In C++, a `std::promise` is a mechanism that allows you to set a value or an exception from one thread and retrieve it in another thread through a `std::future`.
#include <iostream>
#include <thread>
#include <future>
void setPromise(std::promise<int>& p) {
p.set_value(42); // Set the value in the promise
}
int main() {
std::promise<int> promise;
std::future<int> future = promise.get_future();
std::thread t(setPromise, std::ref(promise)); // Create a thread to set the promise value
t.join(); // Wait for the thread to finish
std::cout << "Promise value: " << future.get() << std::endl; // Retrieve the value from future
return 0;
}
What is a Promise?
A promise in C++ is an abstraction used to manage asynchronous operations. It acts as a placeholder for a value that will be made available in the future. The primary purpose of a promise is to provide a way to retrieve the result of an operation that has not yet completed. Promises are particularly powerful in scenarios where tasks can run concurrently, allowing your program to continue working without blocking.
Why Use Promises in C++?
The use of promises enables developers to write more manageable asynchronous code. When compared to traditional threading models, promises offer several advantages:
- Simpler Code Structure: Promises eliminate the need for intricate callback functions, making the flow of your code more straightforward.
- Error Handling: Promises facilitate a clean way to handle exceptions in asynchronous operations, allowing you to manage errors at a higher level of abstraction.
- Concurrency Support: Promises integrate well with C++'s threading features, supporting modern multi-threaded programming styles.

Understanding the Basics of C++ Promises
What Happens When You Create a Promise?
When you create a promise in C++, it can exist in one of three states:
- Pending: The initial state, where the outcome (value or exception) is not yet available.
- Fulfilled: The state when the promise has successfully resolved with a value.
- Rejected: The state when the promise has been completed with an error.
Promise Syntax in C++
The syntax for creating a promise is straightforward. Here’s a basic example of promise creation:
#include <future>
std::promise<int> prom;
std::future<int> fut = prom.get_future();
This snippet demonstrates how to create a promise of type `int` and obtain a future object tied to the promise. The future can later be used to retrieve the value set by the promise.

How Promises Work Under the Hood
The Lifecycle of a Promise
The lifecycle of a promise can be visualized as a state machine transitioning between its three states. When you first create a promise, it starts in the pending state. As you call `set_value()` or `set_exception()`, it transitions to either fulfilled or rejected. This behavior allows you to manage the outcome of asynchronous operations systematically.
Associated Futures
Futures are directly tied to promises. They are used to access the value once the promise is fulfilled. Here’s an example:
prom.set_value(10);
std::cout << "Future value: " << fut.get() << std::endl; // Outputs 10
In this example, the promise is fulfilled with the value `10`, which can then be retrieved using the future's `get()` method.

Creating and Managing Promises
How to Create a Promise
Creating a promise involves declaring an instance and associating it with a future. This lifecycle ensures that whenever the promise's state changes, the future can access the new state.
Setting Values and Errors
You can fulfill a promise with a value or handle an error. Here's how you might manage an exception:
try {
prom.set_value(20);
} catch (...) {
prom.set_exception(std::current_exception());
}
This example sets a value for the promise. In the event of an error, it utilizes `set_exception()` to store the current exception, allowing for better error handling later.

Using Promises for Asynchronous Programming
Combining Promises with Threads
Promises shine in asynchronous programming, especially when combined with threads. By utilizing promises, you can fetch data or perform computations without blocking the main thread. Here’s an illustrative example:
std::thread t([&prom]() {
try {
// Simulating long computation
std::this_thread::sleep_for(std::chrono::seconds(1));
prom.set_value(30);
} catch (...) {
prom.set_exception(std::current_exception());
}
});
In this code snippet, a new thread is launched to perform a long-running task. After a delay, it fulfills the promise, allowing the main thread to move forward without waiting for the computation.
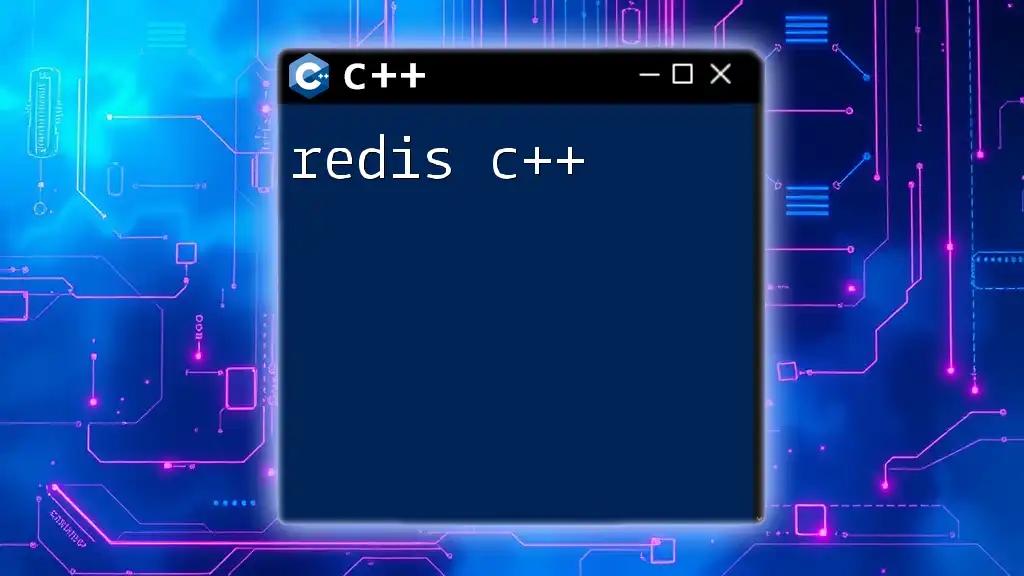
Advanced Concepts of C++ Promises
Chaining Promises
Chaining is a powerful concept involving multiple promises where one promise’s fulfillment leads to the initiation of another. This technique allows for complex workflows to be constructed with relative ease.
Combining Promises with `async`
`std::async` is a utility that allows you to run tasks asynchronously and can be used effectively with promises. Here’s how you might use them together:
auto result = std::async(std::launch::async, []() {
// Long task
return 42;
});
The `std::async` function runs a task in a separate thread, and the result can be accessed directly through it, providing a convenient way to manage asynchronous operations without explicit promise management.
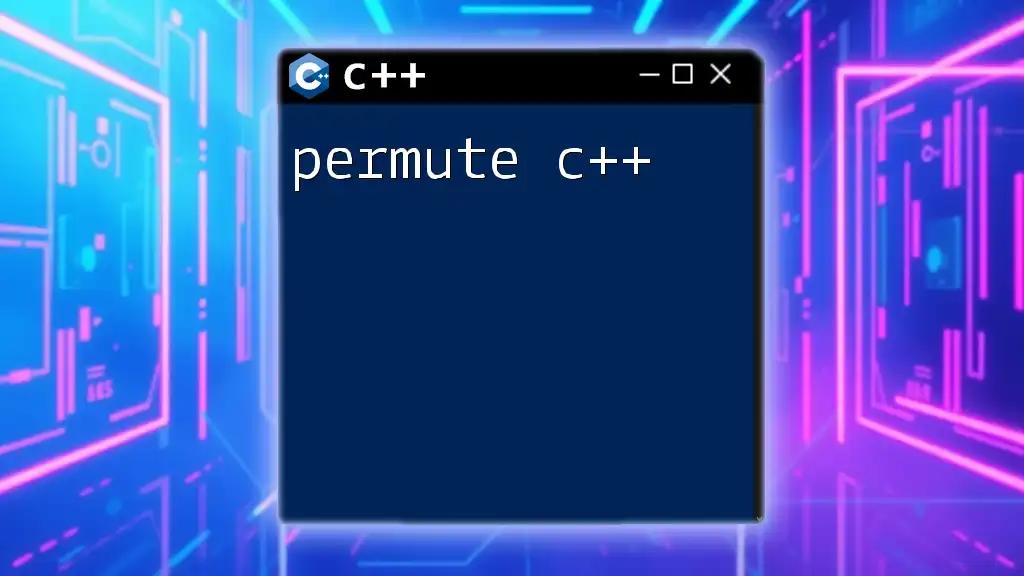
Common Use Cases and Best Practices
When to Use Promises
Promises are particularly suitable in scenarios where tasks can be executed independently of the main program flow. Examples include:
- Fetching data from an external API.
- Performing heavy computations in the background while maintaining UI responsiveness.
Best Practices for Promise Management
When working with promises, consider the following tips for effective management:
- Avoid Blocking: Always try to retrieve values from a future using `get()` in a non-blocking way when possible to maintain program responsiveness.
- Exception Safety: Ensure proper exception handling by always using `set_exception()` on a promise when an error occurs.

Conclusion
In summary, promises in C++ provide a robust mechanism for managing asynchronous operations. Their ability to simplify error handling, improve code structure, and facilitate concurrency makes them an essential tool for modern C++ programmers. As you delve further into asynchronous programming, consider exploring advanced patterns and libraries that leverage the power of promises for even greater flexibility and ease of use in your applications.

Code Repository and Examples
For practical implementations, you can access a GitHub repository filled with comprehensive code examples discussed in this article. By experimenting with these samples, you'll gain a deeper understanding of how to utilize promises effectively in your projects.