The Singleton Pattern in C++ ensures that a class has only one instance and provides a global point of access to that instance.
Here’s a simple implementation example:
#include <iostream>
class Singleton {
private:
static Singleton* instance;
// Private constructor to prevent instantiation
Singleton() {}
public:
// Public method to provide access to the instance
static Singleton* getInstance() {
if (instance == nullptr) {
instance = new Singleton();
}
return instance;
}
void displayMessage() {
std::cout << "Hello, I am a Singleton instance!" << std::endl;
}
};
// Initialize the static member
Singleton* Singleton::instance = nullptr;
int main() {
Singleton* singleton = Singleton::getInstance();
singleton->displayMessage();
return 0;
}
Understanding the Singleton Pattern in C++
Characteristics of the Singleton Pattern in C++
The singleton pattern in C++ is defined by several important characteristics that distinguish it from other design patterns:
-
Unique Instance: The singleton class ensures that only one instance of the class exists throughout the application's lifecycle. All requests for the instance will return the same object.
-
Controlled Access to the Instance: Instead of exposing the instance directly, the singleton pattern provides a public static method for clients to access the instance. This encapsulation controls instance creation and provides a global access point.
-
Lazy vs. Eager Instantiation: Singleton instances can be created lazily (upon first request) or eagerly (at program start). Defining which approach to use depends on specific application needs.
When to Use the Singleton Pattern in C++
Applying the singleton pattern in C++ can be highly advantageous in scenarios requiring controlled access to shared resources, such as:
-
Configuration Management: When you need a centralized configuration object that is read-only throughout your application.
-
Logging System: A logging class that ensures all parts of the application write to the same output stream.
-
Connection Handling: Database connection pools where maintaining a single connection instance is crucial for resource management.
Implementing the Singleton Pattern in C++
Basic Structure of a Singleton Class in C++
To create a singleton class in C++, consider the following crucial components:
- Private Constructor: Prevents clients from instantiating the class directly.
- Static Instance Variable: Holds the singleton instance.
- Public Static Method: Provides access to the instance.
Here’s a basic structure of the singleton pattern implemented in C++:
class Singleton {
private:
static Singleton* instance; // Pointer to the single instance
Singleton() {} // Private constructor ensures no direct instantiation
public:
static Singleton* getInstance() {
if (!instance) {
instance = new Singleton(); // Lazy instantiation
}
return instance;
}
};
// Definition of the static instance outside the class
Singleton* Singleton::instance = nullptr;
In this code, the constructor `Singleton()` is private, ensuring that no external code can create an instance of `Singleton` directly. The static method `getInstance()` checks if an instance exists; if not, it creates one.
C++ Singleton Example: A Thread-Safe Singleton
For multithreaded applications, it's essential to implement the singleton pattern with care to avoid creating multiple instances in concurrent environments. A typical solution involves using mutexes to synchronize access to the singleton instance.
Here’s how you can implement a thread-safe singleton:
#include <mutex>
class ThreadSafeSingleton {
private:
static ThreadSafeSingleton* instance;
static std::mutex mutex_; // Mutex to ensure thread safety
ThreadSafeSingleton() {} // Private constructor
public:
static ThreadSafeSingleton* getInstance() {
std::lock_guard<std::mutex> lock(mutex_); // Lock for thread safety
if (!instance) {
instance = new ThreadSafeSingleton(); // Lazy instantiation
}
return instance;
}
};
// Definitions of static variables
ThreadSafeSingleton* ThreadSafeSingleton::instance = nullptr;
std::mutex ThreadSafeSingleton::mutex_;
In this implementation, the `std::lock_guard` is used to manage the mutex automatically, ensuring that the lock is released when the method exits.
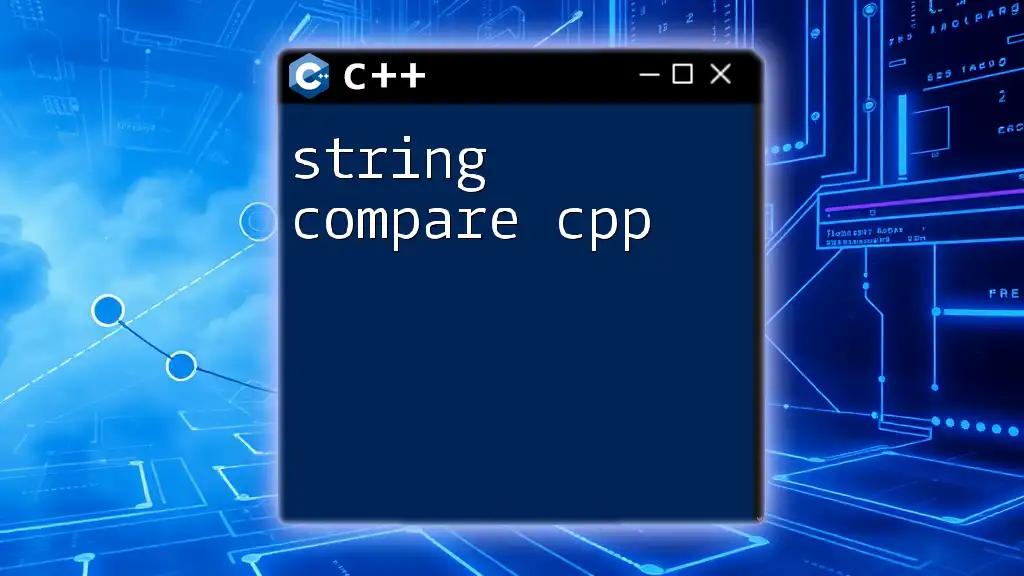
Singleton Design Pattern in C++: Benefits and Drawbacks
Advantages of Using the Singleton Design Pattern
Implementing the singleton pattern in C++ brings several benefits:
-
Controlled Access: It restricts instantiation of a class to a single instance, enabling controlled access throughout the application.
-
Resource Management: Reducing resource usage is often essential, especially in memory-constrained environments. A singleton can prevent unnecessary overhead.
-
Global Point of Access: The singleton pattern guarantees a single global point of access, simplifying the interaction of components across applications.
Disadvantages and Limitations of Singleton Pattern in C++
Despite its advantages, the singleton pattern is not without downsides:
-
Global State: Singletons create global state, which can complicate unit testing. Dependencies on global instances can lead to unpredictable behavior if state is modified unexpectedly.
-
Subclassing Challenges: The singleton pattern typically disallows subclassing due to its strict control over instantiation.
-
Memory Management Concerns: If you use dynamic allocation (e.g., `new`), you must manage memory carefully to avoid leaks. Alternatively, consider using smart pointers.
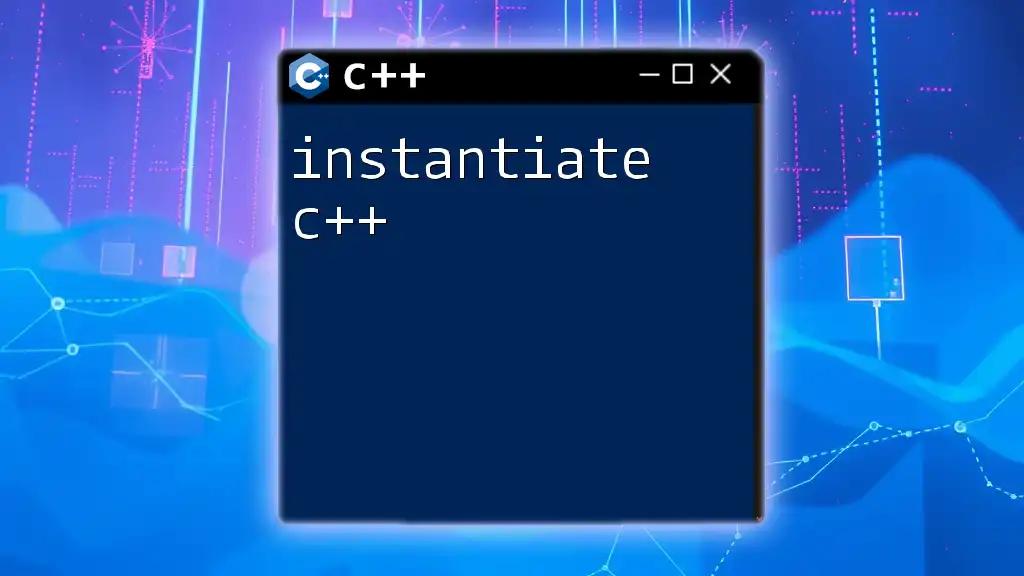
Best Practices for C++ Singleton Implementation
Safe Instantiation
To mitigate memory leaks when creating a singleton, it’s wise to leverage smart pointers like `std::shared_ptr`:
#include <memory>
class SafeSingleton {
private:
static std::shared_ptr<SafeSingleton> instance; // Shared pointer to instance
SafeSingleton() {} // Private constructor
public:
static std::shared_ptr<SafeSingleton> getInstance() {
if (!instance) {
instance = std::shared_ptr<SafeSingleton>(new SafeSingleton());
}
return instance;
}
};
// Definition of the static variable
std::shared_ptr<SafeSingleton> SafeSingleton::instance = nullptr;
Avoiding Common Pitfalls
When implementing the singleton pattern:
-
Consider Thread Safety: Ensure that you properly handle multi-threaded access to uphold integrity.
-
Design for Testability: Incorporate dependency injection where possible to facilitate easier testing of components that depend on the singleton.
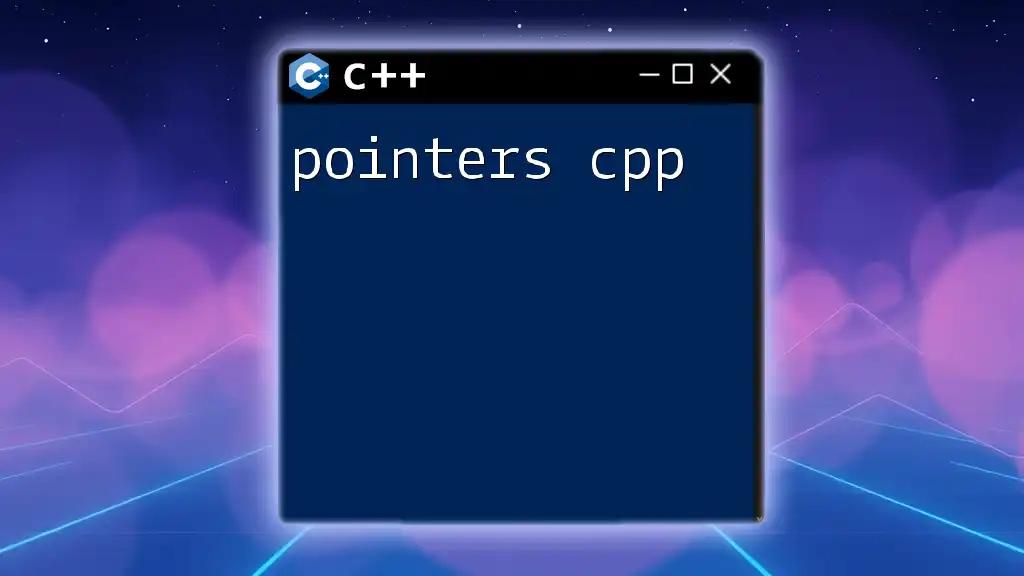
C++ Singleton Class in Practice
Example: Logger Class Using the Singleton Pattern
A common real-world use case for the singleton pattern in CPP is a logger class. Below is a simple implementation that ensures all logging is managed by a single instance.
#include <iostream>
#include <fstream>
class Logger {
private:
static Logger* instance; // Pointer to the single instance
std::ofstream logFile; // File stream for logging
Logger() {
logFile.open("log.txt", std::ios::app); // Open log file in append mode
}
public:
~Logger() {
logFile.close(); // Close the log file
}
static Logger* getInstance() {
if (!instance) {
instance = new Logger(); // Lazy instantiation
}
return instance;
}
void log(const std::string& message) {
logFile << message << std::endl; // Log message to file
}
};
// Definition of the static variable
Logger* Logger::instance = nullptr;
This `Logger` class ensures that all logging operations utilize the same file, thus maintaining a single point of logging across your application. Here’s a basic usage example:
int main() {
Logger::getInstance()->log("Application started.");
Logger::getInstance()->log("Performing some operations...");
Logger::getInstance()->log("Application finished.");
return 0;
}

Conclusion
The singleton pattern in C++ offers a robust design solution for ensuring a single, controlled instance of a class exists. By understanding its characteristics, advantages, and potential drawbacks, developers can make informed decisions about when to employ this pattern effectively. The key implementation strategies discussed, along with practical examples, empower you to leverage the singleton pattern within your own C++ applications. Embrace the singleton pattern, but remember to weigh its implications on design and maintainability as you work.