C++ variable arguments allow functions to accept a variable number of arguments, enabling flexibility in function calls, which can be achieved using the `stdarg.h` library.
#include <iostream>
#include <cstdarg>
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; i++) {
std::cout << va_arg(args, int) << " ";
}
va_end(args);
}
int main() {
printNumbers(5, 1, 2, 3, 4, 5);
return 0;
}
Understanding Variable Arguments in C++
C++ variable arguments allow a function to accept an arbitrary number of arguments. This feature is particularly useful when you want to create functions that can operate with different numbers of inputs without having to overload them all or define numerous variations. Variable arguments enhance flexibility and can lead to more elegant and maintainable code.
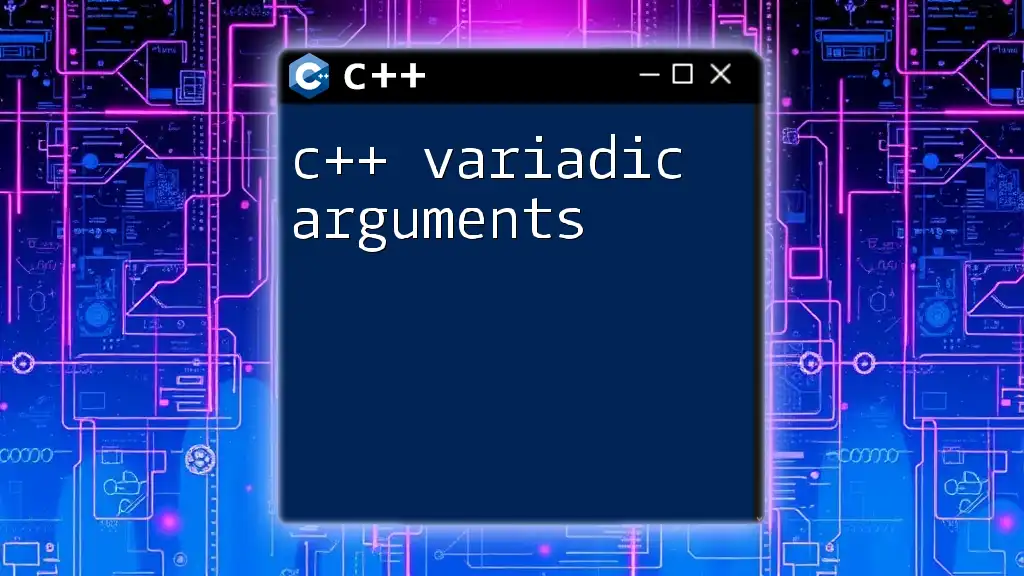
Traditional Functions vs. Functions with Variable Arguments
Traditional function parameters are defined explicitly in the function signature. For example, a function to calculate the sum of two integers would look like this:
int sum(int a, int b) {
return a + b;
}
However, if you wanted to calculate the sum of multiple integers, you would have to create several overloading versions of this function, which can become cumbersome. Variable arguments address this issue by allowing you to write a single function that can handle any number of parameters.
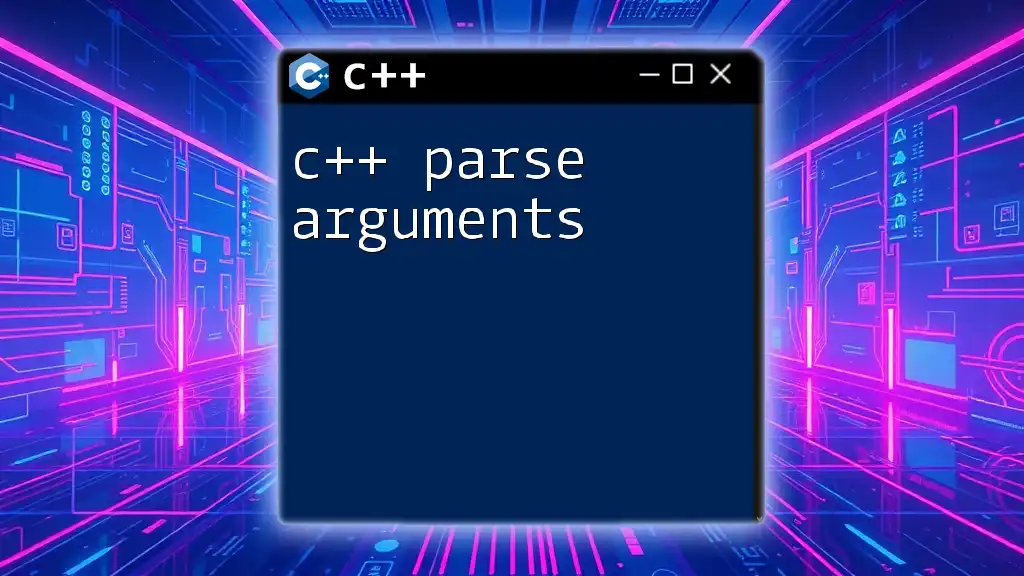
Syntax for Variable Arguments
Using va_list, va_start, va_arg, and va_end (C-style)
The C-style approach for handling variable arguments involves utilizing the `<cstdarg>` header. This method is straightforward but requires careful handling of argument types and counts.
- Include the header: Make sure to include `<cstdarg>` for variable argument processing.
- Set up the function: Define a function that takes an integer count followed by an ellipsis (...) for variable arguments.
- Access arguments: Use `va_list`, `va_start`, `va_arg`, and `va_end` macros to process these arguments.
Here’s a practical example:
#include <iostream>
#include <cstdarg>
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; i++) {
std::cout << va_arg(args, int) << " ";
}
va_end(args);
}
In this function `printNumbers`, we pass a count of how many integers will follow. Inside the function, we initialize a `va_list`, and then loop through the arguments using `va_arg` to access each integer in succession. Finally, `va_end` is called to clean up.
Using Variadic Templates (C++11 and beyond)
C++11 introduced variadic templates, which provide a type-safe and more powerful method to handle variable arguments. They allow you to accept an arbitrary number of arguments of any type, enhancing both flexibility and safety by using template metaprogramming.
Here’s an example of how to utilize variadic templates:
#include <iostream>
template<typename... Args>
void printNumbers(Args... args) {
(std::cout << ... << args) << std::endl;
}
In this code snippet, the `printNumbers` function template takes a parameter pack `Args...` that can accept any number of arguments. The fold expression `(std::cout << ... << args)` effectively prints all the passed arguments sequentially. This solution eliminates the need to manually count and handle different types, leading to cleaner and more maintainable code.
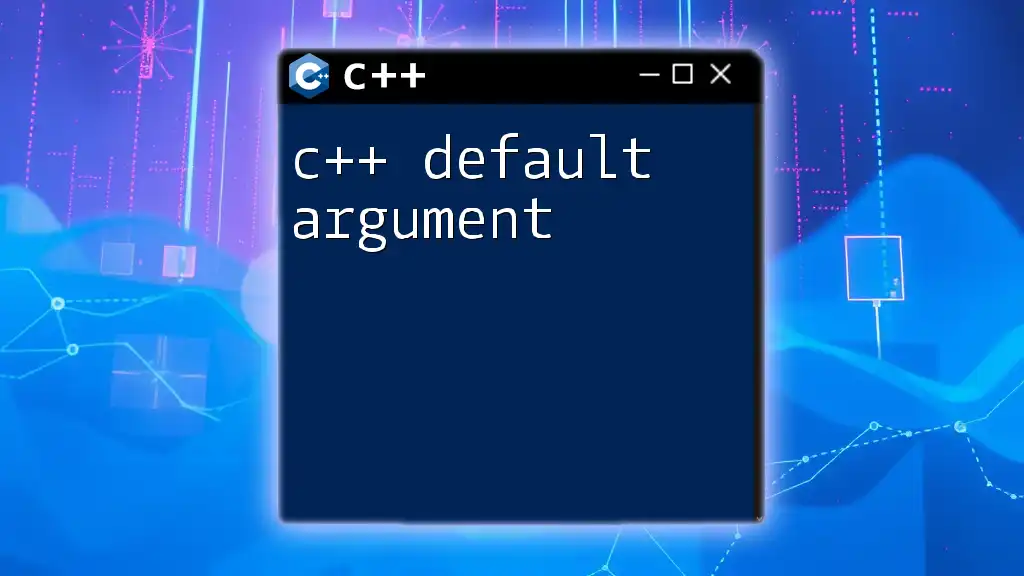
When to Use Variable Arguments
Using C++ variable arguments is beneficial in various scenarios:
- Logger Functions: If the logging mechanism needs to accommodate varying numbers of parameters (e.g., different log levels might require different numbers of context data).
- Mathematical Computations: For functions like `average`, which might be called with a varying number of numerical parameters.
Here’s how a logger function might look using variadic templates:
template<typename... Args>
void log(const std::string& message, Args... args) {
std::cout << message;
(std::cout << ... << args);
std::cout << std::endl;
}
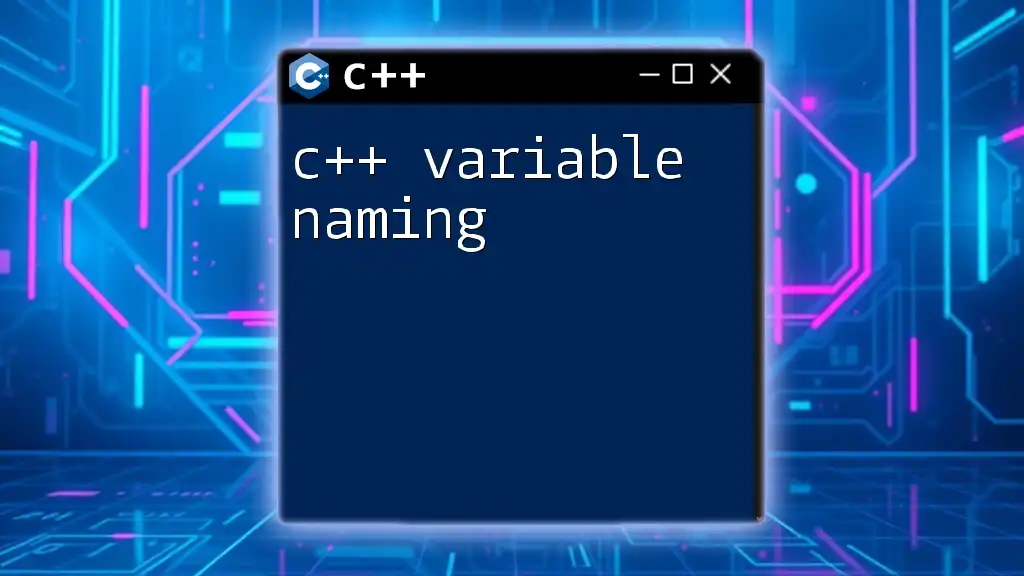
Best Practices for Using Variable Arguments
When working with C++ variable arguments, keeping the following best practices in mind will help maintain code quality:
- Type Safety: Prefer using variadic templates instead of the C-style approach whenever possible, as they ensure type safety and improved readability.
- Readability and Maintainability: Create clear function names and documentation. Complexity increases when handling variable arguments, so strive for clarity in function usage and expectations.
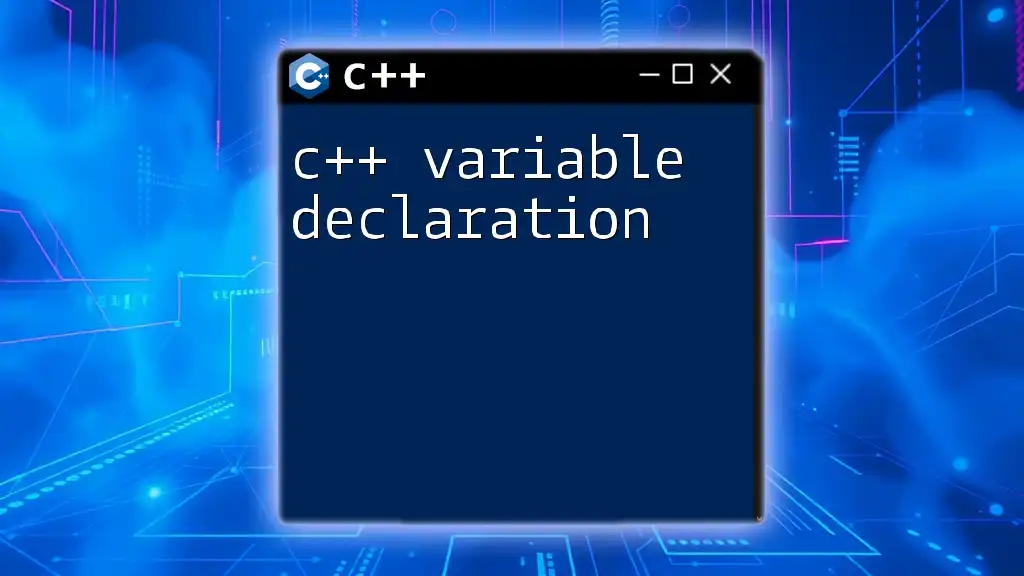
Common Pitfalls & How to Avoid Them
While using C++ variable arguments, several pitfalls may arise:
- Type Confusion: In C-style variable arguments, types are not checked at compile-time. Always ensure that the types are explicitly known to prevent runtime errors.
- Memory Management: Be cautious about dynamically allocated resources. Improper handling of memory can lead to leaks or crashes.
- Recursion with Variable Arguments: Avoid using variable arguments recursively, as it can complicate argument handling and lead to stack overflow.
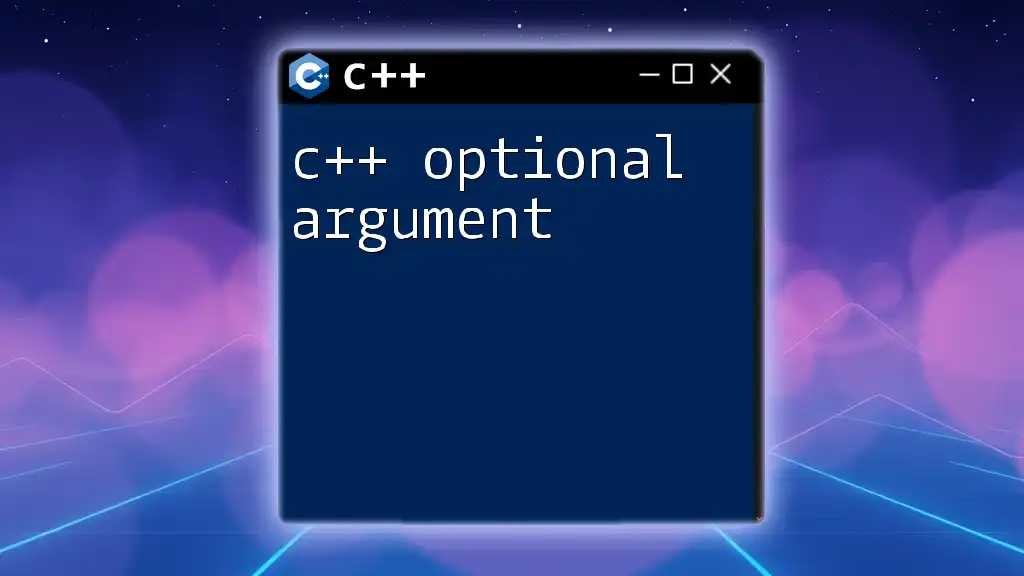
Advanced Use: Combining Variable Arguments with Other Features
Combining variable arguments with lambdas can create powerful, concise functions. Here’s a simple logger function using a lambda that accepts variable arguments:
auto logger = [](const std::string& format, auto... args) {
printf(format.c_str(), args...);
};
This allows you to specify a format similar to that used in C’s `printf`, but customized to your own data structure.
In advanced scenarios, consider leveraging `std::optional` or `std::variant` to improve flexibility and safety around the variable types used.
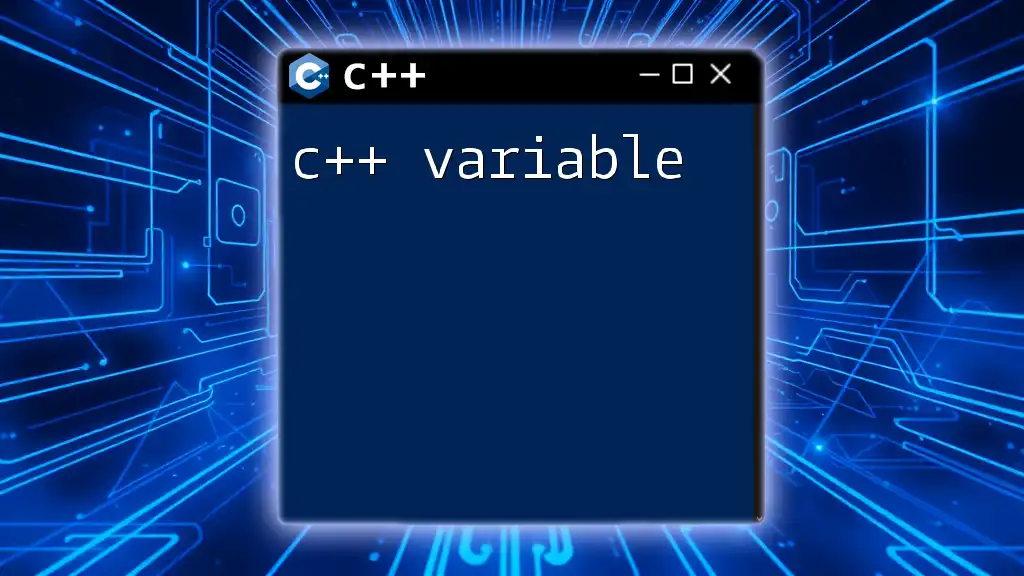
Conclusion
C++ variable arguments empower developers to create flexible and efficient functions that can handle varying numbers of parameters. Whether using the C-style approach or modern variadic templates, understanding how to properly implement and manage variable arguments is critical for any C++ programmer. By adhering to best practices and being aware of common pitfalls, you can harness the full potential of C++ variable arguments in your applications.
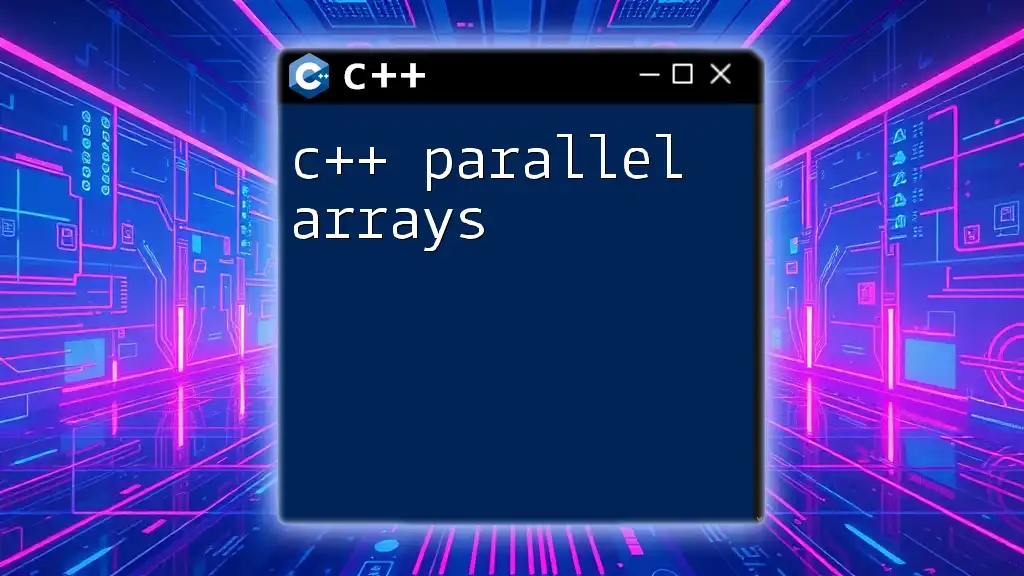
Additional Resources
For further reading, explore more about C++ variable arguments through recommended books and online documentation. Resources such as the official C++ standard library documentation and community-contributed C++ tutorials can provide deeper insights and practical applications.