In C++, you can determine the type of a variable using the `typeid` operator from the `<typeinfo>` header, which provides the type information at runtime.
Here's a code snippet demonstrating how to print the type of a variable:
#include <iostream>
#include <typeinfo>
int main() {
int myVar = 42;
std::cout << "The type of myVar is: " << typeid(myVar).name() << std::endl;
return 0;
}
Understanding Variable Types in C++
What is a Variable?
A variable in C++ is essentially a storage location identified by a name that can hold a value. It represents a fundamental concept in programming, allowing developers to create flexible and reusable code. Variables enable you to store user input, perform calculations, and manage large datasets.
Importance of Knowing Variable Types
Understanding variable types is crucial in C++ for several reasons:
- Type Safety: C++ is a statically typed language, meaning that the type of a variable is determined at compile time. This helps catch errors early, increasing robustness.
- Memory Management: Different data types require different amounts of memory. Knowing their sizes can help minimize memory usage, crucial for performance in larger applications.
- Performance Optimization: Certain operations can be optimized based on the type of data being handled, resulting in more efficient code execution.
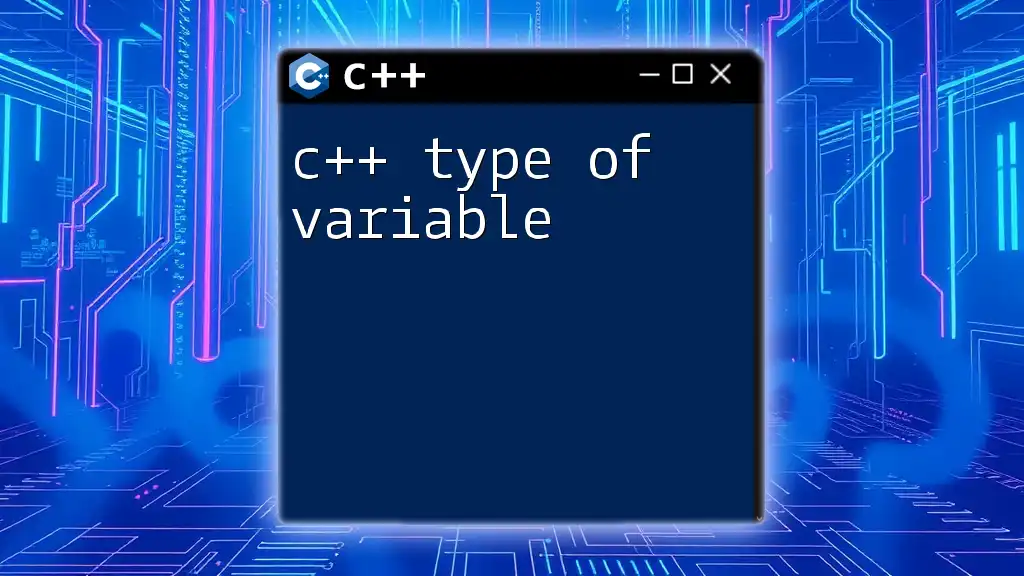
C++ Data Types Overview
Built-in Data Types
In C++, there are several built-in data types you should be familiar with:
- int: Used for whole numbers. For example, `int age = 25;`
- float: Represents single-precision floating-point numbers, useful for storing decimals. For example, `float pi = 3.14f;`
- double: For double-precision floating-point numbers, offering greater precision. For example, `double e = 2.71828;`
- char: Stores individual characters. For instance, `char initial = 'A';`
- bool: Represents Boolean values, either `true` or `false`. For example, `bool is_valid = true;`
User-defined Data Types
In addition to built-in types, C++ allows you to create user-defined data types:
-
Structs: A way to group variables of different types under a single name. For example:
struct Person { std::string name; int age; };
-
Classes: Object-oriented programming features that encapsulate data and functions. A simple example would be:
class Car { std::string model; int year; };
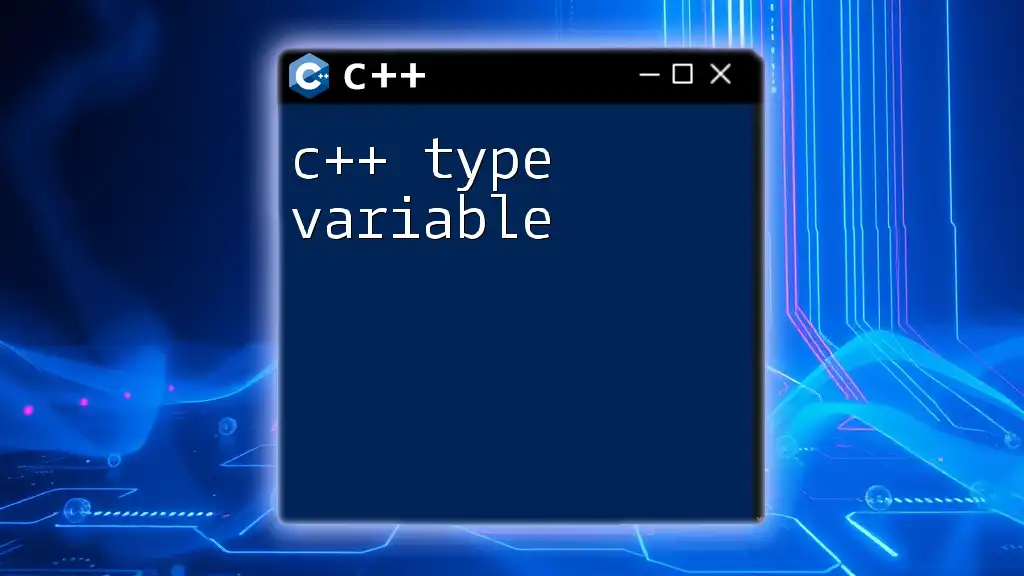
How to Use `typeid` to Identify Variable Types
Introduction to `typeid`
The `typeid` operator in C++ is an invaluable tool used to retrieve the type information of a variable at runtime. This operator is defined in the `<typeinfo>` header and can assist developers in debugging and understanding their code better.
Basic Syntax and Implementation
To use `typeid`, simply include the relevant header and invoke it on your variable. Here's a straightforward example:
#include <iostream>
#include <typeinfo>
int main() {
int var = 10;
std::cout << "The type of var is: " << typeid(var).name() << std::endl;
return 0;
}
In this example, when executed, it will output something like `int` or a mangled name that represents the type, depending on the compiler.
Understanding `typeid` Output
The output of `typeid` might vary depending on the platform or compiler, as different compilers may provide a mangled version of type names. Tools like `c++filt` can be used to demangle these names for better understanding.
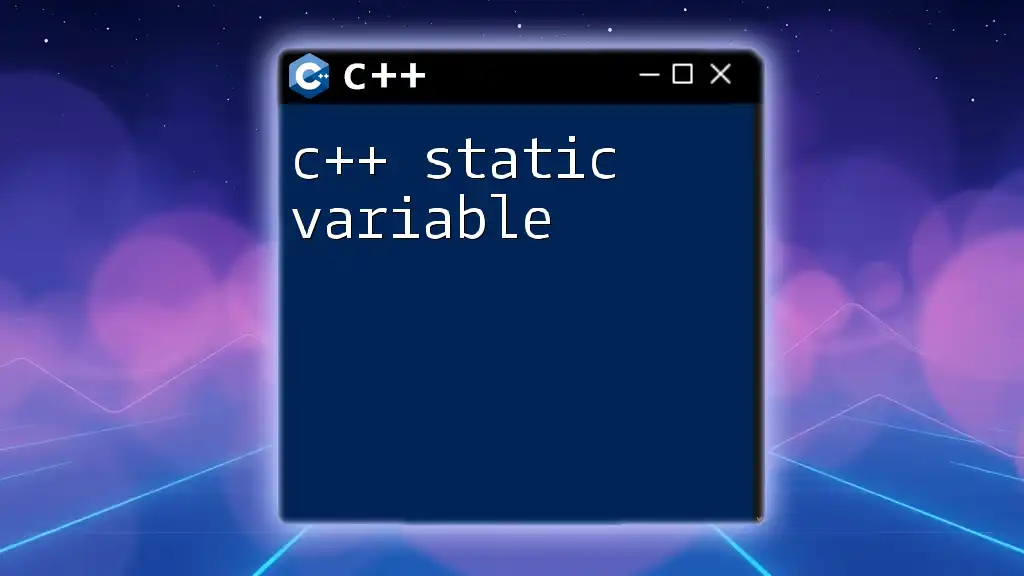
Using `decltype` for Type Deduction
Introduction to `decltype`
The `decltype` keyword allows you to query the type of a variable without explicitly defining it. This can significantly simplify code by allowing for automatic type inference, especially in complex applications.
Practical Example
Consider the following example that demonstrates `decltype`:
#include <iostream>
int main() {
auto var = 5.5;
decltype(var) anotherVar = 10.0;
std::cout << "The type of anotherVar is: " << typeid(anotherVar).name() << std::endl;
return 0;
}
In this snippet, `anotherVar` will be deduced as a `double`, based on the type of `var`. By using `decltype`, you maintain strong typing while simplifying variable declarations.
Advantages of Using `decltype`
- Ease of Use: You don’t need to manually define types, which reduces redundancy and potential errors.
- Type Inference: As the code evolves, `decltype` ensures that variable types remain consistent without additional edits.
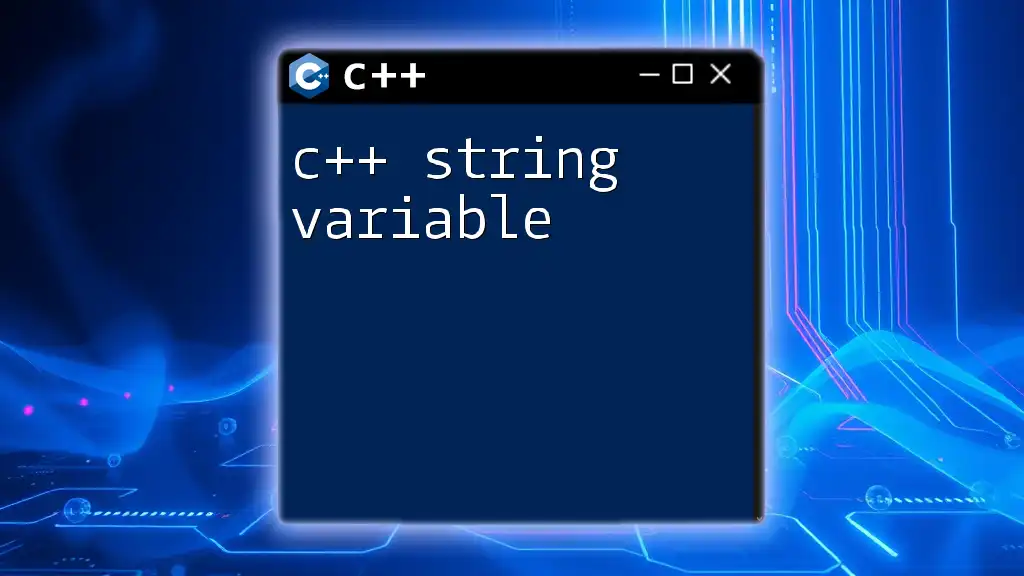
Utilizing `std::variant` for Multiple Types
What is `std::variant`?
Introduced in C++17, `std::variant` is a type-safe union that can hold a value from a set of specified types, making it particularly useful for managing complex data scenarios.
Example of `std::variant`
Here’s how you can utilize `std::variant` to check variable types:
#include <iostream>
#include <variant>
int main() {
std::variant<int, float> var = 10;
if (std::holds_alternative<int>(var)) {
std::cout << "The variable is an int." << std::endl;
} else {
std::cout << "The variable is a float." << std::endl;
}
return 0;
}
In this code, `std::holds_alternative<int>(var)` checks whether the variant currently holds an `int`, showcasing the flexibility and type safety offered by `std::variant`.
Benefits of Using `std::variant`
- Type Safety: With `std::variant`, you can safely store and retrieve different data types without fear of data corruption.
- Flexibility in Handling Different Types: Unlike traditional unions, `std::variant` knows the active member, reducing the risk of errors associated with type mismatches.
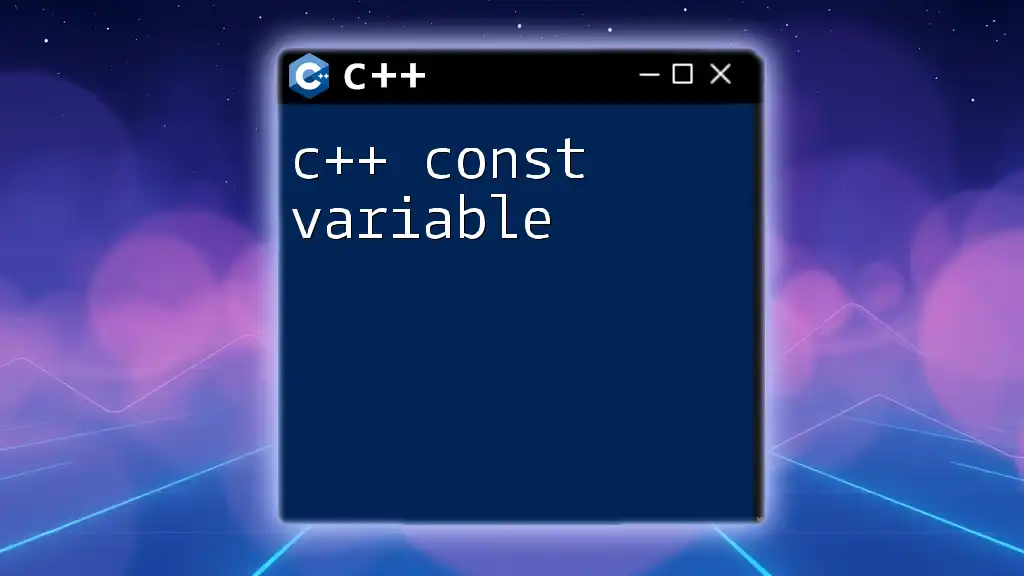
Common Mistakes When Printing Variable Types
Misunderstanding Type Names
Developers often misinterpret the output from `typeid`, especially when dealing with user-defined types. The names you see printed may appear cryptic or mangled. It's essential to consult your compiler's documentation to understand how type names are presented.
Avoiding Type Casting Errors
Type casting errors can lead to undefined behavior in your program. Always double-check that the type you are attempting to cast to is compatible with what the variable currently holds. Utilizing tools like `std::variant` can help minimize such situations.
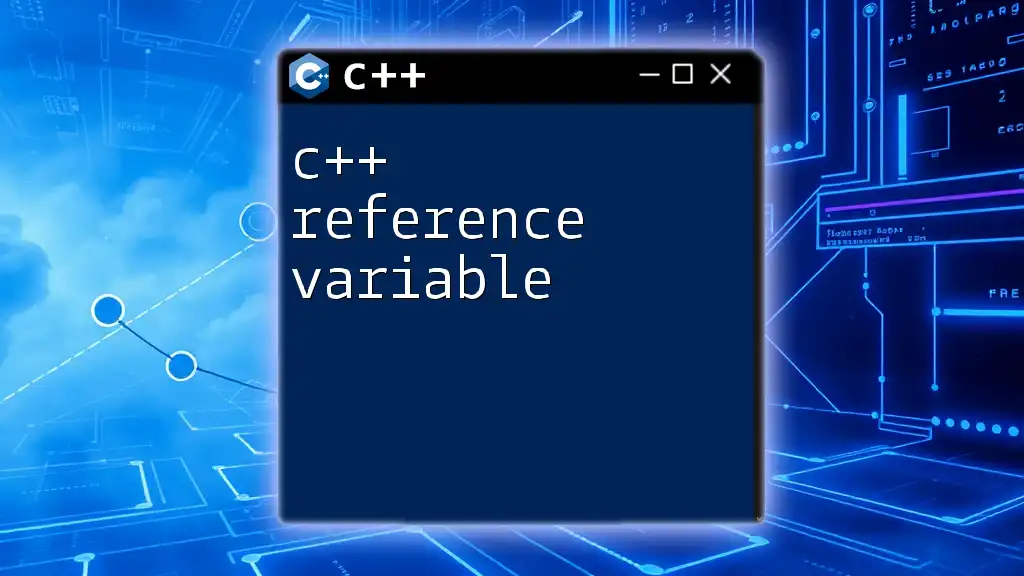
Conclusion
In summary, knowing how to C++ print type of variable is crucial for robust programming. Tools like `typeid`, `decltype`, and `std::variant` provide essential capabilities that not only simplify your code but also enhance type safety. As you continue your C++ journey, embracing these concepts will keep your code efficient and maintainable, empowering you to tackle increasingly complex programming challenges. Don't forget to continue exploring and practicing to develop a deeper understanding of these concepts!