Express C++ refers to a concise approach to coding in C++, emphasizing clarity and efficiency in command usage. Here's a simple example demonstrating the declaration and initialization of a variable:
int main() {
int num = 42; // Declare and initialize an integer variable
return 0;
}
What is Express C++?
Express C++ is a modern framework designed to simplify C++ development, particularly for building web applications and services. It streamlines the syntax and provides developers with an intuitive interface to handle common web tasks, making C++ more accessible and efficient for web-based projects. As the demand for C++ continues to grow in various sectors, the significance of Express C++ lies in its ability to shorten development time and improve code readability.
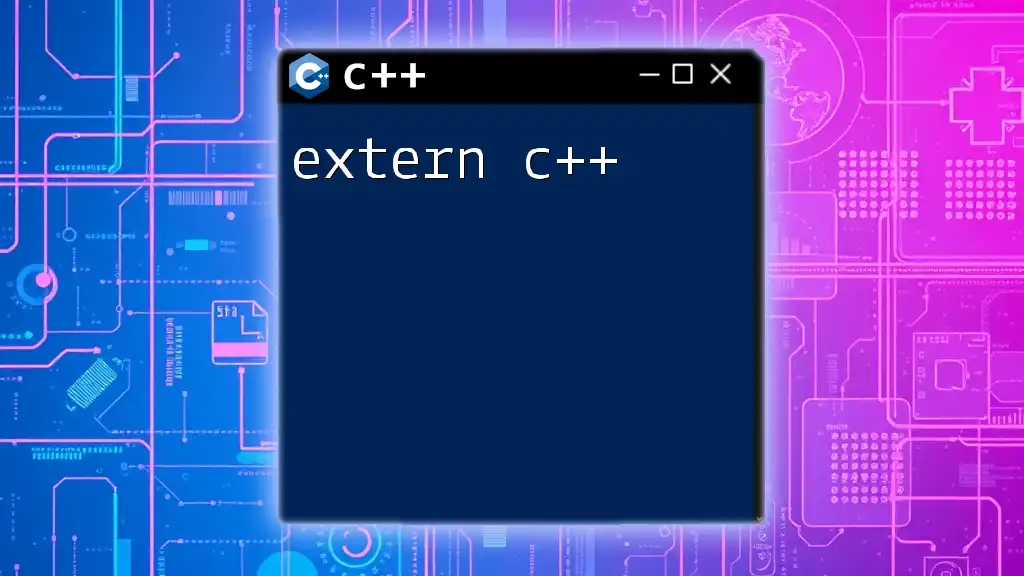
Why Use Express C++?
Using Express C++ offers several decisive advantages:
- Simplifying C++ Syntax: The framework introduces a more straightforward syntax that minimizes complexity, allowing developers to focus on functionality rather than syntax errors.
- Enhancing Code Readability: Code written in Express C++ is generally clearer and easier to understand, which is crucial for collaboration and maintenance in larger projects.
- Speeding Up Development: With built-in functionalities and a clear structure, Express C++ expedites the development process, enabling developers to build robust applications quickly.
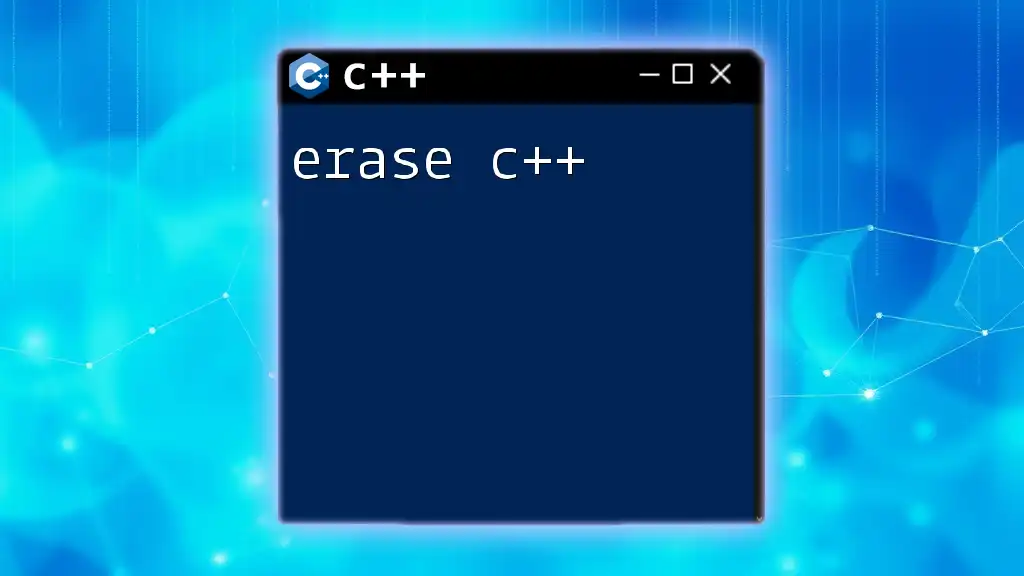
Getting Started with Express C++
Setup Requirements
To begin using Express C++, you need a few essential tools and libraries. Ensure you have the following:
- A C++ compiler (like g++ or clang++)
- The Express C++ library, which can be downloaded from its official repository.
Creating Your First Express C++ Project
Follow these steps to create a simple Express C++ project:
- Create a Project Directory: Begin by setting up a new directory for your project.
- Install Express C++: Download and include the library files in your project.
- Write Your First Code: Here’s a minimalistic example demonstrating the basic structure of an Express C++ application:
#include <express/express.hpp> int main() { express::app app; app.get("/", [](const express::request& req, express::response& res) { res.send("Hello World!"); }); app.run(3000); }
This example sets up a basic server that responds with "Hello World!" when the root URL ("/") is accessed.
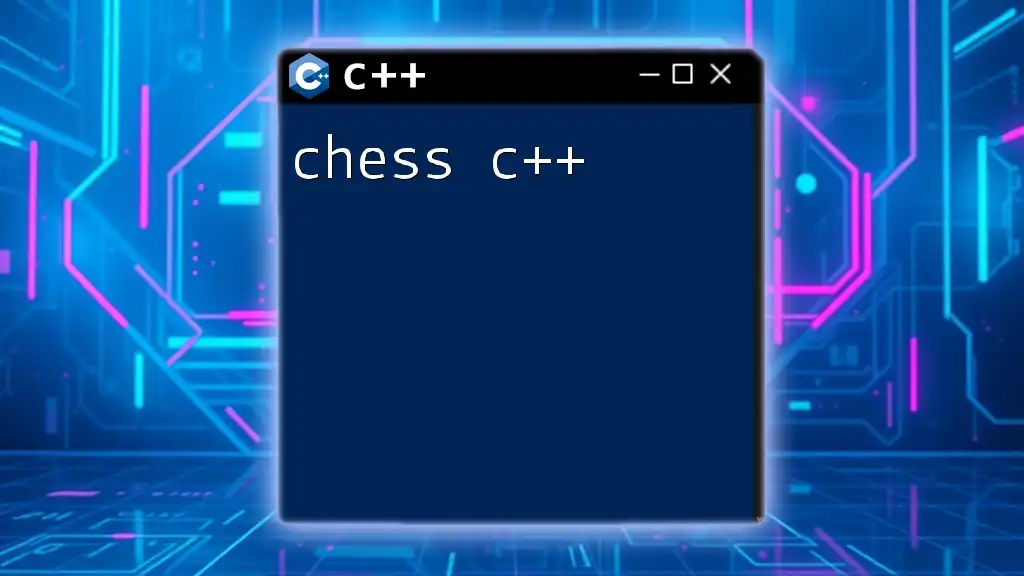
Express C++ Features
Streamlined Syntax
One of the most notable aspects of Express C++ is its streamlined syntax, which reduces boilerplate code typically associated with standard C++. For instance, where standard C++ might require verbose definitions and function declarations, Express C++ allows for a more concise representation.
Chaining Commands
Express C++ allows developers to chain commands seamlessly, leading to cleaner code. For example:
express::app app;
app.get("/hello", [](auto req, auto res) {
res.send("Hello, Express C++!");
}).post("/submit", [](auto req, auto res) {
res.send("Form Submitted!");
}).run();
In this snippet, we define two routes, one for handling GET requests and another for POST requests, all in a single chain of commands.
Middleware Support
Middleware plays a crucial role in web frameworks, and Express C++ excels in this area. Middleware functions can process requests before they reach the main handler. Here’s a simple middleware example:
void logger(express::request& req, express::response& res, const express::next& next) {
std::cout << req.method() << " " << req.path() << std::endl;
next();
}
app.use(logger);
This middleware logs the HTTP method and path of incoming requests before passing control to the next handler.
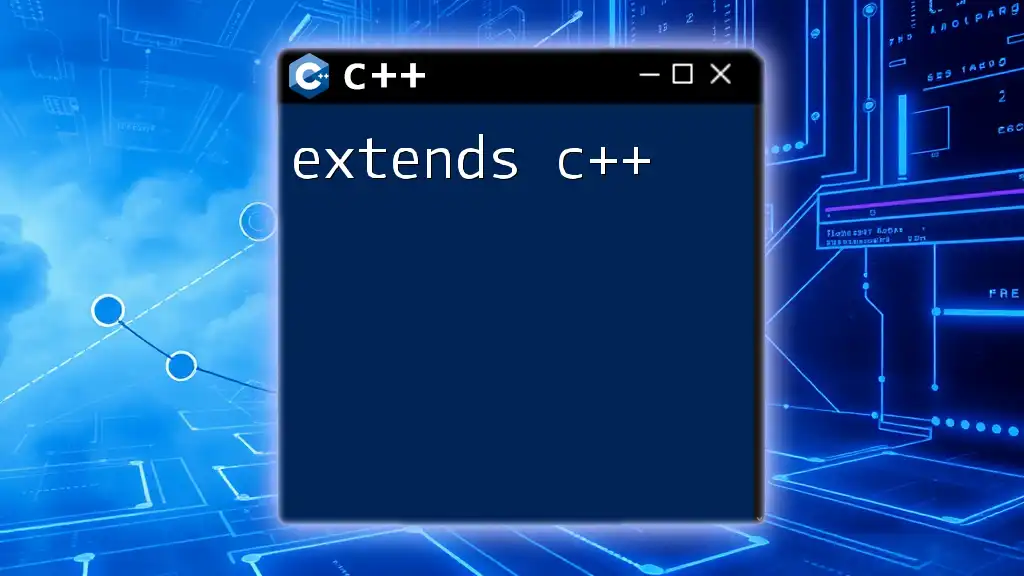
Routing in Express C++
Understanding Routing
Routing is a critical element in web development, as it determines how requests are handled based on the URL and HTTP method. Express C++ provides a straightforward approach to defining routes.
Defining Routes
In Express C++, you can define routes in a simple and clear manner. For instance:
app.get("/users/:id", [](auto req, auto res) {
auto userId = req.params["id"];
res.send("User ID: " + userId);
});
This route captures dynamic parameters, allowing you to handle requests for specific users easily.
Dynamic Routing
Dynamic routing enhances the framework’s capability, enabling developers to create more flexible routes. Here’s an example:
app.get("/products/:category/:id", [](auto req, auto res) {
auto category = req.params["category"];
auto productId = req.params["id"];
res.send("Category: " + category + ", Product ID: " + productId);
});
This code snippet demonstrates how to handle requests that contain dynamic segments, providing valuable data to the response.
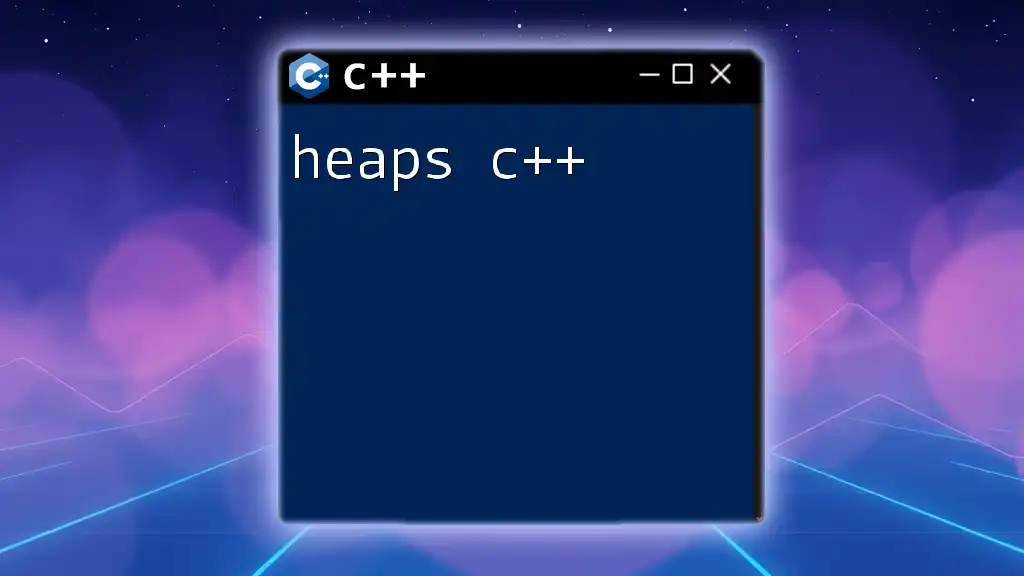
Error Handling in Express C++
Importance of Error Handling
Effective error handling is essential in any web application, ensuring a smooth user experience even when issues arise. Express C++ incorporates robust error handling mechanisms.
Implementing Error Handling
Implementing error handling in Express C++ can be done using middleware. For example:
app.use([](const express::request& req, express::response& res, const express::next& next) {
try {
next();
} catch (const std::exception& e) {
res.status(500).send(e.what());
}
});
In this snippet, the middleware catches exceptions and returns an HTTP 500 status code along with the error message, allowing the developer to handle unforeseen issues gracefully.
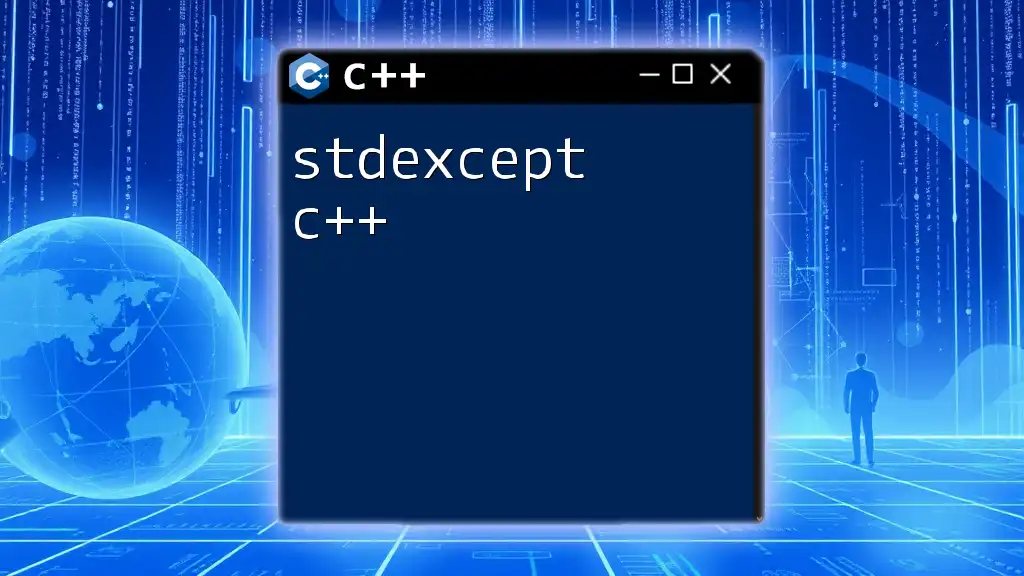
Testing Your Express C++ Application
Unit Testing Basics
Testing is crucial for ensuring the reliability and quality of your application. It helps catch bugs early in the development process.
Setting Up Testing Framework
To effectively test your Express C++ application, consider using frameworks like Catch2 or Google Test that integrate well with C++ projects.
Writing Test Cases
Here’s an example of how to write a simple test case:
TEST_CASE("GET /") {
auto res = request.get("/");
CHECK(res.status() == 200);
CHECK(res.body() == "Hello World!");
}
This test checks whether the root URL successfully returns a 200 status and the correct response body.
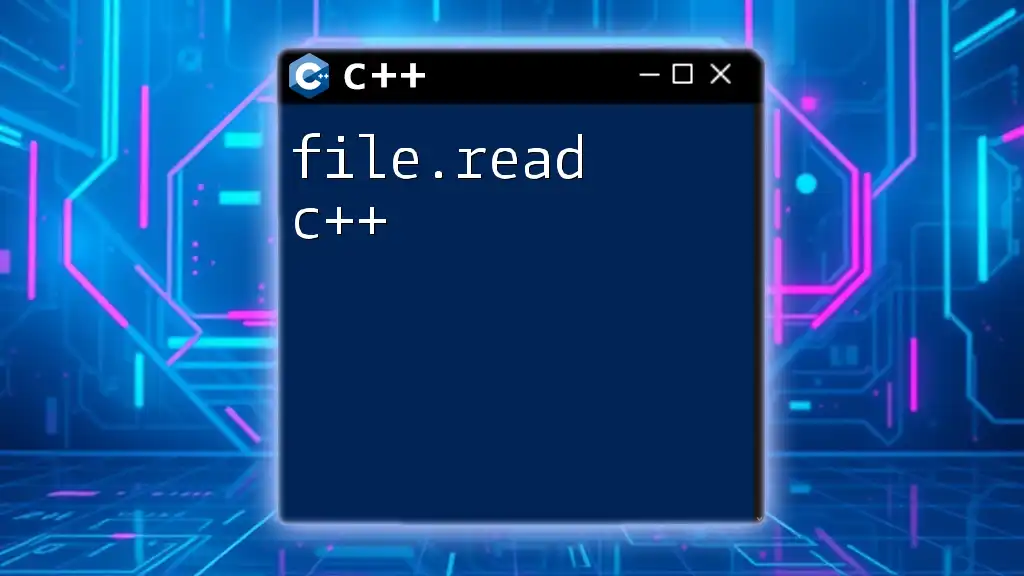
Best Practices for Express C++ Development
Code Organization
Keeping your code modular and organized is crucial for maintaining a clean codebase. Structure your files and directories logically to separate concerns, making your project easier to navigate and manage.
Performance Optimizations
To ensure optimal performance, consider using asynchronous programming techniques, caching frequently accessed resources, and regularly profiling your application to identify bottlenecks.
Security Considerations
Web applications can be exposed to various vulnerabilities. Familiarize yourself with common security threats such as SQL injection and cross-site scripting (XSS), and implement measures like input validation, output encoding, and secure authentication mechanisms to protect your application.
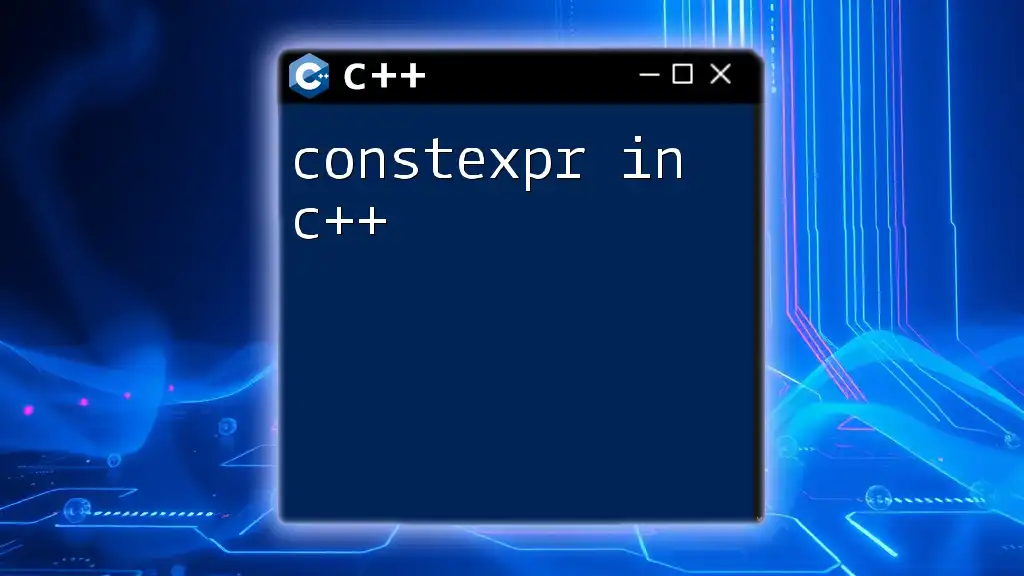
Conclusion
Express C++ serves as a powerful tool for C++ developers, offering a simplified syntax and robust features for building web applications. With its emphasis on ease of use, efficient routing, and liberal middleware support, Express C++ makes web development in C++ accessible and efficient.
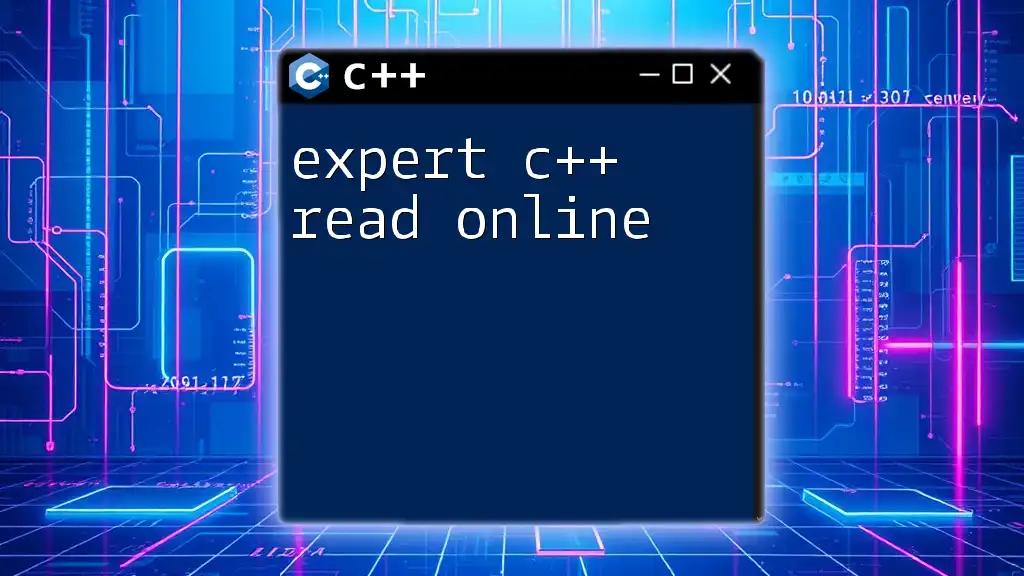
Additional Resources
For further exploration of Express C++, consider consulting the official documentation, engaging with community forums, or enrolling in specialized courses aimed at deepening your understanding of this remarkable framework.