Dart and C++ share some syntactical similarities, particularly in their use of classes and basic control structures, but Dart is primarily designed for web and mobile development with a focus on ease of use and garbage collection.
Here's a simple comparison of class declaration in both languages:
// C++ Class Example
class Animal {
public:
void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
// Dart Class Example
class Animal {
void speak() {
print("Animal speaks");
}
}
Understanding the Basics
What is C++?
C++ is a powerful, high-performance programming language developed by Bjarne Stroustrup in the early 1980s as an enhancement of the C programming language. It incorporates object-oriented features, strong type checking, and a robust standard library, making it suitable for a variety of applications, from system software to game development.
Key features of C++ include:
- Object-Oriented Programming (OOP): Support for classes and objects, enabling code reusability and modular design.
- Low-Level Manipulation: Ability to manipulate hardware resources and memory directly.
- Efficiency: C++ is known for its performance optimization capabilities.
Here's a simple C++ program to illustrate its syntax:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
What is Dart?
Dart is a modern programming language introduced by Google. Initially developed for web applications, Dart has evolved to become a key player in building cross-platform applications, especially with frameworks like Flutter for mobile app development.
Key features of Dart include:
- Just-in-Time (JIT) and Ahead-of-Time (AOT) compilation: This leads to fast development cycles and optimized app performance.
- Strongly Typed: Dart enforces type safety, reducing runtime errors and improving code quality.
- Asynchronous Programming: Built-in support for asynchronous operations, making it excellent for applications that require non-blocking I/O.
Here’s a simple Dart program that demonstrates its syntax:
void main() {
print('Hello, World!');
}
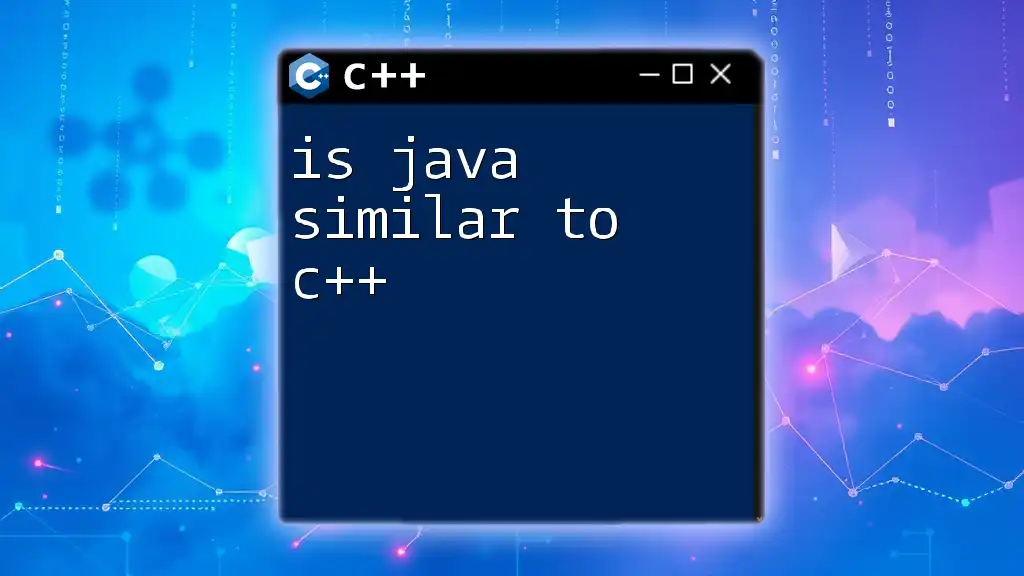
Language Paradigms
Object-Oriented Programming
Both C++ and Dart embrace the principles of Object-Oriented Programming (OOP), though they implement them differently.
In C++, OOP allows for the creation of classes and inheritance. Here’s a common structure:
class Animal {
public:
void speak() {
cout << "Animal speaks" << endl;
}
};
class Dog : public Animal {
public:
void speak() {
cout << "Woof" << endl;
}
};
In Dart, the OOP structure is similar but with some syntactical differences:
class Animal {
void speak() {
print('Animal speaks');
}
}
class Dog extends Animal {
void speak() {
print('Woof');
}
}
Functional Programming
Functional programming concepts are present in both languages, but their support and usage differ.
In C++, functions can be treated as first-class citizens, meaning they can be passed as parameters. However, the syntax may feel more verbose compared to Dart. Dart, on the other hand, makes function handling very intuitive with its first-class function support, allowing functions to be assigned to variables easily and enabling cleaner code for callback functions.
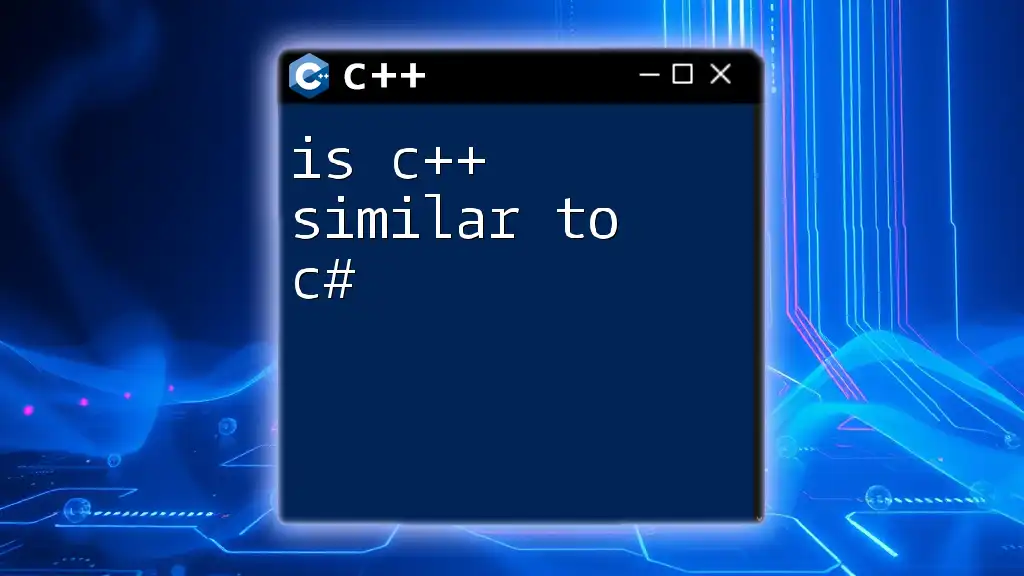
Syntax Comparison
Variable Declaration
C++ requires specific type declarations:
int x = 5;
Dart simplifies this with the `var` keyword, allowing for easier variable declaration regardless of the type:
var x = 5;
Control Structures
Control structures like if-else statements work similarly in both languages:
C++ if-else example:
if (x > 0) {
cout << "Positive" << endl;
}
Dart if-else example:
if (x > 0) {
print('Positive');
}
Looping constructs also have similarities. For instance, here’s a for loop demonstration:
C++ for loop:
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
Dart for loop:
for (var i = 0; i < 5; i++) {
print(i);
}
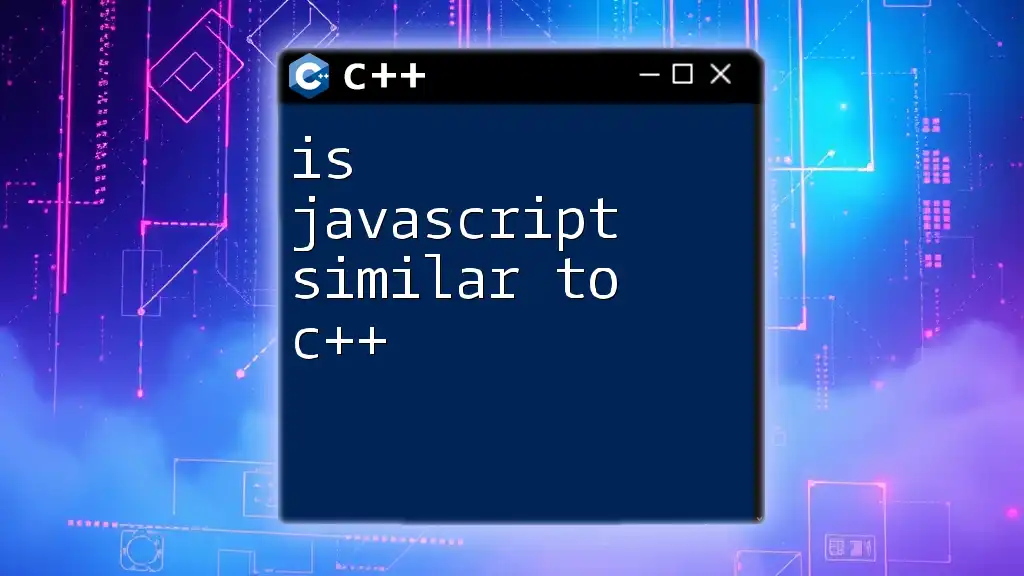
Error Handling
Exception Handling in C++
C++ uses try-catch blocks for error handling, allowing for graceful recovery from runtime errors:
try {
throw 20;
} catch (int e) {
cout << "Error: " << e << endl;
}
Exception Handling in Dart
Dart utilizes a similar approach, simplifying error handling while maintaining clarity:
try {
throw 20;
} catch (e) {
print('Error: $e');
}
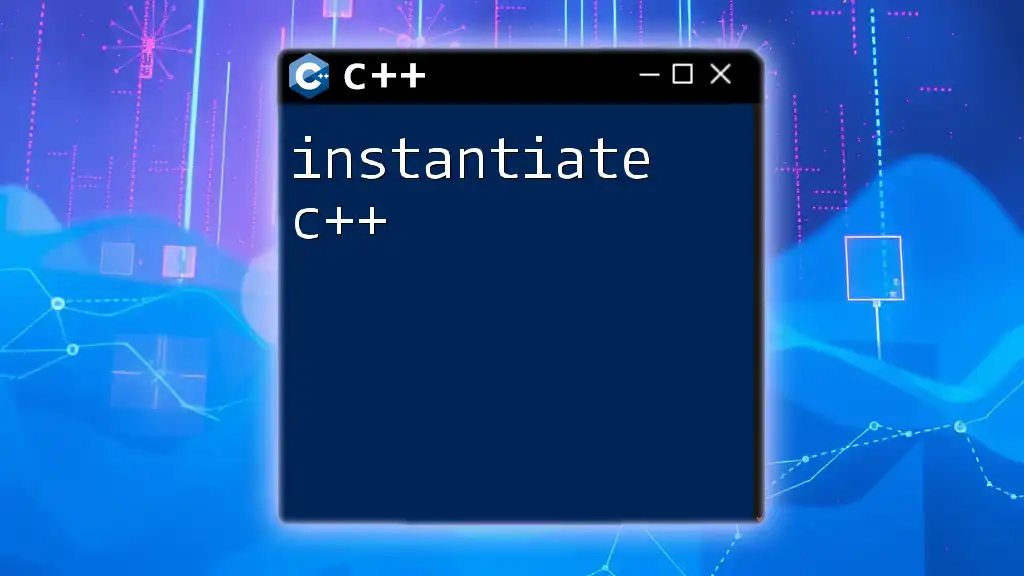
Libraries and Package Management
Standard Libraries in C++
C++ has extensive standard libraries that offer a range of functionalities. For instance, the `<vector>` library is commonly used for dynamic array manipulation:
#include <vector>
vector<int> numbers = {1, 2, 3};
Packages in Dart
Dart has an effective package management system with the `pub` tool, making it easy to use third-party libraries. Importing a package looks like this:
import 'package:http/http.dart' as http;
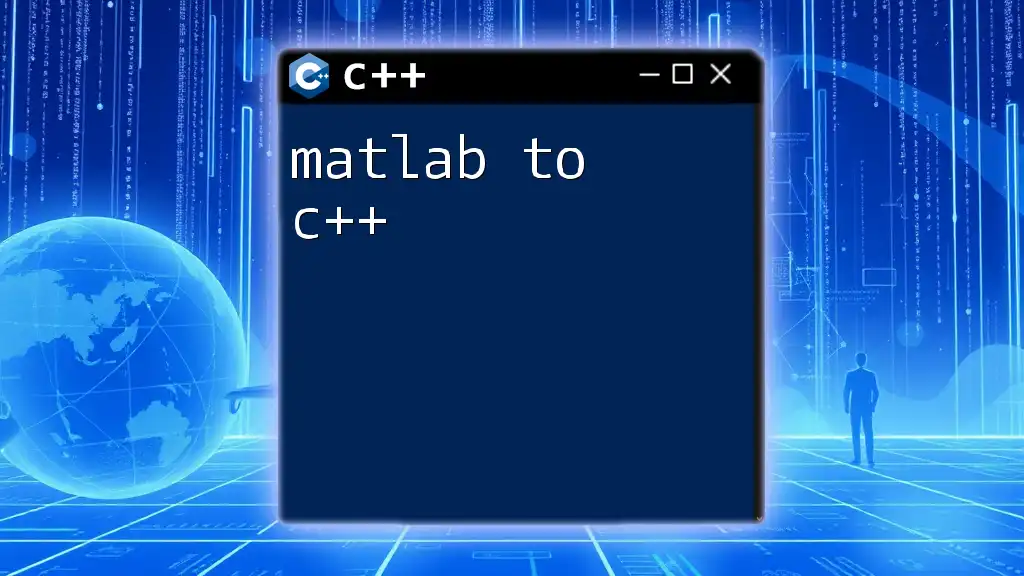
Performance Considerations
C++ Performance
C++ is known for its high performance due to manual memory management and direct access to hardware resources. This control allows developers to optimize their applications for speed, crucial for resource-intensive applications like gaming or real-time systems.
Dart Performance
Dart, with its garbage collection and JIT/AOT compilation, provides a balance between ease of use and performance. While it may not match C++ in raw performance for every scenario, Dart’s efficient runtime and development speed make it a solid choice for applications requiring rapid iteration.
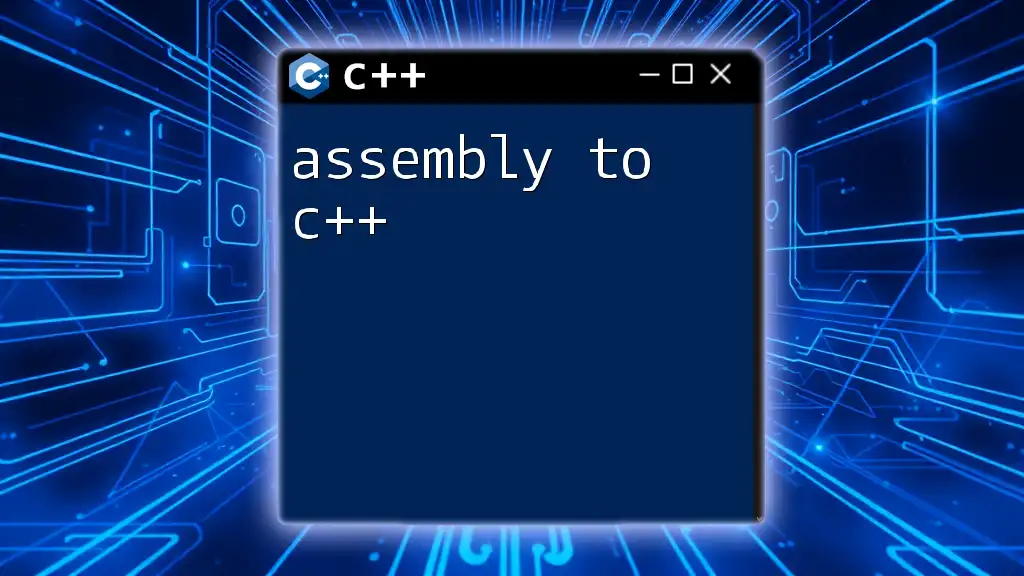
Use Cases and Applications
C++ Applications
C++ is extensively used in domains such as:
- Game Development: Many game engines are built on C++ due to its performance capabilities.
- System Software: Operating systems, drivers, and firmware often utilize C++ for efficiency.
Dart Applications
Dart has garnered attention primarily in mobile and web development:
- Mobile Apps: With Flutter, Dart enables the creation of beautiful, performant applications across platforms.
- Web Applications: Dart is also used for browser-based applications, offering a modern approach to web programming.
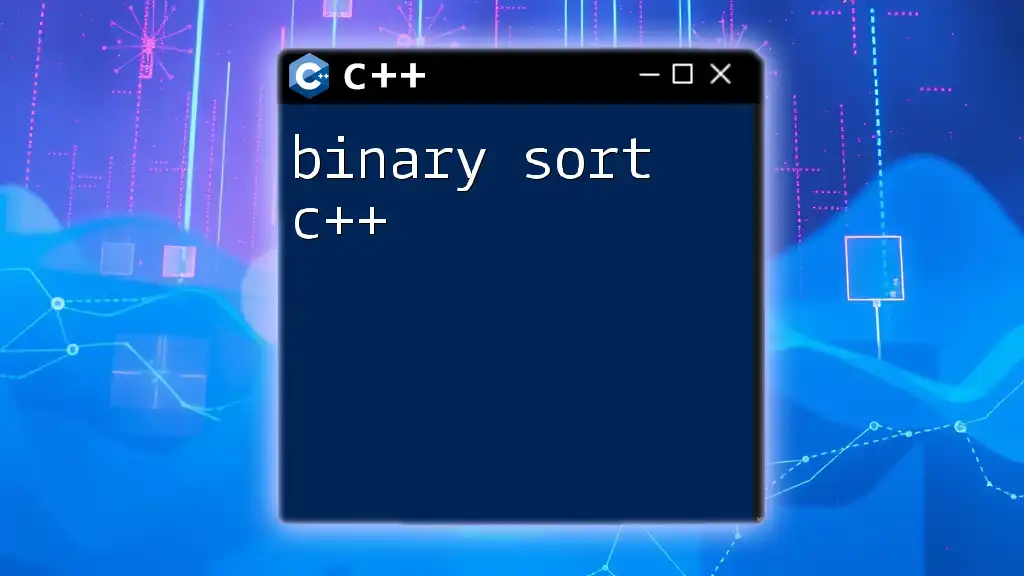
Conclusion
When exploring the question, "is Dart similar to C++," it's evident that while both languages share fundamental programming principles such as OOP and strong typing, they cater to different needs and scenarios. C++ excels in performance-critical applications, whereas Dart shines in modern application development thanks to its simplicity and built-in features. Understanding their similarities and differences can assist developers in choosing the right tool for their projects.
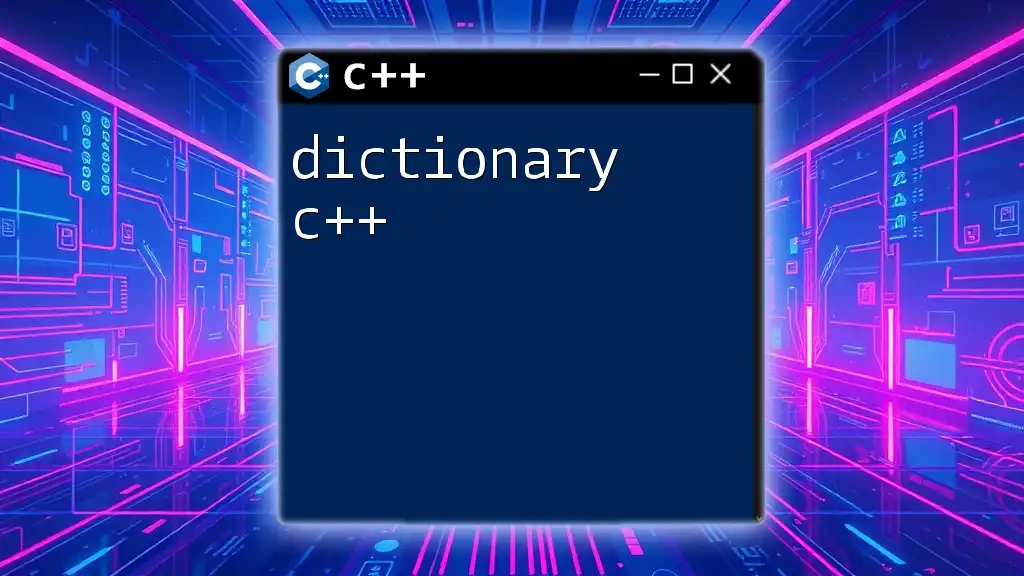
Additional Resources
For further reading, developers are encouraged to explore the official documentation of both C++ and Dart. Tutorials and courses are available to deepen understanding and enhance skills in either language.
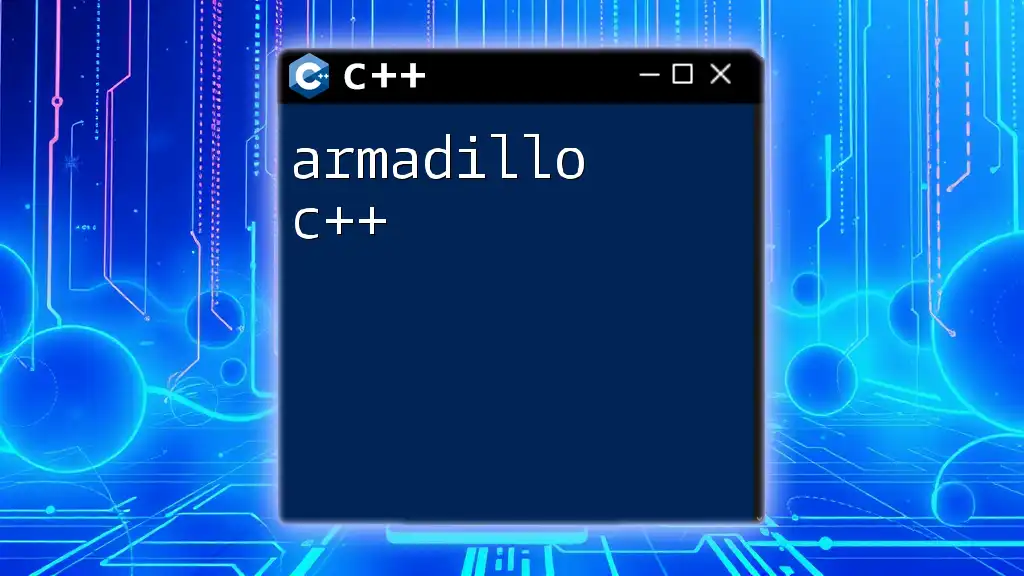
Call to Action
As you venture into the world of C++ and Dart, we invite you to experiment with both languages, share your thoughts, and ask any questions you may have about their capabilities and application. Each language has its unique strengths waiting for your exploration!