In C++, you can use the `typeid` operator along with `std::cout` to print the data type of a variable in a quick and concise manner. Here's an example:
#include <iostream>
#include <typeinfo>
int main() {
int number = 42;
std::cout << "The type of number is: " << typeid(number).name() << std::endl;
return 0;
}
Understanding Print Type in C++
What is Print Type in C++?
Print type in C++ refers to how data is outputted to the console or any other output stream. It plays a vital role in displaying information in a readable format, aiding both the developer and the end-user. Having a deep understanding of print type is essential as it helps in crafting clear and effective user interfaces, and debugging your programs efficiently.
Why Knowing Print Types is Essential?
Understanding how different data types can be printed allows developers to effectively convey messages, results, and error feedback. Properly displaying information can drastically enhance user experiences, making applications more intuitive and easier to use.
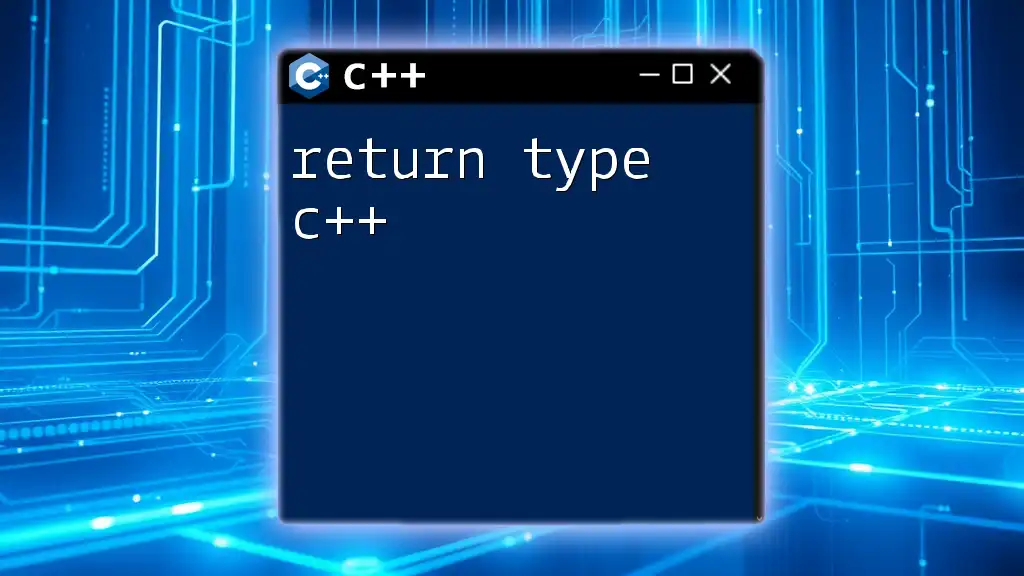
Basic Concepts of Printing in C++
The Role of the `iostream` Library
The `iostream` library is the cornerstone of input and output (I/O) operations in C++. It provides functionality for reading from and writing to standard input and output streams. To utilize this library in your program, include it at the beginning of your C++ file:
#include <iostream>
Output Stream: `std::cout`
The `std::cout` object is a powerful tool that allows for printing text and variables to the console. It is essential for displaying data, making it a critical component in most C++ applications. The syntax for using `std::cout` is straightforward. For example:
std::cout << "Hello, World!" << std::endl;
This line outputs the string "Hello, World!" to the console, followed by a newline.
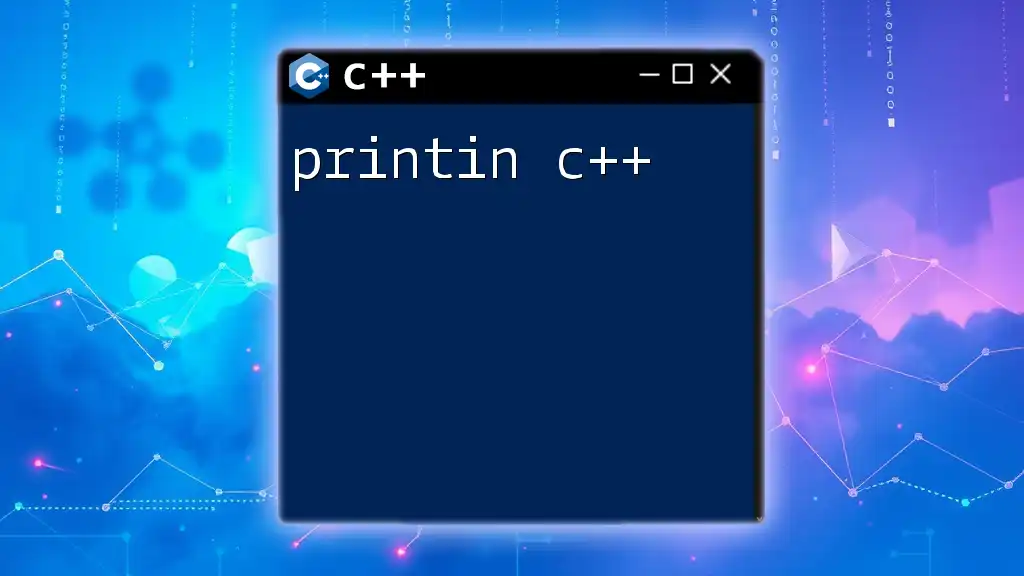
Printing Data Types in C++
Printing Primitive Data Types
Printing Integers
Printing integers is as simple as passing the integer variable to `std::cout`. For example:
int number = 42;
std::cout << "The number is: " << number << std::endl;
The output will clearly show "The number is: 42", making it easy to utilize integers in your output.
Printing Floating-Point Numbers
For floating-point values, the process remains similar. Consider this example:
float pi = 3.14f;
std::cout << "Value of Pi: " << pi << std::endl;
This outputs "Value of Pi: 3.14", demonstrating how `std::cout` can handle float types effectively.
Printing Characters
Printing characters follows the same pattern. Here's how it looks:
char letter = 'A';
std::cout << "The letter is: " << letter << std::endl;
The output "The letter is: A" showcases how to display single character values.
Printing Composite Data Types
Printing Strings
Utilizing the `std::string` class enhances string manipulation and output:
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
This prints "Hello, World!" directly, showcasing the ease of string handling in C++.
Printing Arrays
Printing arrays requires a loop to iterate over each element. Here’s an example using a simple for-range loop:
int numbers[] = {1, 2, 3};
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
The result shows "1 2 3", illustrating how to print multi-element data.
Using Format Specifiers
Formatting Outputs
C++ provides several formatting options to enhance output clarity. You can set precision for floating-point numbers using manipulators:
#include <iomanip> // Include this for std::setprecision
std::cout << std::fixed << std::setprecision(2) << pi << std::endl;
This will output "3.14", ensuring that the float number only shows two decimal places, improving data presentation.
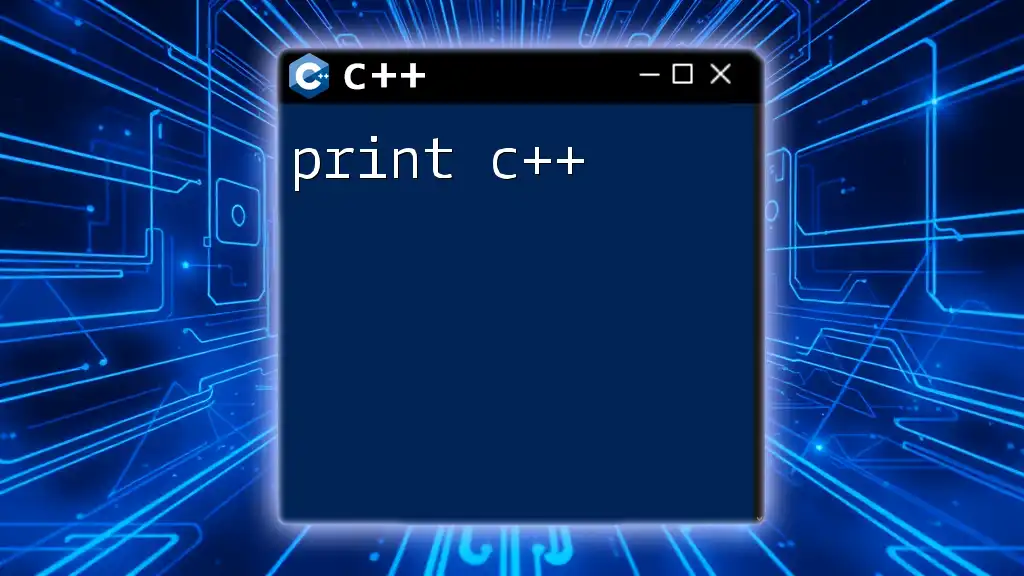
Advanced Printing Techniques
User-defined Data Types
Printing Structures
When working with structures, you can access and print its members directly. For example:
struct Point {
int x;
int y;
};
Point p = {10, 20};
std::cout << "Point coordinates: (" << p.x << ", " << p.y << ")" << std::endl;
This line outputs "Point coordinates: (10, 20)", effectively demonstrating how to represent structured data.
Printing Classes
Classes in C++ come with member functions that can aid in displaying object data. Here's an example:
class Person {
public:
std::string name;
int age;
void printInfo() {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
};
Person p;
p.name = "Alice";
p.age = 30;
p.printInfo(); // Outputs: Name: Alice, Age: 30
By encapsulating the print logic inside a class method, you can maintain clearer and more maintainable code.
Overloading the Output Operator
C++ allows for the overloading of operators, including the output operator, which can be implemented for more customized output methods. Here’s how it’s done:
std::ostream& operator<<(std::ostream& os, const Point& p) {
return os << "(" << p.x << ", " << p.y << ")";
}
Once defined, you can simply use `std::cout << yourPoint;` for clean data representation.
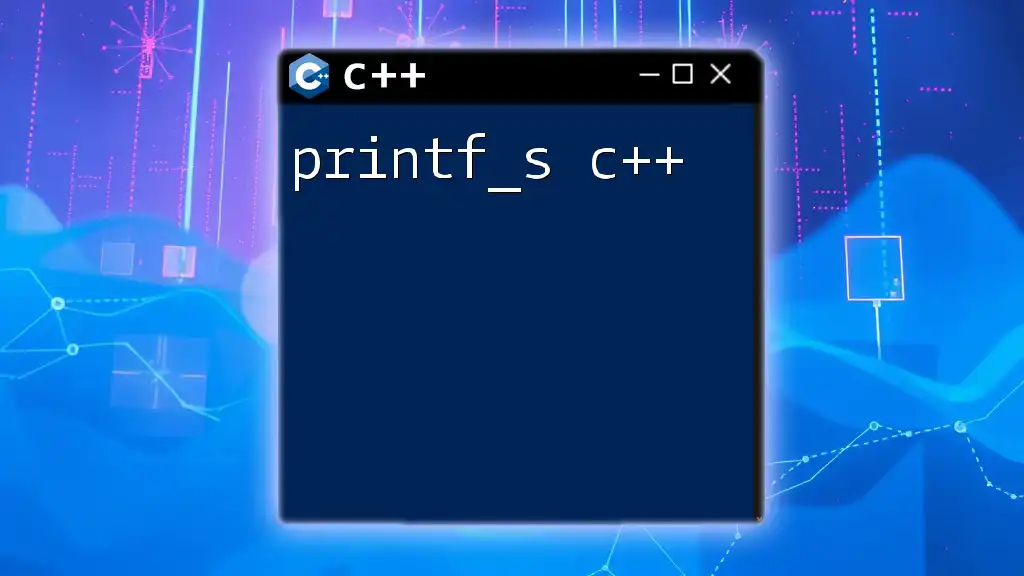
Common Challenges and Solutions
Common Errors in Printing
Some frequent pitfalls include type mismatches or forgotten libraries. Ensuring that you include the correct headers, such as `#include <iostream>` and `#include <iomanip>`, is vital. It's also essential to match your data types with suitable print statements.
Enhancing Output Readability
To improve the readability of your printed output, consider using formatting options effectively. Also, you can introduce line breaks or section headers in longer outputs to avoid cluttered interfaces.
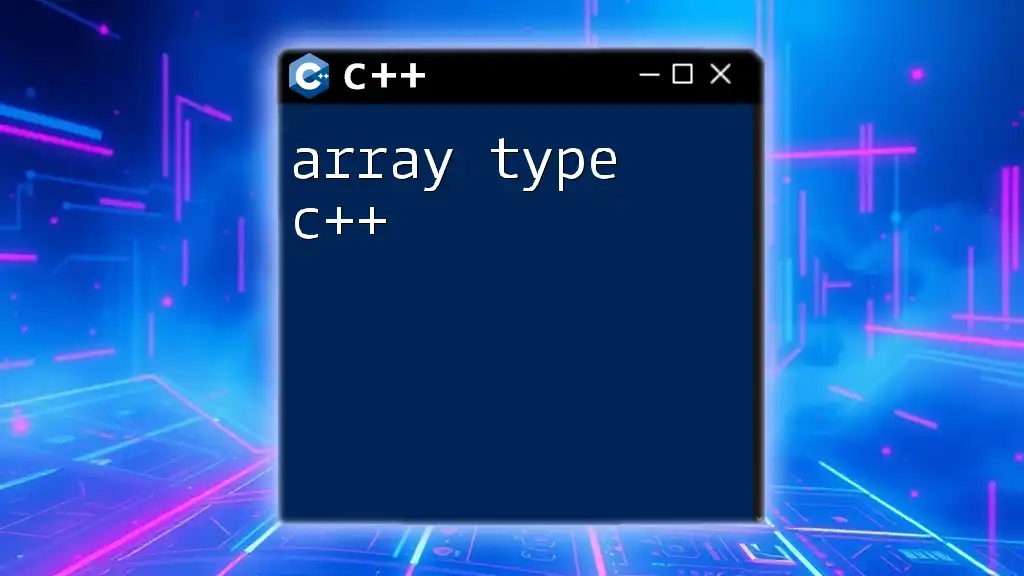
Conclusion
Understanding how to correctly implement print type C++ is essential for effective programming. It enables users to craft intuitive applications where information is conveyed clearly and effectively. Practicing different data types, as well as output methods, will empower you as a developer and enhance your coding proficiency.
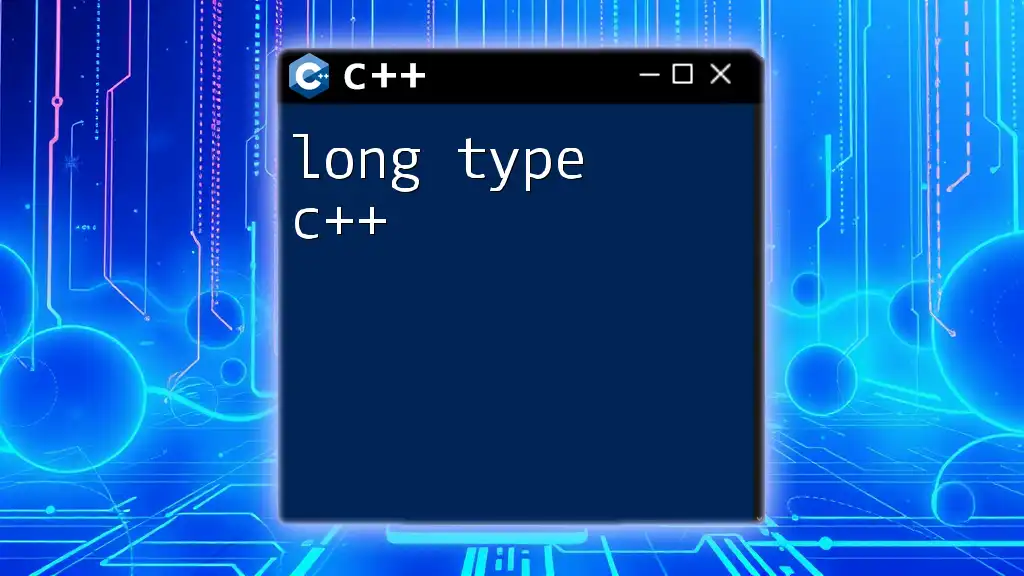
Additional Resources
Integrate various resources like online tutorials, documentation, and recommended books into your learning path to deepen your understanding of C++ fundamentals.
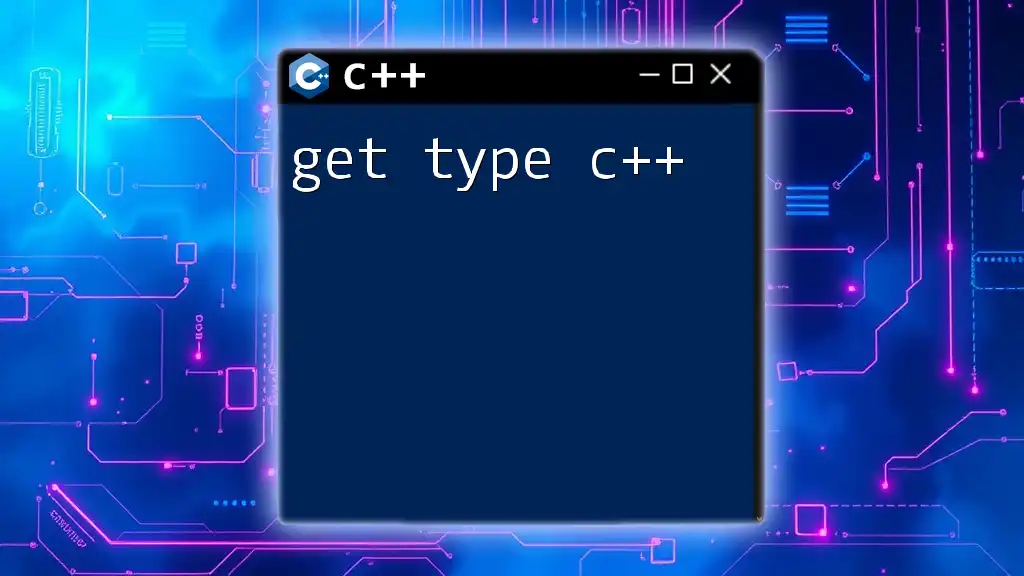
Call to Action
Experiment with various print types in your personal projects and explore community forums for collaborative learning. Whether you're debugging a small program or designing a complex application, mastering print types will serve you well in your C++ journey.