The "RKNN CPP" refers to the RKNN toolkit's C++ interface, which allows developers to efficiently deploy and run deep learning models on various platforms, with a focus on ease of use and performance.
Here's a simple code snippet demonstrating how to initialize and run inference using the RKNN C++ API:
#include "rknn_api.h"
// Example function to load the model and run inference
void runInference(const char* modelPath, const float* inputData, float* outputData) {
rknn_context ctx;
rknn_input inputs[1];
rknn_output outputs[1];
// Load RKNN model
rknn_init(&ctx, modelPath, 0, 0);
// Configure input
inputs[0].index = 0;
inputs[0].buf = (void*)inputData;
inputs[0].size = sizeof(float) * input_data_size;
inputs[0].pass_through = 0;
// Run inference
rknn_run(ctx, inputs, 1, outputs, 1);
// Get output
outputs[0].buf = (void*)outputData;
rknn_outputs_get(ctx, 1, &outputs[0], NULL);
// Clean up
rknn_outputs_release(ctx, 1, &outputs[0]);
rknn_destroy(ctx);
}
What is rknn?
Definition of rknn
rknn stands for Rockchip Neural Network, a framework designed for the efficient deployment of deep learning models on Rockchip devices. It offers a set of tools that allow developers to convert, optimize, and run neural network models tailored for various applications.
One of the key features of rknn is its ability to support various model formats, including TensorFlow, PyTorch, and Caffe, making it highly versatile. This framework leverages hardware acceleration offered by Rockchip's systems on chip (SoCs), resulting in high performance and low latency in inference tasks.
Applications of rknn
The rknn framework finds utility across multiple domains:
- Embedded Systems: Ideal for edge devices like smart cameras, IoT sensors, and drones.
- Automotive Industry: Enables real-time object detection and decision-making systems in autonomous vehicles.
- Healthcare: Facilitates medical image analysis and diagnostic tools.
- Smart Retail: Enhances customer experience through facial recognition and inventory management.
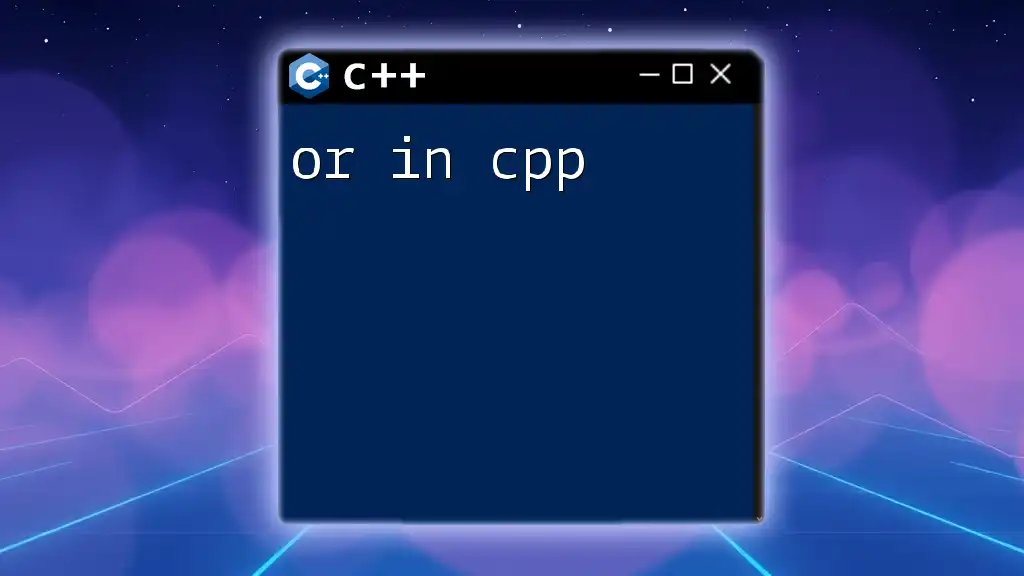
Getting Started with rknn cpp
Setting Up Your Environment
To begin working with rknn cpp, it is crucial to have the right environment set up.
First, ensure that you have the following installations:
- rknn framework: The core of your development work. You can download it from the official Rockchip site.
- C++ compiler: A modern compiler like GCC or Clang is recommended.
After installing, you can verify that everything is working correctly by running a simple test command:
#include <rknn_api.h>
// Your test code here.
Basic Commands in rknn cpp
Initializing rknn
Before utilizing any features, it is essential to initialize the rknn context.
rknn_context ctx;
This command sets up the context you will be working in, allowing you to access various rknn functions.
Loading a Model
After initializing, the next crucial step is to load your pre-trained model into the rknn context.
rknn_load_model(ctx, 'model.rknn');
Use this command to load models that you have previously converted to the rknn format. Supported formats include TensorFlow models saved as `.pb` files or PyTorch models converted via ONNX.
Running Inference
Input Preparation
Input data must be correctly formatted to ensure successful inference.
To format the input data correctly, ensure that it matches the input dimensions required by your model. This usually involves resizing images or normalizing data values.
Example code snippet for preparing input data might look as follows:
// Example for preparing image input
uint8_t* input_data = (uint8_t*)malloc(height * width * channels);
memcpy(input_data, your_image_data, height * width * channels);
Executing Inference Command
Once the input data is prepared, execute the inference command to run your model.
rknn_run(ctx, input_data);
This command triggers the model to process the provided input data. It is essential as it initiates the inference process.
Processing Output
After running inference, you need to retrieve the output.
You can process the output data using the following command:
rknn_query(ctx, RKNN_QUERY_OUTPUT, output_data, &output_size);
This command retrieves the results, with explanation points indicating how you should interpret the output depending on your model's purpose—like classification probabilities or bounding box coordinates.
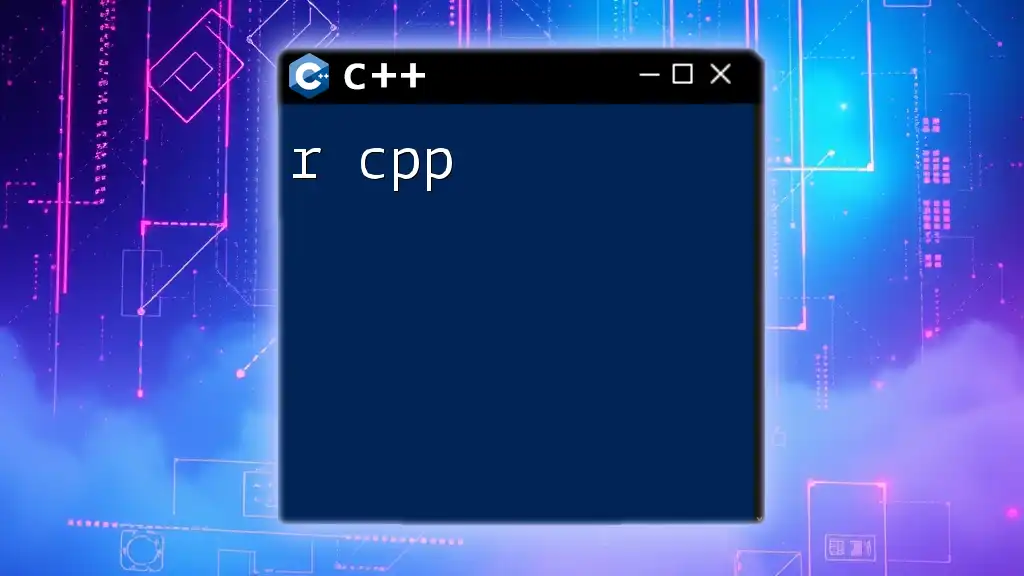
Advanced rknn cpp Commands
Model Optimization Techniques
To enhance performance, consider applying optimization techniques to reduce model size and speed up inference.
Common techniques include:
- Quantization: Reducing model weights from floating-point to fixed-point representation.
- Layer Fusion: Combining operations to minimize the number of layers and enhance computational efficiency.
Employing such techniques can notably improve the overall performance of your application.
Error Handling in rknn cpp
Common Errors
As with any development framework, encountering errors is common. Some typical errors you might face include:
- Model loading fails due to unsupported formats.
- Inconsistent input sizes or types.
- Memory allocation issues.
Implementing Error Checks in Your Code
Incorporating error handling into your code is vital for robust development. You can use the following structure to manage errors effectively:
if (rknn_get_error_code(ctx) != RKNN_SUCCESS) {
// Handle error accordingly
}
By checking for error codes throughout your workflow, you can identify and troubleshoot issues promptly.
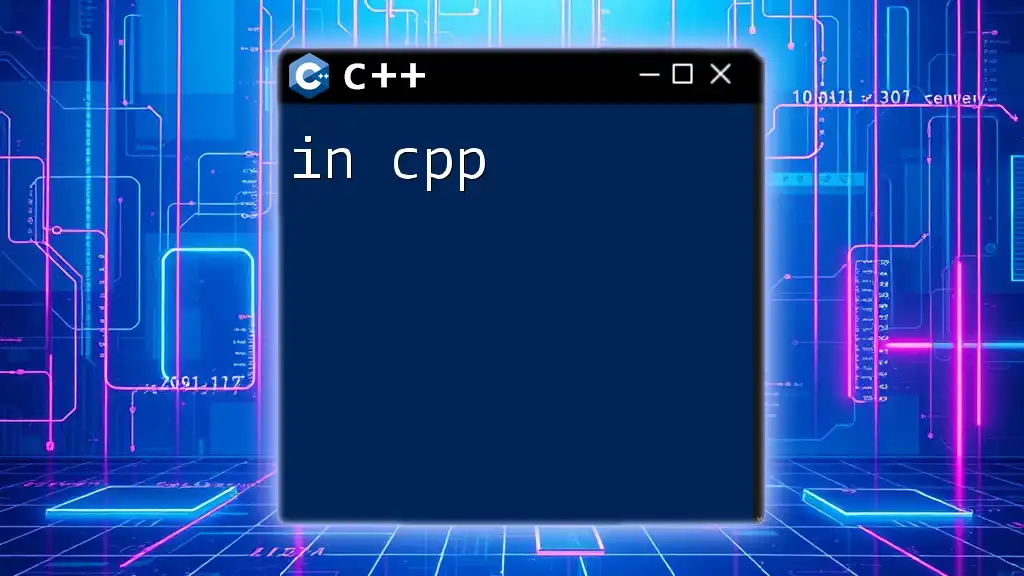
Performance Tuning in rknn cpp
Benchmarking Your Model
Performance evaluation is crucial in determining how well your model performs in real-world scenarios.
Use metrics like inference time and accuracy to benchmark your models. Create a baseline for performance and iterate on improvements by analyzing where time is spent for each inference run.
Optimization Strategies
Optimizing inference speed while maintaining model accuracy often involves making strategic trade-offs. Consider techniques such as:
- Reducing image size during preprocessing.
- Adjusting batch sizes for input data to maximize throughput.
These strategies can significantly enhance performance without sacrificing the integrity of your outputs.
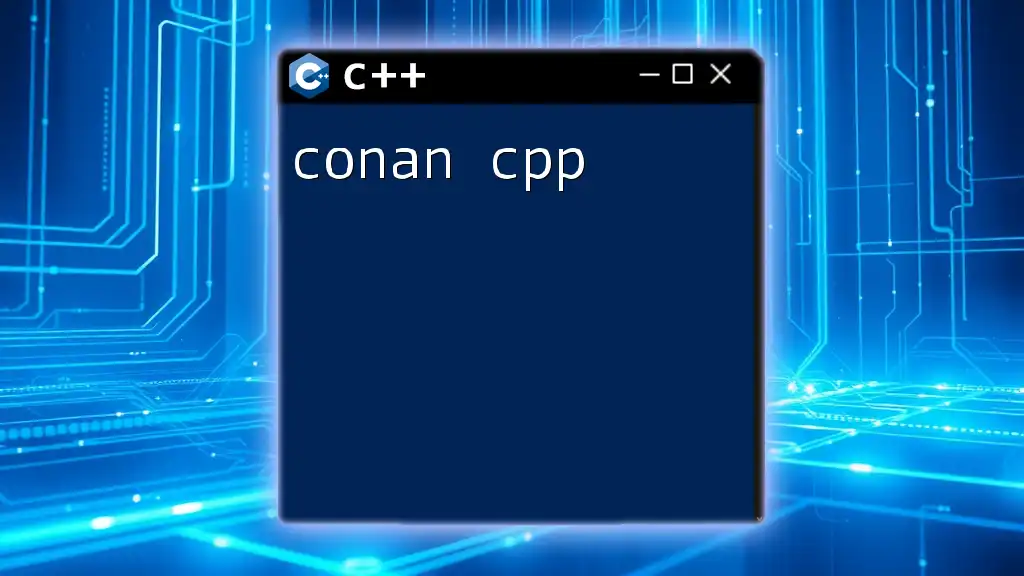
Debugging rknn cpp Applications
Common Debugging Techniques
When debugging rknn cpp applications, utilize the following techniques to trace issues:
- Check logging outputs to identify where failures occur.
- Use debugging tools like gdb or Valgrind to investigate memory-related issues.
Example Debugging Scenario
Consider a scenario where the model fails to load. You might check model compatibility and ensure that you’ve correctly specified the file path.
Implementing print statements or using a debugger can assist in identifying whether the model was correctly converted and if it is the accepted format.
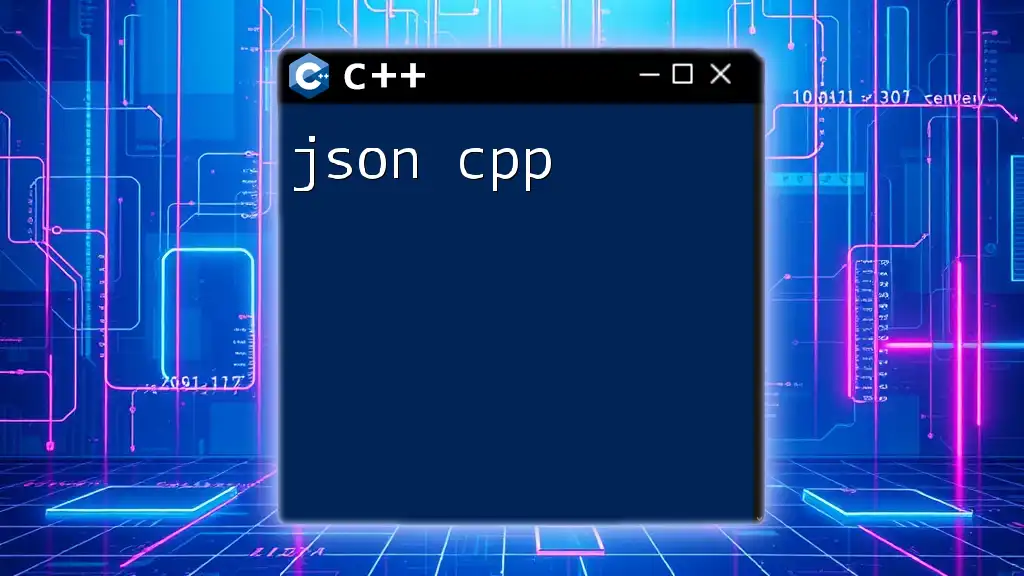
Best Practices for rknn cpp Development
To enhance the quality of your rknn cpp projects, follow these best practices:
- Code Structure: Keep your code modular to improve readability and ease maintenance.
- Documentation: Document function purposes and usage examples to provide clarity for users and future developers.
- Version Control: Use platforms like Git to manage changes and maintain versions of your project.
Conclusion
The rknn cpp framework empowers developers to effectively harness the capabilities of deep learning on Rockchip hardware. Mastering its commands and features allows you to build powerful applications that can function seamlessly on the edge or in the cloud.
FAQs
As you delve deeper into rknn cpp, a myriad of commonly asked questions can surface. Look for further insights and community advice to bolster your understanding and implementation ways.
Additional Resources
For extended learning, consult the official rknn documentation, join community forums, or explore online courses that dive into advanced rknn concepts. Happy coding!