To write to a file in C++, you can use the `ofstream` class to create and manipulate files easily. Here's a simple example:
#include <fstream>
int main() {
std::ofstream outFile("example.txt");
outFile << "Hello, World!" << std::endl;
outFile.close();
return 0;
}
Understanding File Streams in C++
What are File Streams?
File streams are essential components for managing input and output operations in C++. They allow programs to read from and write to files seamlessly, providing a way to handle data persistence. By using file streams, you can store information, retrieve it later, and manipulate it as needed, making them fundamental for any application that requires data storage.
Types of File Streams
In C++, there are three primary types of file streams:
-
ifstream: This is used for reading data from files. It opens the specified file for input and allows you to retrieve data from it.
-
ofstream: This stream is specifically designed for writing to files. It opens the file for output, enabling you to save data to it.
-
fstream: This versatile stream can handle both input and output, allowing you to read from and write to the same file. It’s useful for applications where you need to both process and produce data.
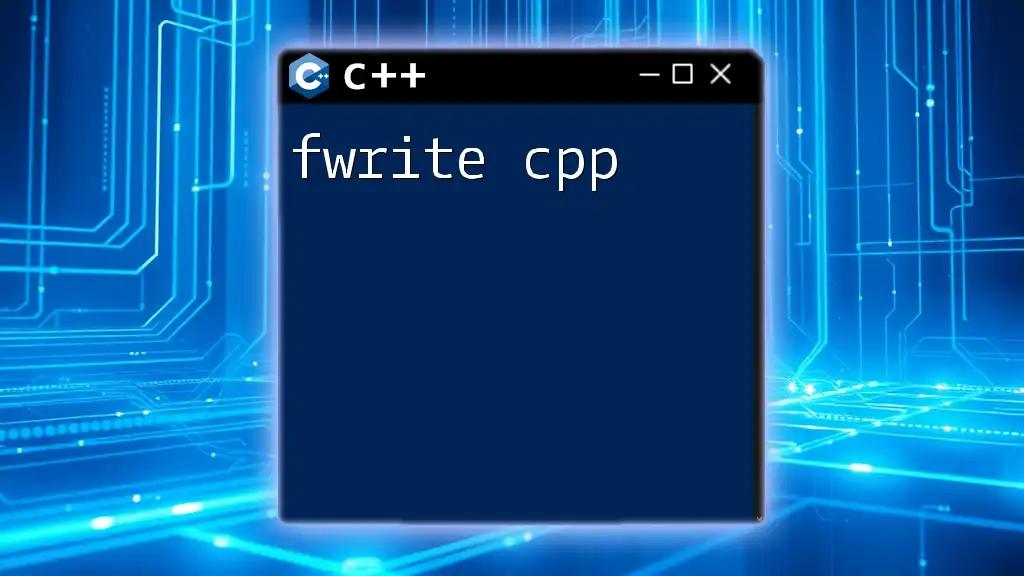
Setting Up Your Environment
Required Libraries
To perform file operations in C++, you need to include the `<fstream>` library. This header file contains the definitions for the various file stream classes. You can import it into your program by adding the following line at the top of your source code:
#include <fstream>
This inclusion gives your program the ability to utilize file stream functionalities needed for writing to files.
Writing a Simple C++ Program to Write to a File
Before delving into writing files, let's establish a basic C++ program structure for file operations. Here is a template to get you started:
#include <iostream>
#include <fstream>
int main() {
// Declare an output file stream
std::ofstream outFile("output.txt");
// Logic for writing to the file will go here
// Don't forget to close the file
outFile.close();
return 0;
}
This code sets up an output file named `output.txt` and prepares to write data to it. Each section will be filled in as we expand on writing to files.
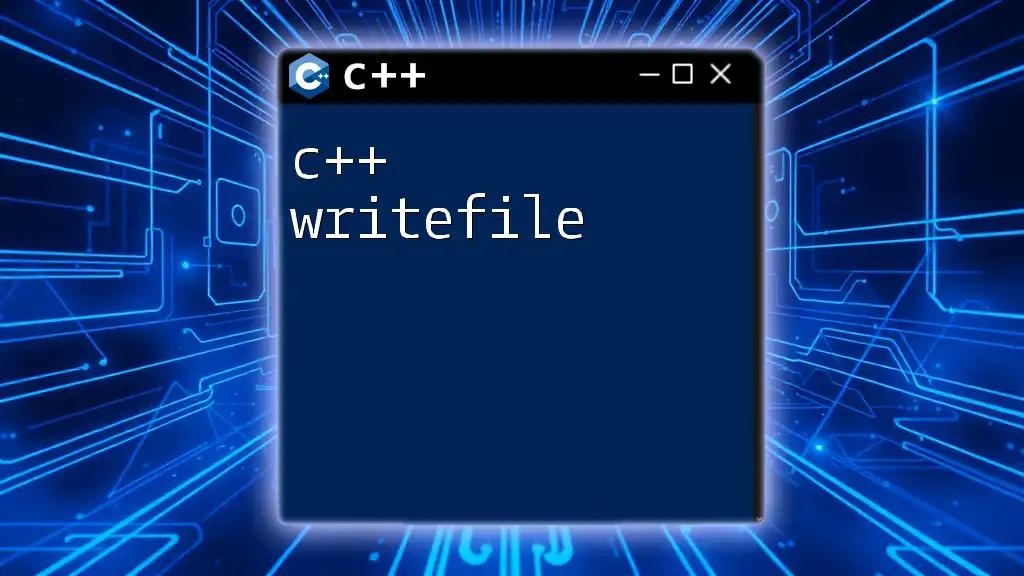
Writing to a File in C++
Opening a File for Writing
To write data to a file, you need to open it using `ofstream`. This is the backbone of the file operation that allows you to specify the file you want to write to. Here’s a fundamental example:
#include <fstream>
int main() {
std::ofstream outFile("example.txt"); // Default mode is std::ios::out
if (!outFile) {
std::cerr << "Error opening file" << std::endl;
return 1; // Exit if the file could not be opened
}
// Writing logic goes here
outFile.close(); // Remember to close the file
return 0;
}
In this example, the file `example.txt` is opened for writing. Always ensure that you check whether the file opened successfully, as failing to do so can lead to runtime errors when trying to write to an uninitialized stream.
Writing Data to the File
Using the Insertion Operator
Once your file is opened correctly, you can use the insertion operator `<<` to write data to it. The operator functions similarly to how it works with standard output (like `std::cout`), making it intuitive to use. Here is how you can write a simple line of text:
outFile << "Hello, World!" << std::endl;
This line will insert the string `"Hello, World!"` followed by a newline character into `example.txt`. The `std::endl` is important as it ensures the output buffer is flushed, and a new line is created in the file.
Writing Other Data Types
You can write multiple data types to files using the insertion operator. Here’s an example of writing an integer to the file:
int number = 42;
outFile << "The number is: " << number << std::endl;
This command writes the string `"The number is: "` followed by the value of `number` to the file, resulting in the line:
The number is: 42
You can similarly write floating-point numbers, characters, and strings, making the insertion operator a versatile choice for file output.
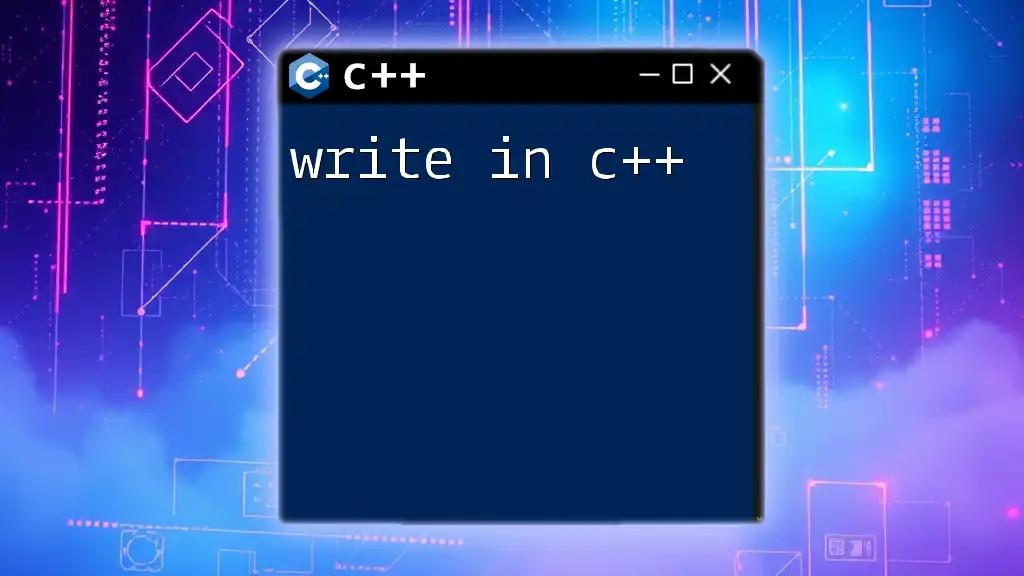
Manage Output Files in C++
Checking If File Opened Successfully
After attempting to open a file, it’s crucial to verify that the file stream is in a good state. This serves to prevent unexpected crashes in your application. You can check if the file opened successfully with the following code:
if (!outFile) {
std::cerr << "Error opening file" << std::endl;
return 1; // Handle the failure case appropriately
}
This verification ensures that your program will take appropriate action if the file cannot be opened, such as displaying an error message and gracefully exiting.
Closing the File
Closing your opened file is just as important as opening it. Leaving files open can lead to memory leaks and data loss. The function `close()` is used to properly close the file:
outFile.close();
Always remember to close your streams when you finish with them to maintain resource integrity.

Writing to a File in C++: Best Practices
Choosing the Right File Mode
When opening a file, it's essential to select the correct mode. For instance, if you want to append data to an existing file rather than overwrite it, use `std::ios::app`:
std::ofstream outFile("example.txt", std::ios::app);
This mode opens the file in a way that new data is written at the end of the file instead of overwriting existing content.
Handling Exceptions
Robust error handling is vital. Utilizing `try` and `catch` blocks can help manage exceptions that arise during file operations. Here's an example:
#include <fstream>
#include <iostream>
int main() {
try {
std::ofstream outFile("output.txt");
if (!outFile) {
throw std::ios_base::failure("Error opening file");
}
outFile << "Writing data to the file." << std::endl;
outFile.close();
} catch (const std::exception &e) {
std::cerr << e.what() << std::endl;
}
}
In this code, if the file cannot be opened, an exception is thrown and caught in the `catch` block, which outputs the error message.
File Path Considerations
Be mindful of file paths when specifying the location of your output file. You can use both relative and absolute paths, depending on your project's structure. For example:
std::ofstream outFile("C:/myfolder/example.txt"); // Absolute path
This approach ensures that your program writes to the intended directory, avoiding potential misplacement of your output files.
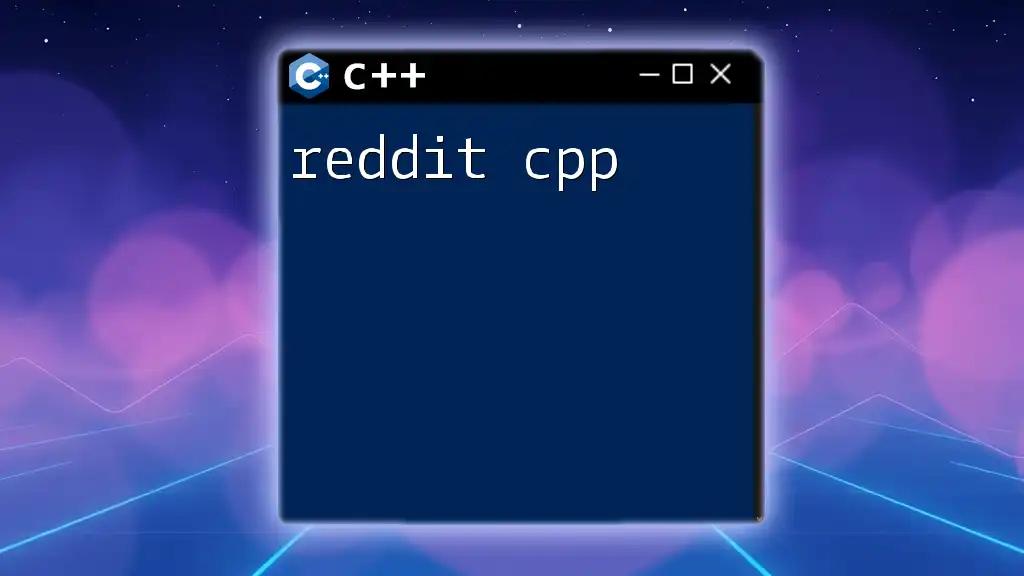
Common Mistakes to Avoid
While working on file operations, developers may encounter specific pitfalls. Here are some common mistakes to watch out for:
- Forgetting to check if the file opened successfully: Always validate that the file stream is ready for use.
- Neglecting to close the file: Ensure that you call `close()` to prevent resource leaks.
- Using incorrect file modes: Be aware of the implications of each file mode when opening a file to avoid unintentional data loss.
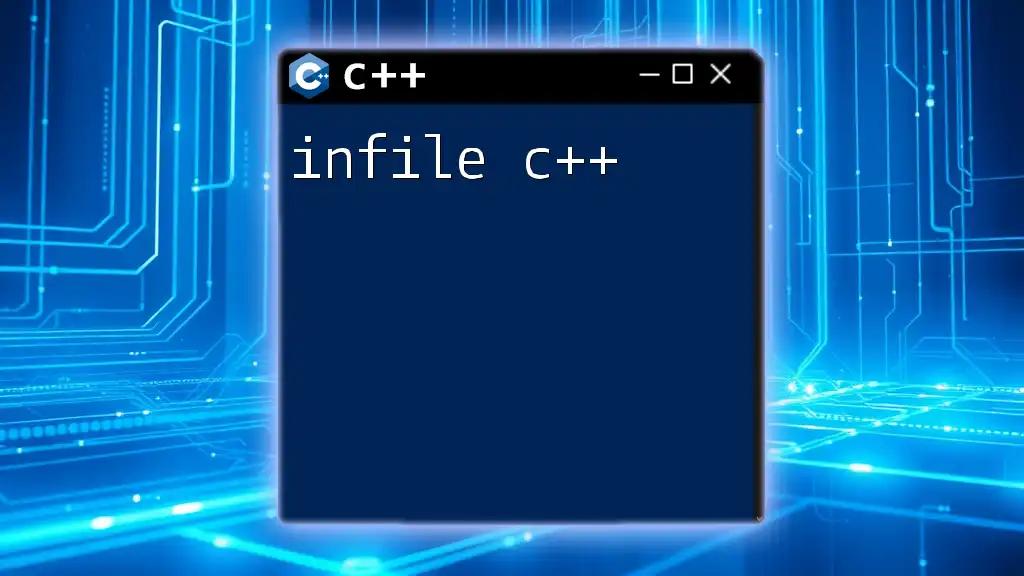
Conclusion
Understanding how to write file cpp is a significant step in mastering C++. By grasping the fundamentals of file streams, opening, writing to, and properly managing output files, you can leverage this knowledge to enhance your applications. Practice writing to files with the examples provided and experiment with different data types and file modes. As you gain confidence, you can delve into more complex file operations like binary writing and structured data management. Happy coding!
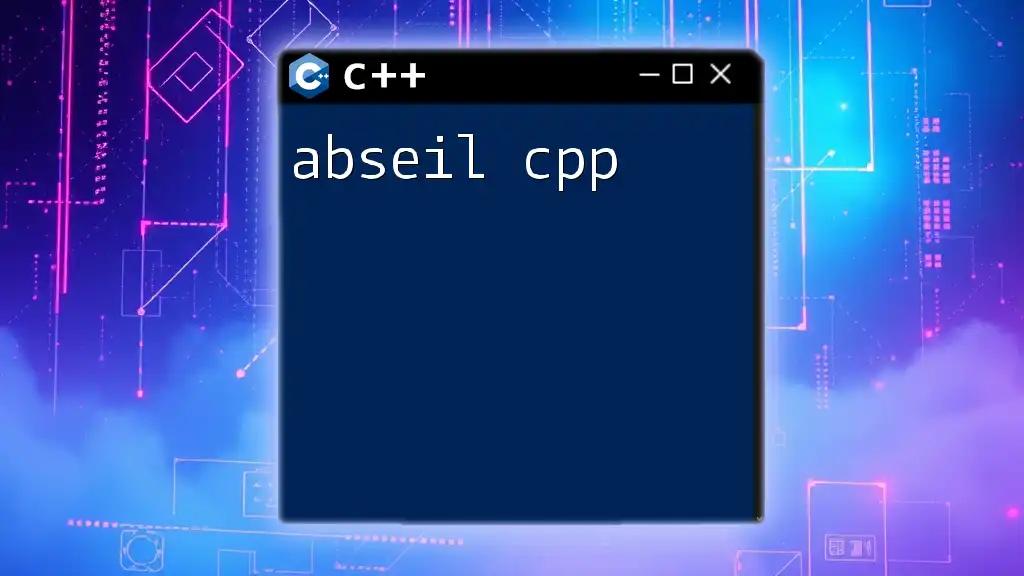
Additional Resources
For those looking to further their knowledge, consider exploring the following resources:
- C++ File I/O Documentation: [cplusplus.com](http://www.cplusplus.com)
- C++ Programming Tutorials: [LearnCPP.com](https://www.learncpp.com)
- Online C++ Courses and Tutorials: [Udemy](https://www.udemy.com), [Coursera](https://www.coursera.org)
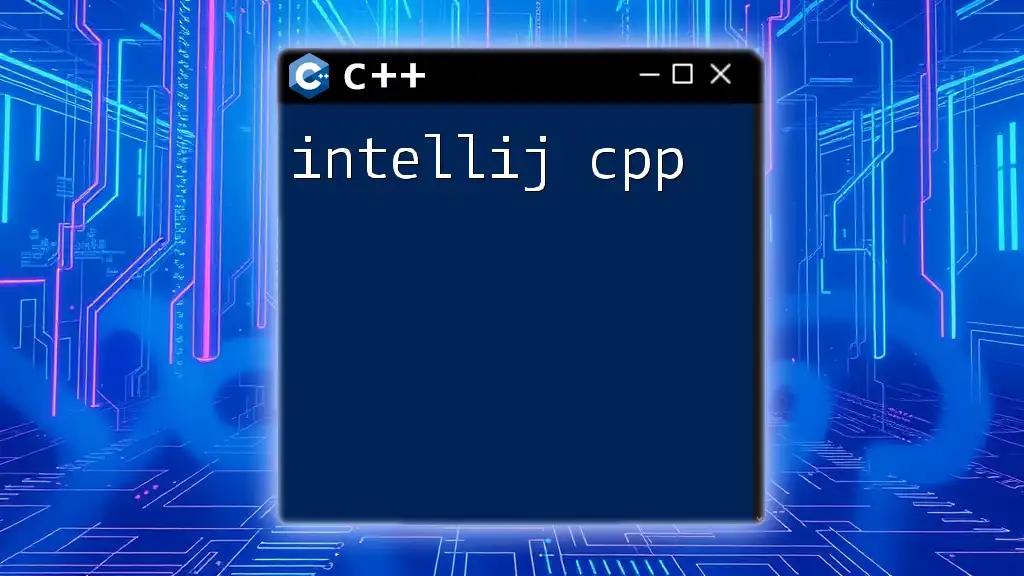
FAQs
What is the difference between `ofstream` and `fstream`?
- ofstream is for output only, while fstream can handle both input and output operations on files.
How do I append data to an existing file?
- Open the output file with `std::ios::app` mode:
std::ofstream outFile("example.txt", std::ios::app);
Can I write binary data to a file in C++?
- Yes, you can write binary data by opening the file in binary mode. Use `std::ios::binary` in conjunction with your file opening code.
By following the guidance in this article, you will be well-equipped to handle file operations in C++, enhancing your programming toolkit and enabling innovative data management solutions.