JavaScript and C++ share some similarities in syntax and programming concepts, but they differ significantly in their design paradigms, execution environments, and type systems.
Here’s a simple example showcasing variable declaration and a function in both languages:
// C++ code
#include <iostream>
using namespace std;
void greet() {
cout << "Hello, World!" << endl;
}
int main() {
int number = 5;
greet();
return 0;
}
// JavaScript code
function greet() {
console.log("Hello, World!");
}
let number = 5;
greet();
Understanding the Basics
C++ Basics
What is C++?
C++ is a high-performance, general-purpose programming language that supports object-oriented programming principles. It is compiled and statically typed, which means variables need to be declared with their data types before being used. This can lead to more optimized performance but requires developers to manage data types carefully.
Key features of C++ include:
- Object-Oriented Programming (OOP): Facilitates code reusability and organization through classes and objects.
- General-Purpose: Suitable for systems programming, game development, and applications requiring high performance.
Core Concepts
Understanding core concepts of C++ is crucial as it lays the foundation for advanced functionalities:
- Classes and Objects: The building blocks of OOP, enabling encapsulation.
- Inheritance and Polymorphism: Allowing for interface-driven design and code extensibility.
JavaScript Basics
What is JavaScript?
JavaScript is a versatile, high-level programming language primarily used for web development. Unlike C++, it is interpreted at runtime and is dynamically typed, meaning that variables can change types during execution. This feature contributes to its flexibility.
Key features of JavaScript include:
- Event-Driven: Ideal for creating interactive web pages.
- Functional Programming: Supports first-class functions, enabling functional programming paradigms alongside OOP.
Core Concepts
JavaScript employs different concepts focused on its unique environment:
- Objects and Prototypes: Unlike classical inheritance in C++, JavaScript uses prototypes for inheritance, improving performance and memory efficiency.
- Asynchronous Programming: JavaScript’s ability to manage asynchronous operations through callbacks, promises, and async/await enhances its capability for web applications.
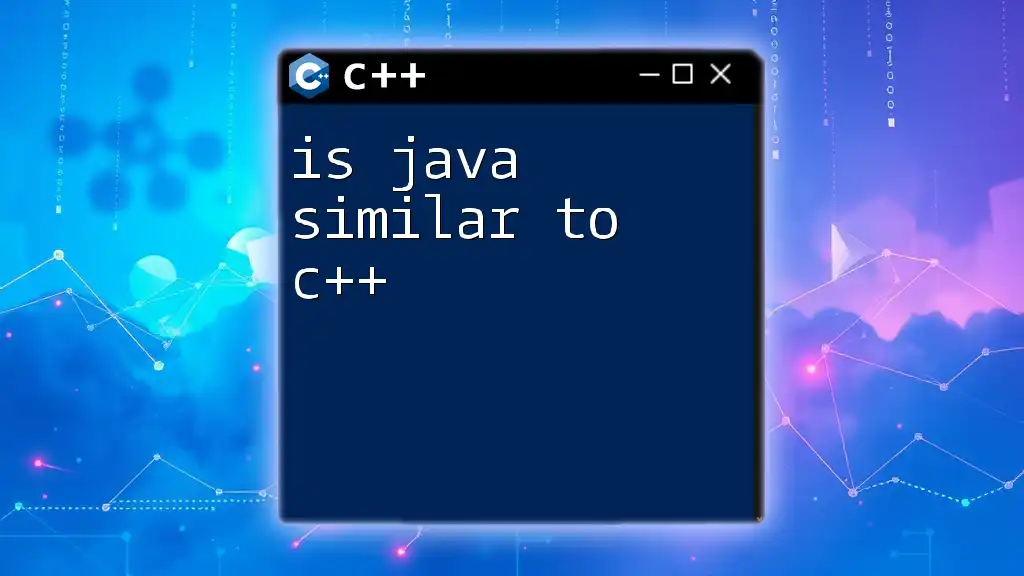
Syntax Comparison: JavaScript vs C++
Variable Declarations
C++ requires explicit data type declaration for variables, enhancing performance:
int number = 5;
In contrast, JavaScript allows for more flexibility with variable declarations using `let`, `const`, or `var`:
let number = 5;
Data Types
C++ features a range of data types including both primitive types, such as `int`, `double`, and `char`, and user-defined types like structs and classes. JavaScript, however, has a more simplified set of data types that include primitive types (number, string, boolean, null, undefined, symbol) and complex types (objects and arrays).
This distinction highlights the strongly typed nature of C++ versus the dynamically typed nature of JavaScript, which can be both advantageous and a potential pitfall for inexperienced developers.
Control Structures
Control flow statements such as if-else structures are abundant in both languages, but their syntax varies slightly.
C++ Example:
if (number > 5) {
std::cout << "Greater than 5";
}
JavaScript Example:
if (number > 5) {
console.log("Greater than 5");
}
Loops also show a similar structure but with different syntax. Both C++ and JavaScript utilize `for`, `while`, and `do-while` loops, although their implementation details can differ.
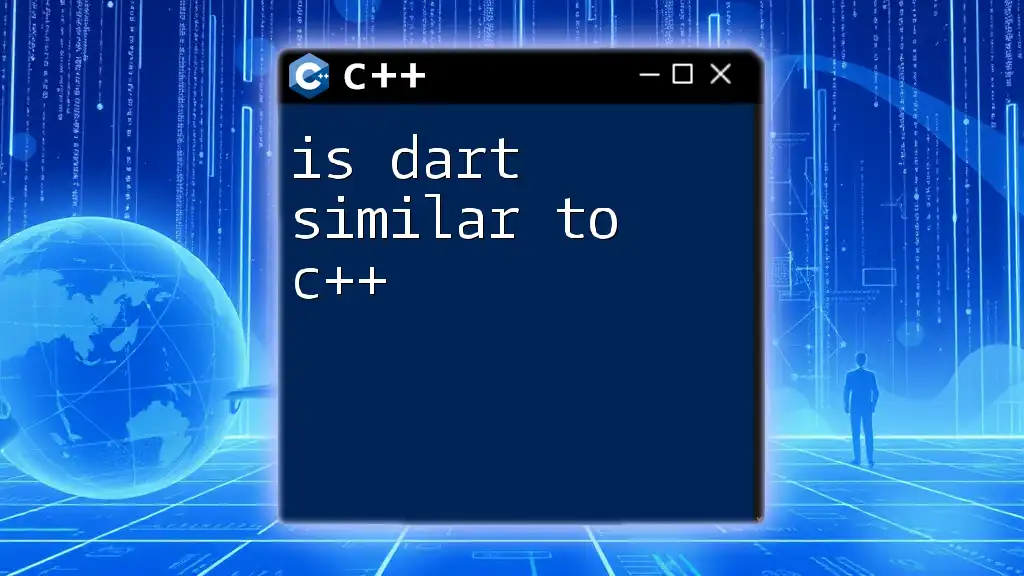
Object-Oriented Programming Differences
Classes and Instances
C++ class structure requires explicit visibility attributes to encapsulate functionality:
class Dog {
public:
void bark() {
std::cout << "Woof!";
}
};
Conversely, JavaScript uses a more streamlined approach to class definitions, which often emphasizes brevity:
class Dog {
bark() {
console.log("Woof!");
}
}
Inheritance
C++ supports inheritance explicitly through keywords like `public`, `protected`, and `private`:
class Animal {};
class Dog : public Animal {};
In JavaScript, inheritance can be established using the `extends` keyword, emphasizing its prototype-based inheritance model:
class Animal {}
class Dog extends Animal {}
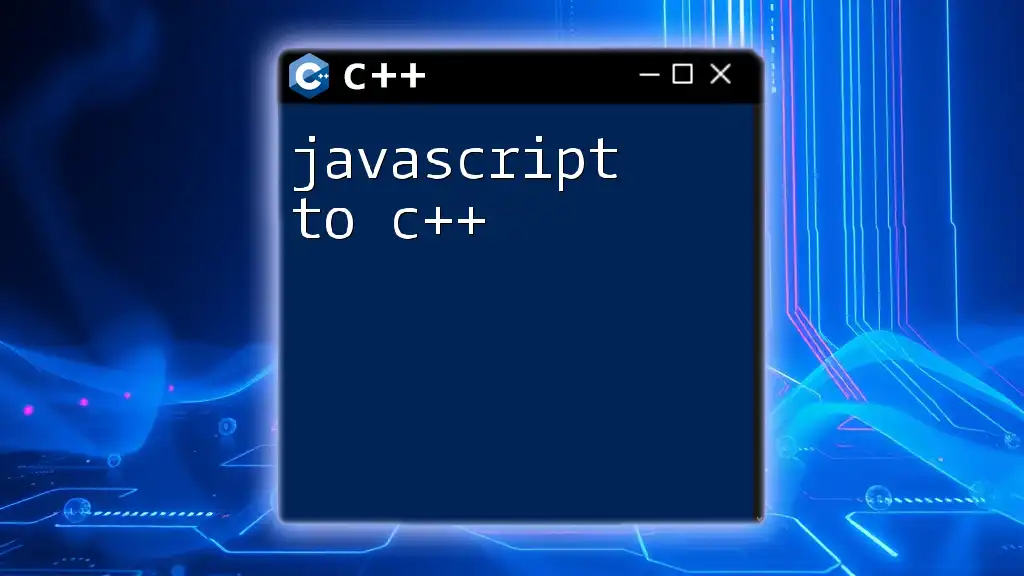
Memory Management
C++ Memory Management
One of C++'s defining features is manual memory management. Programmers are responsible for allocating and deallocating memory, resulting in high efficiency but potential risks such as memory leaks or segmentation faults. C++ employs pointers and references, and dynamic memory allocation can be achieved with `new` and deallocation with `delete`.
JavaScript Memory Management
JavaScript simplifies memory management through automatic garbage collection. This feature abstracts the complexities of managing memory, allowing developers to focus more on application logic rather than memory allocation. However, developers must still be aware of how closures work in JavaScript, as they can create unintentional references that the garbage collector cannot recycle.
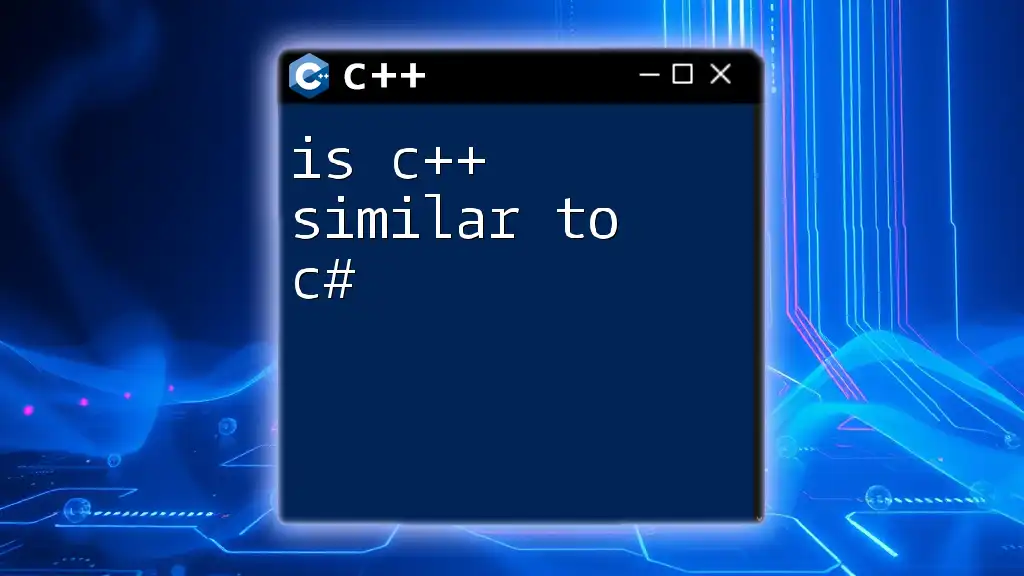
Performance Considerations
Compilation vs Interpretation
C++ is a compiled language that transforms source code into machine code before execution, resulting in high execution speed. This compilation can lead to optimizations that make C++ suitable for resource-intensive applications.
In contrast, JavaScript is interpreted at runtime, which may lead to slower execution compared to compiled languages. However, modern JavaScript engines employ Just-In-Time (JIT) compilation techniques to improve performance.
Use Cases and Performance
C++ shines in applications requiring intensive computation, such as game development, system-level programming, and real-time simulations. Meanwhile, JavaScript excels in scenarios such as web applications, server-side scripting with Node.js, and any event-driven applications where user experience is paramount.
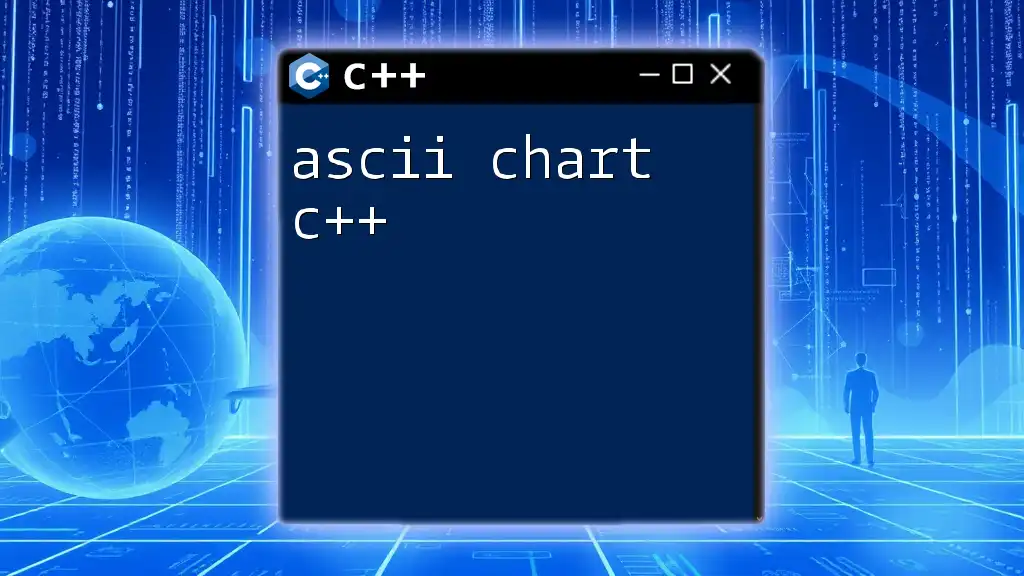
Community and Ecosystem
Libraries and Frameworks
Both languages boast rich ecosystems with numerous libraries and frameworks:
C++ Libraries include the Standard Template Library (STL) for algorithms and data structures, and frameworks like Qt for GUI applications.
JavaScript Libraries encompass a plethora of options, including jQuery for DOM manipulation, React for building user interfaces, and Node.js for server-side programming.
Common Use Cases
C++ remains the choice for:
- Game Development: Due to performance needs.
- Systems Programming: Harnessing direct access to hardware.
JavaScript dominates in:
- Web Development: Providing interactive experiences.
- Server-Side Development: Using Node.js to build scalable network applications.
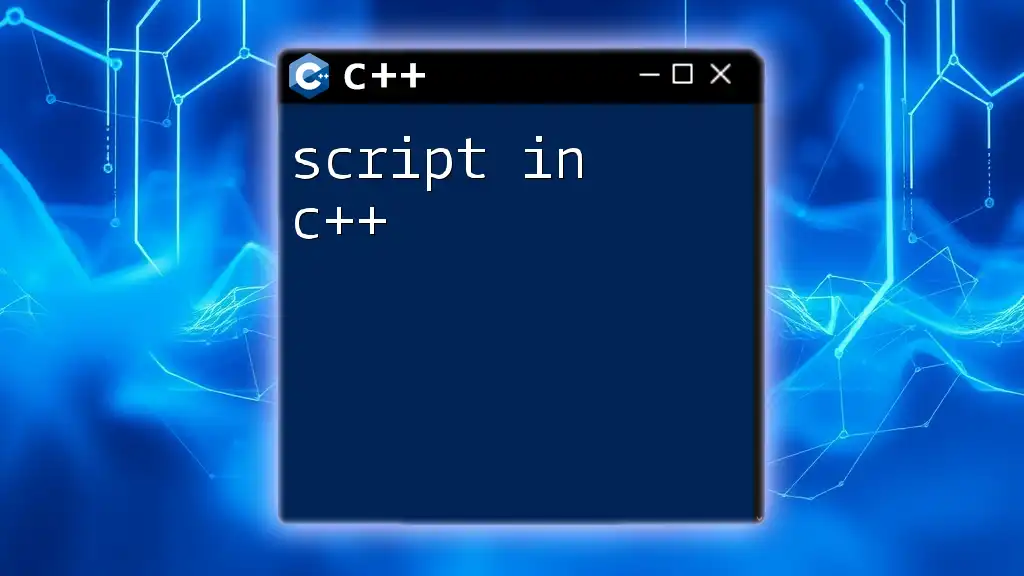
Conclusion
When evaluating is JavaScript similar to C++, it is clear that while both languages can achieve similar goals, they differ significantly in their approach, syntax, and use cases. C++ offers more control and performance, making it ideal for high-efficiency applications. Meanwhile, JavaScript provides ease of use and flexibility, particularly in web environments.
Choosing the right language ultimately depends on the project's requirements, the developer's proficiency with each language, and the specific use case at hand. Understanding these differences equips developers with the necessary insights to make informed decisions in their programming journey.
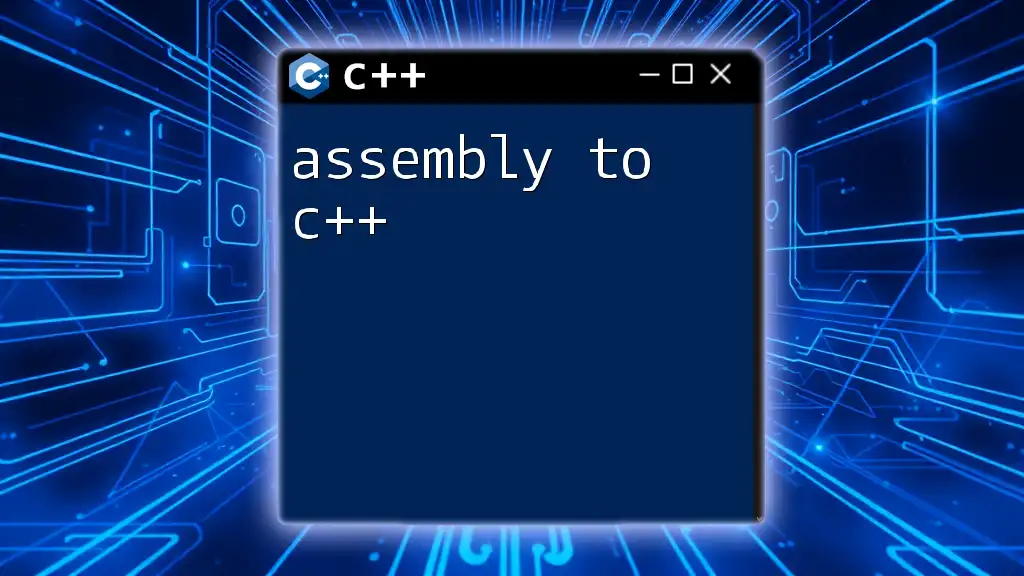
FAQs about C++ and JavaScript
-
Is JavaScript easier to learn than C++?
JavaScript is generally considered more accessible, especially for beginners, due to its forgiving syntax and dynamic nature. -
Can JavaScript be used for system programming?
While JavaScript can perform certain system-level tasks (especially using Node.js), it is not designed for low-level hardware interactions typical in systems programming, where C++ excels.