The `fwrite` function in C++ is used to write binary data to a file stream, allowing efficient storage of data structures.
Here's a code snippet demonstrating its usage:
#include <cstdio>
int main() {
FILE *file = fopen("output.bin", "wb");
int data[] = {1, 2, 3, 4, 5};
fwrite(data, sizeof(int), 5, file);
fclose(file);
return 0;
}
Understanding the `fwrite` Function
What is `fwrite`?
`fwrite` is a standard library function in C++ included in `<cstdio>`, primarily used for writing binary data to files. It offers a straightforward approach to handling raw data, making it essential in scenarios where performance and data integrity are critical. Unlike text-based operations that handle format conversions and character encodings, `fwrite` directly writes the byte representation of data to the specified file, thus enabling efficient access to binary data.
Syntax of `fwrite`
The general syntax of `fwrite` is as follows:
size_t fwrite(const void *ptr, size_t size, size_t count, FILE *stream);
In this syntax:
- `ptr`: A pointer to the data you want to write.
- `size`: The size (in bytes) of each element you want to write.
- `count`: The number of elements to write.
- `stream`: A pointer to the file where the data will be written.
This function returns the total number of elements successfully written, which may be less than `count` when an error occurs or the end of the file is reached.
Return Value of `fwrite`
The return value is crucial for error handling. If `fwrite` returns a number less than `count`, it indicates that not all requested bytes were written. This can occur due to several issues, including a full disk or closed stream. Therefore, it’s essential to call `ferror()` after `fwrite` to check for errors.
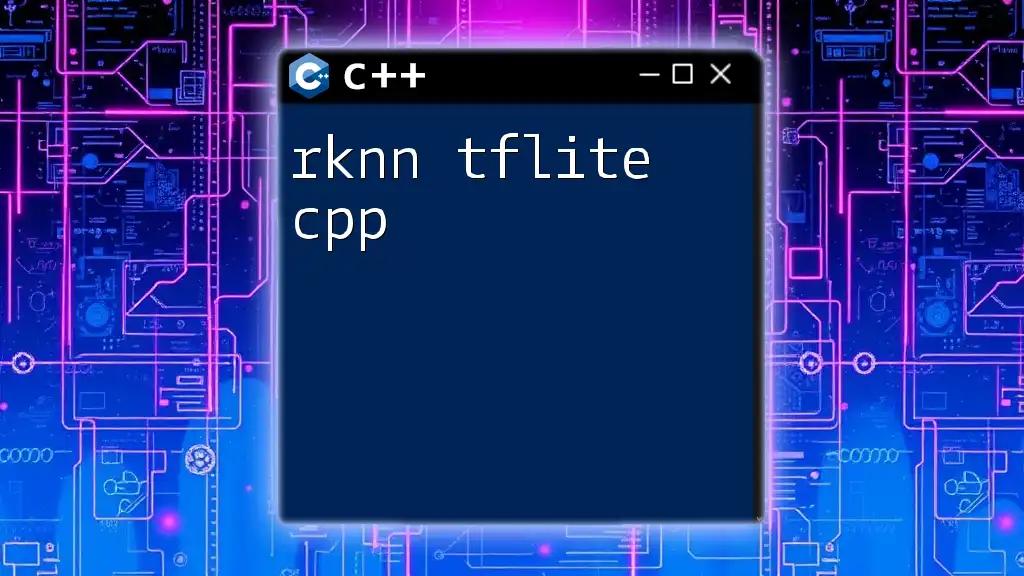
Setting Up for `fwrite`
Including Required Headers
To utilize `fwrite`, you need to include the appropriate headers in your program. The most common header is:
#include <cstdio> // For file handling functions
Opening a File for Writing
Before writing data, ensure that the file is opened correctly. The mode in which you open the file dictates how you can interact with it.
To open a file for binary writing, use `"wb"`:
FILE *file = fopen("data.bin", "wb");
if (file == nullptr) {
perror("Error opening file");
return EXIT_FAILURE;
}
In this example, if the file does not open successfully, the program will output an error message using `perror`.
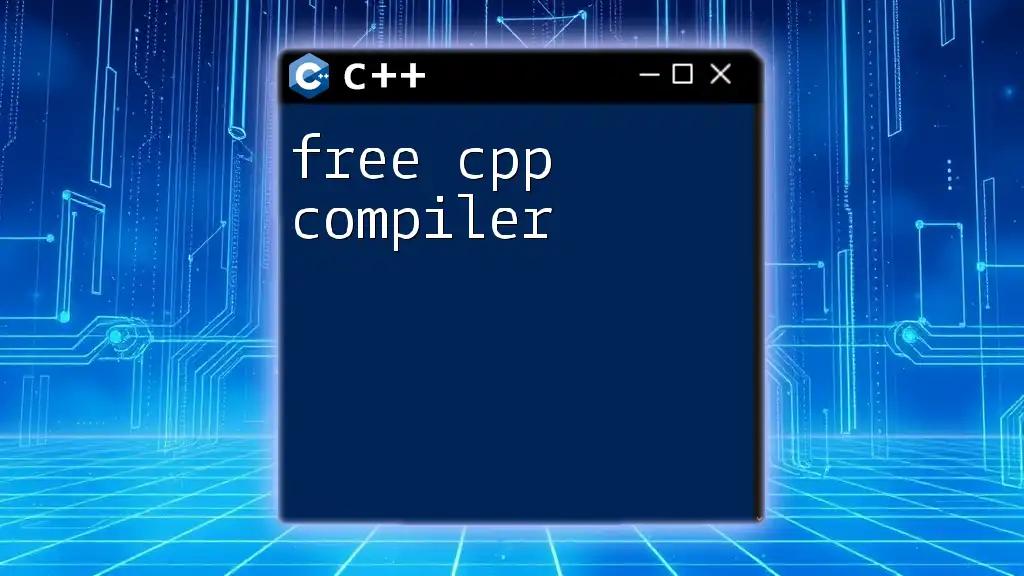
Writing Data Using `fwrite`
Writing Basic Data Types
To demonstrate writing a basic data type, consider an integer:
int number = 42;
size_t result = fwrite(&number, sizeof(int), 1, file);
In this snippet:
- `&number` serves as a pointer to the integer data.
- `sizeof(int)` defines how many bytes to write per element.
- The second parameter indicates that we want to write one element.
Tip: Always check the return value of `fwrite` to ensure that the intended data was successfully written to the file.
Writing Complex Data Types
When dealing with structures or arrays, `fwrite` shines. Let’s consider writing an array of structs:
struct Data {
int id;
float value;
};
Data dataArray[3] = { {1, 10.5}, {2, 15.2}, {3, 30.7} };
size_t written = fwrite(dataArray, sizeof(Data), 3, file);
In this case:
- A structure `Data` is defined with an integer and a float.
- `dataArray` holds multiple instances of `Data`.
- This example writes the entire array in one shot, showcasing `fwrite`'s ability to handle complex and multi-element data efficiently.

Closing the File
Importance of Proper Closure
After writing, it is vital to close the file to ensure all buffers are flushed and resources are released. Not closing the file can lead to data loss or corruption.
fclose(file);
This line of code properly closes the file stream, ensuring that the data is written to disk and memory is freed.

Error Handling with `fwrite`
How to Handle Errors
Error handling is paramount when working with file operations. After executing `fwrite`, make sure to evaluate its success:
if (result < 1) { // If result is less than expected
if (ferror(file)) {
perror("Error writing to file");
}
}
Using `ferror()`, you can diagnose any problems that occurred during the writing process, allowing for better debugging and stability in your code.
Best Practices for Error Handling
Implementing comprehensive error handling ensures that issues are caught and managed gracefully. Log or handle errors appropriately to inform users of potential problems without crashing your application.
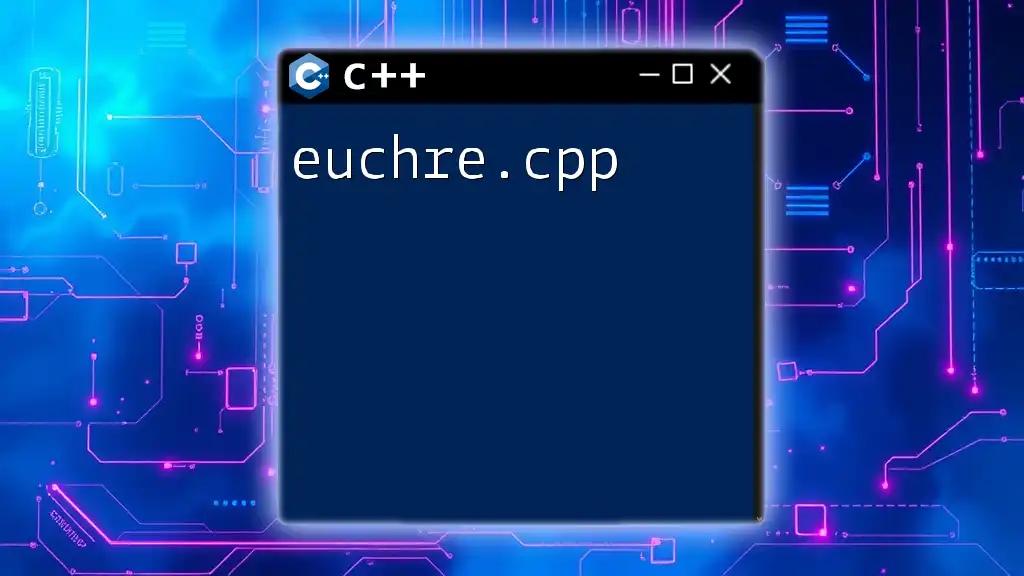
Performance Considerations
When to Use `fwrite`
`fwrite` is most beneficial when you need to handle large quantities of data quickly and without the overhead associated with text parsing. For instance, scientific computations often involve writing binary data for performance reasons.
Buffer Sizes and Optimization
When it comes to performance, buffer sizes can greatly impact speed and efficiency. Adjusting buffer sizes or utilizing custom buffers can lead to optimized performance when working with large files.
const int BUFFER_SIZE = 1024;
char buffer[BUFFER_SIZE];
size_t elements_written = fwrite(buffer, sizeof(char), BUFFER_SIZE, file);
This approach allows you to write data in larger blocks, minimizing the number of I/O operations and thus increasing speed.

Conclusion
The `fwrite` function provides a powerful and efficient method for writing binary data to files in C++. With proper understanding and implementation, you can leverage this function for various data handling tasks effectively. Embracing the nuances of binary file operations will enhance your programming skills and enable you to handle data more proficiently.

FAQs about `fwrite` in C++
Common Questions and Answers
-
What happens if I try to write a string using `fwrite`? Writing a string directly will not work as expected since `fwrite` interprets the string as a series of characters. You need to handle strings based on their byte representation.
-
Can `fwrite` be used for text files? While it is possible to write text data using `fwrite`, it is generally not recommended. Text should be handled through text-based functions like `fprintf` or `fputs` to avoid encoding issues.
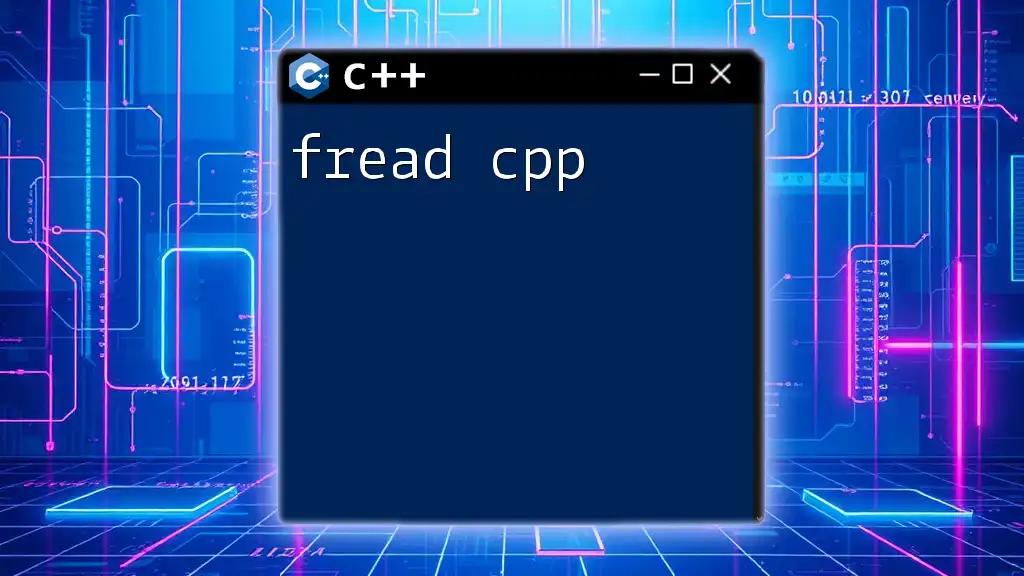
Further Resources
Learning Materials
To deepen your understanding of file I/O in C++, consider consulting these resources:
- Classic books on C++ programming and file handling.
- Online tutorials that provide hands-on examples and exercises.
- Documentation sites like cppreference.com for technical insights and further information.
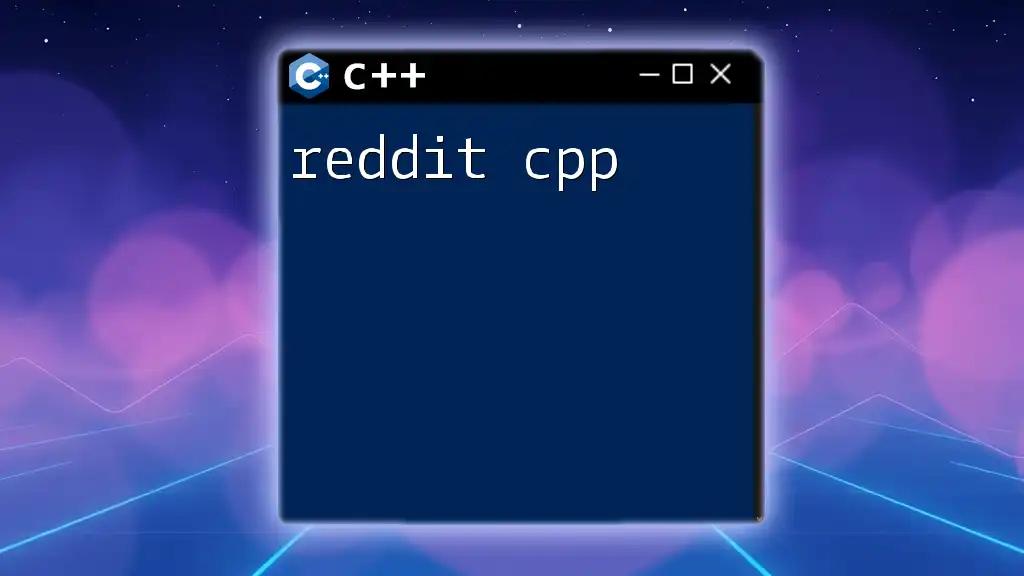
Final Thoughts
Experimenting with `fwrite` in different scenarios will bolster your understanding and mastery of file operations in C++. Embrace the learning journey, and don’t hesitate to share your experiences or ask questions as you explore this powerful function in C++.