C++ variadic arguments allow functions to accept an arbitrary number of arguments, enabling more flexible function calls.
Here's a simple example:
#include <iostream>
#include <cstdarg>
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; i++) {
std::cout << va_arg(args, int) << " ";
}
va_end(args);
}
int main() {
printNumbers(5, 1, 2, 3, 4, 5); // Output: 1 2 3 4 5
return 0;
}
What are Variadic Arguments?
Variadic arguments are a unique feature in C++ that allows functions to accept a variable number of parameters. Unlike regular functions, which require a predefined number of arguments, functions with variadic arguments can adapt to the needs of the caller. This flexibility can be particularly useful in scenarios where the quantity of input parameters may vary, such as in logging, mathematical operations, or when interfacing with a wide range of user inputs.
Use Cases of Variadic Arguments
- Logging Functions: Log messages that may vary in verbosity.
- Mathematical Functions: Perform calculations on a varying number of inputs.
- Dynamic Event Handlers: Respond to different events or user actions.
By leveraging variadic arguments, developers can write cleaner, more maintainable code, reducing redundancy and enhancing scalability.
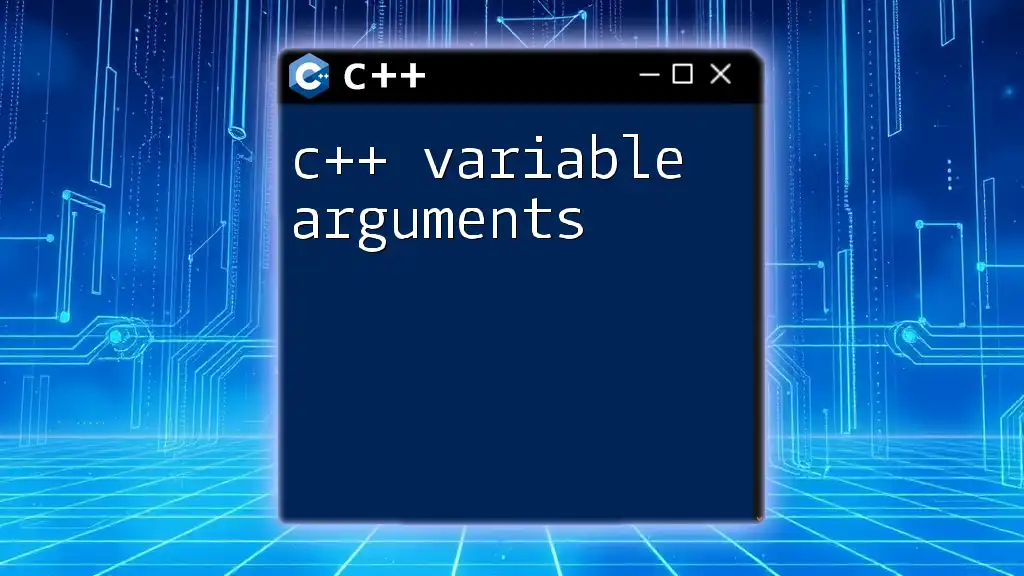
Understanding Variadic Functions
What is a Variadic Function?
A variadic function is a function that can take a variable number of arguments. In C++, this is represented using the ellipsis (`...`) syntax. When a function is defined to accept variadic arguments, it can work with differing amounts of input without prior specification.
Examples of Variadic Functions
A classic example is the `printf` function in C, which can print different types and numbers of arguments:
#include <cstdio>
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for(int i = 0; i < count; ++i) {
printf("%d ", va_arg(args, int));
}
va_end(args);
printf("\n");
}
In this code, `printNumbers` takes an integer `count` followed by a variable number of integer arguments. The `va_list`, along with `va_start()` and `va_end()`, are used to manage the arguments safely. The `va_arg()` macro is then utilized to iterate through each argument provided.
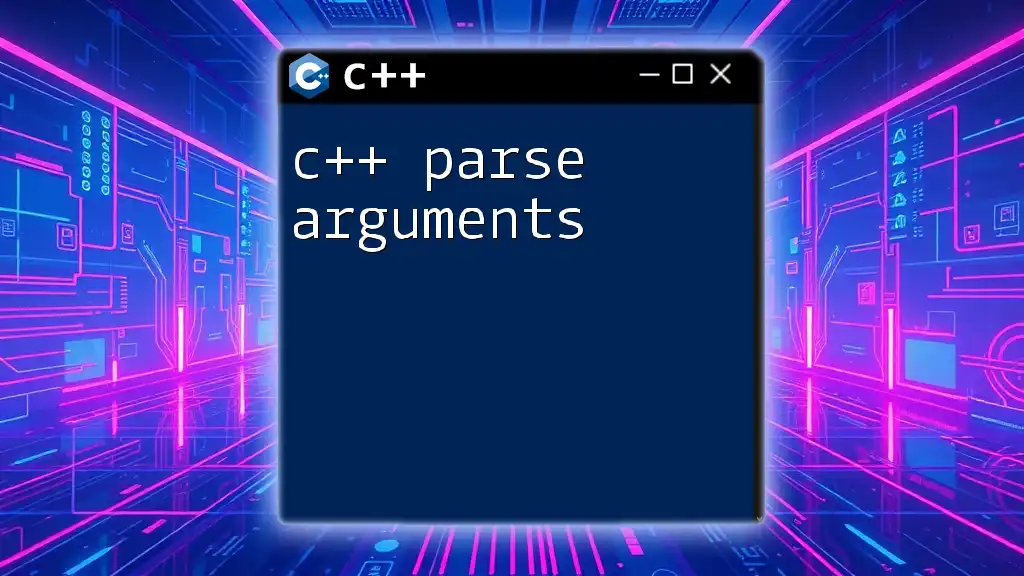
Using Variadic Templates
Introduction to Variadic Templates
Variadic templates are a powerful feature in C++14 and later that extend the concept of variadic arguments to templates. This allows functions and classes to accept a variable number of template parameters.
Creating a Variadic Template Function
Consider how variadic templates enable more concise and type-safe functions. Here’s an example of a function that sums a variable number of parameters using a fold expression:
template<typename... Args>
int sum(Args... args) {
return (args + ...); // Fold expression
}
In this example, `sum` can accept any number of arguments and summing them is achieved through a fold expression, which calculates the total in a single line of code. This not only reduces complexity but also enhances code clarity.
Explanation of Fold Expressions
Fold expressions are a C++17 feature that significantly simplifies the processing of variadic parameters. They allow developers to operate on a parameter pack in a straightforward manner, such as summing or multiplying values. This leads to concise and intuitive code that is easier to read and maintain.
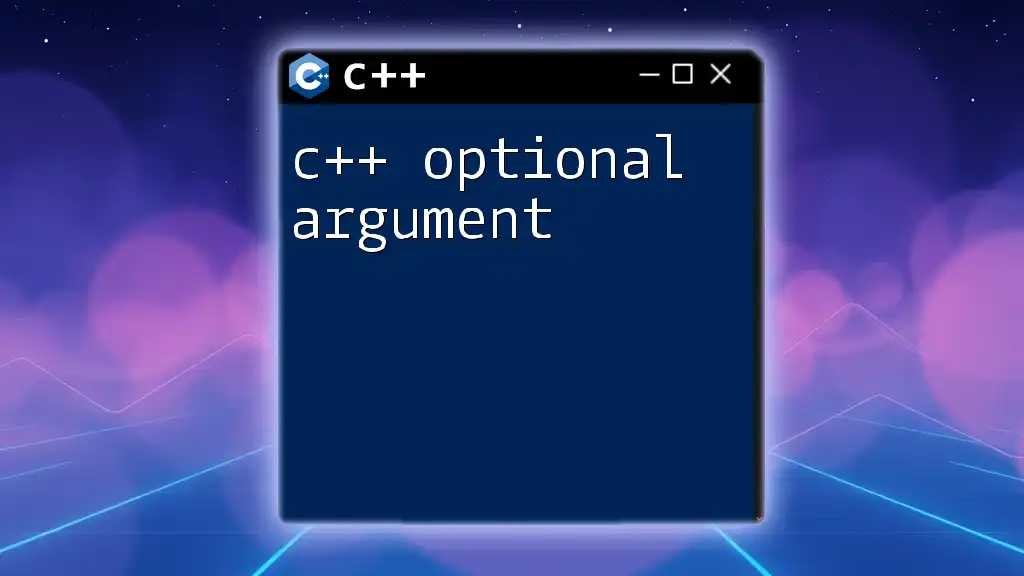
Benefits of Using Variadic Arguments
Flexibility in Function Design
The primary advantage of using C++ variadic arguments is their inherent flexibility. They allow functions to accept a dynamic number of parameters, making them ideal for scenarios where the amount of input is uncertain.
Code Reduction and Reusability
By adopting variadic arguments, developers can substantially reduce code duplication. Instead of creating multiple overloaded functions tailored for different parameter counts, a single variadic function can handle various needs. This streamlines code maintenance.
Dynamic Behavior in Functions
Variadic functions can modify their behavior based on the input they receive. This dynamic nature enables sophisticated applications where adaptability is crucial. Consider a logging system that tailors its output based on the number of details specified by the user.
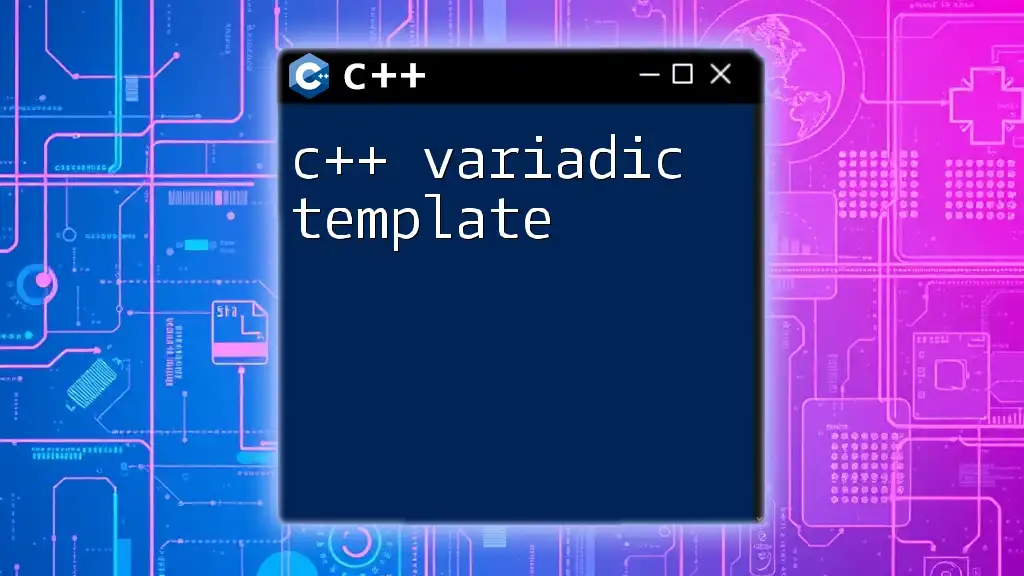
Limitations and Considerations
Drawbacks of Variadic Functions
While C++ variadic arguments offer versatility, they come with drawbacks. Unlike traditional parameters, variadic arguments lack compile-time type checking, leading to potential runtime errors.
Static vs Dynamic Type Checking
Regular functions benefit from static type checking—each argument is checked against the expected type at compile time. With variadic functions, this type safety is diminished as the function must handle potentially different types at runtime.
Best Practices
To effectively use variadic arguments, consider the following best practices:
- Limit the Use: Use them judiciously to maintain clarity.
- Maintain Clear Documentation: Ensure that the purpose and use of each variadic function are documented clearly to avoid misuse.
- Leverage Variadic Templates: When appropriate, use variadic templates for added type safety and clarity.
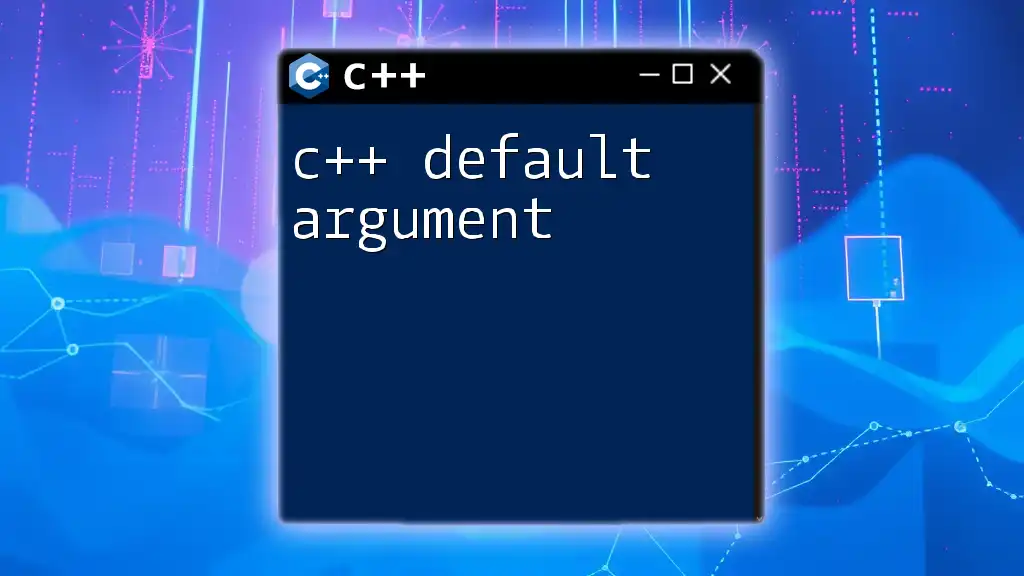
Practical Examples and Use Cases
Building a Logger with Variadic Arguments
A practical example of C++ variadic arguments is creating a logging function:
void log(const char* format, ...) {
va_list args;
va_start(args, format);
vprintf(format, args);
va_end(args);
}
In this example, the `log` function allows for formatted logging, similar to `printf`, with the added flexibility of passing in various types and numbers of arguments. This enhances the usefulness of the logging function by accommodating different logging requirements seamlessly.
Variadic Methods in Real-World Libraries
Many standard C++ libraries, including features like `std::initializer_list`, utilize variadic templates to simplify operations on collections or to create flexible APIs. Understanding how these libraries implement variadic templates can provide deeper insight into writing cleaner, more efficient code.
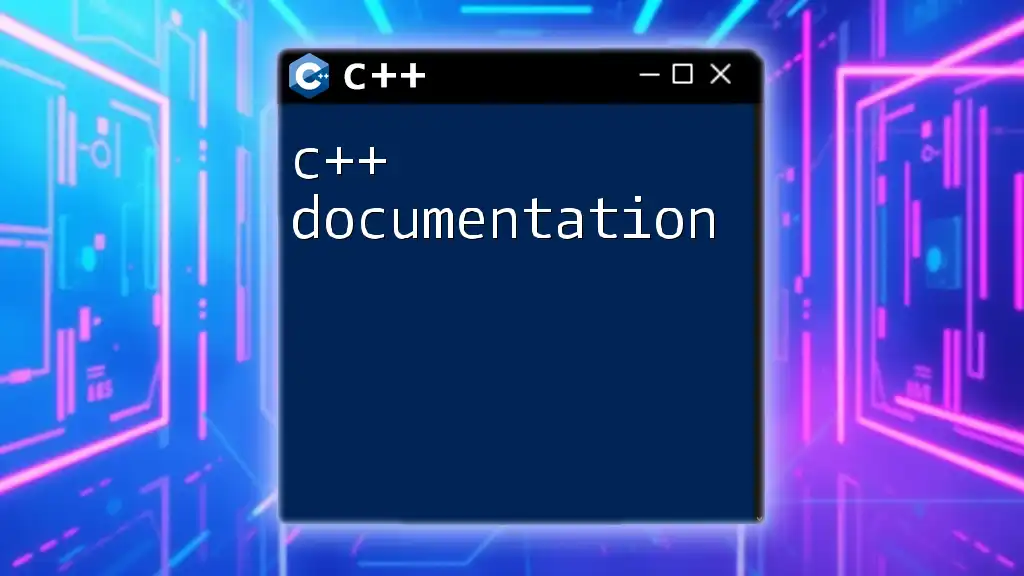
Conclusion
Mastering C++ variadic arguments empowers developers to write versatile and maintainable code. Through the understanding of variadic functions, templates, and practical applications, programmers can harness the full potential of this powerful feature in their C++ applications. By embracing the flexibility and scalability that variadic arguments offer, you can enhance your coding skills and deliver robust solutions.
Call to Action
Have you experimented with variadic arguments in your code? Share your experiences or questions in the comments below! For those keen to learn more, stay tuned for additional articles on C++ programming techniques and best practices.
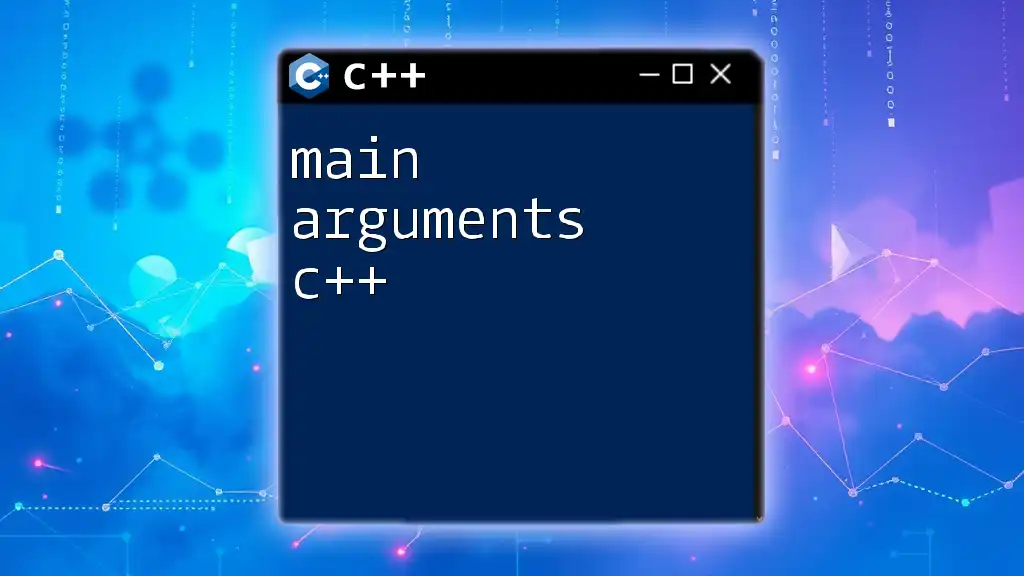
Additional Resources and References
To further your understanding of C++ variadic arguments, consider exploring:
- C++ documentation and tutorials
- Advanced C++ programming books
- Online forums for discussion and troubleshooting with fellow C++ enthusiasts