Sierra Chart is a powerful trading platform that allows developers to create custom studies and functionality using C++ commands to enhance their trading strategies.
Here's a simple example of a C++ script to create a custom study in Sierra Chart:
// Example of a custom study in Sierra Chart
#include "sierrachart.h"
SCDLLName("MyCustomStudy")
// Custom study function
SCSFExport void scsf_MyCustomStudy(SCStudyInterfaceRef sc) {
if (sc.SetDefaults) {
sc.GraphName = "My Custom Study";
sc.StudyDescription = "This is a simple custom study example.";
sc.AutoLoop = 1; // Enable AutoLoop
return;
}
// Main calculation (example: a simple moving average)
sc.Data[0][sc.Index] = (sc.Close[sc.Index] + sc.Close[sc.Index - 1]) / 2; // Average of last two closes
}
What is Sierra Chart?
Sierra Chart is a powerful and flexible trading platform designed primarily for professional traders. It offers advanced charting capabilities, real-time data feeds, and robust tools for market analysis. An essential feature of Sierra Chart is its ability to accept custom programming through C++, making it a favorite among algorithmic and quantitative traders. By leveraging the flexibility of C++, traders can develop personalized tools, indicators, and automated trading strategies, enabling them to gain a competitive edge in the financial markets.

Why Use C++ with Sierra Chart?
Utilizing C++ within Sierra Chart opens up a myriad of possibilities for customization. One primary benefit is performance optimization; C++ is known for its speed and efficiency, which is crucial for trading applications that require real-time processing. Additionally, the versatility of C++ allows traders to create unique trading solutions tailored to their specific needs.
Moreover, with C++, you can tap into Sierra Chart's comprehensive API, enabling deeper integrations and beyond standard functionalities. This level of customization can lead to significant improvements in your trading strategies and overall trading experience.

Getting Started with Sierra Chart C++
Setting Up Your Environment
Before diving into Sierra Chart C++, ensure your environment is set up correctly. You’ll need the Sierra Chart platform and a C++ development environment. The recommended tools often include Visual Studio or Code::Blocks, along with the appropriate compilers to build your projects.
Once Sierra Chart is installed, configure your C++ development tools to connect with Sierra Chart's API. This connection involves setting paths for your project's include directories and linking appropriate libraries. This setup is crucial, as it lays the foundation for successful C++ development within the Sierra Chart environment.
Understanding the Sierra Chart API
The Sierra Chart API provides the structure and functions needed to create custom indicators and studies. Understanding how the API works is critical for efficient development. The API consists of various classes, methods, and data structures you can leverage in your C++ scripts.
A key to effective usage is accessing the comprehensive documentation available on the Sierra Chart website. It covers all aspects of the API, including sample code snippets which can significantly accelerate learning and implementation.
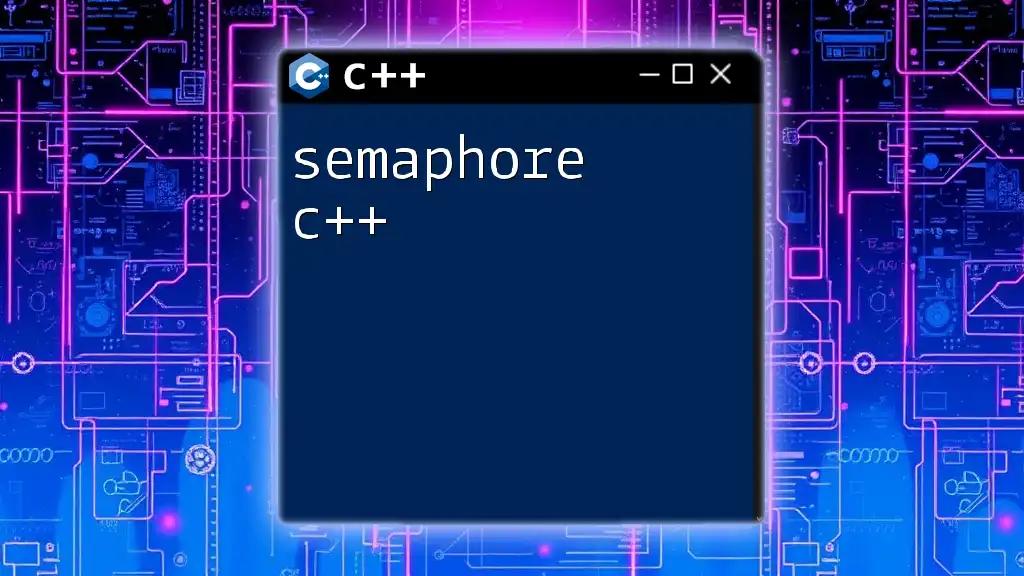
Basic Concepts in Sierra Chart C++
Overview of Custom Studies
Custom Studies in Sierra Chart refer to user-defined indicators created using the platform's C++ programming capabilities. These studies allow traders to implement complex trading strategies and technical analysis that may not be available out of the box.
The importance of Custom Studies cannot be overstated, as they provide the flexibility to adapt analysis and strategy to individual trading styles.
Basic Structure of a Custom Study
To create a custom study, you need to understand the basic structure and syntax. Here’s a simple template you can start with:
// Example custom study template
class MyCustomStudy : public SCStudyInterface
{
public:
void GetStudyName(SCStudyInterfaceRef& sc)
{
sc.SetStudyName("My Custom Study");
}
// Add further functionality here
};
In this template, you declare a class inheriting from `SCStudyInterface`, which is essential for any custom study. The `GetStudyName` method is used to provide a name for the study.

Key C++ Commands in Sierra Chart
Working with Price Data
Accessing price data is fundamental in Sierra Chart C++. You can easily retrieve various price points using the `SCData` structure. For instance, getting the current closing price is straightforward:
double ClosePrice = sc.Close[sc.ArraySize - 1];
The `ArraySize` parameter represents the number of data elements available, and by indexing the last element, you can access the most recent closing price.
Custom Indicator Development
Creating indicators with C++ in Sierra Chart requires knowledge of how to manipulate price data and perform calculations. Here's how to develop a basic moving average indicator as an example.
Moving Averages Example
A simple moving average (SMA) indicator calculates the average of a selected number of closing prices over a defined period. Here's a straightforward implementation:
void CalculateSMA(SCStudyInterfaceRef& sc)
{
int period = sc.Input[0]; // Assuming the input period is set
for (int i = period - 1; i < sc.ArraySize; i++)
{
double sum = 0;
for (int j = 0; j < period; j++)
{
sum += sc.Close[i - j];
}
sc.Subgraph[0][i] = sum / period; // Store the calculated SMA
}
}
This code calculates the SMA by summing up the closing prices of the last `N` periods and dividing them by `N`, where `N` is the period defined by the user.
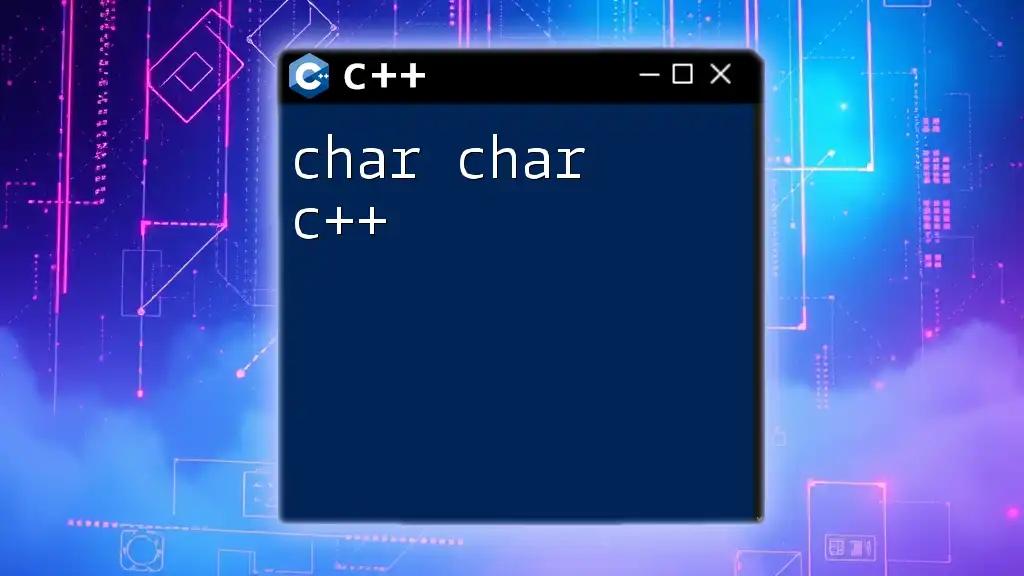
Advanced Topics
Integrating External Libraries
One major benefit of using C++ is the ability to integrate external libraries. This expands your capabilities significantly, allowing you to implement advanced mathematical functions, data handling, or even machine learning algorithms that can enhance your trading strategies.
When using libraries, ensure you have the necessary licenses and dependencies set up properly in your project settings.
Event Handling in Sierra Chart
Understanding events in Sierra Chart is crucial when developing interactive studies. For instance, you may want to handle trade events to trigger specific actions based on market activities. Below is how you can set up a basic event handler:
void OnTradeEvent(SCStudyInterfaceRef& sc)
{
if (sc.IsNewTradeAvailable())
{
// Handle trade actions here
double tradePrice = sc.LastTradePrice;
// Additional Logic
}
}
This method checks whether a new trade is available and executes the defined logic based on the most recently recorded trade price.
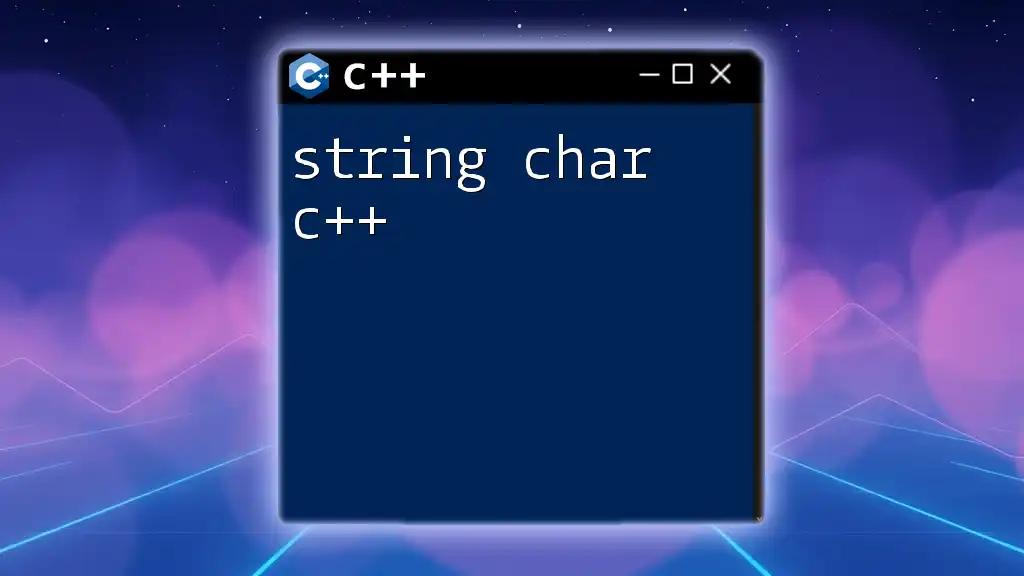
Debugging and Testing Your Code
Common Debugging Techniques
Debugging is an essential part of development. When programming in C++, using tools such as breakpoints, logging, and assertions can assist in identifying issues. Always ensure that you test your code after making changes to catch any errors early.
Writing Unit Tests for Custom Studies
Testing is vital for ensuring that your custom studies work as intended. Unit tests can help verify that individual components of your code function correctly. Here’s an example of a simple unit test for the moving average calculation:
void TestMovingAverage()
{
SCStudyInterfaceRef sc;
// Set up test data
// Call CalculateSMA
// Validate results
}
This unit test structure outlines how to validate the accuracy of your calculations. Ensure that your tests cover various scenarios to guarantee robustness.

Best Practices for Sierra Chart C++
Coding Standards and Conventions
Adhering to consistent coding standards is vital when working with C++. This includes using meaningful variable and function names, adhering to proper indentation, and ensuring comments are clear and concise. Well-structured code is easier to read, maintain, and debug.
Performance Optimization Techniques
Optimizing performance is crucial, especially for trading applications. Keep an eye out for inefficient loops, excessive memory allocation, and unnecessary calculations. Use profiling tools to identify bottlenecks in your code and refactor them for better performance. An optimized algorithm can make all the difference during volatile market conditions.
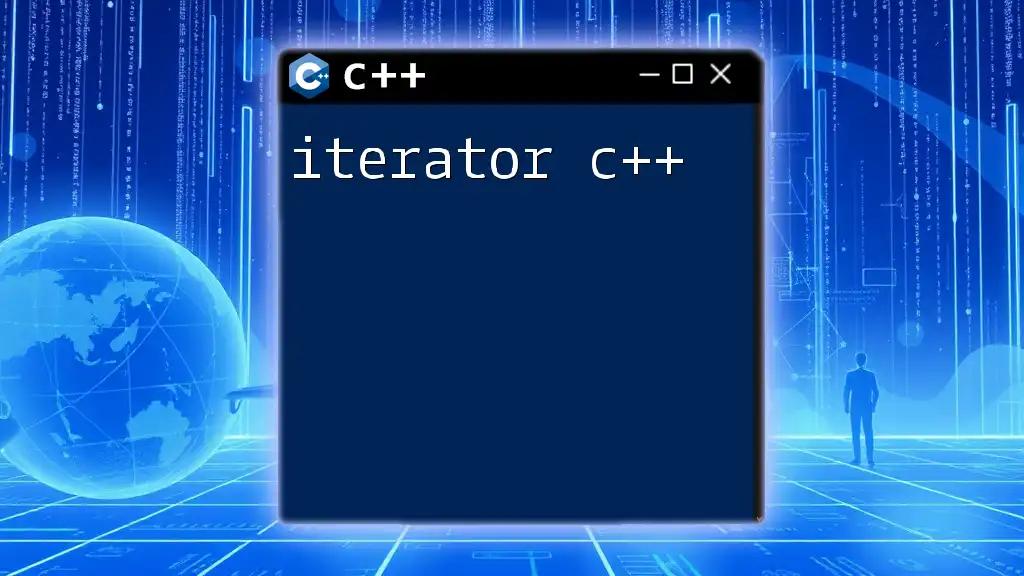
Conclusion
In this guide, we've examined the potential of Sierra Chart C++ for creating customized trading solutions. From understanding the API to writing your first custom study, the steps outlined here provide a solid foundation for developing your trading strategies. As you venture further into C++ programming, remember to continually test, optimize, and innovate.
Next Steps for Learners
To deepen your knowledge, explore additional resources, coding forums, and communities focused on Sierra Chart and C++. With practice and persistence, you can leverage C++ to elevate your trading strategies to new heights.