In C++, you can convert an integer to a character by adding it to the ASCII value of '0', as shown in the following code snippet:
char myChar = '0' + myInt; // where myInt is the integer you want to convert
Understanding Data Types in C++
What is an `int`?
An `int`, short for integer, is a fundamental data type in C++. It represents whole numbers, both positive and negative, as well as zero. Integers are commonly used in programming to handle countable quantities, perform mathematical operations, and serve as index values within arrays.
What is a `char`?
A `char` represents a single character in C++. It is typically used to store letters, digits, symbols, and other characters in the Unicode character set. In the context of text manipulation, `char` plays a key role, particularly when dealing with strings and direct character representation.
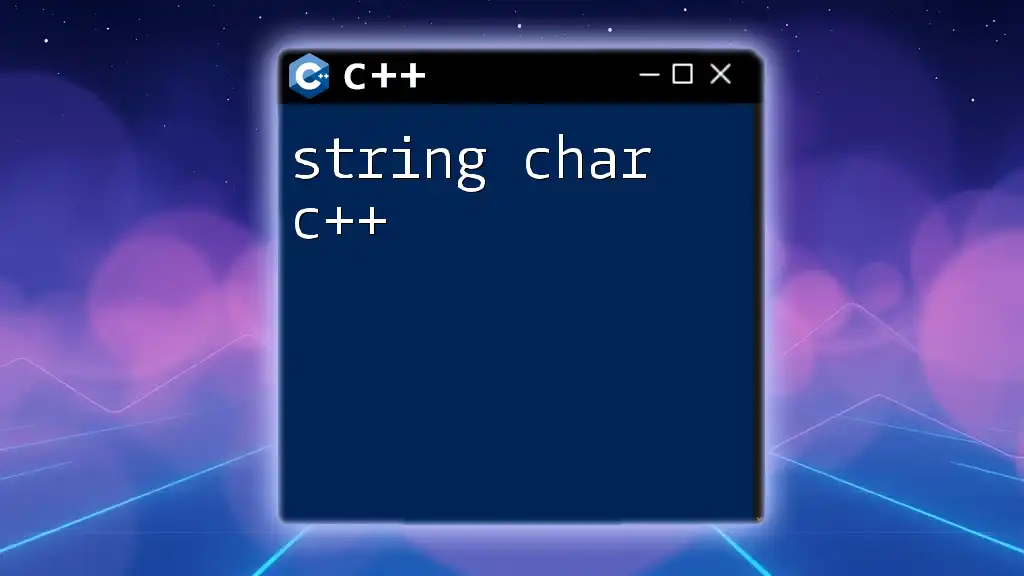
Why Convert `int` to `char`?
Data representation
Converting an `int` to a `char` is vital in representing numeric values in a human-readable format. For instance, when rendering the value of an integer as a character in a user interface, one needs to perform this conversion. The characters corresponding to their ASCII values become the visual representation of these numbers.
Use cases
There are numerous practical applications where this conversion is necessary. For example:
- User Interfaces: Displaying numeric scores.
- Communication Protocols: Sending data across a network, in which integers may need to be converted to characters for transmission.
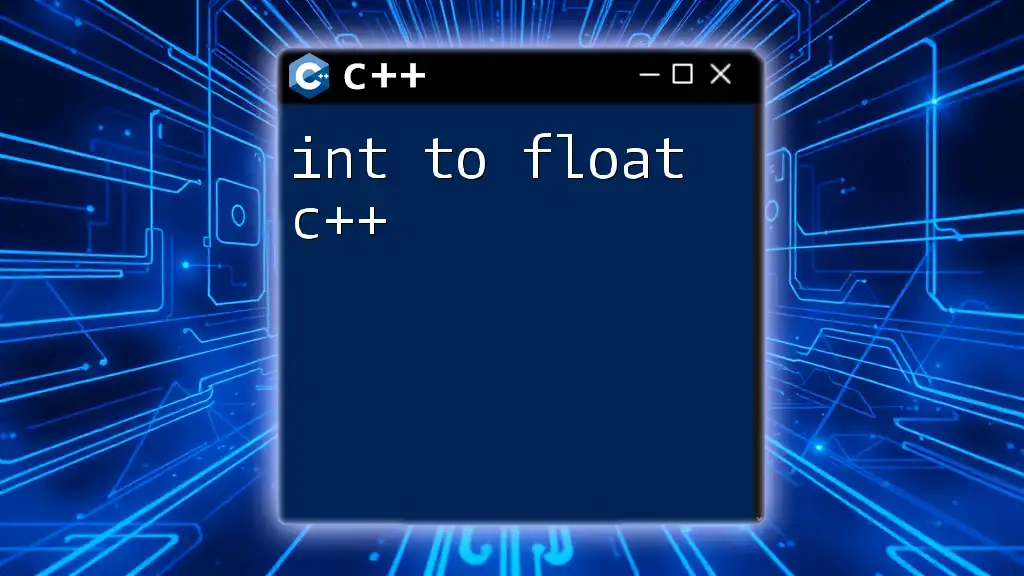
Methods to Convert `int` to `char`
Implicit Conversion
In C++, the automatic type conversion occurs when an integer value is assigned directly to a character variable. This behavior often simplifies development, as the conversion happens seamlessly.
For example:
int myInt = 65;
char myChar = myInt; // Implicit conversion
In the above code snippet, the integer `65` corresponds to the ASCII value for the character `A`. Thus, `myChar` would hold `A` after the assignment.
Explicit Conversion
For scenarios where you need definitive control over the conversion process, using explicit conversion is advisable.
Using `static_cast`
The `static_cast` operator allows for safe type conversion in C++. This form of explicit conversion enhances code clarity, as it indicates the programmer's intention.
Example of converting an integer to a character using `static_cast`:
int myInt = 66;
char myChar = static_cast<char>(myInt); // Explicit conversion using static_cast
Here, `myChar` would hold the character `B`, represented by the ASCII value `66`.
Using `char()` Constructor
Another method for conversion involves utilizing the `char()` constructor. This empowers developers to create a character representation from an integer with simple syntax.
Here's how it looks in code:
int myInt = 67;
char myChar = char(myInt); // Use of char() constructor
This also results in `myChar` holding `C`, corresponding to ASCII value `67`.
Conversion Through Function
For more reusable code, a conversion function can be developed. This can be particularly useful when multiple conversions are needed throughout your program.
Here’s an example of such a function:
char convertIntToChar(int number) {
if(number < 0 || number > 255) {
throw std::out_of_range("Number out of valid char range");
}
return static_cast<char>(number);
}
This function first checks if the input value falls within the valid range for characters (0-255). If not, it throws an exception, ensuring safety during conversions.
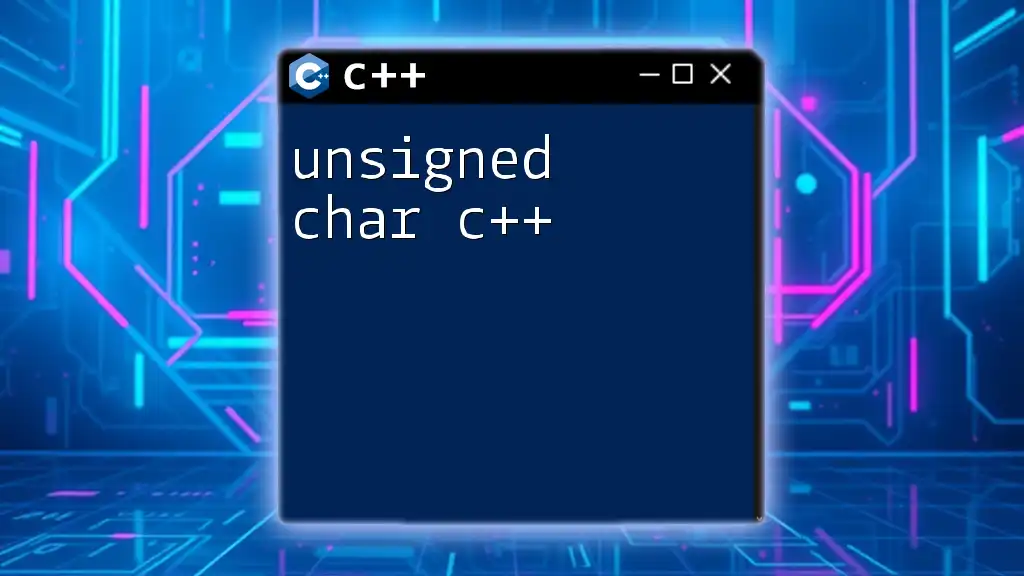
Important Considerations
Valid Range of Characters
Understanding the ASCII Values is crucial when converting an `int` to a `char`. The valid range for a `char` in C++ is generally between `0` and `255`. The ASCII table offers a reference for what characters correspond to each integer within this scope.
Handling Errors
As demonstrated with the function above, it is essential to manage errors during conversion. Converting an integer that exceeds the range of valid characters can lead to undefined behavior. Utilizing proper exception handling helps maintain robustness in your code.
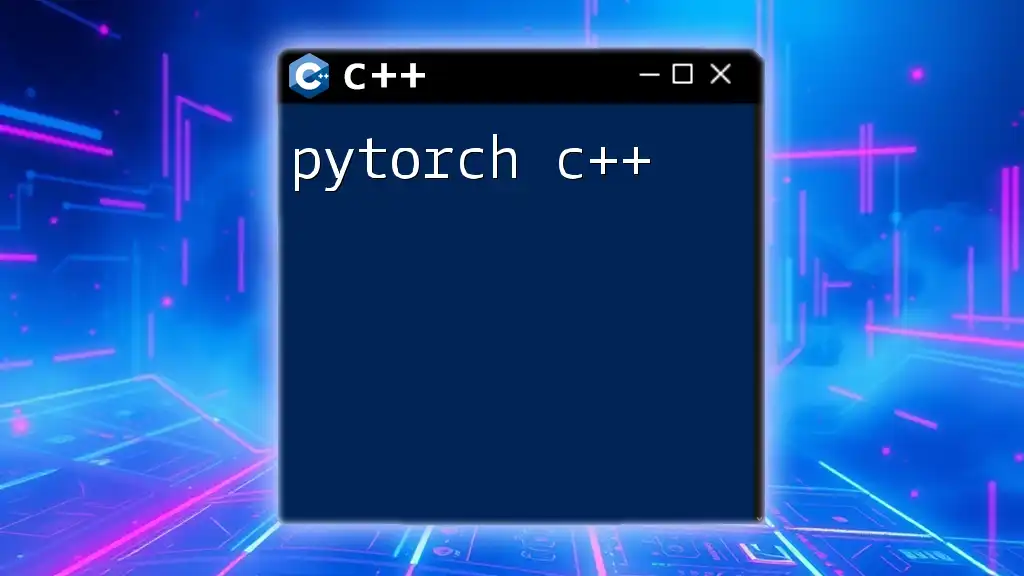
Conclusion
In summary, the conversion from `int to char` in C++ is not only a common necessity but also an essential technique to represent and manipulate data effectively. By understanding the methods available—ranging from implicit conversions to explicit casting—you can choose an approach that suits your needs best. Practicing these techniques and applying them to real-world scenarios will significantly enhance your programming skills in C++.