C++ can be translated into JavaScript for web development, allowing developers to leverage C++ logic while maintaining the flexibility of JavaScript; for example, a simple function that adds two numbers can be expressed in both languages as follows:
// C++ version
int add(int a, int b) {
return a + b;
}
// JavaScript version
function add(a, b) {
return a + b;
}
Understanding the Basics of C++
What is C++?
C++ is a powerful, high-performance programming language that supports procedural, object-oriented, and generic programming. Developed in the early 1980s, it is widely used for system/software development and game programming due to its advanced control over system resources and performance.
C++ Syntax and Structure
C++ syntax comprises several core elements such as data types, variables, control structures, and functions. Understanding these basics is crucial when translating C++ to JavaScript.
For instance, primitive data types include `int`, `float`, `char`, and `bool`. Declaring variables is straightforward:
int number = 5;
float pi = 3.14;
Control structures, including loops and conditionals, are vital in C++. A simple `if` statement looks like this:
if (number > 0) {
// code
}
Functions are defined with a return type followed by the function name and parameters:
int add(int a, int b) {
return a + b;
}

Understanding the Basics of JavaScript
What is JavaScript?
JavaScript is a dynamic, high-level programming language primarily utilized for enhancing web interfaces. It is an integral part of web development and enables interactive features such as form validation, animations, and asynchronous requests.
JavaScript Syntax and Structure
JavaScript also consists of essential elements, but with a focus on being flexible and forgiving in syntax. Variables can be defined using `let`, `const`, or `var`, allowing for varying scopes:
let number = 5;
const pi = 3.14;
Conditional statements mirror those in C++, for example:
if (number > 0) {
// code
}
Functions in JavaScript can be defined using the `function` keyword or arrow function syntax:
function add(a, b) {
return a + b;
}

Key Differences Between C++ and JavaScript
Language Paradigms
C++ is primarily object-oriented, emphasizing data encapsulation and inheritance. JavaScript, while supporting object-oriented programming, is often categorized as a prototype-based language, relying on prototype chains for inheritance.
Memory Management
C++ requires manual memory management through allocating and deallocating memory using `new` and `delete`. In contrast, JavaScript employs automatic garbage collection, allowing developers to focus less on memory management tasks.
Execution Environment
C++ is a compiled language; code is translated into machine language before execution. JavaScript, however, is an interpreted language, executed at runtime by browsers, which adds to its flexibility and cross-platform compatibility.
Typing Systems
C++ uses static typing, where type checking is performed at compile time, making it strict regarding data types. JavaScript embraces dynamic typing, wherein types are determined at runtime, allowing variables to hold different types of values throughout their lifecycle.
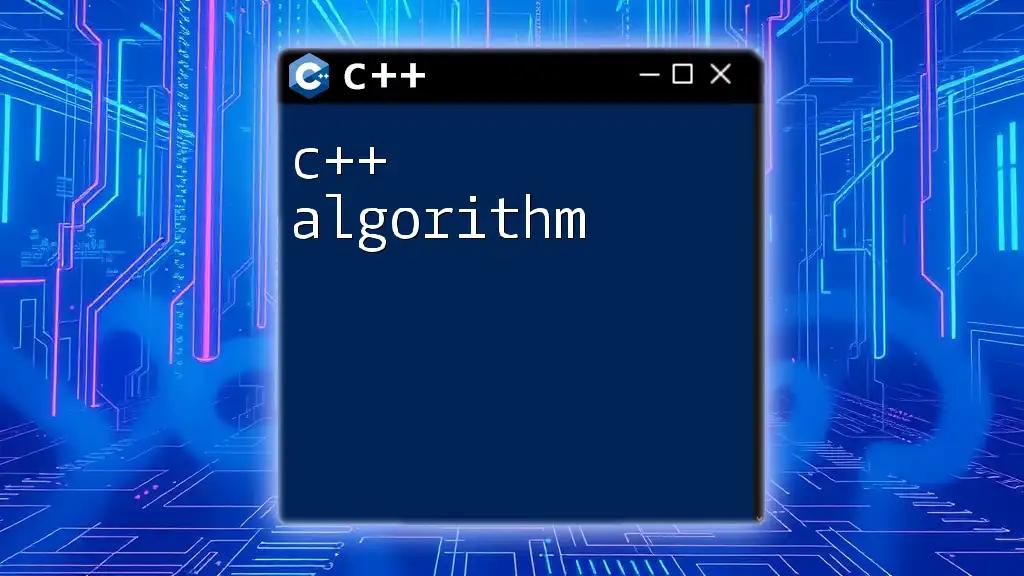
Translating C++ Concepts to JavaScript
Data Types and Variables
The translation of C++ data types to JavaScript is essential. While both languages support basic types such as `int` and `float`, JavaScript consolidates several types into a single `Number` type:
int num = 5; // C++
let num = 5; // JavaScript
Control Structures
Conditional Statements
In both C++ and JavaScript, `if` statements function similarly:
if (x > 10) {
// code
}
if (x > 10) {
// code
}
Loops
Looping constructs like `for` loops are analogous:
for (int i = 0; i < 10; i++) {
// code
}
for (let i = 0; i < 10; i++) {
// code
}

Converting C++ Functions to JavaScript
Defining Functions
Functions can easily be converted. In C++, a function is defined with a return type followed by the name and parameters:
int add(int a, int b) {
return a + b;
}
In JavaScript, the syntax is more flexible:
function add(a, b) {
return a + b;
}
Returning Values
Both languages allow for value returning. Understanding the return keyword is crucial, and both implementations share this feature.
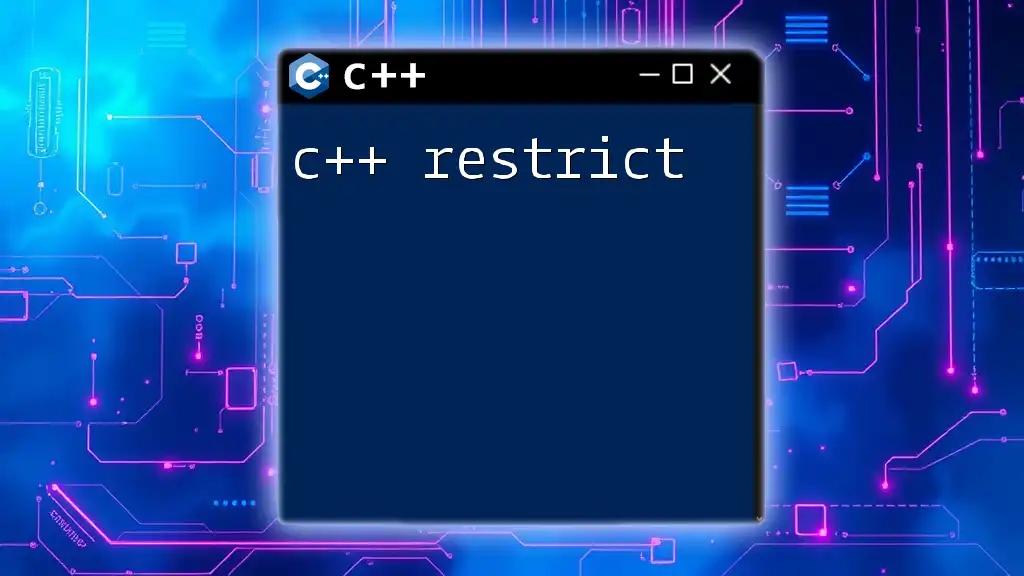
Object-Oriented Programming: C++ vs. JavaScript
Classes and Objects
C++ establishes classes with the `class` keyword, encapsulating data and functions:
class Dog {
public:
void bark() { }
};
In JavaScript, the class syntax introduces similar functionality:
class Dog {
bark() { }
};
Inheritance
C++ supports single and multiple inheritance. For instance, demonstrating single inheritance:
class Animal {};
class Dog : public Animal {};
JavaScript achieves inheritance through prototypes:
function Animal() {}
function Dog() {}
Dog.prototype = Object.create(Animal.prototype);

Common Libraries and Frameworks for C++ and JavaScript
C++ Libraries
C++ offers an array of robust libraries, such as the Standard Template Library (STL), which provides data structures and algorithms, and Boost, extending C++ capabilities across numerous applications.
JavaScript Frameworks
JavaScript thrives on a multitude of frameworks, such as React for UI development and Node.js for server-side programming, enhancing functionality and speed in web applications.
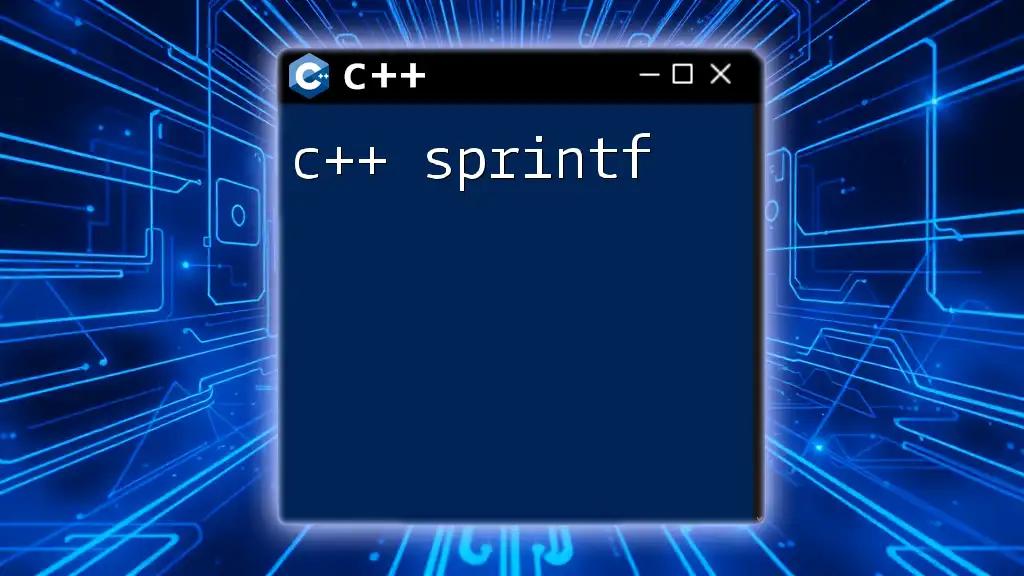
Best Practices for Transitioning from C++ to JavaScript
Planning Your Workflow
When transitioning from C++ to JavaScript, planning your workflow is essential. Understand the differences in execution environments and adjust your programming paradigm accordingly. Set up your development environment, install relevant tools, and familiarize yourself with browser development consoles.
Debugging JavaScript Code
Debugging tools in JavaScript, such as browser dev tools, are invaluable. Utilize console logging, breakpoints, and step-through debugging to identify and resolve issues efficiently.
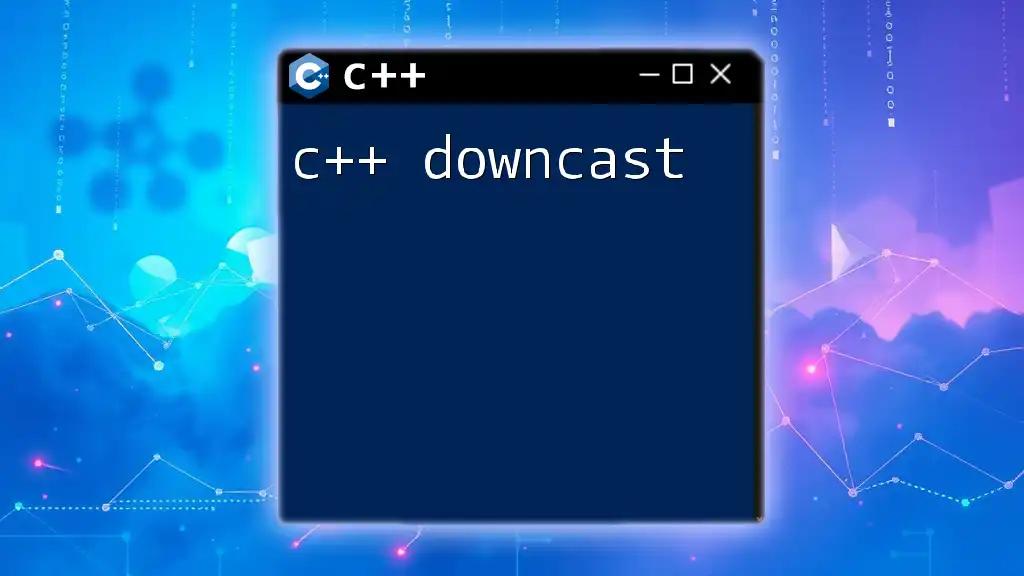
Conclusion
By exploring the differences and similarities between C++ and JavaScript, you are better equipped to transition between these two powerful languages. Embrace both languages to enhance your programming toolkit.
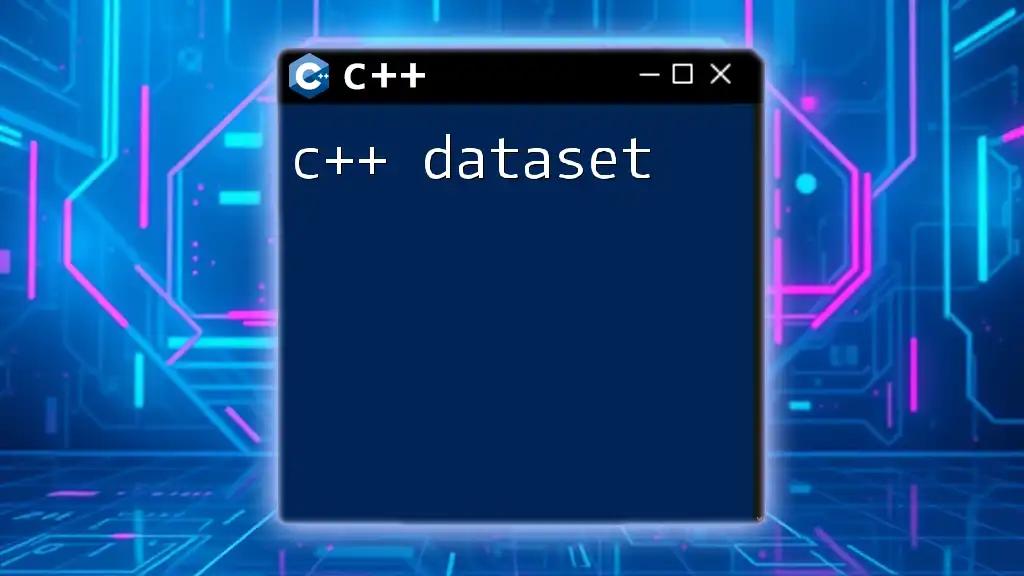
Further Resources
Books and Tutorials
Consider diving into comprehensive books or tutorials specifically focusing on C++ and JavaScript to deepen your understanding.
Online Courses
Enroll in online courses on platforms like Coursera, Udemy, or edX to gain hands-on experience and build projects that utilize both languages.

Call to Action
If you found this guide helpful, consider signing up for our courses that specialize in mastering the transition from C++ to JavaScript. Share your thoughts or experiences with us in the comments below!