In C++, `char` is a data type used to store a single character, and it can be declared using the syntax `char c;` where `c` is the name of the variable.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
char c = 'A'; // Declare a character variable and assign it the value 'A'
std::cout << "The character is: " << c << std::endl;
return 0;
}
Understanding Char in C++
What is a Char?
In C++, a `char` is a fundamental data type used to represent a single character. It can store any single character letter, digit, or symbol, and is commonly used in text processing. The flexibility of the `char` data type allows it to serve as a cornerstone for building strings and handling character data.
Why Use Char?
Using `char` is essential for multiple reasons:
- Memory Efficiency: A `char` typically requires only one byte of memory, making it an ideal choice for storing small amounts of textual data. When compared to more complex data types, such as `std::string`, it offers a more lightweight alternative.
- Performance: Operations on `char` types are generally faster due to their simplicity, especially when processing large datasets.

Declaring and Initializing Char Variables
Basic Declaration
Declaring a `char` variable is straightforward. You specify the type followed by the variable name and assign a character:
char letter = 'A';
In this example, `letter` is a variable of type `char` assigned the character ‘A’. Remember, when indicating a character, you must use single quotes.
Multiple Char Declarations
You can also declare multiple `char` variables in a single line:
char a = 'a', b = 'b', c = 'c';
This approach increases code efficiency by reducing clutter from repeated declarations.
Initializing with ASCII Values
Chars can be initialized using their ASCII values, which provides an underlying numeric representation of characters:
char asciiChar = 65; // Corresponds to 'A'
The ASCII table lists the numeric values assigned to characters, and using these values can simplify operations where you need numeric representation for comparison or calculations.

Working with Char in C++
Basic Operations on Char
You can perform several basic operations with chars:
-
Comparison: You can compare two char variables using relational operators. For example:
char a = 'A', b = 'B'; if (a < b) { // This block executes as 'A' is less than 'B' }
-
Assignment: Changing the value of a char variable is as simple as another assignment:
char letter = 'C'; letter = 'D'; // Now letter holds 'D'
Char Arrays and Strings
Declaring Char Arrays
A `char` array is a collection of characters, and it's often used to store string data in C++:
char name[10] = "John";
In this example, an array named `name` can hold up to 10 characters, and its contents can be manipulated through indexing.
Manipulating Char Arrays
You can iterate over the elements in a char array using loops. This is vital for operations like string searches or pattern matching. Here is how to print each character in a `char` array:
for (int i = 0; i < 10; i++) {
std::cout << name[i];
}
Be careful: in C++, strings must be null-terminated (`'\0'`). Forgetting to do this can lead to unexpected behaviors.
Converting Between Char and Other Types
At times, you may want to convert between `char` and other data types, primarily integers:
char digit = '5';
int number = digit - '0'; // Converts char to int
This conversion is rooted in ASCII. By subtracting the ASCII value of ‘0’ from the character, you retrieve the integer value.
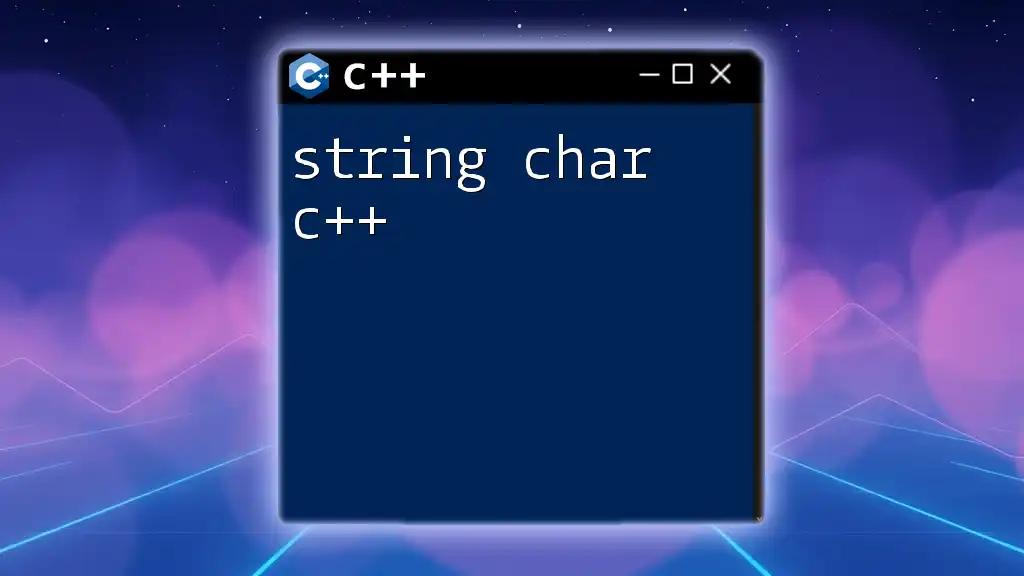
Advanced Char Usage
Char Pointers and Dynamic Memory
Working with pointers expands the versatility of `char` usage. You can dynamically allocate memory for a char array as follows:
char* ptr = new char[10];
This line allocates memory for 10 characters, allowing for greater flexibility. Remember to manage this memory carefully to avoid leaks.
Understanding Character Encoding
C++ does not classify characters strictly; understanding different encoding systems is essential. Two common encodings are ASCII and UTF-8.
- ASCII: Primarily used for English characters, representing 128 characters.
- UTF-8: Supports a broader range of characters, including symbols and characters from various languages.
The significance of character encoding lies in ensuring that text is handled correctly across different platforms and languages.
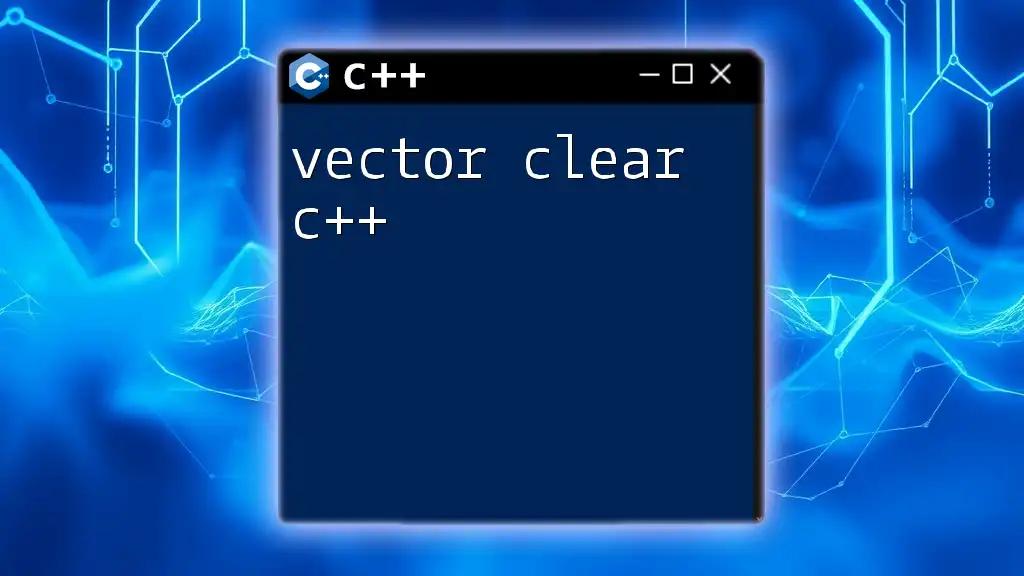
Practical Examples
Using Char in a Program
Here’s a simple example program that effectively utilizes `char`:
#include <iostream>
int main() {
char initial = 'J';
std::cout << "My initial is: " << initial << std::endl;
return 0;
}
This program initializes a `char` variable and outputs its value, encapsulating the essence of using `char` in basic C++ applications.
User Input with Char
Receiving user input in the form of a char can also be easily implemented:
char input;
std::cout << "Enter a character: ";
std::cin >> input;
std::cout << "You entered: " << input << std::endl;
In this snippet, the program waits for the user to input a character and then displays it, demonstrating the interactivity commonly needed in applications.

Best Practices
When to Use Char vs. Other Types
Using `char` over `std::string` is preferable in scenarios requiring a single character or when low memory usage is critical. However, if your data involves multiple characters or requires manipulation, `std::string` may be a better choice.
Error Handling with Char
Avoid common pitfalls such as incorrect initialization or not accounting for null-terminators, which can lead to buffer overflows or unexpected behavior in your programs.

Conclusion
Understanding how to effectively utilize `char` in C++ is pivotal for any programmer. The power and simplicity of the `char` data type, coupled with its memory efficiency and performance, make it a valuable tool in your programming arsenal. By mastering `char` and its applications, you set the stage for more advanced text manipulation in C++.

Additional Resources
For a deeper dive into the world of C++, resources like the official documentation, online programming courses, and extensive programming communities can provide valuable insights.

Call to Action
Practice using `char` in your projects and share your experiences. Exploring different usages will enhance your programming expertise and encourage discovery in the versatility of C++. Keep learning and experimenting with C++ to unlock its full potential!