In C++, the `round` function rounds a floating-point number to the nearest integer, with halfway cases rounding away from zero.
#include <iostream>
#include <cmath>
int main() {
double value = 3.6;
std::cout << "Rounded value: " << std::round(value) << std::endl; // Output: 4
return 0;
}
Understanding Rounding in C++
Rounding is a mathematical technique used to reduce the number of decimal places in numerical data. This is particularly important in programming, where precise calculations can significantly affect results, whether you're representing monetary values, scientific data, or user inputs.
Various rounding techniques exist, including rounding up, rounding down, and rounding to the nearest integer. In C++, the `round` function provides a simple way to round floating-point numbers to the nearest whole number.
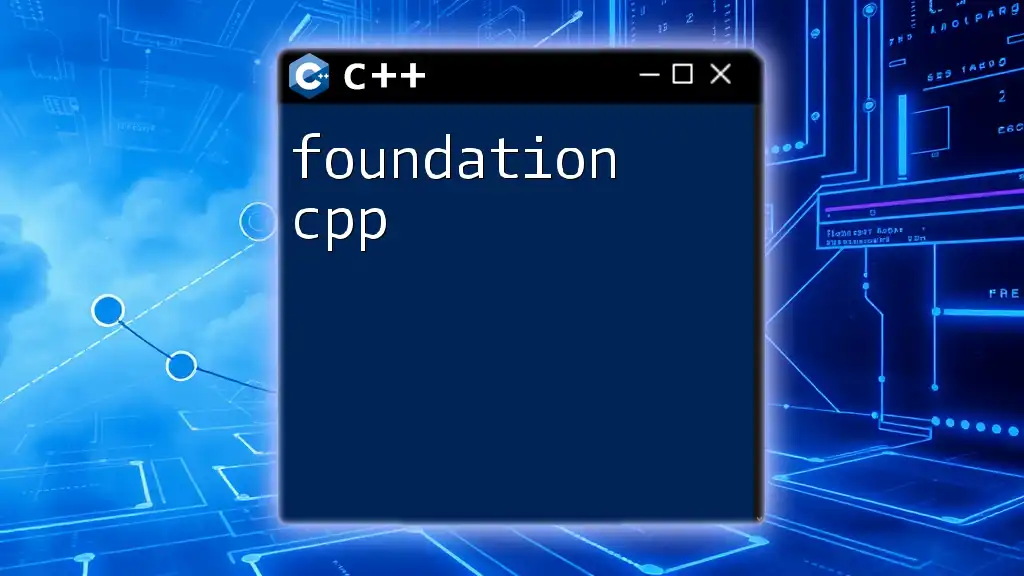
The C++ Round Function
What is the `round` Function in C++?
The `round` function in C++ is a standard library function defined in the `<cmath>` header. This function takes a floating-point number as input and returns the nearest integer as a double. The function rounds halfway cases away from zero. It's a crucial tool for developers who need a precise way to handle numerical data rounding.
Syntax of the C++ Round Function
The syntax for using the `round` function is straightforward:
double round(double x);
Parameters and Return Values
- Parameter: The function takes a single parameter `x`, which is a floating-point number that you want to round.
- Return Value: The return value is the nearest integer to `x`, and it is returned as a double.
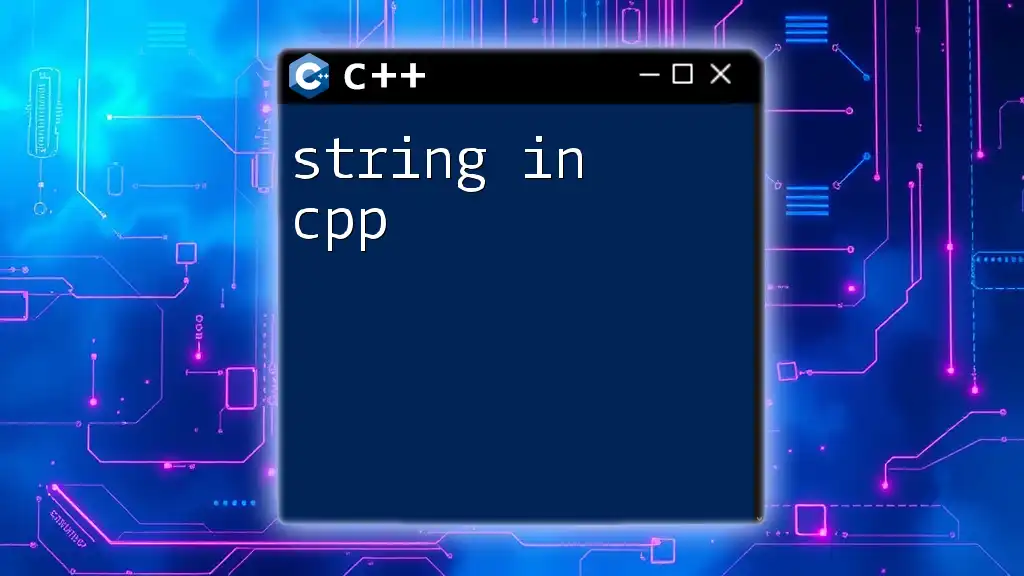
Using `round` in C++
Basic Examples of C++ `round`
To demonstrate the basic usage of the `round` function, consider the following code:
#include <iostream>
#include <cmath> // Required for round function
int main() {
double number = 5.8;
std::cout << "Rounded value of " << number << " is " << round(number) << std::endl;
return 0;
}
In this example, the output will be:
Rounded value of 5.8 is 6
The `round` function correctly rounds 5.8 up to 6, which illustrates its basic functionality.
Rounding Negative Numbers
The behavior of the `round` function with negative numbers is also essential to understand. For instance, consider the following example:
#include <iostream>
#include <cmath>
int main() {
double negativeNumber = -2.3;
std::cout << "Rounded value of " << negativeNumber << " is " << round(negativeNumber) << std::endl;
return 0;
}
In this case, the output will be:
Rounded value of -2.3 is -2
Here, the `round` function rounds -2.3 up (closer to zero) to -2, showcasing how it treats negative numbers differently than positive ones.
Rounding to Different Precision Levels
Sometimes, you might want to round a number to a specific number of decimal places. While the `round` function itself does not directly provide this functionality, you can achieve it through a simple formula. Here’s an example of rounding to two decimal places:
#include <iostream>
#include <cmath>
int main() {
double value = 3.14159;
double roundedValue = round(value * 100) / 100; // Rounding to two decimal places
std::cout << "Rounded value to two decimal places: " << roundedValue << std::endl;
return 0;
}
This code snippet produces the output:
Rounded value to two decimal places: 3.14
The multiplication by 100 before applying `round` shifts the decimal point, and dividing by 100 afterward restores it.
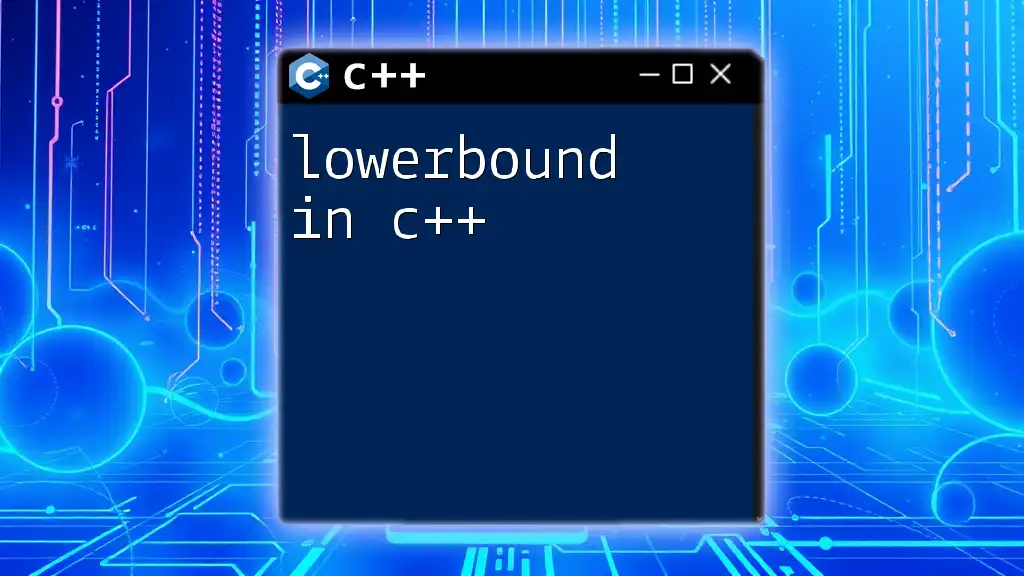
Comparing C++ Round with Other Rounding Functions
`floor` and `ceil` Functions
While the `round` function is useful, it's important to note that C++ also provides `floor` and `ceil` functions. The `floor` function rounds down to the nearest integer, while the `ceil` function rounds up to the nearest integer.
Usage Examples of `floor` and `ceil`
Here is an example to compare these functions:
#include <iostream>
#include <cmath>
int main() {
double value = 3.7;
std::cout << "Floor of " << value << " is " << floor(value) << std::endl;
std::cout << "Ceil of " << value << " is " << ceil(value) << std::endl;
return 0;
}
The output will be:
Floor of 3.7 is 3
Ceil of 3.7 is 4
This demonstrates how each function serves a distinct purpose, expanding your rounding toolkit in C++.
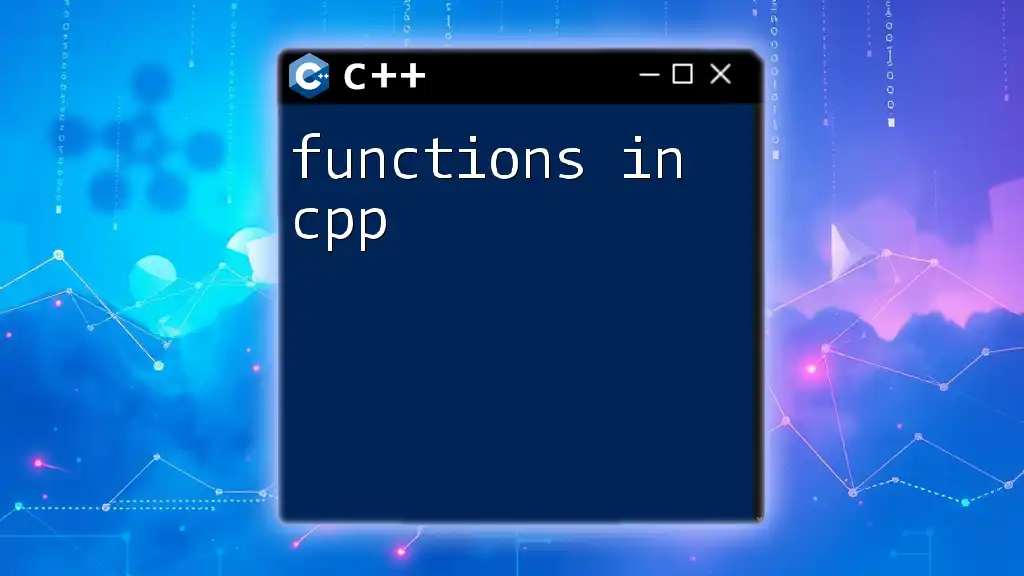
Common Use Cases of the Round Function in C++
Rounding in Financial Applications
In financial applications, the accuracy of numerical data is paramount. When working with monetary values, it is essential to round correctly to avoid misrepresentation. For example:
#include <iostream>
#include <cmath>
int main() {
double price = 19.99;
double tax = 0.075;
double totalCost = price + (price * tax);
double roundedTotal = round(totalCost * 100) / 100; // Rounding to the nearest cent
std::cout << "Total cost after tax: " << roundedTotal << std::endl;
return 0;
}
The output will show the total cost rounded to a manageable figure, ensuring that it accurately reflects the customer's total due.
Rounding in Scientific Calculations
Rounding also plays a significant role in scientific calculations, where precision is critical. For instance, when displaying results of calculations, rounding can help present results clearly without unwieldy decimal places.
#include <iostream>
#include <cmath>
int main() {
double measurement = 0.123456789;
double roundedMeasurement = round(measurement * 1000) / 1000; // Rounding to three decimal places
std::cout << "Rounded measurement: " << roundedMeasurement << std::endl;
return 0;
}
This example will yield a measurement rounded to three decimal places, perfect for use in scientific reporting.
Rounding in Graphics and Game Development
In graphical applications or game development, rounding can help in adjusting coordinates for visual elements. When positions are calculated, rounding those values can ensure they align to the nearest pixel, improving the visual layout.
#include <iostream>
#include <cmath>
int main() {
double coordinateX = 150.75;
double coordinateY = 200.60;
int roundedX = static_cast<int>(round(coordinateX));
int roundedY = static_cast<int>(round(coordinateY));
std::cout << "Rounded Coordinates: (" << roundedX << ", " << roundedY << ")" << std::endl;
return 0;
}
The output will produce the adjusted coordinates:
Rounded Coordinates: (151, 201)
This demonstrates how rounding impacts graphics positioning, leading to a cleaner visual output.
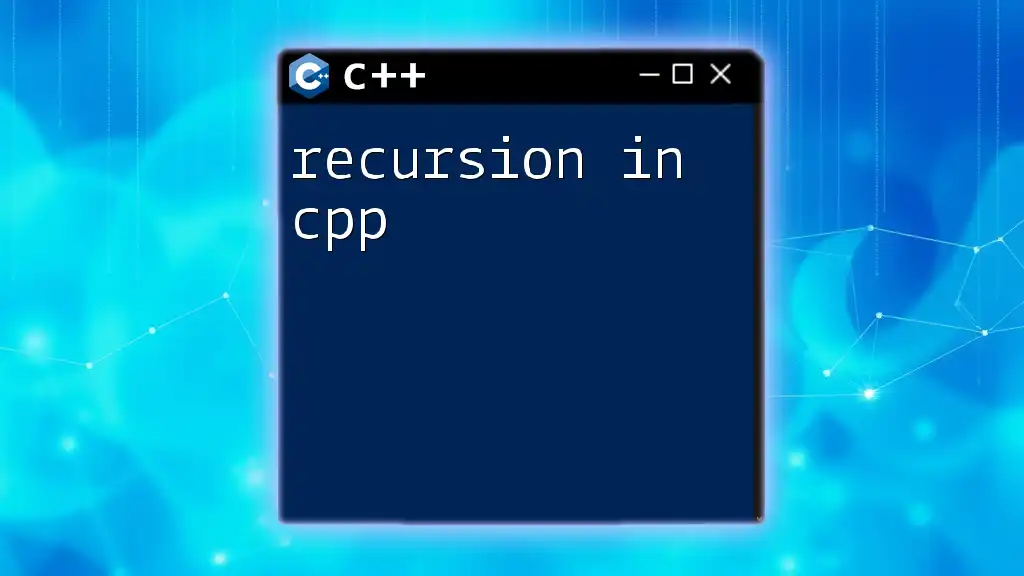
Best Practices for Rounding in C++
To effectively use the `round` function, consider these best practices:
- Understand the Context: Before applying rounding, consider the context in which the output will be used. Financial applications may require different rounding strategies than scientific applications.
- Use Appropriate Functions: Knowing when to use `round`, `floor`, or `ceil` can directly influence the accuracy of your outcomes. Understand the differences between these functions and apply them accordingly.
- Test Your Outputs: Always validate your rounding outputs to ensure they meet the requirements of your application. Test various input scenarios, such as positive/negative numbers and different decimal places.
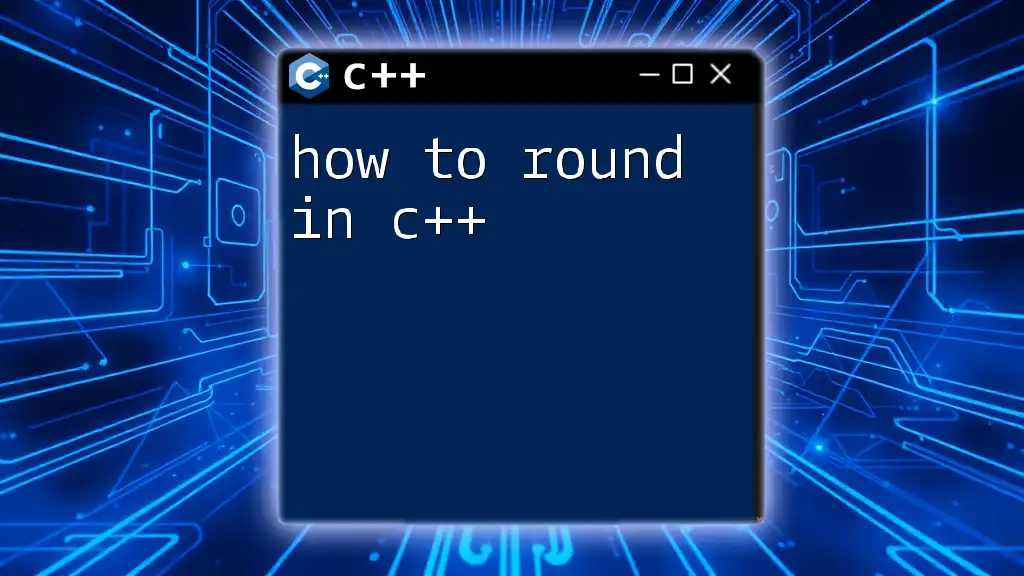
Conclusion
The `round` function in C++ provides a reliable method to handle floating-point rounding effectively. Whether you're working in finance, science, or graphics, understanding how to use `round` can enhance the quality and accuracy of your numerical data. Mastering this function and comparing it to alternatives like `floor` and `ceil`, along with being mindful of best practices, can significantly improve your coding skills in C++.
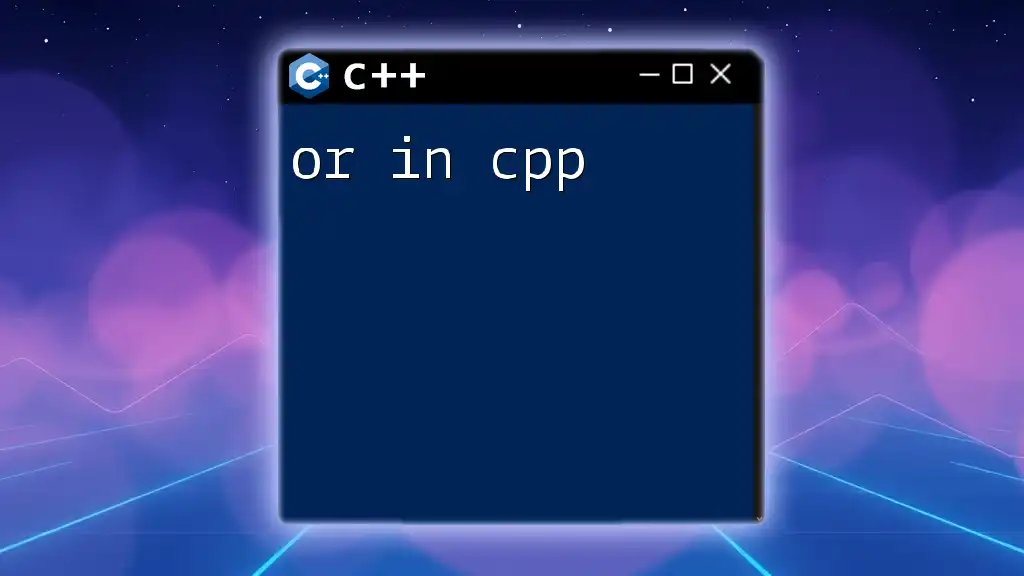
Additional Resources
To further explore the `round` function and its applications, consider consulting the C++ Standard Library documentation and engaging in practical coding exercises. This will help solidify your understanding and prepare you for real-world programming challenges.
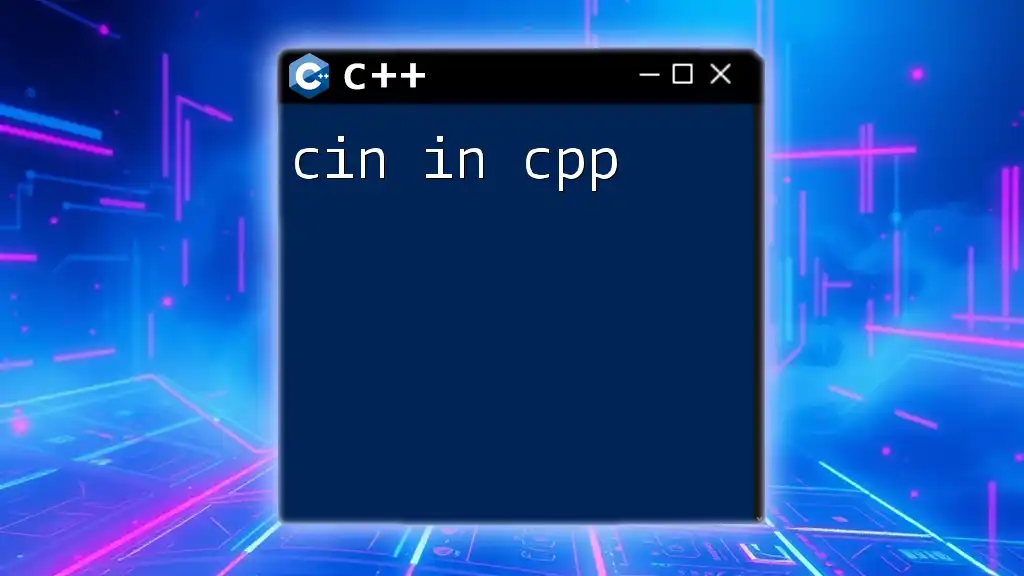
Call to Action
If you found this guide helpful, be sure to follow our blog for more insights and tutorials on C++ programming. Don’t hesitate to leave your comments or questions regarding the `round` function or any other C++ topics!