A linked list in C++ is a dynamic data structure that consists of nodes, where each node contains data and a pointer to the next node, allowing for efficient insertion and deletion operations.
#include <iostream>
struct Node {
int data;
Node* next;
};
class LinkedList {
public:
Node* head;
LinkedList() { head = nullptr; }
void insert(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = head;
head = newNode;
}
void display() {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
}
};
int main() {
LinkedList list;
list.insert(10);
list.insert(20);
list.display(); // Output: 20 10
return 0;
}
What is a Linked List?
A linked list is a fundamental data structure that consists of a sequence of elements, each containing a reference (or link) to the next one. Unlike arrays, which have fixed sizes, linked lists can grow and shrink dynamically, making them suitable for scenarios where the number of elements may frequently change.
Benefits of Using Linked Lists
Using a linked list can offer several advantages:
- Dynamic Size: Unlike arrays, linked lists can easily expand or contract in size. This dynamic allocation reduces wasted memory.
- Ease of Insertions and Deletions: Adding or removing elements from a linked list can be done in constant time \(O(1)\) if the position is known, whereas arrays may require reorganization.
- Memory Efficiency: Linked lists can be more memory-efficient for certain applications, as they allocate memory as needed.

Types of Linked Lists
Singly Linked List
A singly linked list consists of nodes where each node contains data and a pointer to the next node in the sequence. This structure makes it easy to implement basic operations like insertion, deletion, and traversal.
Basic Operations
- Insertion at the Beginning: This removes the need to traverse the list.
- Insertion at the End: Requires traversal if the list is not maintained with a tail pointer.
- Traversal: Iterating through the list is straightforward, as each node points to the next node.
Doubly Linked List
A doubly linked list contains nodes with two pointers: one pointing to the next node and the other to the previous node. This bi-directional connection allows for easier backward traversal and can significantly enhance the performance of certain operations.
Advantages
- Easier insertion and deletion at both ends.
- Can traverse the list in both directions.
Circular Linked List
In a circular linked list, the last node points back to the first node, forming a loop. This structure is beneficial for applications requiring continuous iteration over the list without a defined endpoint.

Implementing a Singly Linked List in C++
To implement a linked list in C++, we start by defining a basic structure for the node.
Node Structure
struct Node {
int data;
Node* next;
};
Creating a Singly Linked List
To initialize our linked list, we define a class containing a head pointer.
class LinkedList {
private:
Node* head;
public:
LinkedList() : head(nullptr) {}
};
Inserting Elements
Insertion at the Beginning
To insert an element at the beginning, we create a new node and adjust pointers accordingly.
void insertAtBeginning(int data) {
Node* newNode = new Node();
newNode->data = data;
newNode->next = head;
head = newNode;
}
Insertion at the End
When inserting at the end, we must traverse the list until we reach the last node.
void insertAtEnd(int data) {
Node* newNode = new Node();
newNode->data = data;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
return;
}
Node* last = head;
while (last->next != nullptr) {
last = last->next;
}
last->next = newNode;
}
Deleting Elements
Deleting nodes requires careful management of pointers to ensure no memory leaks occur. For instance, to delete a node by value:
void deleteNode(int key) {
Node* temp = head, *prev = nullptr;
if (temp != nullptr && temp->data == key) {
head = temp->next;
delete temp;
return;
}
while (temp != nullptr && temp->data != key) {
prev = temp;
temp = temp->next;
}
if (temp == nullptr) return;
prev->next = temp->next;
delete temp;
}
Traversal of Linked List
To print all elements of the linked list, we traverse from the head to the last node using:
void printList() {
Node* current = head;
while (current != nullptr) {
std::cout << current->data << " ";
current = current->next;
}
}

Implementing a Doubly Linked List in C++
The structure of a doubly linked list is similar to a singly linked list, but each node has an additional pointer to the previous node.
Node Structure
struct DNode {
int data;
DNode* next;
DNode* prev;
};
Basic Operations
For a doubly linked list, the insertion and deletion methods involve adjustments to both the next and previous pointers.
Insertion
To insert a node after a given node:
void insertAfter(DNode* prev_node, int new_data) {
if (prev_node == nullptr) return;
DNode* newNode = new DNode();
newNode->data = new_data;
newNode->next = prev_node->next;
prev_node->next = newNode;
newNode->prev = prev_node;
if (newNode->next != nullptr) {
newNode->next->prev = newNode;
}
}
Traversal
Traversal in either direction can be achieved with straightforward loops, allowing traversal from head to tail and vice versa.
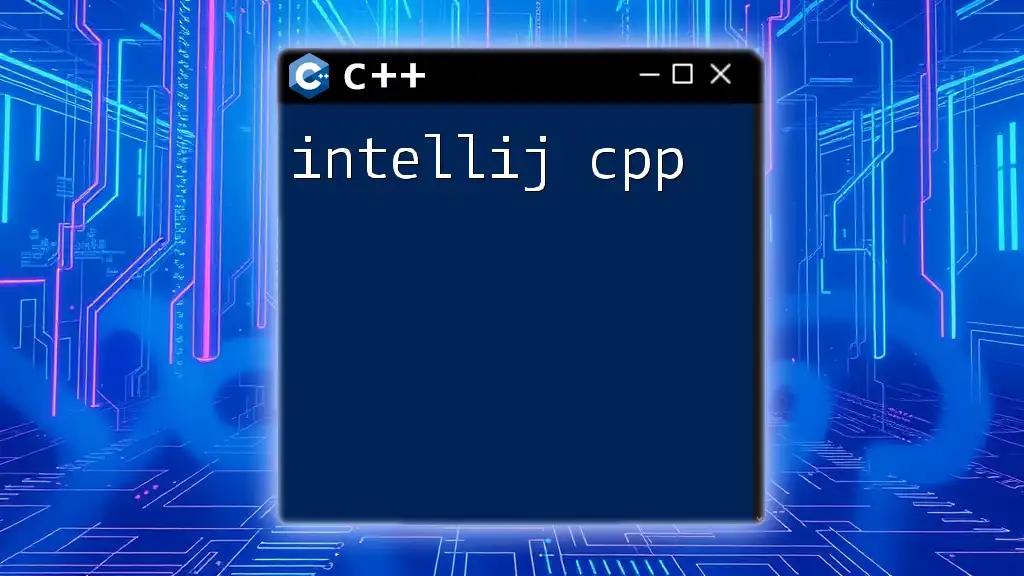
Implementing a Circular Linked List in C++
A circular linked list uses a similar node structure but makes notable adjustments to how we link nodes.
Node Structure
The node retains its structure:
struct Node {
int data;
Node* next;
};
Creating a Circular Linked List
To form a circular structure, the last node's next pointer should point back to the head. Here’s an example of how to insert a new node:
void insertAtEnd(int data) {
Node* newNode = new Node();
newNode->data = data;
if (head == nullptr) {
head = newNode;
newNode->next = head; // Point to itself
} else {
Node* temp = head;
while (temp->next != head) {
temp = temp->next;
}
temp->next = newNode;
newNode->next = head; // Complete the circle
}
}
Basic Operations
With a circular linked list, we can insert and delete elements much like in a singly linked list, with the consideration that we must ensure the last node points back to the head for circular integrity.

Common Problems and Solutions with Linked Lists
Detecting a Loop in a Linked List
Detecting loops is crucial when working with linked lists. The Floyd’s Cycle-Finding Algorithm, commonly known as the "tortoise and hare" method, utilizes two pointers moving at different speeds to determine if a loop exists.
Reversing a Linked List
Reversing a linked list is a common interview question. The process involves adjusting the next pointers of each node. Below is a method to reverse a singly linked list:
void reverse() {
Node* prev = nullptr;
Node* current = head;
Node* next = nullptr;
while (current != nullptr) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
head = prev;
}
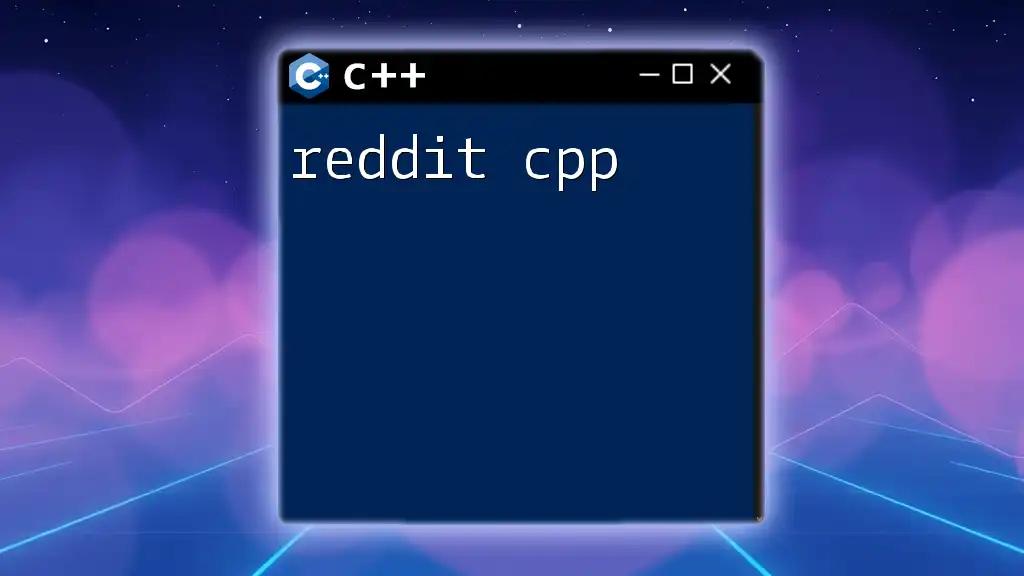
Conclusion
Linked lists are a versatile and essential data structure in C++. Understanding their implementation and benefits—such as dynamic sizing, efficient insertions and deletions, and flexibility—can significantly enhance your programming skills and application design. By engaging with linked lists in C++, you can solve complex problems with more elegance and efficiency. Implementing these structures not only builds a strong foundation in data structures but also prepares you for advanced applications and algorithms.
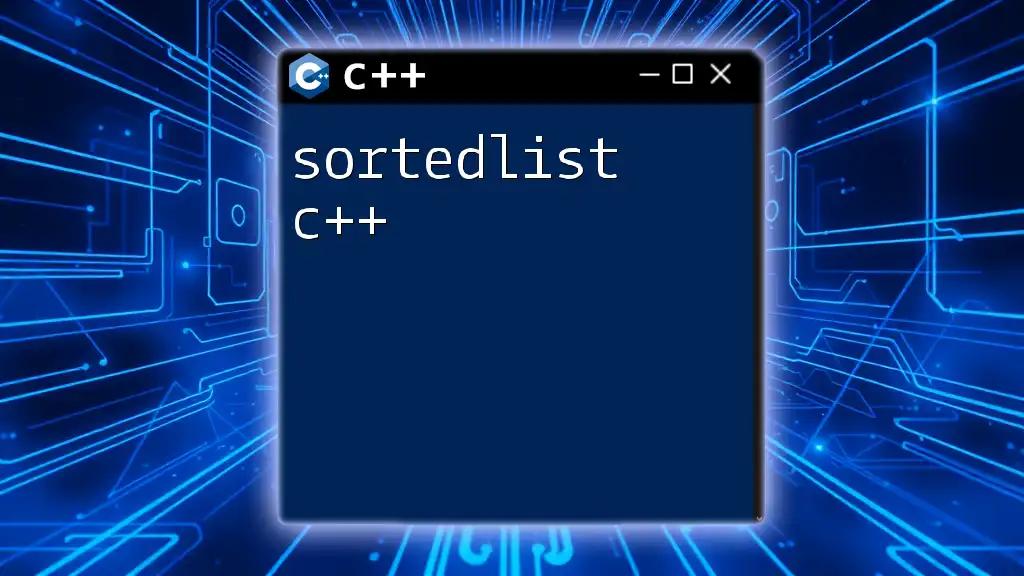
References and Further Reading
For those looking to dive deeper into linked lists and enhance their programming proficiency, consider consulting specialized books on data structures, exploring online coding platforms, or studying the extensive documentation available for the C++ Standard Library. Engaging with a community of learners and experienced developers can also offer practical insights and additional resources.