When deciding whether to write in C++ or Java, consider that C++ offers more control over system resources and performance, while Java provides a simpler syntax and built-in memory management.
Here’s a simple example of the "Hello, World!" program in both languages:
// C++ Hello, World!
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
// Java Hello, World!
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Understanding C++
What is C++?
C++ is a powerful programming language that was developed as an extension of the C language. Created by Bjarne Stroustrup in the early 1980s, C++ has evolved significantly and has become a cornerstone in systems programming, game development, and performance-critical applications. Its capacity for low-level memory manipulation and resource management allows developers to create highly efficient software solutions.
Features of C++
One of the standout features of C++ is its ability for low-level manipulation. This means you can manage memory directly using pointers, which provides critical control over how resources are allocated and managed. For example, allocating dynamic memory in C++ can be done as follows:
int* ptr = new int; // Allocates memory dynamically
*ptr = 10; // Assigns a value to the allocated memory
This level of control can be incredibly beneficial in performance-sensitive applications. Another prominent feature is performance. C++ is known for its execution speed, making it ideal for scenarios where efficiency is paramount. When compared to Java, C++ often outperforms in computational tasks due to its compiled nature.
Use Cases for C++
C++ shines in systems programming, where it is used to develop operating systems and device drivers. It gives developers the tools to interact closely with hardware, making it ideal for embedded systems.
In game development, C++ is widely used in popular game engines like Unreal Engine. The language's efficiency and performance make it possible to create graphics-intensive applications that run smoothly across various platforms.
Moreover, for performance-critical applications, such as financial trading systems and simulations, C++ is often the go-to choice due to its speed and flexibility.
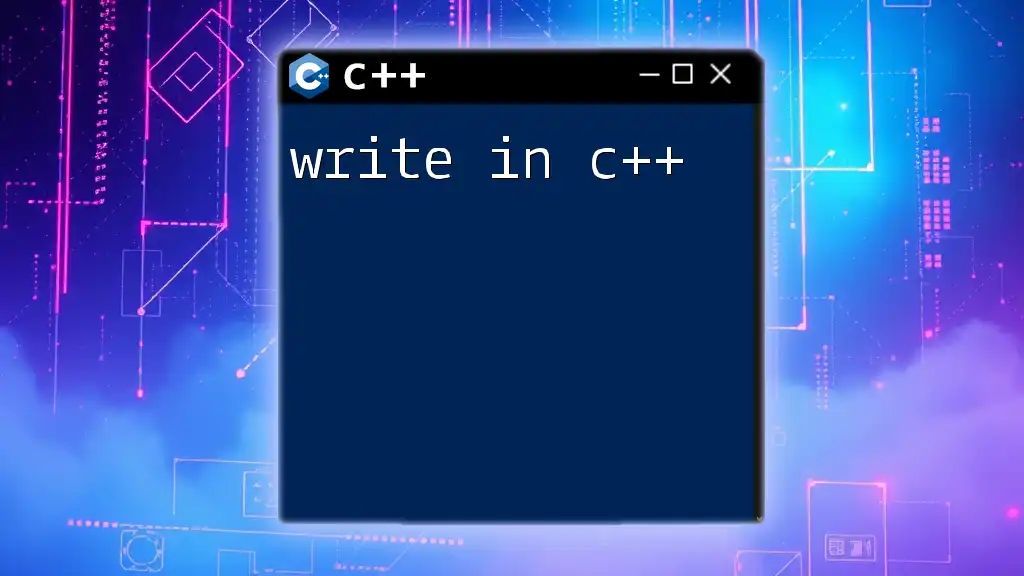
Understanding Java
What is Java?
Java is a high-level, object-oriented programming language that was developed by Sun Microsystems in the mid-1990s. With its mantra of Write Once, Run Anywhere (WORA), Java provides a significant advantage through its platform independence, meaning that code can run on any system that has a Java Virtual Machine (JVM).
Features of Java
One of Java's key features is automatic memory management facilitated by garbage collection. This abstracts much of the complexity of memory allocation and deallocation away from developers, allowing them to focus on other aspects of their applications. A simple example in Java illustrates this:
String str = new String("Hello"); // Memory management is handled by the JVM
Another prominent feature is its rich standard library. Java offers a vast array of libraries and frameworks that aid in rapid application development. For instance, the Java Collections Framework provides built-in data structures such as lists, sets, and maps, allowing developers to implement complex algorithms easily.
Use Cases for Java
Java is widely used in enterprise solutions, particularly for large-scale applications reliant on Java EE (Enterprise Edition). Many banks and financial institutions leverage Java's robust capabilities for mission-critical applications.
In the realm of web applications, frameworks like Spring and Hibernate streamline the development process, making Java a preferred choice for backend development. Additionally, Java is fundamental in mobile development—specifically for Android apps—given its versatility and performance across different devices.
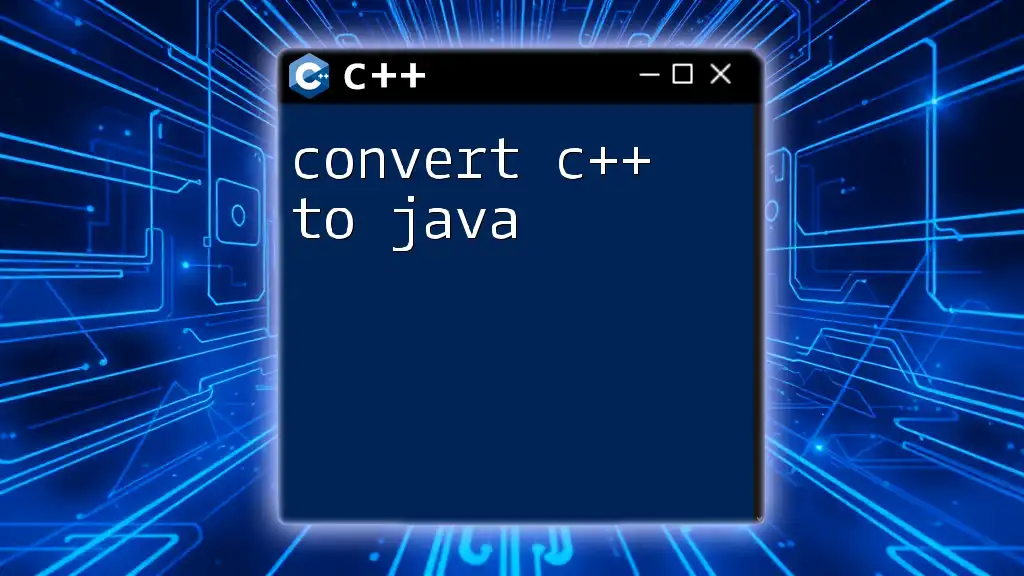
Comparing C++ and Java
Performance
When it comes to performance, C++ typically outperforms Java. While C++ is compiled directly into machine code, Java is compiled into bytecode, which the JVM interprets. This difference often leads to faster execution in C++ applications, an important consideration when developers choose how to write in C++ or Java.
Memory Management
In C++, developers are responsible for memory management, providing a means to optimize resource use but also introducing potential pitfalls such as memory leaks and pointer errors. Conversely, Java employs automatic garbage collection, significantly simplifying the programming experience by handling memory for developers but potentially introducing latency during runtime.
Syntax and Ease of Use
Java's syntax is often perceived as more readable and user-friendly compared to C++. For example, a simple "Hello, World!" program in both languages looks as follows:
// C++
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
// Java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
From this comparison, we can see that Java's structure is more streamlined for beginners, making it an appealing option for those just starting out.
Community and Support
Both C++ and Java possess substantial communities, but the nature of their support can differ significantly. Java has a rich ecosystem with numerous libraries and frameworks that are readily available, making it easier for developers to find pre-built solutions to common problems. On the other hand, C++ has a robust community focused on systems-level programming, providing extensive resources that facilitate low-level manipulation and performance tuning.
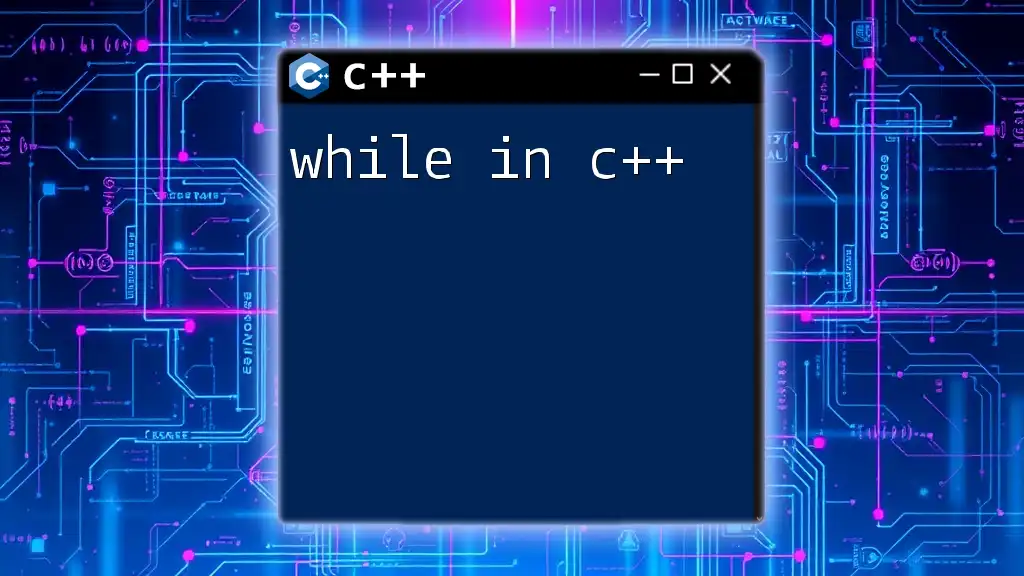
When to Use C++
Choosing to write in C++ is often best when developing systems-level applications, performance-critical programs, or exploring real-time graphics in game development. If you need fine control over system resources and memory management, C++ offers unmatched capabilities.
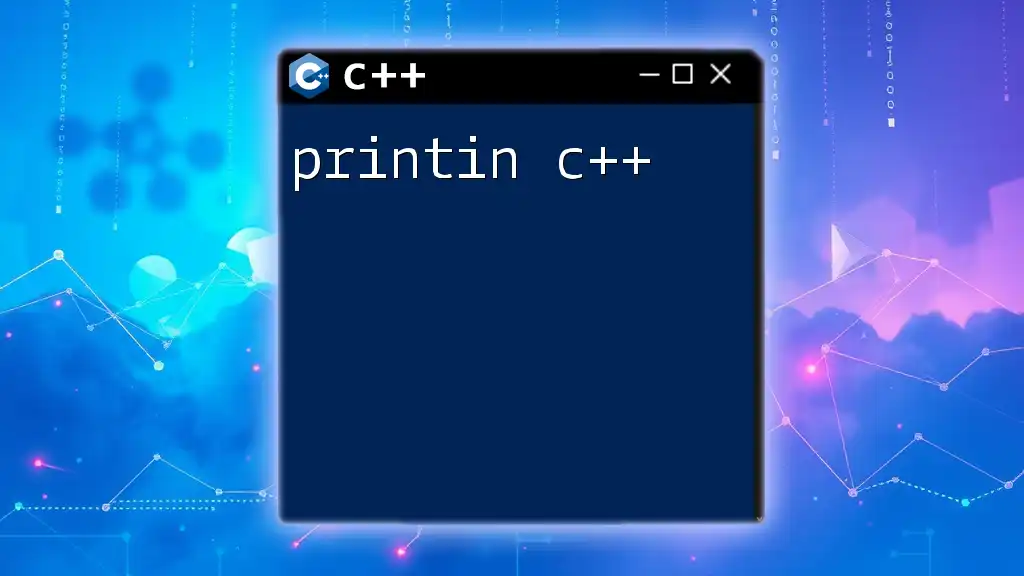
When to Use Java
Java is generally the language of choice for applications requiring platform independence or those being developed for the enterprise sector. When building large-scale applications, web environments, or Android mobile apps, Java's vast library support and built-in features significantly accelerate development.
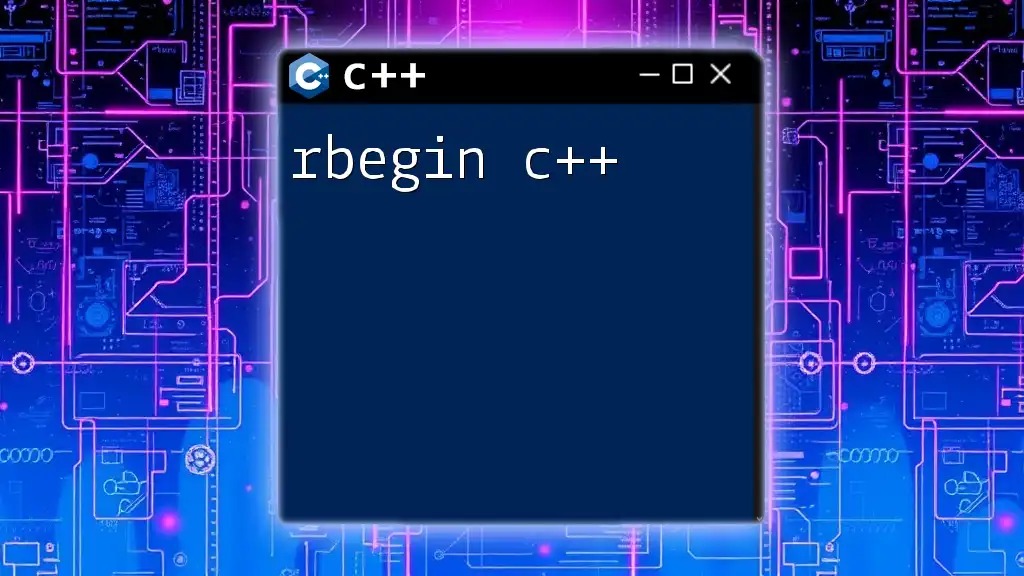
Conclusion
Ultimately, the question of whether to write in C++ or Java hinges on the specific needs of your project. Both languages have unique strengths and weaknesses that can influence their suitability for your applications. Consider the type of project, performance requirements, and development timelines when deciding.
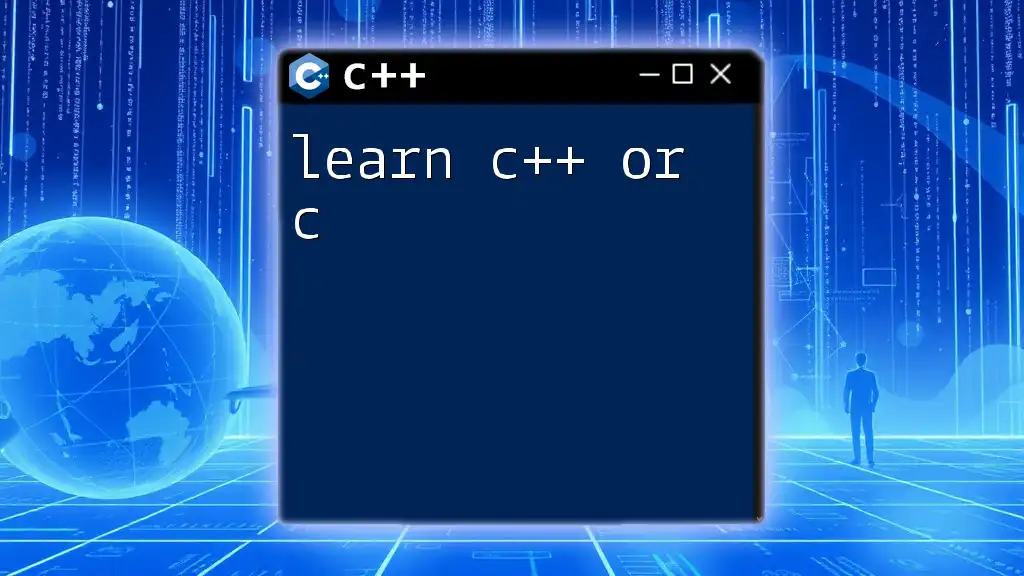
Additional Resources
For those eager to dive deeper into either language, numerous resources, tutorials, and coding platforms (like LeetCode and Codecademy) can provide valuable insights and practice opportunities. Taking the time to explore both C++ and Java will enhance your programming skills and empower you in the fast-evolving landscape of software development.