In C++, `long long` is a data type that is used to store large integer values, typically with a size of at least 64 bits.
Here's a simple code snippet demonstrating how to declare and use a `long long` variable:
#include <iostream>
int main() {
long long largeNumber = 9223372036854775807; // Maximum value for a signed long long
std::cout << "The large number is: " << largeNumber << std::endl;
return 0;
}
What is `long long int` in C++?
Definition and Purpose
In C++, `long long int` is a data type that extends the capacity of the standard integer type. While a regular `int` typically provides a range of values from -2,147,483,648 to 2,147,483,647, the `long long` data type offers a much larger range that can hold values significantly beyond this limit. This is particularly useful in applications that require handling very large numbers, such as scientific computations, financial modeling, and certain algorithmic challenges.
Size and Range
The `long long int` data type is designed to store 64-bit integers. This means you can efficiently perform calculations with extremely large numbers, as its range typically spans from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. The increased size allows programmers to handle more complex and larger datasets without risking overflow errors, which can occur when using smaller data types.
Understanding `long long` in the Context of Data Types
Understanding how `long long` compares to other integral data types in C++ is vital. Unlike `int` or `long`, `long long` is explicitly guaranteed to be at least 64 bits long on all platforms, making it the best choice for consistently handling large numbers across various systems. In situations where an integer overflow could lead to catastrophic errors in results, using `long long` avoids these pitfalls.

How to Use `long long int` in C++
Declaring a `long long int`
The declaration of a `long long int` is straightforward. The syntax looks like this:
long long int myNumber;
You can also initialize the variable in the same line:
long long int bigNumber = 123456789012345;
This assignment illustrates how easily you can work with very large numbers, and the `long long int` type can comfortably contain them without any loss of precision.
Performing Operations with `long long int`
Arithmetic operations on `long long int` follow the same rules as those applied to regular integers. Here’s a simple example:
long long int a = 1;
long long int b = 2;
long long int sum = a + b; // sum = 3
This simplicity allows developers to incorporate large integer calculations into their coding efficiently.
When it comes to more involved computations, consider a scenario where you are working with very large numbers:
long long int population = 8000000000; // Approximate world population
long long int increase = 150000000; // Annual increase
long long int newPopulation = population + increase; // Expected new population in a year
In this example, using `long long` helps avoid overflow, which might arise if a smaller data type like `int` were used, given the massive magnitude of population figures.
Input and Output of `long long int`
Reading and displaying `long long int` values in C++ is as simple as any other data type. Here's how you can read a `long long int` from user input:
long long int userInput;
std::cin >> userInput;
To output this value using `std::cout`, the code appears as follows:
std::cout << "The large number is: " << userInput << std::endl;
This flexibility allows developers to interact with users and handle large integers seamlessly in their applications.
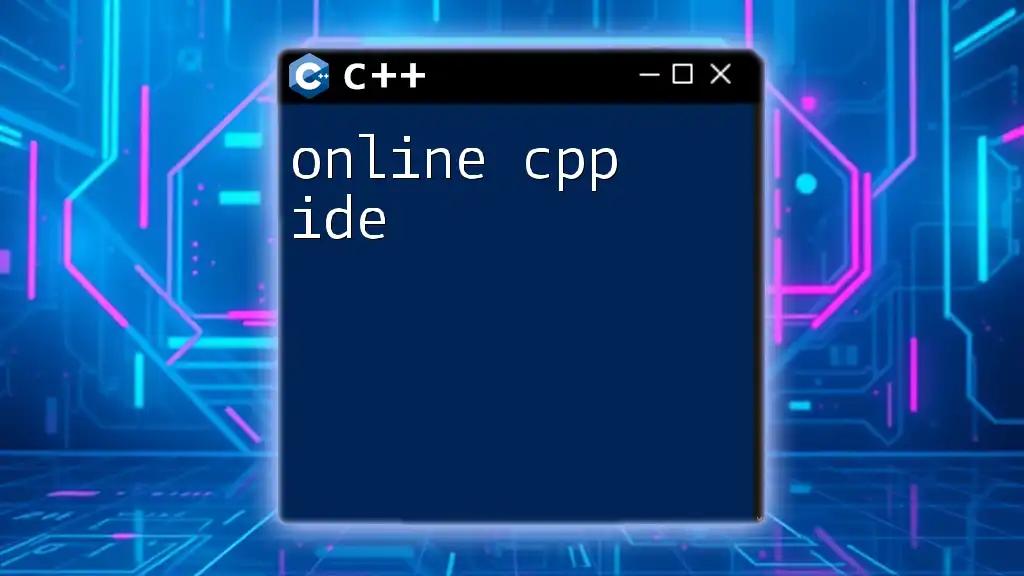
Best Practices When Using `long long`
When to Use `long long int`?
Employ `long long int` whenever you anticipate that the numbers you are dealing with may exceed the boundaries of a standard `int`, especially in fields like finance, scientific research, or competitive programming. Frequent scenarios where overflow is a risk can occur during astronomical calculations, simulation modeling, or when aggregating large sets of data.
Common Mistakes to Avoid
Even seasoned developers can make oversights when working with `long long`. One typical mistake is forgetting to include necessary headers like `<iostream>`, which can lead to compilation errors. Another common pitfall is mixing `long long int` with other data types in expressions, which can inadvertently change the expected type of the resulting expression. For example, mixing `int` and `long long int` might require explicit casting to ensure the correct data type is used for calculations.

Advantages of Using `long long` in Your Projects
Precision in Calculations
One of the significant advantages of using `long long int` is precision in calculations. When dealing with large datasets, the risk of overflow is ever-present. By using `long long int`, you minimize this risk, allowing for accurate results and better reliability in applications.
For example, if you're calculating a factorial of 20, the result is a huge number that could easily exceed what an `int` can handle. Here’s a quick demonstration of this risk:
int fact = 1; // would overflow
for (int i = 1; i <= 20; ++i) {
fact *= i; // potential overflow here
}
With `long long int`:
long long int fact = 1; // safe from overflow
for (int i = 1; i <= 20; ++i) {
fact *= i; // handles large calculations
}
Enhanced Performance
When you examine performance, it might seem that smaller data types are faster. However, modern compilers are optimized such that using `long long` does not incur a noticeable performance penalty, and the benefits of avoiding overflow outweigh potential downsides. Operations on `long long` can be just as efficient, making them a wise choice for computations requiring a larger range without significant performance trade-offs.
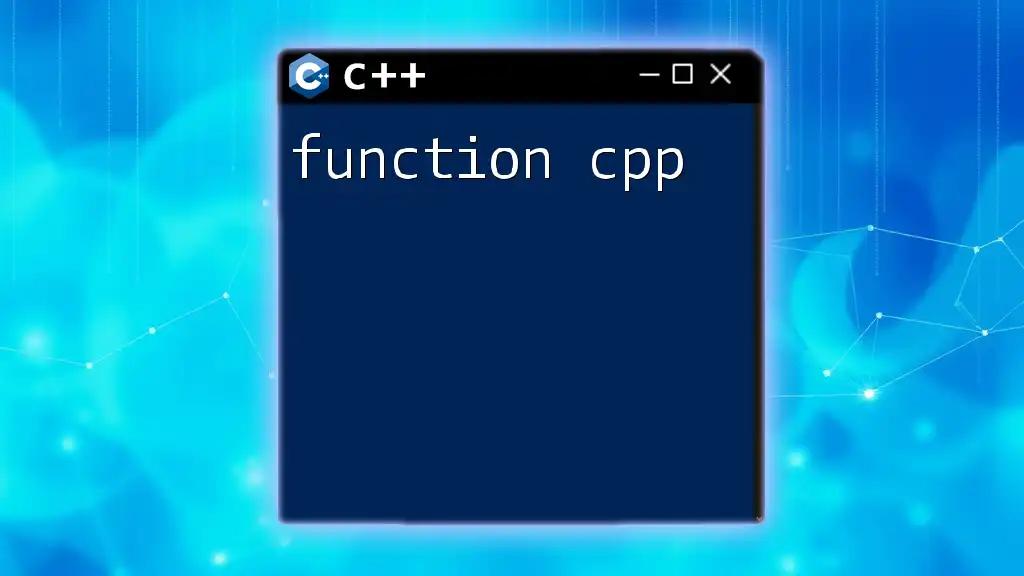
Common Use Cases of `long long int`
Applications in Competitive Programming
In competitive programming, where every millisecond counts, using `long long int` is often a necessity. Many problems deal with large numbers, such as those encountered in combinatorial problems or complex algorithms that compute sums of large iterative sequences. Failing to use `long long` can lead to incorrect results, ultimately affecting scores and outcomes.
Real-World Applications
In real-world scenarios, `long long int` is indispensable in fields like finance and engineering. Financial applications often require handling large monetary amounts or calculations involving significant figures, where using smaller data types risks truncating values. In scientific fields, simulations that model complex systems generate vast amounts of data, where `long long` ensures results remain precise.
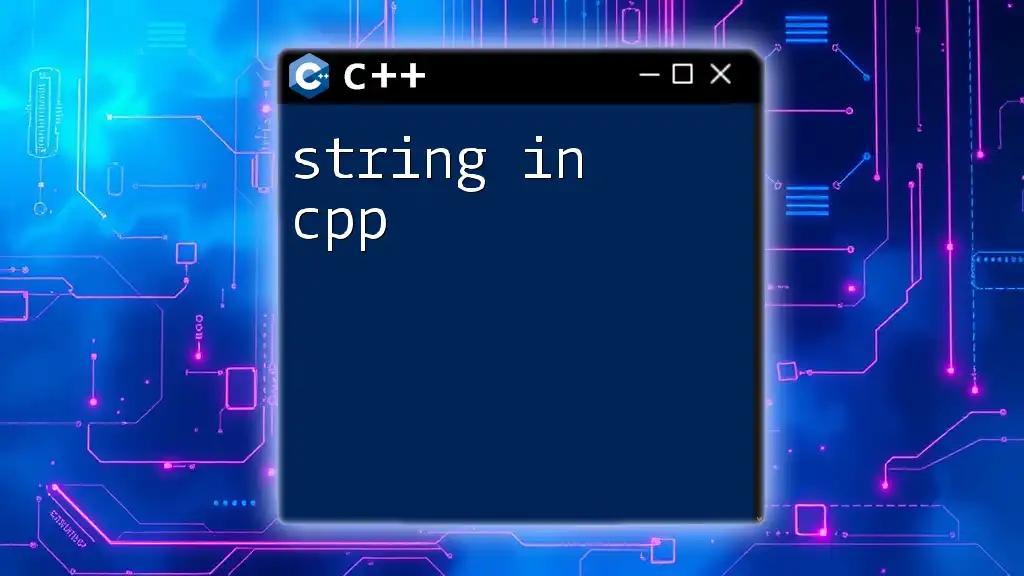
Conclusion
Understanding how to effectively utilize `long long int` enhances your programming capability in C++, especially when working with large datasets or intricate calculations. By mastering this data type, you can write robust and scalable code that prevents overflow and maintains precision, proving invaluable across various domains from competitive programming to real-world applications.

Frequently Asked Questions (FAQs)
What is the maximum value for `long long int`?
The maximum value for `long long int` is typically 9,223,372,036,854,775,807. This vast range ensures you can handle an extensive quantity of large numbers without worrying about overflow.
Can I mix `long long int` with other data types?
While it is technically possible to mix data types in C++, doing so is not recommended as it can lead to unexpected results. Always strive to use compatible types or perform explicit casting to avoid confusion and potential errors.
What libraries do I need to use `long long`?
To utilize `long long int`, you primarily need the standard input-output library in C++, which includes `<iostream>`. There are no additional libraries required specifically for `long long`, making it accessible in any standard C++ environment.