In C++, a default argument allows a function to be called with fewer arguments than it is defined to accept, automatically using the specified default values for any omitted arguments.
Here's a code snippet demonstrating this concept:
#include <iostream>
using namespace std;
void greet(string name, string greeting = "Hello") {
cout << greeting << ", " << name << "!" << endl;
}
int main() {
greet("Alice"); // Uses default greeting
greet("Bob", "Hi"); // Uses custom greeting
return 0;
}
Understanding Default Arguments in C++
What is a Default Argument?
A default argument is a value provided in a function definition. If the caller does not provide a value for that parameter when calling the function, the default value will be used. This feature simplifies function calls and can make function signatures cleaner and more readable.
Syntax of Default Arguments
To specify a default argument, you assign a value to the parameter in the function declaration. The syntax looks like this:
return_type function_name(parameter_type parameter_name = default_value);
How Default Arguments Work
When a function with default arguments is called, C++ checks if an argument is supplied. If not, the default value is automatically used. This allows for more flexible function calls where handlers can be invoked with fewer parameters.
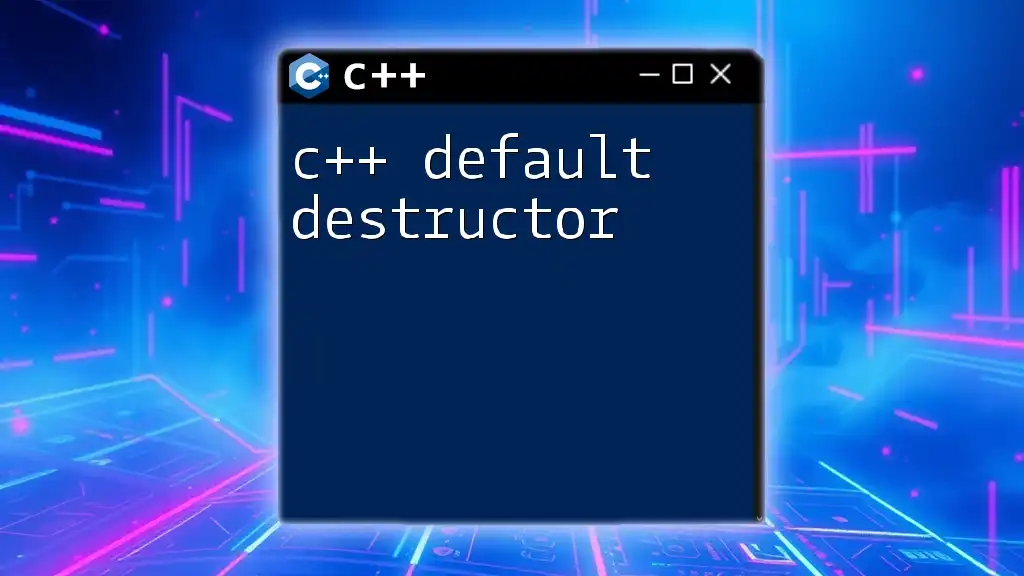
Benefits of Using Default Arguments in C++
Enhancing Code Readability
Default arguments help to keep function calls concise and readable. This clarity fosters better understanding among programmers who read the code later. For example, rather than overloading a function for every single parameter combination, a single function with default arguments can serve multiple purposes.
Reducing Function Overloading
By using default arguments, programmers can reduce the number of overloaded functions. This can simplify the codebase significantly, making it easier to maintain and understand.
Simplifying Function Calls
Functions with default arguments allow users to omit parameters that are not critical for every call. This flexibility promotes cleaner and more manageable client code.
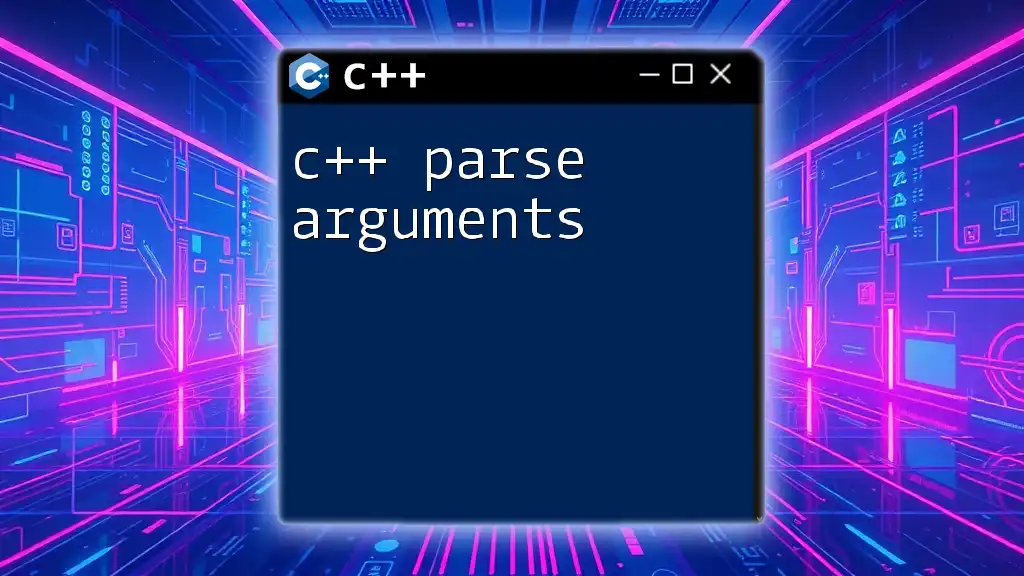
How to Use Default Arguments in C++
Setting Default Values
To set a default value for a function parameter, you simply specify the default when declaring the function.
Example: Basic Function with Default Argument
void display(int num = 10) {
cout << "Number: " << num << endl;
}
In this example, if you call `display()` without an argument, it will output `Number: 10`. If you call it with a specific value, that value will replace the default.
Multiple Default Arguments
You can set default values for multiple parameters in a single function.
Example: Function with Multiple Default Arguments
void show(int a = 1, float b = 2.5) {
cout << "Integer: " << a << ", Float: " << b << endl;
}
Calling `show();` will result in `Integer: 1, Float: 2.5`. Calling it with one argument, like `show(5);`, will output `Integer: 5, Float: 2.5`. Providing two arguments will completely override the defaults.
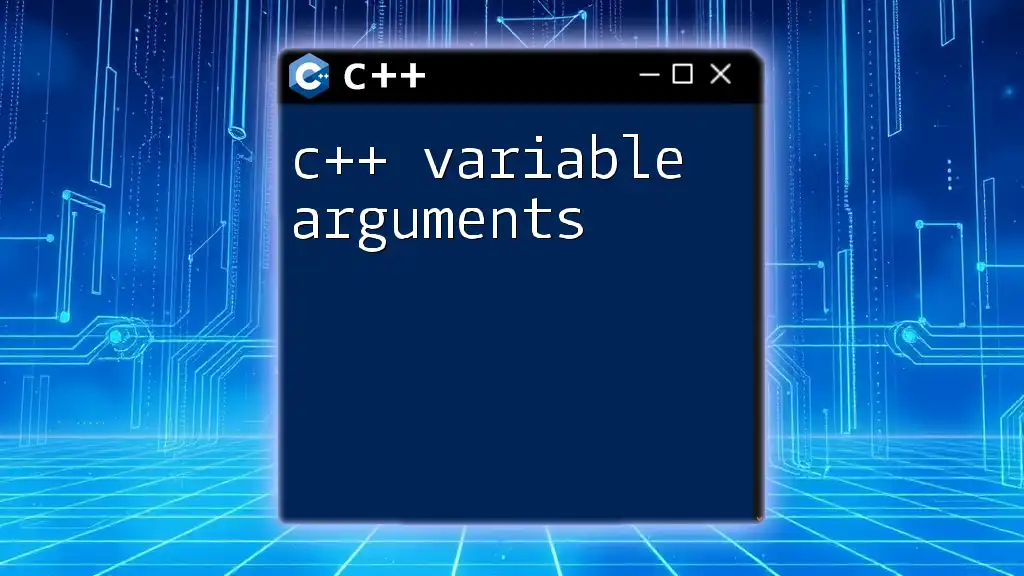
Rules and Best Practices for Default Arguments
Rule 1: Default Arguments Should Appear Only Once
Make sure each default argument is specified only once in the function (preferably in the header).
Example and Explanation
void func(int a, int b = 5); // Correct
void func(int a = 2, int b); // Incorrect
The first declaration is valid because `b` has a default value while `a` is required. The second declaration is invalid because `a` cannot be called without a specific value when `b` is defaulted.
Rule 2: Order of Parameters
The order of parameters matters. Default arguments should always be placed at the end of the parameter list.
Example: Function with Mixed Parameters
void example(int x, int y = 0, int z = 1); // Correct
In this case, `y` and `z` have default values, while `x` must be provided by the caller.
Rule 3: Default Arguments in Function Overloading
When overloading functions, be cautious with the use of default arguments, as it can lead to ambiguity.
Example: Function Overloading with Default Arguments
void test(int a); // Function 1
void test(int a, int b = 10); // Function 2
In this case, if you call `test(5);`, it is clear which function is invoked. However, if the second function is omitted, the caller might face confusion.
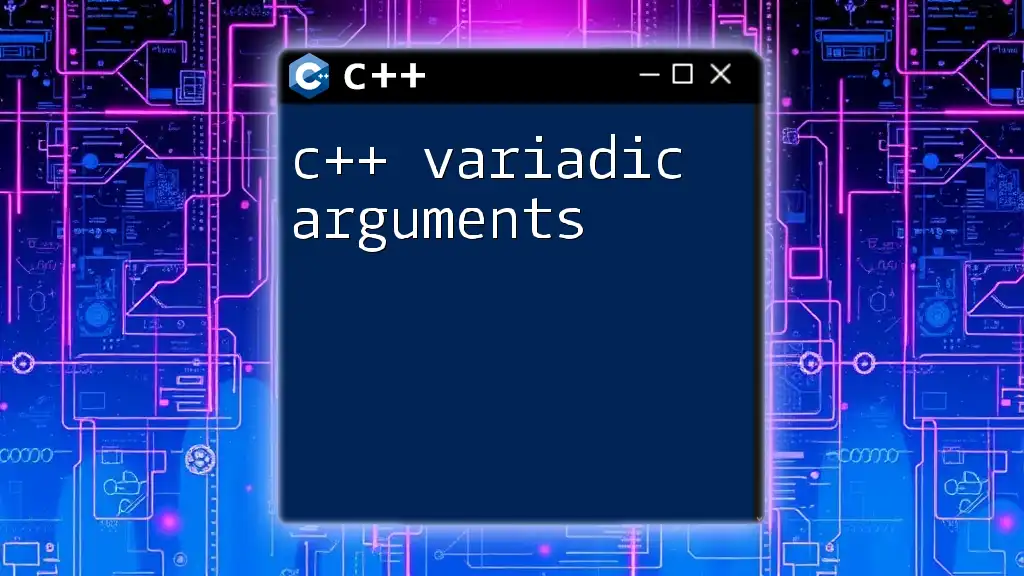
Common Pitfalls to Avoid
When dealing with C++ default arguments, awareness of potential pitfalls is crucial. One common issue is accidental override. In a case where the caller provides a value that conflicts with default values, programmers may inadvertently receive unexpected results.
For instance:
void calculate(int a, int b = 2); // Default argument
calculate(5); // Calls calculate(5, 2)
calculate(5, 3); // Calls calculate(5, 3), overriding b
Another pitfall relates to confusion arising from function overloading in the context of default arguments. This can lead to ambiguous calls if not handled carefully.
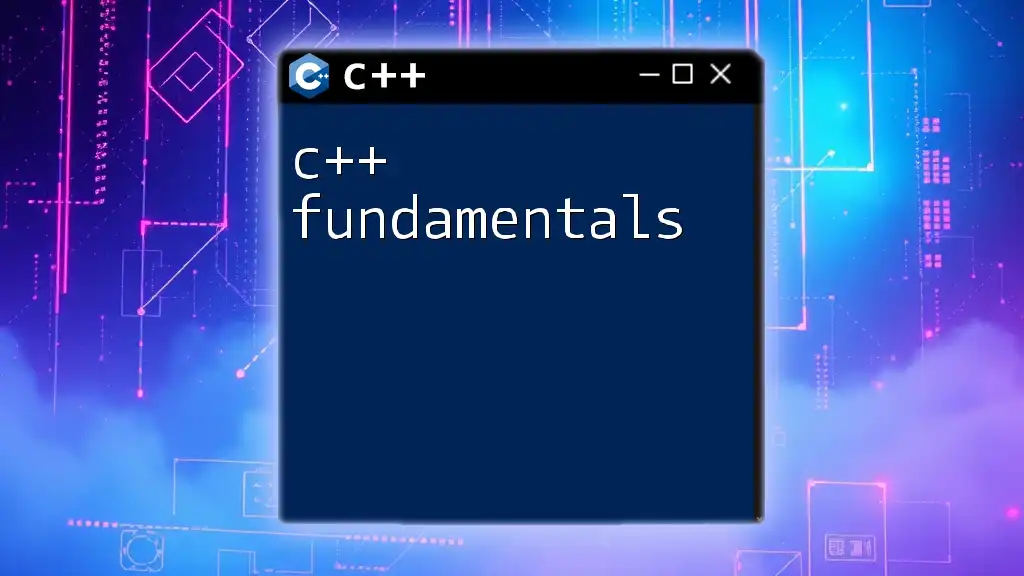
Real-world Applications of Default Arguments
Simplifying API Design
APIs utilize default arguments for functions that might have extensive configurations. By enabling default parameters, API developers create elegant, user-friendly function signatures. This design decision allows users to focus on essential parameters rather than getting bogged down by numerous options.
Implementing Default Configurations
Libraries and frameworks often leverage default arguments to establish sensible defaults. For instance, a graphics library might offer a `draw()` function with defaults like color or line thickness to streamline drawing operations without excessive configuration.
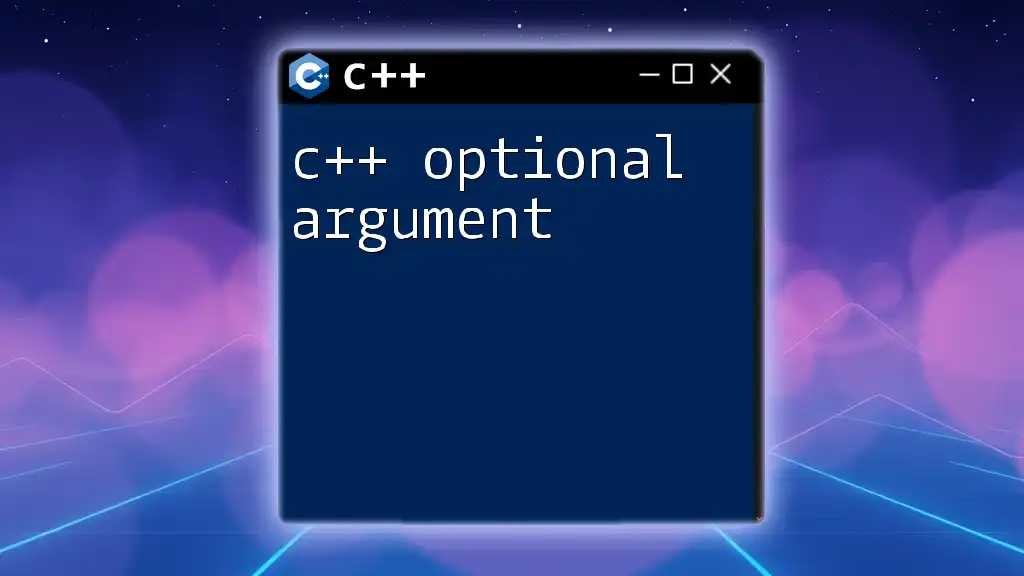
Conclusion
In summary, utilizing C++ default arguments can vastly improve the readability and maintainability of your code. By understanding the syntax and rules governing their use, you can simplify function calls, minimize overloading, and enhance your coding practice. Default arguments are a powerful feature that, when effectively employed, can enhance both your programming efficiency and code clarity.
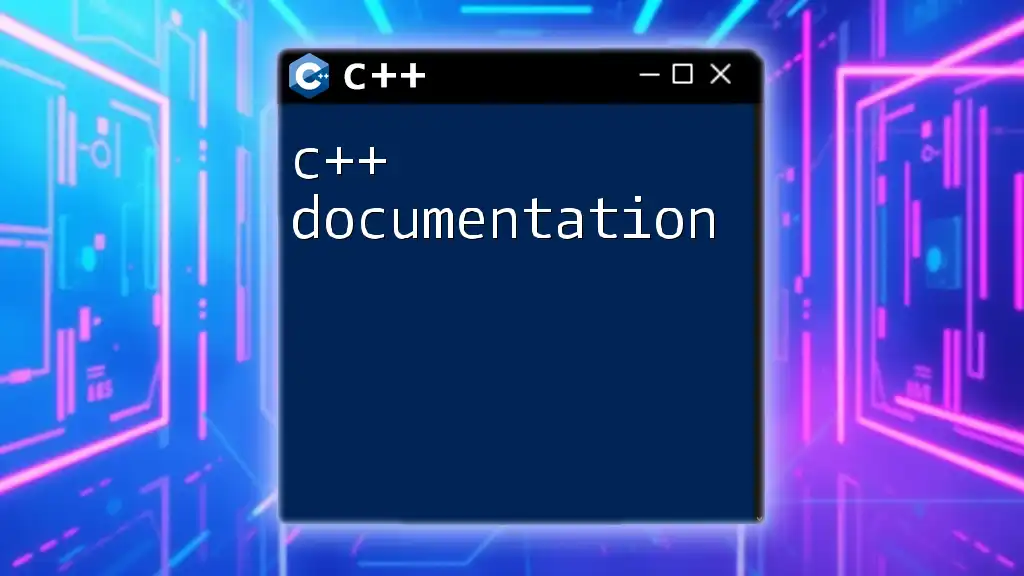
Additional Resources
For those eager to delve deeper into the subject of default arguments, consider exploring the official C++ documentation and various programming literature that offers valuable insights into best practices and advanced usage.