The Facade Pattern in C++ simplifies complex subsystems by providing a unified interface, allowing users to interact with a simplified view of the underlying functionality.
#include <iostream>
// Subsystem classes
class SubsystemA {
public:
void operationA() {
std::cout << "Subsystem A, Operation A\n";
}
};
class SubsystemB {
public:
void operationB() {
std::cout << "Subsystem B, Operation B\n";
}
};
// Facade
class Facade {
private:
SubsystemA* subsystemA;
SubsystemB* subsystemB;
public:
Facade() {
subsystemA = new SubsystemA();
subsystemB = new SubsystemB();
}
~Facade() {
delete subsystemA;
delete subsystemB;
}
void doOperation() {
subsystemA->operationA();
subsystemB->operationB();
}
};
int main() {
Facade facade;
facade.doOperation(); // Simplified interface
return 0;
}
Understanding the Basics
What is the Facade Pattern?
The Facade Pattern is a structural design pattern that provides a simplified interface to a complex system of classes, libraries, or frameworks. Its primary function is to offer a unified interface that allows easier interaction with the underlying subsystems, thereby reducing the complexity faced by the user. By abstracting the intricate workings of the system, the facade enables clients to communicate with multiple components without needing to understand the internal logic and dependencies.
Core Principles of the Facade Pattern
- Encapsulation of Complexity: The facade hides the intricacies of the subsystem and presents a straightforward interface, which helps avoid overwhelming clients with unnecessary details.
- Simplification of Interfaces: By exposing only essential functionalities, the facade pattern can streamline interactions and help users focus on what they need to accomplish.
- Improvement of Code Readability and Usability: A clean and simple interface promotes better code readability, fostering an easier understanding of the system's functionality for developers.
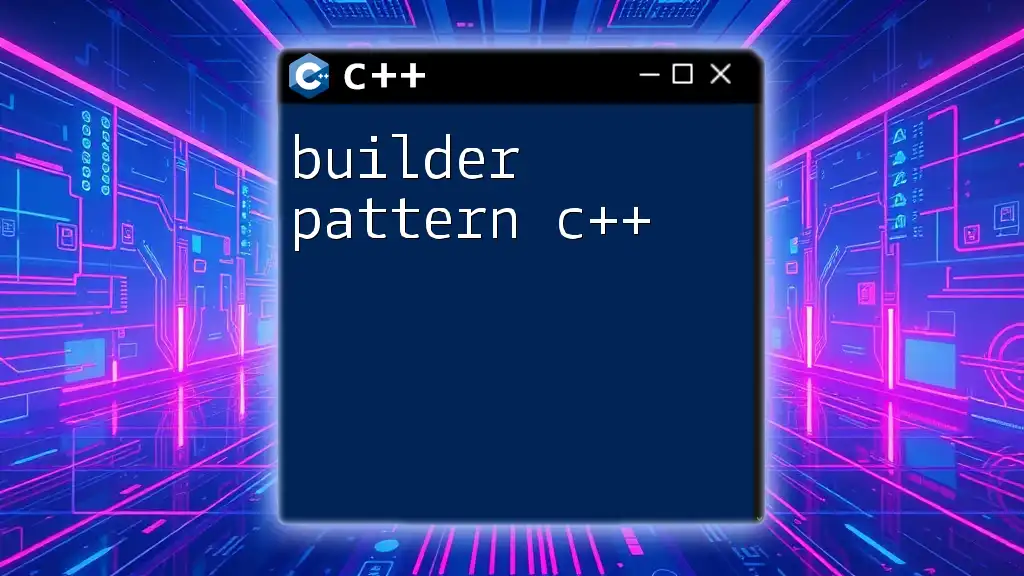
Real-world Scenarios for Using the Facade Pattern
The Facade Pattern is particularly useful in various situations, such as:
- Library Integration: When working with complex libraries, the facade can help clients access functionalities without needing to delve into intricate APIs or documentation.
- System Configuration: In applications where multiple subsystems need to be set up, a facade can simplify the configuration process, allowing clients to initialize the system with minimal input.
- Complex Applications: For software systems with numerous objects or classes, a facade can act as a single point of interaction, managing and controlling the various components seamlessly.
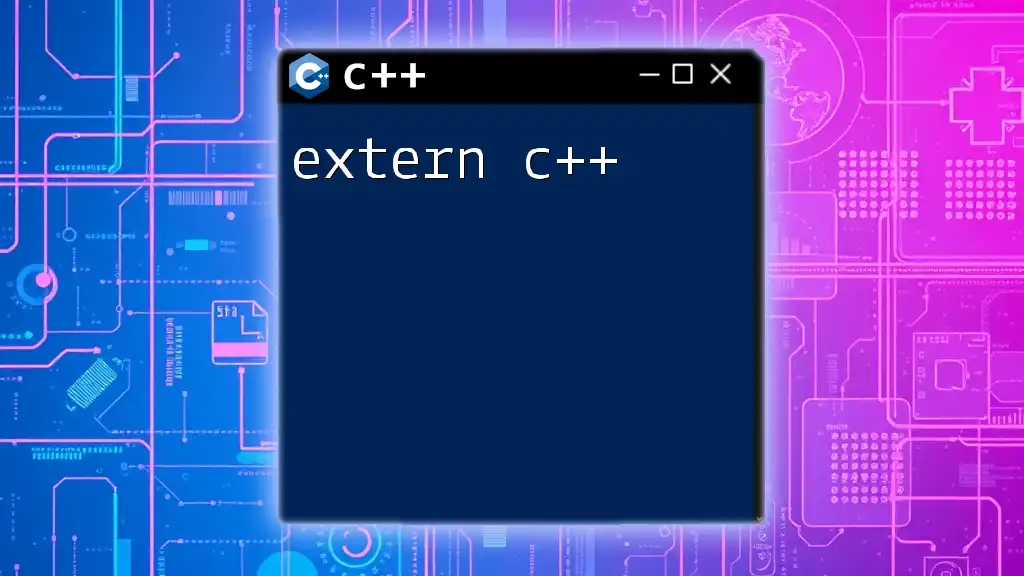
Implementing the Facade Pattern in C++
Key Components of the Facade Pattern
The Facade Class
The facade class is the heart of the facade pattern. It is responsible for coordinating and managing the interactions between different subsystem classes. Its primary responsibility is to provide a simplified interface that encapsulates complex interactions.
Subsystem Classes
Subsystem classes are the underlying components that perform the actual work. These classes contain detailed functionalities that the facade exposes through its interface.
Step-by-step Implementation
Defining the Subsystem Classes
Let's start by creating a couple of subsystem classes. In this example, we will define two classes, `SubsystemA` and `SubsystemB`, each containing a distinct method that performs specific tasks.
class SubsystemA {
public:
void operationA() {
// Implementation for operation A
std::cout << "SubsystemA: Performing operation A" << std::endl;
}
};
class SubsystemB {
public:
void operationB() {
// Implementation for operation B
std::cout << "SubsystemB: Performing operation B" << std::endl;
}
};
In this code snippet, `SubsystemA` and `SubsystemB` are basic classes that implement specific operations that might be too complex to interact with directly.
Creating the Facade Class
Now that our subsystem classes are defined, we can create the facade class, which will provide a simplified interface for these subsystems.
class Facade {
private:
SubsystemA* subsystemA;
SubsystemB* subsystemB;
public:
Facade() {
subsystemA = new SubsystemA();
subsystemB = new SubsystemB();
}
void simplifiedOperation() {
subsystemA->operationA();
subsystemB->operationB();
}
~Facade() {
delete subsystemA;
delete subsystemB;
}
};
Here, the `Facade` class initializes instances of `SubsystemA` and `SubsystemB` and offers the `simplifiedOperation` method, which internally calls the operations from both subsystems. This hides the complexity from the client.
Using the Facade in Client Code
Finally, let’s see how a client can use our facade.
int main() {
Facade facade;
facade.simplifiedOperation();
return 0;
}
In this example, the client uses the facade to perform operations on the subsystems without needing to concern itself with the details of how these operations are implemented.
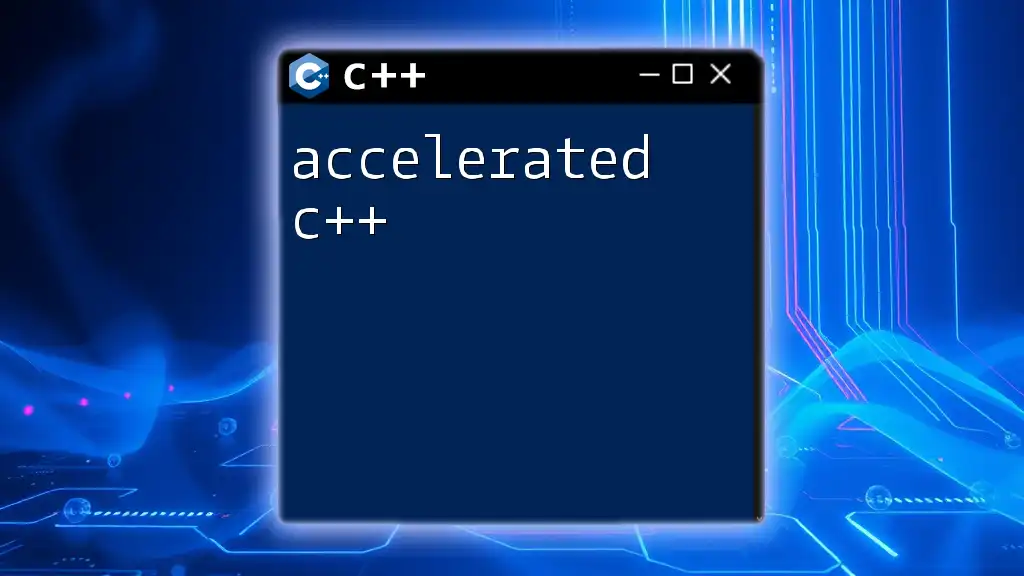
Advantages of Using the Facade Pattern
The Facade Pattern brings with it several significant benefits:
Simplifies Client Interaction
By reducing the number of dependencies and simplifying the interface, clients can interact with complex subsystems more intuitively. This encourages cleaner and more maintainable code since clients only need to know about the facade's methods rather than the details of each subsystem.
Promotes Loose Coupling
Loose coupling is a critical design principle. The facade separates the client from the subsystems, meaning changes in the subsystems do not affect the client. This flexibility allows for easier updates and maintenance of individual components.
Facilitates Testing
Testing can be made simpler due to the isolation provided by the facade. Units can be tested independently by focusing on the facade’s interface rather than the entire complexity of the subsystems.
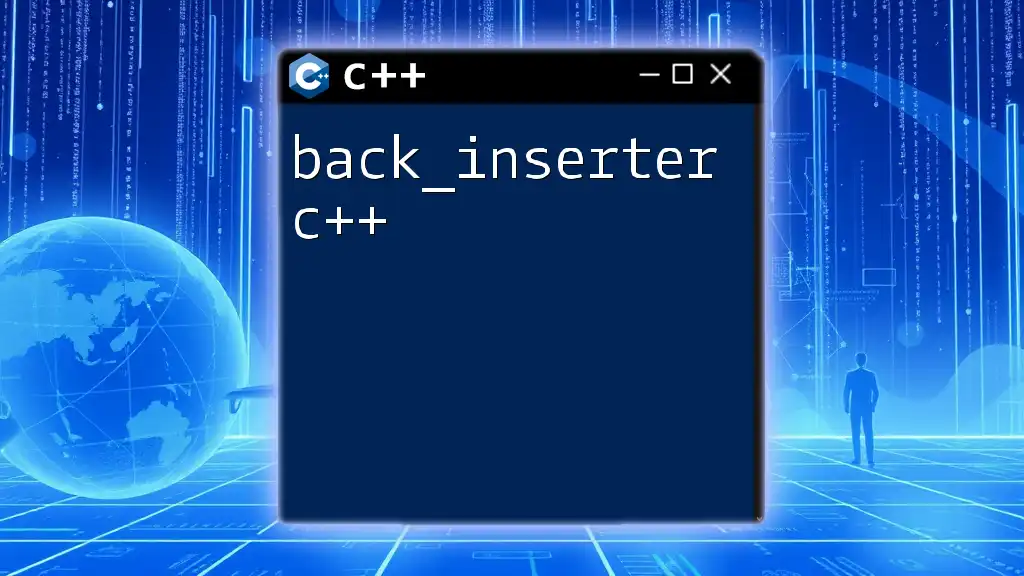
Potential Drawbacks of the Facade Pattern
While the facade pattern has many advantages, it’s essential to be aware of its potential drawbacks:
Over-simplification
If a facade is designed without careful consideration, it may over-simplify the underlying processes, masking essential functionality. This can lead to a situation where clients have limited control or flexibility.
Maintenance Challenges
A facade can become a "God Object" if it contains too much functionality, making it difficult to maintain. It can also lead to a scenario where all changes must be managed through the facade, countering the benefits of loose coupling.
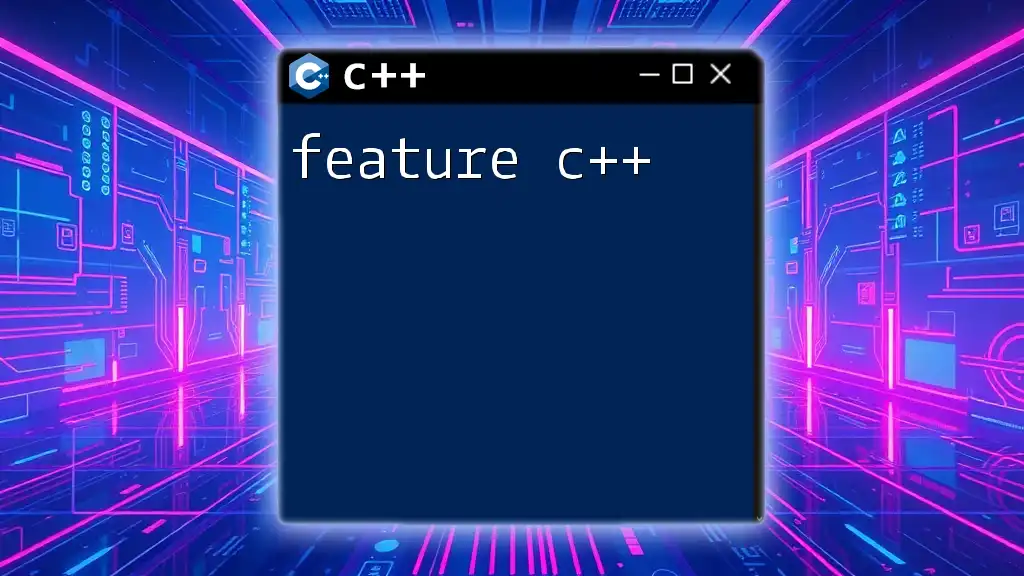
Conclusion
The facade pattern in C++ is an effective design pattern that simplifies complex systems through encapsulation and abstraction. By providing a unified interface, it allows developers to create clean, maintainable, and readable code. As you're venturing into software design, consider the benefits of the facade pattern in your projects for better architecture and user experience. Embracing design patterns like the Facade can enhance the quality of your applications, making them easier to develop and maintain.
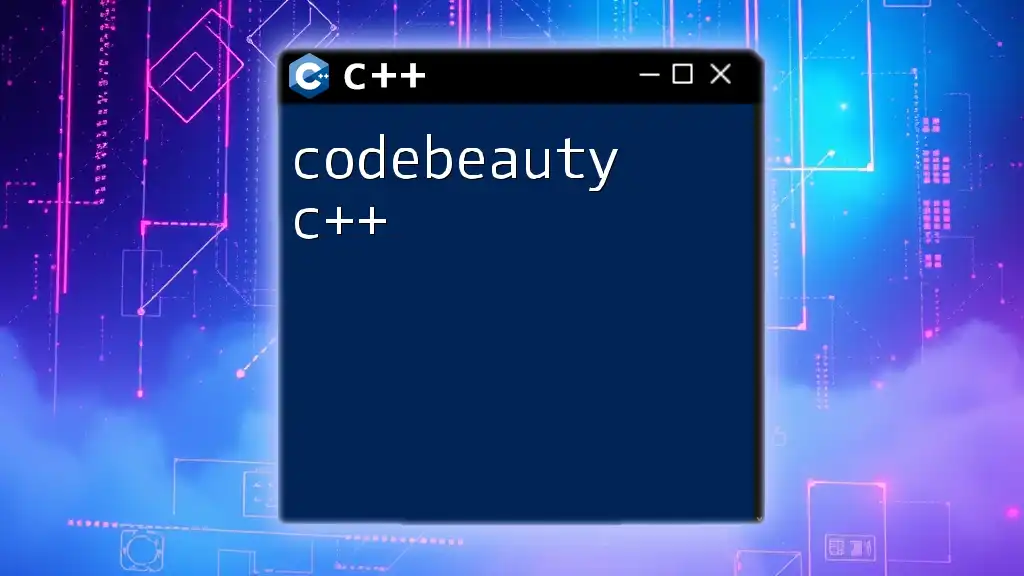
Additional Resources
To deepen your understanding, consider exploring books or online courses dedicated to design patterns and C++. Engaging with online communities can also provide valuable insights and support as you implement these concepts in your coding journey.
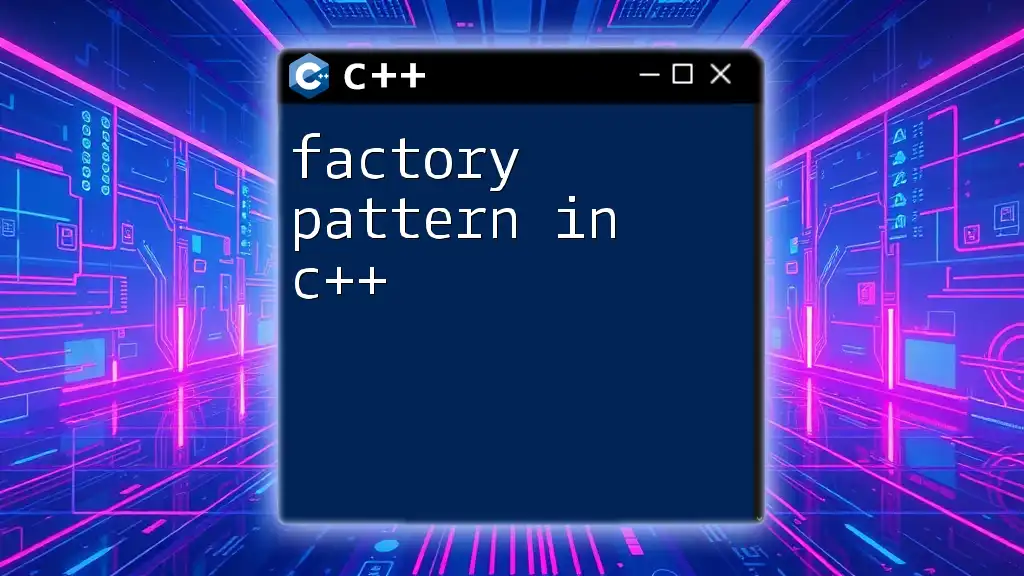
Call to Action
Have you implemented the facade pattern in your projects? Share your experiences and insights in the comments below or reach out for any questions or personalized learning assistance!