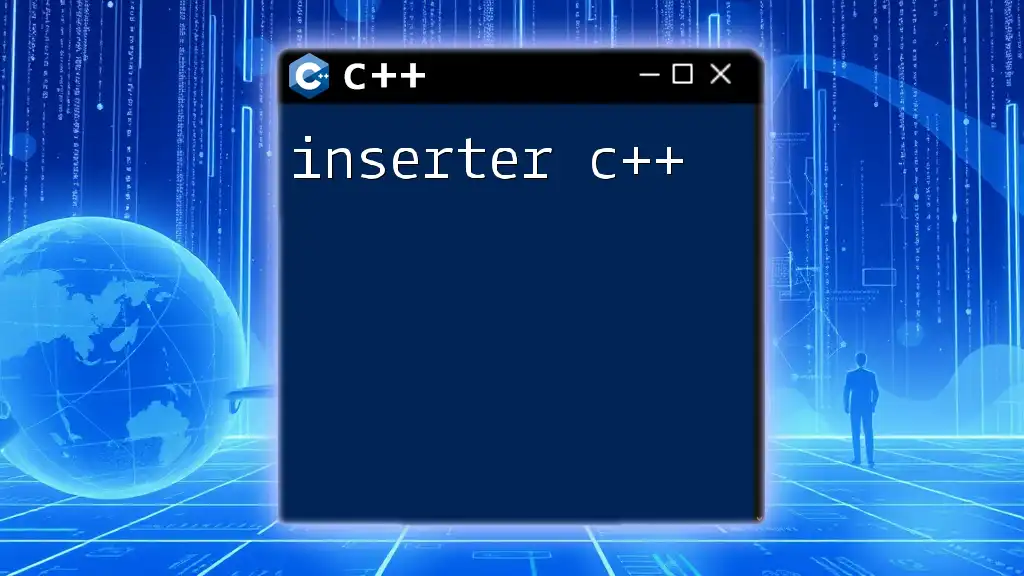
Implementing the Builder Pattern in C++
Step-by-Step Implementation Guide
To implement the builder pattern in C++, it is crucial to set up the building blocks correctly. Each component should work harmoniously to construct the product, as illustrated in the previous snippets.
Code Snippet: Using the Builder
Here is an example of utilizing the builder pattern to create a `House`:
int main() {
ConcreteHouseBuilder* builder = new ConcreteHouseBuilder();
ConstructionDirector* director = new ConstructionDirector();
director->construct(builder);
House* house = builder->getHouse();
house->show();
delete builder;
delete director;
delete house;
return 0;
}
In this code, a `ConcreteHouseBuilder` builds a `House` and the `ConstructionDirector` orchestrates the process. The `House` object is then displayed, clearly showcasing the builder pattern in action.
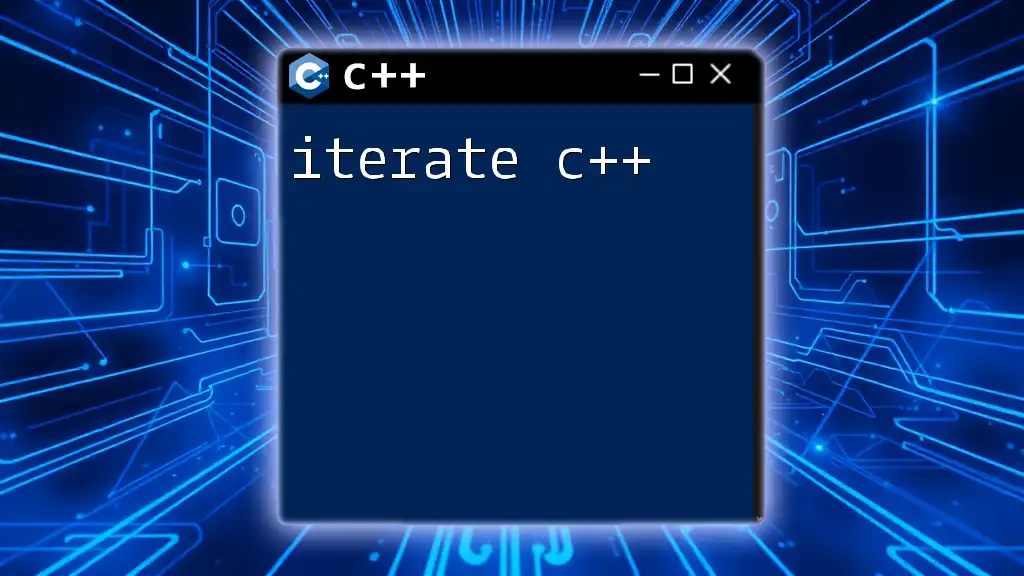
Best Practices for Implementing the Builder Pattern in C++
Encapsulation and Abstraction
When implementing the builder pattern, prioritize encapsulation and abstraction. Ensure that the internal mechanisms of the builders and the product remain hidden from the client code. This promotes a cleaner interface and minimizes the risk of errors.
Fluent Interface Design
A fluent interface allows method chaining, improving readability. You can design your builder to return a reference to itself to enable this feature, making your construction code more expressive:
class FluentHouseBuilder : public HouseBuilder {
public:
FluentHouseBuilder* buildFoundation(const std::string& foundation) {
this->house->foundation = foundation;
return this;
}
FluentHouseBuilder* buildFloors(int numberOfFloors) {
this->house->floors = numberOfFloors;
return this;
}
FluentHouseBuilder* buildGarage(bool hasGarage) {
this->house->hasGarage = hasGarage;
return this;
}
House* getHouse() override {
return this->house;
}
};
Resolving Common Pitfalls
Some common mistakes include:
- Overcomplicating the Builder: Avoid making the builder too complex. Keep it straightforward to ensure clarity.
- Ignoring Optional Parameters: Design the builder to handle optional parameters efficiently, possibly by using method overloading or default values.
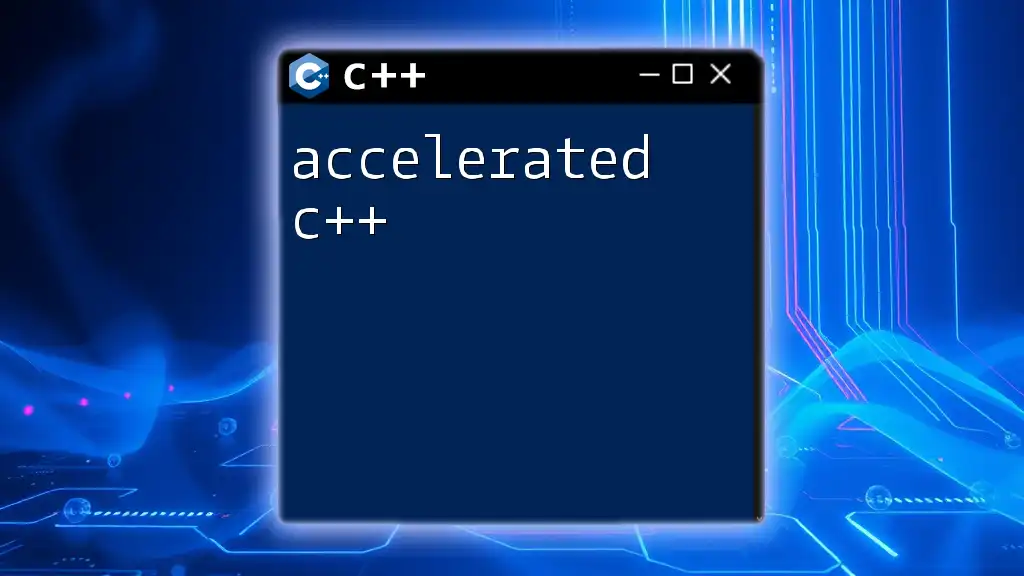
Conclusion
The builder pattern in C++ is a potent design pattern that simplifies the construction of complex objects while enhancing the code's readability and maintainability. By separating the construction logic from the object itself, developers can produce various representations without compromising clarity.
The build process becomes straightforward, allowing for greater flexibility in design. By adhering to the best practices highlighted in this article, you can effectively implement the builder pattern in your projects, resulting in cleaner and more maintainable code.
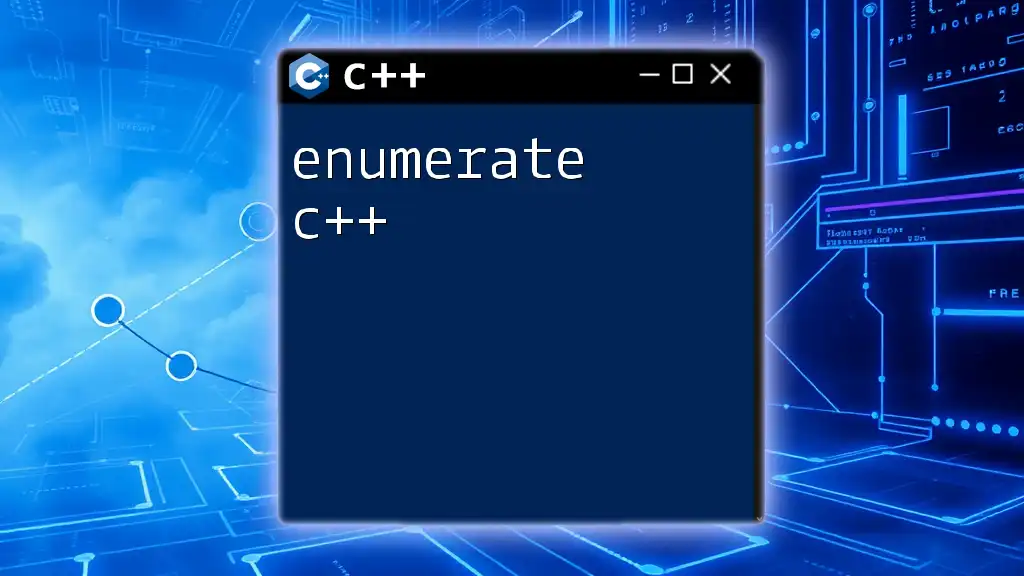
FAQs about Builder Pattern in C++
What is the difference between Builder and Factory patterns?
While both patterns deal with object creation, the Builder Pattern focuses on constructing a complex object piece by piece, whereas the Factory Pattern is concerned with creating instances of objects based on their type.
Can the Builder Pattern be used with other languages?
Absolutely! The builder pattern is not language-specific and can be effectively employed in various programming languages, including Java, Python, and C#.
How does the Builder Pattern help in multi-threading?
The builder pattern promotes immutability in object construction, making it easier to share and construct objects across multiple threads without synchronization issues. Each builder can create a complete instance independently, allowing for safe parallel processing.
In exploring these concepts, you can integrate the builder pattern in your designs effectively, thereby enhancing the overall architecture of your applications.