The `[[maybe_unused]]` attribute in C++ allows you to indicate that a variable, parameter, or function may be intentionally left unused, helping to suppress compiler warnings about unused entities.
[[maybe_unused]] int unusedVariable = 42;
void someFunction([[maybe_unused]] int unusedParam) {
// Function body can ignore the parameter
}
What is [[maybe_unused]]?
The `[[maybe_unused]]` attribute in C++ serves a crucial role in modern programming practices. Introduced in C++17, this attribute allows developers to indicate that certain variables, function parameters, or return values may not be used within the code, thereby suppressing the warnings that compilers typically generate for unused items. This attribute improves the overall readability of the code and supports developers in maintaining a clean and efficient codebase.
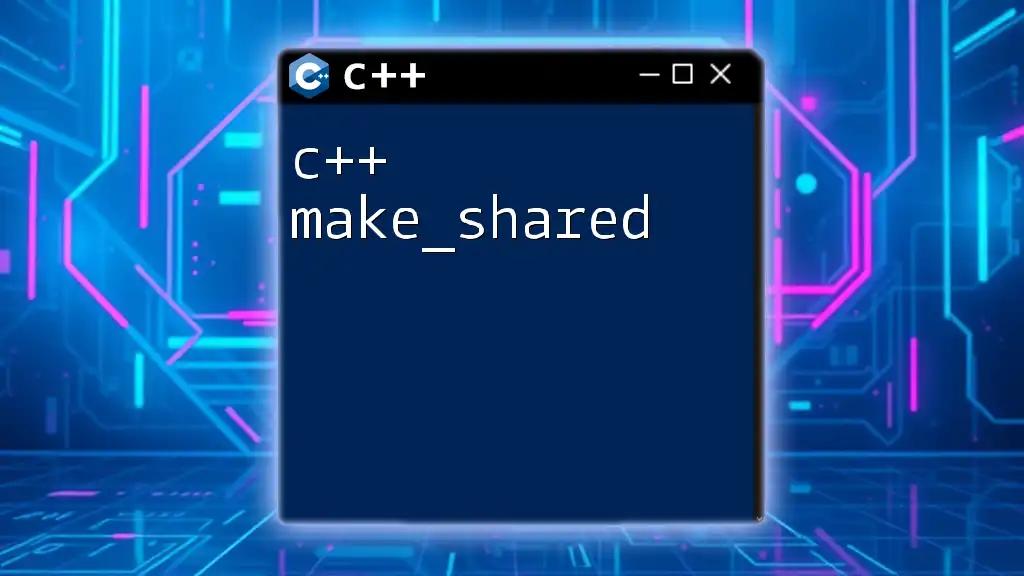
Why Use [[maybe_unused]]?
In many programming scenarios, especially during development or refactoring, certain variables or parameters may remain unutilized. This can happen for reasons such as:
- Incomplete Features: During the development of a new feature, you may leave some parameters unutilized.
- Refactoring Code: After refactoring or updating a function, some parameters may no longer be needed.
- Forward Declaration: In callbacks or API definitions, a parameter might be necessary for interface compatibility, even if it's not directly used.
Using `[[maybe_unused]]` in these situations offers several benefits:
- Cleaner Compiler Output: It helps reduce "noise" from compiler warnings about unused variables, enabling developers to focus on significant warnings.
- Clear Developer Intent: It signals to anyone reading the code that while a parameter or variable is not being used, it may be intentionally left in place for a specific reason.
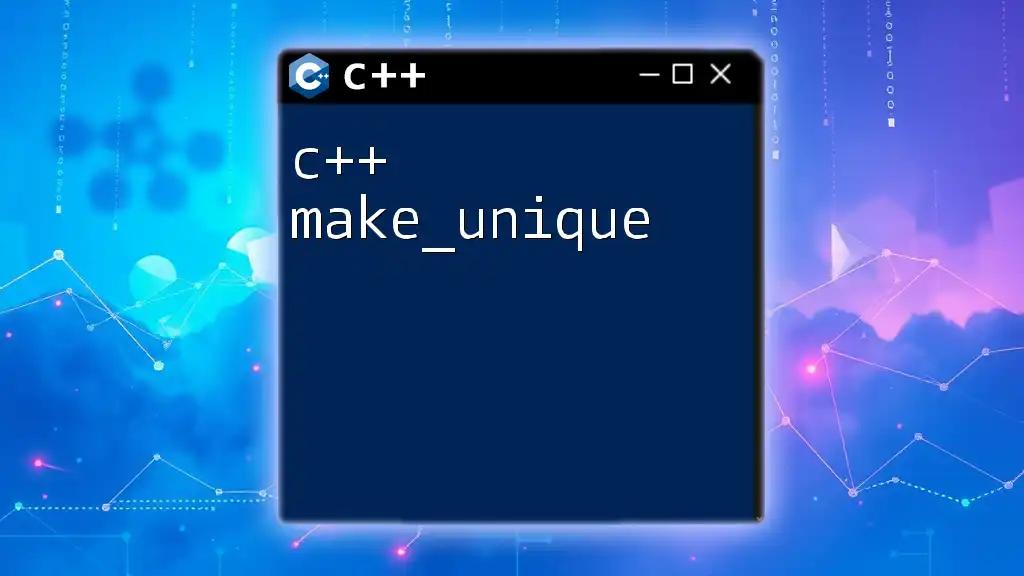
Understanding C++ Attributes
Overview of Attributes in C++
Attributes in C++ are annotations that provide additional information to the compiler. They are useful for influencing compiler behavior or conveying developer intent. Unlike traditional macros, attributes enable more expressive and less error-prone code.
Common C++ Attributes
Some of the widely used attributes include:
- `[[nodiscard]]`: Prevents the compiler from discarding the return value of a function.
- `[[deprecated]]`: Marks a feature as outdated, encouraging developers to avoid using it.
- `[[maybe_unused]]`: Indicates that a variable or parameter might be unused intentionally.

Usage of [[maybe_unused]]
Syntax of [[maybe_unused]]
The basic syntax for `[[maybe_unused]]` is straightforward. It can be applied to variables, function parameters, and return types. Here’s an example of applying it to a variable:
[[maybe_unused]] int unusedVariable = 42;
Examples of [[maybe_unused]] in Practice
Example 1: Unused Function Parameters
Sometimes, when creating functions, you may have parameters that are logically part of the function's interface but are not used in the body. In such cases, you can use `[[maybe_unused]]` to prevent unnecessary warnings:
void processData([[maybe_unused]] int id, int value) {
// We can use value here, but id is left unused.
}
This shows that while `id` isn't needed for the function's operation, it is included for structural purposes.
Example 2: Unused Return Values
When designing utility functions, the return values may not always be needed in every context. Using `[[maybe_unused]]` allows for such flexibility without triggering compiler warnings:
[[maybe_unused]] int computeSum(int a, int b) {
return a + b;
}
This way, if the caller chooses to discard the result of `computeSum`, it won’t clutter the compiler output with warnings.
Example 3: Struct and Class Members
In classes or structs, it’s common to have members that might not always be used. The `[[maybe_unused]]` attribute can be applied to these members as follows:
struct Example {
[[maybe_unused]] int unusedMember;
int usedMember;
};
This highlights that the struct has an optional member that might not be relevant for all instances.

Impact on Code Quality
Reducing Compiler Warnings
Utilizing `[[maybe_unused]]` effectively minimizes the clutter of compiler warnings related to unused entities. Rather than sifting through a multitude of warnings, developers can focus on critical issues that impact functionality and performance.
Enhancing Code Readability
Using `[[maybe_unused]]` communicates clear intent to fellow developers. It portrays that the developer acknowledges the existence of unused parameters or variables but has chosen to retain them for clarity, future use, or interface compatibility. This practice fosters a collaborative environment, allowing team members to understand the reasoning behind code choices more intuitively.
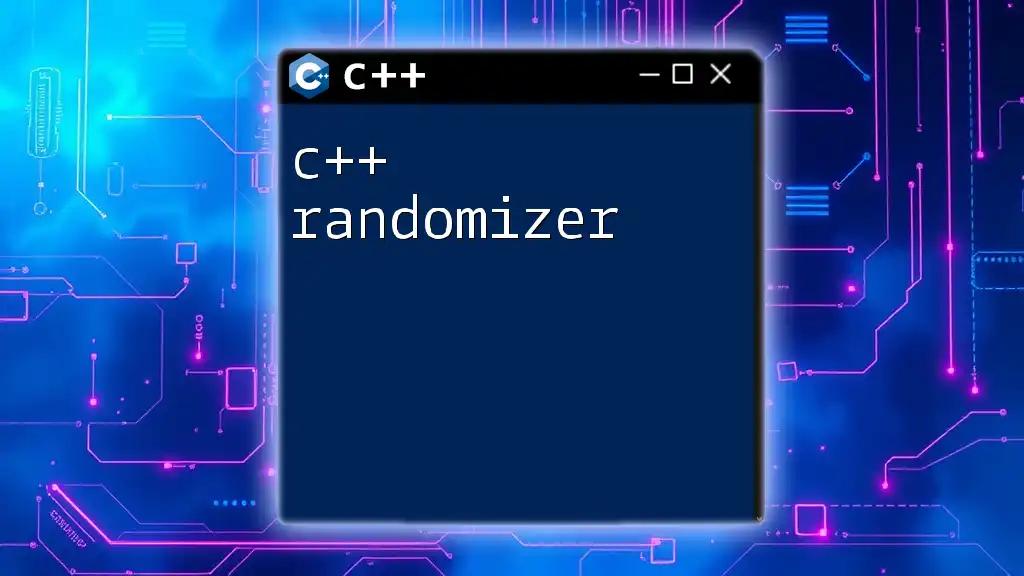
Best Practices for Using [[maybe_unused]]
When to Use [[maybe_unused]]
The `[[maybe_unused]]` attribute should be used judiciously. Here are some guidelines to consider:
- Purposeful Unused Items: If an item is left unused as part of accepted design patterns, such as interfaces, it’s appropriate to use this attribute.
- During Development: It can be beneficial in experimental code or during the early stages of development when the final shape of the code may not yet be defined.
Common Pitfalls to Avoid
One potential pitfall is the misuse or overapplication of `[[maybe_unused]]`. It’s essential not to blanket apply this attribute across the board without understanding the implications. For example, applying it liberally can lead to confusion or hide genuine issues:
[[maybe_unused]] void functionWithUnnecessaryAttribute() {
// Avoid cluttering your code without reason
}
Instead, focus on meaningful applications only where necessary.
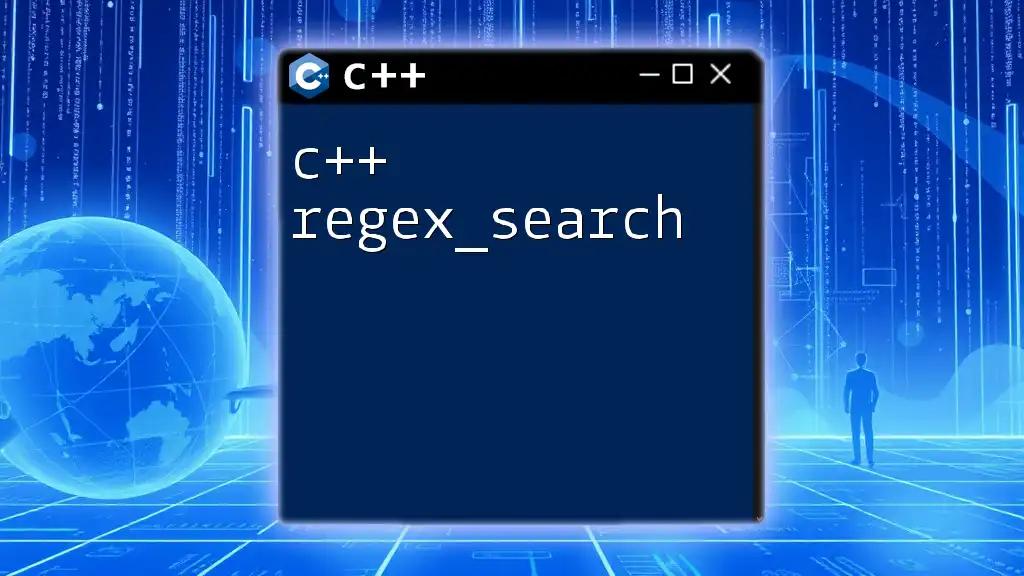
Compatibility and Compiler Support
Compiler Support for [[maybe_unused]]
The `[[maybe_unused]]` attribute is supported in C++17 and later. It is compatible with major compilers such as GCC, Clang, and MSVC, making it widely applicable in modern C++ development.
Performance Considerations
In terms of performance, using `[[maybe_unused]]` has negligible overhead. The attribute does not change the runtime behavior of the code; it solely serves as a tool for conveying developer intent and managing compiler warnings.
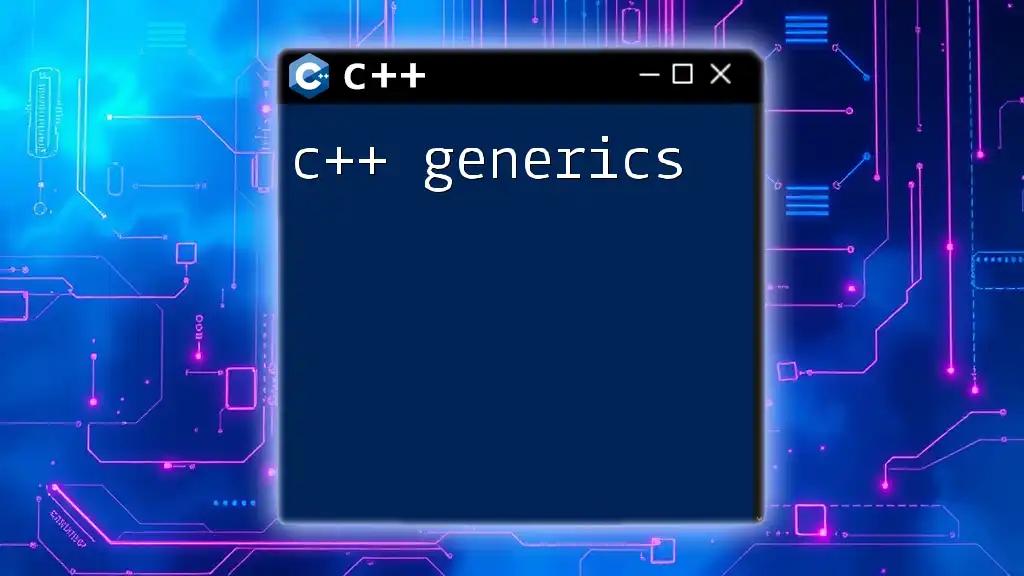
Conclusion
In conclusion, the `[[maybe_unused]]` attribute in C++ is a valuable tool for developers aiming to maintain clean code while conveying intent about unused variables, parameters, or return values. By leveraging this attribute, developers can eliminate unnecessary compiler warnings and enhance the code's readability and maintainability. As you become more familiar with C++ and its attributes, consider adopting `[[maybe_unused]]` in your projects for improved code clarity and professionalism.
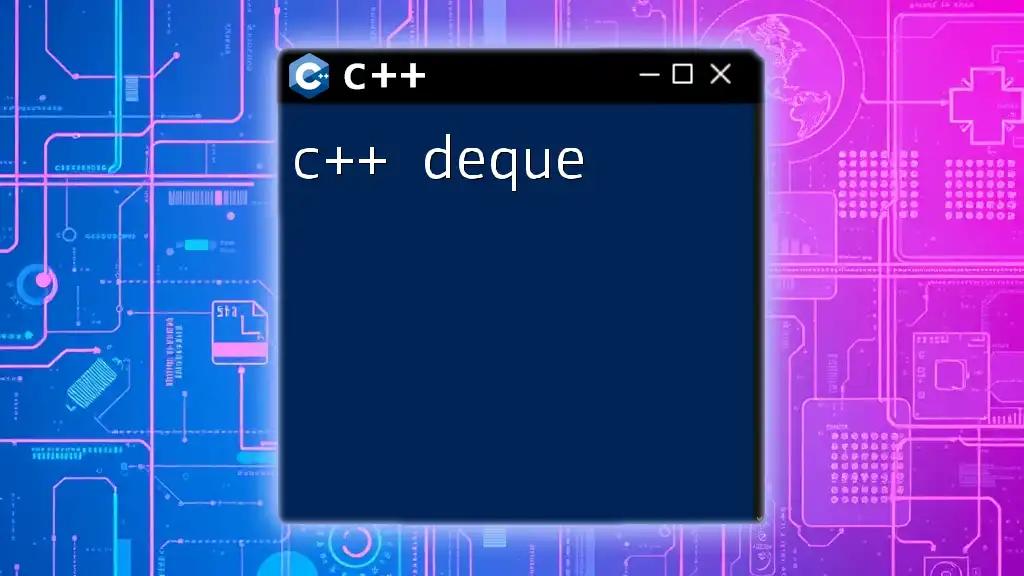
Call to Action
We encourage you to share your experiences with `[[maybe_unused]]` in your own coding projects. Have you found it beneficial, or do you have tips to optimize its use? Let us know your thoughts or questions as you explore this useful C++ feature further.
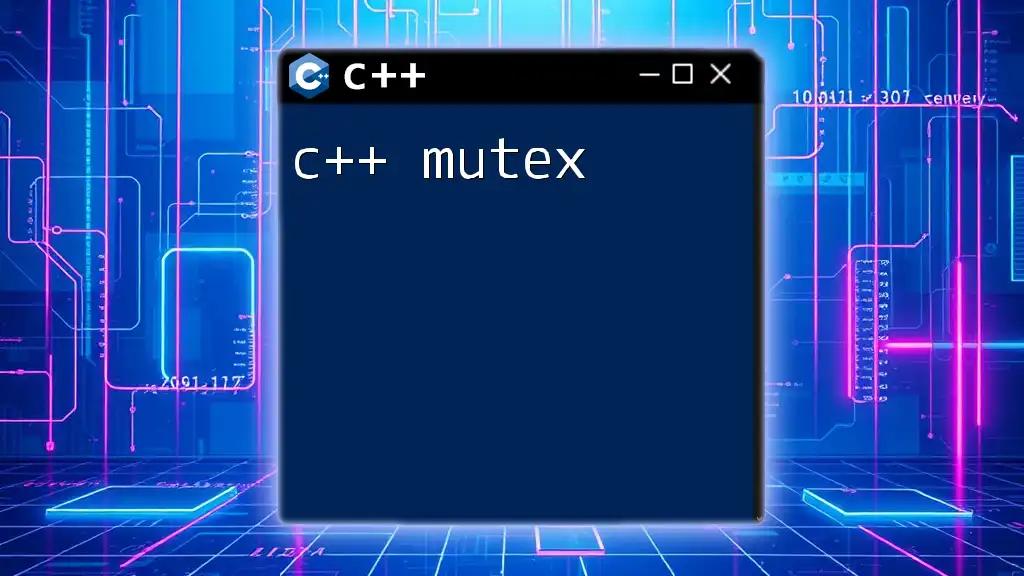
Additional Resources
For further reading and a deeper dive into C++ attributes and best coding practices, check out the official C++ documentation, online programming forums, and courses that focus on modern C++ techniques.