An atomic variable in C++ is a type of variable that provides a way to perform operations on it in a thread-safe manner without requiring explicit locks, ensuring that read and write operations occur without interference from other threads.
Here's a simple example of using an atomic variable in C++:
#include <iostream>
#include <atomic>
#include <thread>
std::atomic<int> atomicCounter(0);
void incrementCounter() {
for (int i = 0; i < 1000; ++i) {
atomicCounter++;
}
}
int main() {
std::thread t1(incrementCounter);
std::thread t2(incrementCounter);
t1.join();
t2.join();
std::cout << "Final counter value: " << atomicCounter << std::endl;
return 0;
}
Understanding Atomic Variables in C++
What Are Atomic Variables?
Atomic variables are a crucial concept in concurrent programming, designed to provide a way to work with shared data across multiple threads without the need for complex locking mechanisms. An atomic variable guarantees that operations on it will be completed in a single step from the perspective of other threads. This means without the risk of interruptions, making them an essential tool for avoiding race conditions in multi-threaded applications.
Using atomic operations over regular variables is critical when you want to ensure thread safety and maintain the integrity of shared data. Unlike regular variables, atomic variables provide built-in operations that are guaranteed to be performed without interference from other threads.
The C++ Standard Library and Atomic Variables
In C++, atomic variables are part of the standard library, specifically included in the `<atomic>` header. This header provides essential support for atomic types that help developers leverage multi-threading capabilities dauntlessly. C++11 introduced these features, establishing a foundation for safer and more efficient concurrent programming.
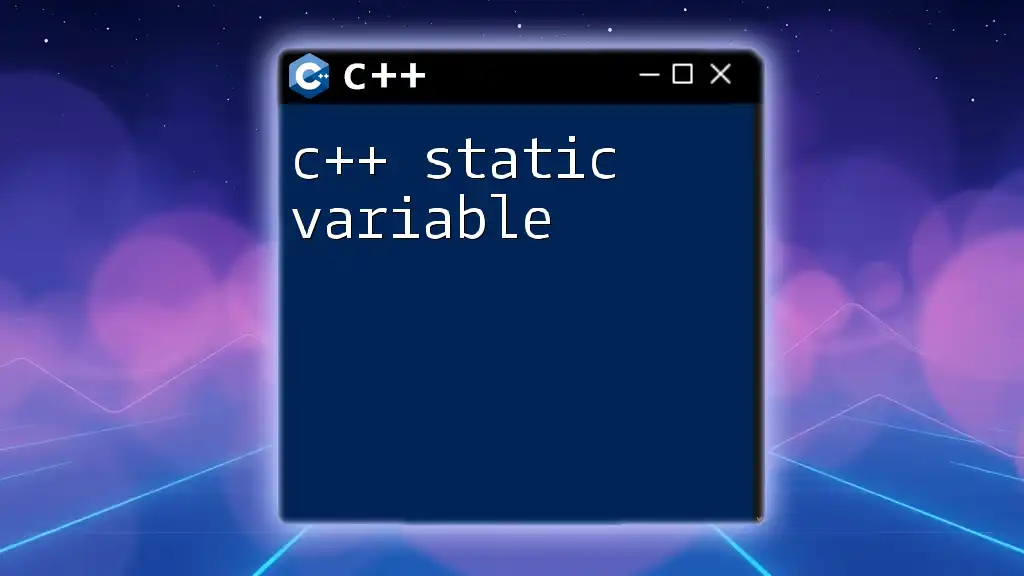
Characteristics of C++ Atomic Variables
Properties of Atomic Variables
Lock-Free Operations serve as one of the primary advantages of using atomic variables. In many threading scenarios, using mutex locks can lead to performance degradation, particularly when lock contention becomes a bottleneck. By utilizing atomic operations, developers can create efficient threads that run concurrently without needing to acquire locks, thus reducing wait times and improving application responsiveness.
However, the decision to use atomic operations requires careful analysis of the specific use case. While they can offer significant performance benefits, atomic variables should not replace mutexes in all situations, particularly when managing complex shared resources.
Memory Orderings
When working with atomic variables in C++, understanding memory orderings is indispensable. Memory orderings dictate how operations are completed concerning memory visibility across different threads.
There are several types of memory ordering in C++:
- Memory Order Relaxed: Operations appear independent, with no specific order guaranteed.
- Memory Order Acquire: Ensures that all previous reads and writes are completed before any operations after this point.
- Memory Order Release: Guarantees that operations at this point are visible to other threads before any subsequent operations.
- Memory Order Sequentially Consistent: Provides the strictest form, ensuring a single total order of operations across all threads.
Here is an example illustrating the difference in memory orderings:
#include <atomic>
#include <thread>
#include <iostream>
std::atomic<int> x{0};
std::atomic<int> y{0};
void thread1() {
x.store(1, std::memory_order_release);
int temp = y.load(std::memory_order_acquire);
}
void thread2() {
y.store(1, std::memory_order_release);
int temp = x.load(std::memory_order_acquire);
}
In this code, different memory orderings influence the visibility of `x` and `y` across threads. Understanding these memory operations deeply ensures developers can manage data integrity while efficiently harnessing concurrency.
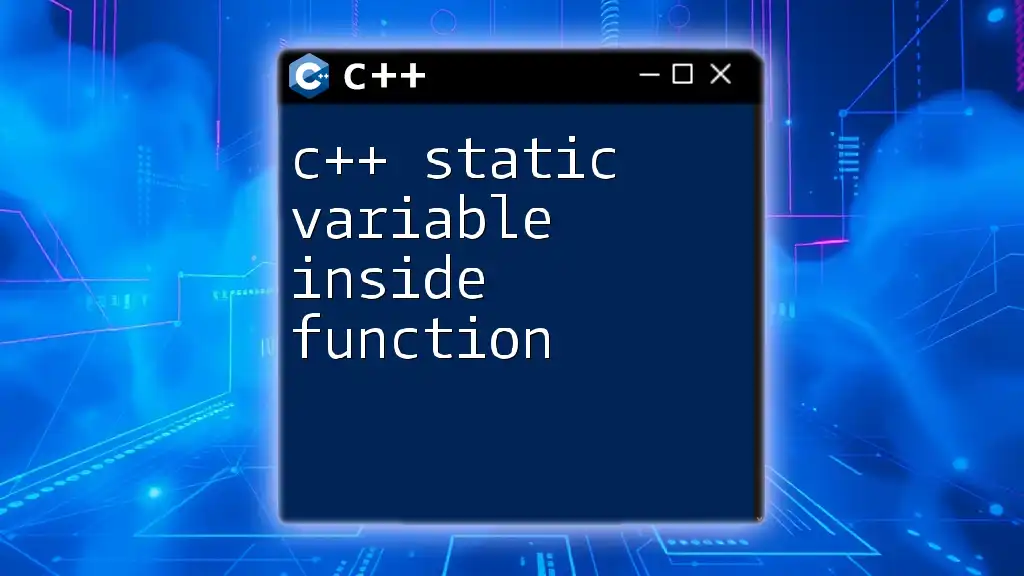
Using Atomic Variables in C++
Declaring Atomic Variables
To declare an atomic variable in C++, the syntax is straightforward. The `std::atomic` template class is used, with the type of the variable specified as a template parameter. For instance:
#include <atomic>
std::atomic<int> atomicCounter{0};
std::atomic<bool> atomicFlag{false};
In these examples, `atomicCounter` is an atomic integer initialized to zero, and `atomicFlag` is an atomic boolean initialized to false. This simple declaration allows both variables to be utilized safely in a multithreaded environment.
Operations on Atomic Variables
Atomic variables allow several operations that are crucial for thread safety. These operations include loading, storing, exchanging, and performing fetch-and-operate functions.
- Load and Store: Basic operations to read and write to an atomic variable.
- Exchange: A method that swaps the current value with a new value.
- Fetch and Operate: These functions include `fetch_add`, `fetch_sub`, which allow you to atomically modify the variable while also returning its original value.
An example of using these operations can be seen below:
std::atomic<int> counter{0};
// Increment the counter atomically
counter.fetch_add(1);
// Retrieve the value, while also storing a new value
int oldValue = counter.exchange(5);
This functionality demonstrates how atomic variables reduce the complexity of managing shared data across threads with minimal overhead.
Thread Safety with Atomic Variables
Thread safety is a critical concern in concurrent programming, where multiple threads may attempt to read or write to common variables simultaneously. Atomic variables provide a solution to ensure that these operations remain safe without cumbersome locks.
When comparing atomic variables to mutexes, it's essential to understand the context in which they shine. Atomic variables excel when dealing with simple shared data that requires a limited number of operations. However, mutexes may still be the optimal choice when you need to coordinate access to complex data structures or multiple shared variables.
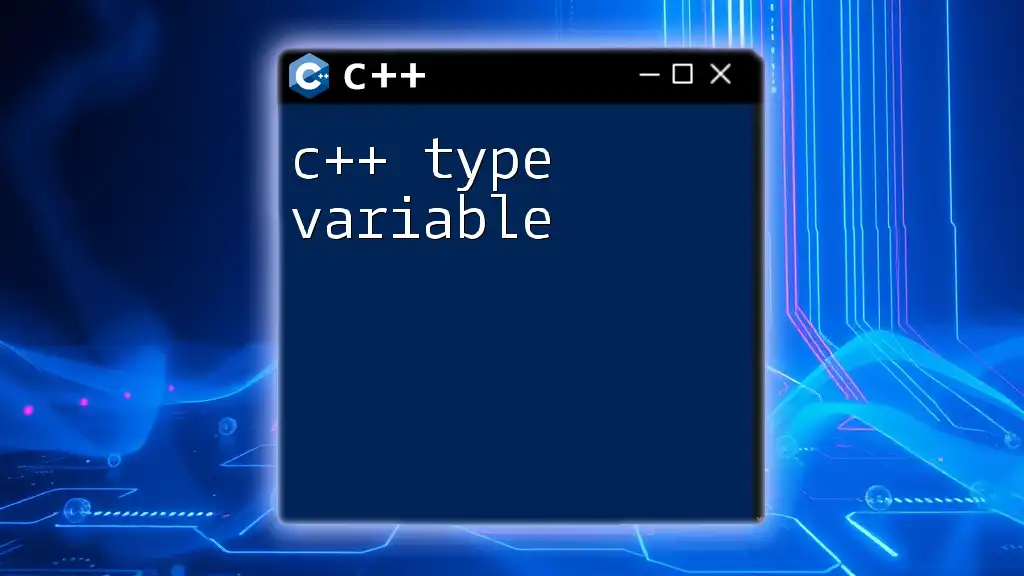
Practical Examples
Example 1: Basic Counter
Let’s consider a basic counter scenario across multiple threads. Using atomic variables allows threads to independently increment the counter without causing race conditions.
#include <iostream>
#include <thread>
#include <atomic>
#include <vector>
std::atomic<int> counter{0};
void incrementCounter() {
for (int i = 0; i < 1000; ++i) {
counter.fetch_add(1);
}
}
int main() {
const int numThreads = 10;
std::vector<std::thread> threads;
for (int i = 0; i < numThreads; ++i) {
threads.emplace_back(incrementCounter);
}
for (auto& thread : threads) {
thread.join();
}
std::cout << "Final Counter Value: " << counter.load() << std::endl;
return 0;
}
In this example, ten threads each increment the shared counter 1000 times, showcasing atomic increment operations that ensure the final count is correctly reported without any race condition issues.
Example 2: Flag Variable
In addition to counters, atomic variables can be employed as flag indicators to control thread execution. Using an atomic boolean flag can signal when threads should stop or start executing based on certain conditions.
#include <iostream>
#include <thread>
#include <atomic>
#include <chrono>
std::atomic<bool> flag{false};
void workerThread() {
while (!flag.load()) {
// Simulate work
std::this_thread::sleep_for(std::chrono::milliseconds(100));
}
std::cout << "Thread exiting.\n";
}
int main() {
std::thread worker(workerThread);
std::this_thread::sleep_for(std::chrono::seconds(1));
flag.store(true); // Signal the worker thread to exit
worker.join();
return 0;
}
In this code snippet, we use an atomic flag to control when the worker thread should stop executing. As soon as the flag is set, the worker thread gracefully exits based on the condition of the atomic flag.
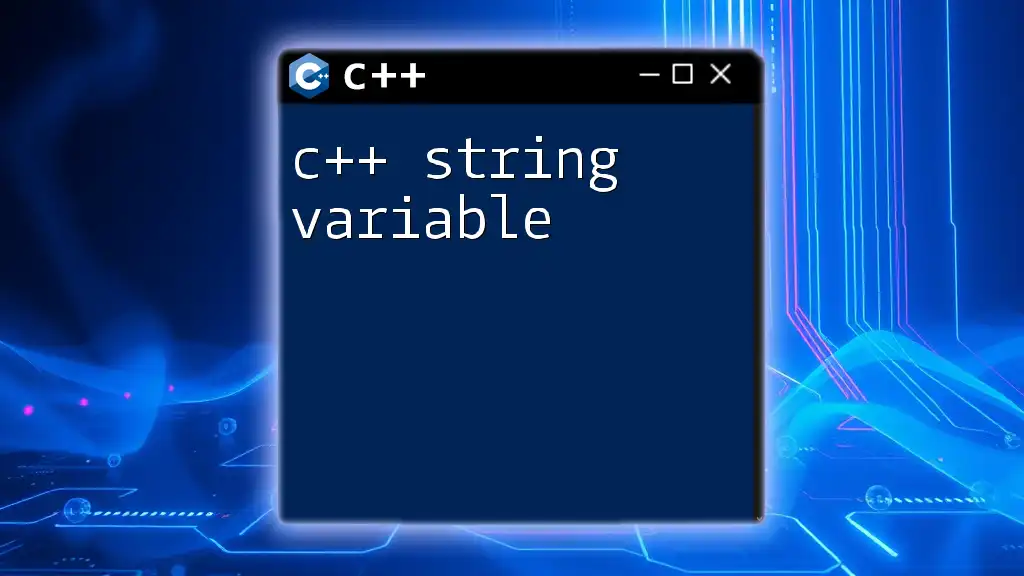
Common Pitfalls with C++ Atomic Variables
Misunderstandings and Misuses
While atomic variables are powerful, there are numerous misconceptions around their usage. Some developers may mistakenly believe that atomic operations can completely replace the need for mutexes or that they are always faster. However, while they simplify the management of simple shared variables, they are not advisable for managing complex data structures, which may still require locking mechanisms to prevent data inconsistency.
Performance Considerations
When it comes to performance, atomic operations are typically faster than mutexes due to the absence of context switches and lock contention. However, they can have detriments if used in inappropriate contexts, particularly when operations become complex. It's crucial to analyze the context thoroughly and make decisions based on the overall performance requirements of the application.
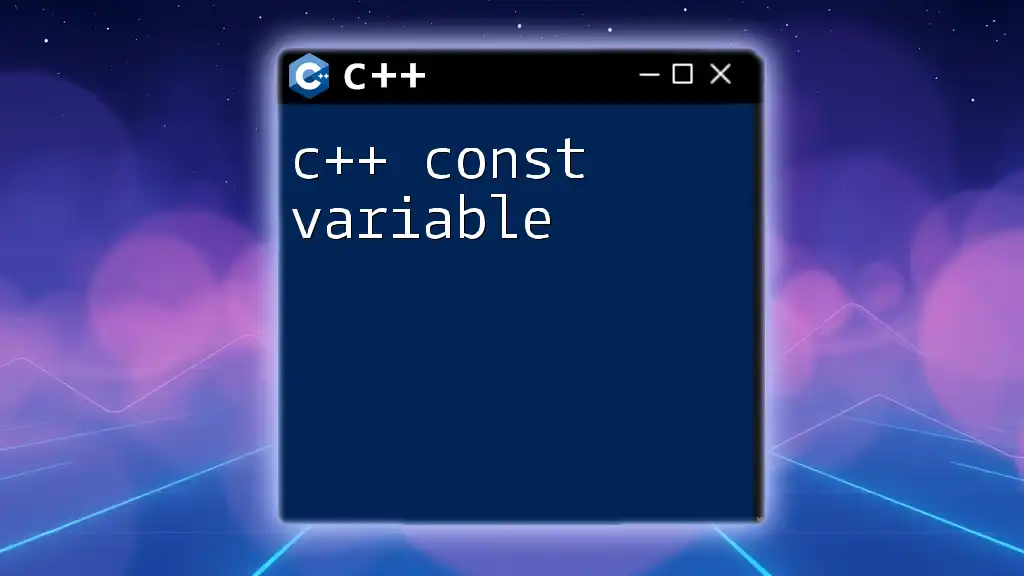
Best Practices for Using Atomic Variables
Guidelines for Effective Usage
To leverage atomic variables effectively, consider the following recommendations:
- Use atomic types for simple, frequently accessed shared data, where the performance overhead of locking mechanisms can be avoided.
- Maintain code readability, as clarity is critical in multithreaded applications. Ensure atomic operations don’t overly complicate the code structure.
- Combine atomic operations with other concurrency mechanisms when necessary. In scenarios needing more complex synchronization, strategic use of locks alongside atomic variables can provide the necessary guarantees about shared resource management.
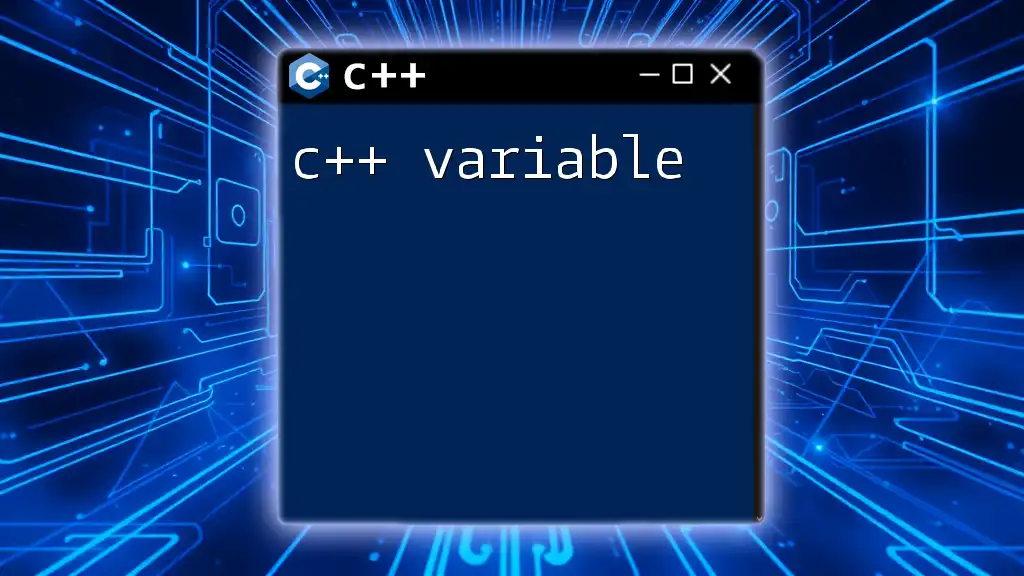
Conclusion
Recap of Atomic Variables
Throughout this guide, we've explored the concept of C++ atomic variables, focusing on their importance in thread safety and efficient concurrent programming. The benefits of atomic operations, including lock-free execution and memory ordering guarantees, position them as a potent tool in the C++ programmer's toolkit.
Call to Action
Now that you have a comprehensive understanding of how c++ atomic variables work, consider integrating them into your projects. They can significantly improve performance and reliability when dealing with shared data across threads. Develop curiosity around ongoing topics in concurrent programming, as it remains a growing area in software development.
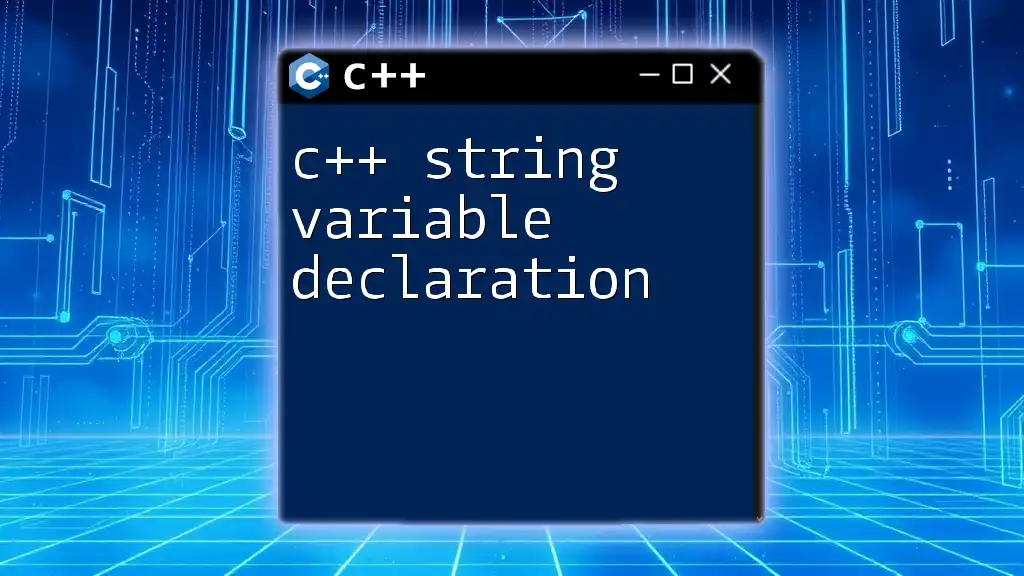
Additional Resources
Recommended Books and Online Resources
To deepen your understanding of atomic variables and concurrency in C++, review the following resources:
- Books on C++ concurrency (such as C++ Concurrency in Action)
- Online tutorials and courses that focus on multi-threading and atomic operations
Community and Support
Join forums and online communities, such as Stack Overflow or Reddit's r/cpp, to share insights and seek assistance on atomic variables and concurrent programming. Engaging with peers can enhance your learning experience and broaden your perspective on best practices.